Analyzing Numerical Sequences with Python: A Flask Application

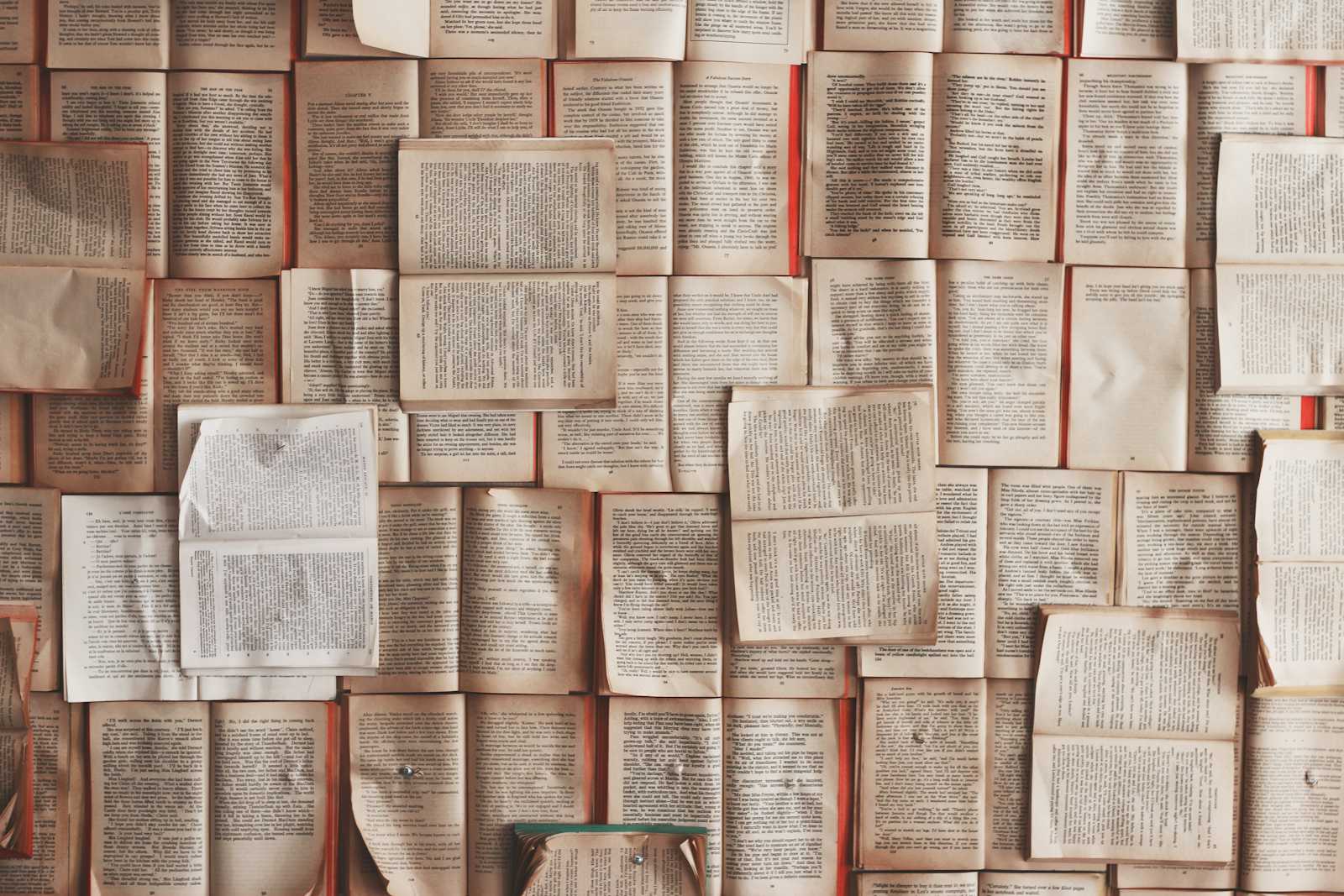
Understanding numerical sequences is essential in fields like mathematics, data science, and machine learning. Sequences help identify patterns, predict future data points, and uncover relationships between datasets. In this blog post, I'll guide you through building a Flask application that helps users analyze numerical sequences using various mathematical fitting techniques. This application allows for polynomial interpolation, exponential fitting, custom fitting, and factorial fitting to derive formulas from sequences. Let's dive in!
Getting Started
Prerequisites
Before we proceed, ensure you have the following libraries installed:
Flask: A lightweight WSGI web application framework for Python.
NumPy: A library for numerical computations.
SymPy: A Python library for symbolic mathematics.
Install these libraries using pip:
pip install Flask numpy sympy
Application Overview
Here's how the application is structured:
User Input: Users input a sequence of integers.
Mathematical Fitting: The app applies various fitting algorithms to analyze the sequence.
Output: The derived formulas are displayed on a webpage.
How It Works
1. The Flask Application Setup
To get started, create a basic Flask application that will handle user input and display the results. We’ll define a route that will manage both the input and output.
from flask import Flask, render_template, request, session, flash
import sympy as sp
from logic import polynomial_interpolation, find_exponential_fit, find_custom_fit, find_factorial_fit
app = Flask(__name__)
app.secret_key = 'your_secret_key'
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
sequence = request.form.get('sequence')
# Convert sequence into integers and calculate x, y values
sequence = list(map(int, sequence.split()))
x_values = list(range(1, len(sequence) + 1))
y_values = sequence
# Perform polynomial interpolation and other fitting techniques
# Store the formulas or outputs in a variable to be used later in the template
return render_template('index.html', formulas=formulas, sequence=sequence)
return render_template('index.html')
2. Polynomial Interpolation
Polynomial interpolation finds the polynomial of the lowest degree that passes through all points provided by the user’s input sequence.
def polynomial_interpolation(x_values, y_values, degree=None):
# Basic implementation using NumPy's polyfit
coefficients = np.polyfit(x_values, y_values, degree)
return np.poly1d(coefficients)
3. Exponential and Custom Fits
The application also performs exponential fitting and custom formula generation.
def find_exponential_fit(x_values, y_values):
# Perform logarithmic transformation and linear regression
log_y_values = np.log(y_values)
coefficients = np.polyfit(x_values, log_y_values, 1)
return sp.exp(coefficients[1]) * sp.exp(coefficients[0] * sp.symbols('x'))
def find_custom_fit(x_values, y_values):
# A custom fitting function that uses symbolic computation
x = sp.symbols('x')
return None # Placeholder for demonstration purposes
Code Snippet to Try It Yourself
To make it easier for you to try, here’s a code snippet that you can run directly in your environment:
# Import necessary libraries
import numpy as np
import sympy as sp
from sympy import symbols, Eq, solve
def polynomial_interpolation(x_values, y_values, degree=None):
# Fit a polynomial to the given data points
coefficients = np.polyfit(x_values, y_values, degree)
return np.poly1d(coefficients)
def find_exponential_fit(x_values, y_values):
# Perform exponential fitting
log_y_values = np.log(y_values)
coefficients = np.polyfit(x_values, log_y_values, 1)
return sp.exp(coefficients[1]) * sp.exp(coefficients[0] * symbols('x'))
# Example usage
x_values = [1, 2, 3, 4, 5]
y_values = [2, 4, 8, 16, 32]
polynomial = polynomial_interpolation(x_values, y_values, degree=2)
exponential = find_exponential_fit(x_values, y_values)
print(f"Polynomial Fit: {polynomial}")
print(f"Exponential Fit: {exponential}")
Explanation of Key Functions
polynomial_interpolation
: Fits a polynomial to the input data.find_exponential_fit
: Computes an exponential fit by performing a logarithmic transformation.
Try the App!
Want to see it in action? Check out the live version of the sequence solver here. Simply enter any sequence of numbers, and the app will generate formulas that best fit the sequence!
Conclusion
This Flask application shows how Python's libraries can solve complex mathematical problems and present them in a user-friendly web format. Whether you're a math enthusiast, a student, or a data scientist, this tool can help you quickly identify patterns in numerical data.
Feel free to expand the functionality, add new types of fits, or even improve the user interface. Experiment, and make it your own!
Next Steps
Enhancements: Add more fitting techniques, such as logarithmic or trigonometric fitting.
User Interface: Improve the design and user experience.
Community Contributions: Open-source the project and welcome contributions from others!
Explore, experiment, and happy coding!
Feel free to comment below with any questions or suggestions!
Subscribe to my newsletter
Read articles from FavourMogbonjubola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

FavourMogbonjubola
FavourMogbonjubola
I'm a passionate full-stack web developer with a strong foundation in front-end and back-end technologies. I've honed my skills in HTML, CSS, JavaScript, Python, C, and more. I’m driven by a love for problem-solving and innovation, particularly in the field of Artificial Intelligence. I believe in the power of technology to shape a better future, and I’m excited to contribute to projects that push boundaries and create meaningful impact