Exploring the React useTransition Hook: Full Guide

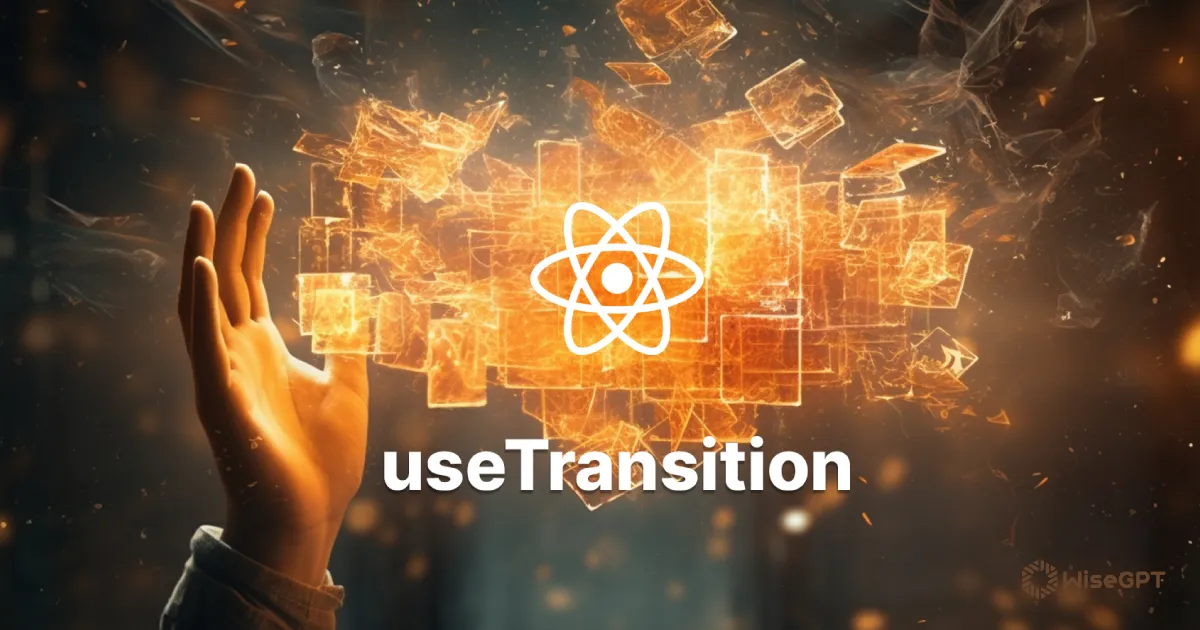
React's useTransition
hook is a powerful tool introduced in React 18 as part of its concurrent rendering features. It enables developers to handle updates with better user experience by allowing certain state transitions to be marked as less urgent. This guide explores how useTransition
works, its applications, and why it’s a valuable addition to your React toolkit.
What is the useTransition
Hook?
In typical React rendering, all state updates are treated with the same urgency, which can sometimes result in a laggy or slow user experience, especially when dealing with complex or heavy updates like re-rendering large lists or navigating between pages. The useTransition
hook helps resolve this by allowing you to label certain updates as "transitions," which React can handle with lower priority.
Syntax
The useTransition
hook returns two values:
const [isPending, startTransition] = useTransition();
isPending: A boolean value that tells you whether the transition is still in progress.
startTransition: A function used to wrap the state update you want to run as a transition.
When to Use useTransition
Consider using useTransition
when you want to:
Improve the responsiveness of your app when updating non-critical UI, like filtering data or navigating between views.
Perform expensive computations or re-render large components in a way that doesn’t block the UI.
Handle loading states better by showing a spinner or placeholder while the UI transitions.
Example: Basic Usage
Let’s look at an example where useTransition
is applied to a search filter feature. Imagine you have a list of items, and typing in a search input triggers an update that filters this list.
import { useState, useTransition } from 'react';
function SearchComponent({ items }) {
const [query, setQuery] = useState('');
const [filteredItems, setFilteredItems] = useState(items);
const [isPending, startTransition] = useTransition();
const handleSearch = (event) => {
const value = event.target.value;
setQuery(value);
// Use startTransition to make this update a transition
startTransition(() => {
const filtered = items.filter(item => item.includes(value));
setFilteredItems(filtered);
});
};
return (
<div>
<input
type="text"
value={query}
onChange={handleSearch}
placeholder="Search items..."
/>
{isPending ? <p>Loading...</p> : <ItemList items={filteredItems} />}
</div>
);
}
In this example:
The
handleSearch
function updates the input query as usual.The filtering operation is wrapped inside
startTransition()
, allowing React to prioritize the input typing over filtering the list.While the filter operation is in progress,
isPending
will betrue
, and you can display a loading message or spinner.
Real-World Use Case: Navigating Between Views
Another common use for useTransition
is navigating between views in single-page applications (SPAs). When a user clicks on a link, you can prioritize the navigation interaction and run the transition to the next view in the background.
function NavigationComponent() {
const [isPending, startTransition] = useTransition();
const handleNavigation = (newPage) => {
startTransition(() => {
// Simulate loading new content
setCurrentPage(newPage);
});
};
return (
<div>
<nav>
<button onClick={() => handleNavigation('home')}>Home</button>
<button onClick={() => handleNavigation('about')}>About</button>
</nav>
{isPending ? <p>Loading...</p> : <PageContent />}
</div>
);
}
In this case, clicking a button triggers a navigation action that React can process as a transition, while keeping the interface responsive.
Benefits of useTransition
Improved User Experience: By deferring less critical updates,
useTransition
ensures the app stays responsive, even during heavy computations.Smoother UI Transitions: Complex UI updates can be performed in the background without blocking user interactions.
Easy to Implement:
useTransition
is simple to use and integrate into existing React code.
Common Pitfalls
Overusing
useTransition
: It should be used for non-urgent updates only. Don’t wrap critical state updates like form submissions or data fetches inuseTransition
, as it could lead to unintended delays.UI Flickering: In some cases, unnecessary re-renders might cause flickering or inconsistencies. Ensure that transitions only affect non-critical parts of the UI.
Conclusion
The useTransition
hook is a valuable tool for optimizing the performance and user experience of React applications. By deferring expensive updates and prioritizing essential interactions, you can create smoother and more responsive UIs. Whether you're dealing with filtering data, navigating between pages, or handling heavy computations, useTransition
allows you to manage these tasks more effectively.
Subscribe to my newsletter
Read articles from Prem Prakash Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
