Mastering Node.js Buffers: A Deep Dive with Practical Applications

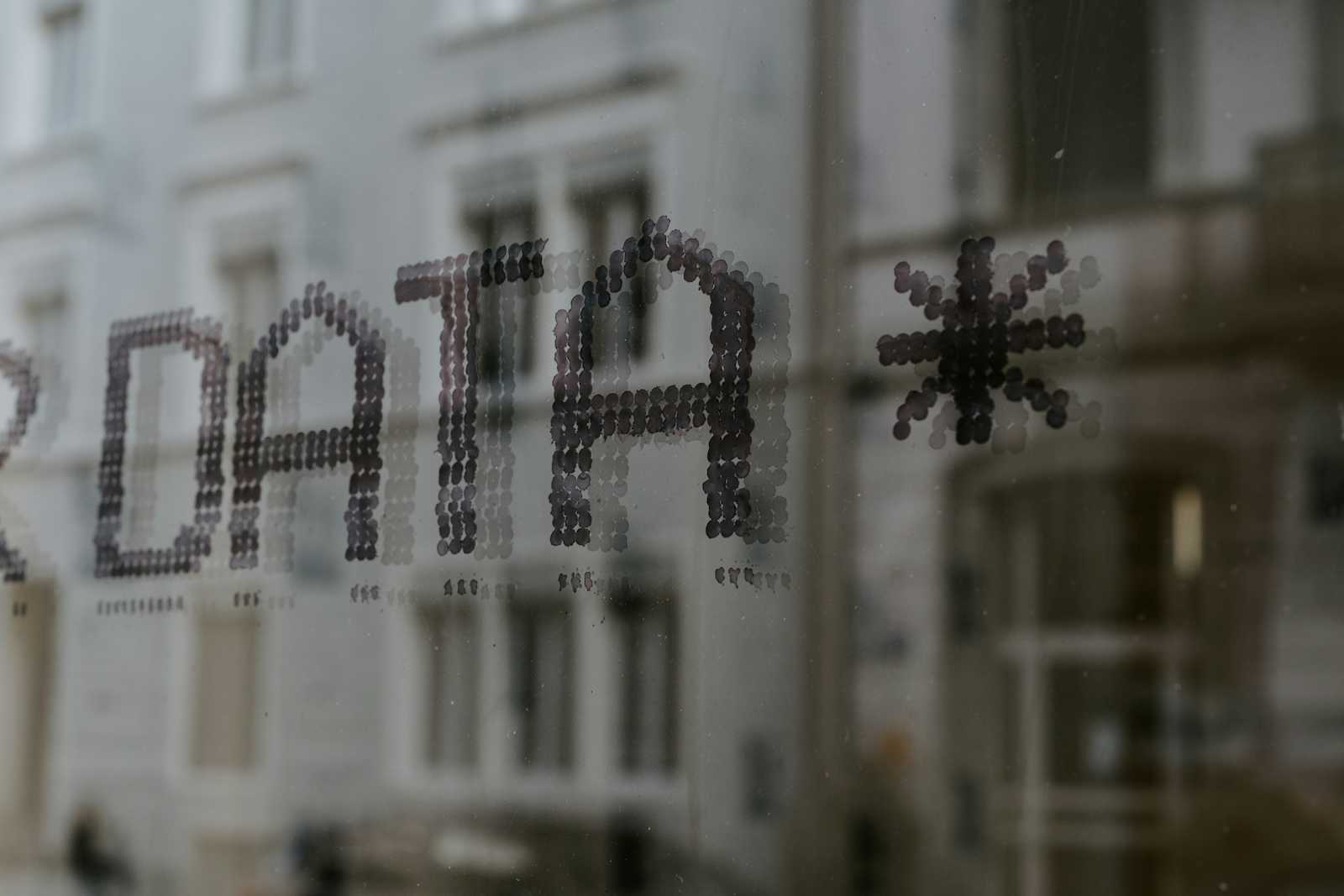
Introduction
Buffer objects in Node.js are crucial for working with raw binary data, especially in scenarios involving file I/O, network communication, or interfacing with external systems. To handle buffers effectively, it is imperative to have a strong grasp of how data is represented at a low level, including binary, hexadecimal numbers, and byte order (endianness).
This comprehensive guide will start by thoroughly exploring foundational concepts before delving into buffers, thoroughly examining each key method with supporting examples. Finally, it will comprehensively explore real-world buffer usage across various applications.
Prerequisites: Understanding Binary, Hexadecimal, and Endianness
Binary Numbers
Binary is the most basic form of data representation in computers, using two digits: 0 and 1.
- Example: The binary number
1101
represents 1×2^3 + 1×2^2 + 0×2^1 + 1×2^0, which equals 8+4+0+1=13.
Hexadecimal Numbers
Hexadecimal, or hex, is a base-16 system that uses the digits 0-9 and letters A-F to represent values. It’s often used to represent binary data because it’s more compact.
- Example: The hex number
0x1A3F
represents 1×16^3 + A×16^2 + 3×16^1 + F×16^0, which equals 4096+2560+48+15=6719.
Big Endian vs Little Endian
Endianness determines how data is ordered in memory:
Big Endian: The most significant byte (MSB) is stored first (e.g.,
0x12345678
becomes12 34 56 78
).Little Endian: The least significant byte (LSB) is stored first (e.g.,
0x12345678
becomes78 56 34 12
).
In Node.js, you can specify endianness when reading or writing numbers.
const buffer = Buffer.alloc(4);
// Big Endian (most significant byte first)
buffer.writeUInt32BE(123456789, 0);
console.log(buffer.readUInt32BE(0)); // Outputs: 123456789
// Little Endian (least significant byte first)
buffer.writeUInt32LE(123456789, 0);
console.log(buffer.readUInt32LE(0)); // Outputs: 123456789
What is a Buffer in Node.js?
A Buffer in Node.js is a region of memory designed to store raw binary data. Buffers are especially useful when data needs to be manipulated at the byte level, such as file I/O, working with streams, or handling binary data from networks.
Basic Example: Creating a Buffer
const buf = Buffer.from('Hello, World!');
console.log(buf);
// Outputs: <Buffer 48 65 6c 6c 6f 2c 20 57 6f 72 6c 64 21>
console.log(buf.toString());
// Outputs: Hello, World!
Here, the string 'Hello, World!'
is stored as a buffer containing its raw bytes. When logged, it’s displayed in hexadecimal format, and toString()
is used to convert it back into a readable string.
Buffer Methods with Examples
Let's dive into some common methods provided by the Buffer
class, along with practical examples.
1. Buffer.alloc(size)
Allocates a new buffer of a given size. The buffer is initialised to zeros.
const buf = Buffer.alloc(10); // Creates a buffer of 10 bytes
console.log(buf);
// Outputs: <Buffer 00 00 00 00 00 00 00 00 00 00>
Usage: When you need a buffer of a fixed size but haven't filled it with data yet. This is safer than using allocUnsafe
as it ensures the buffer is cleared.
2. Buffer.allocUnsafe(size)
Allocates a buffer without clearing the memory. It’s faster than Buffer.alloc
but can contain old data.
const buf = Buffer.allocUnsafe(10);
// Creates an uninitialized buffer of 10 bytes
console.log(buf);
// Outputs: <Buffer ...random values...>
Usage: Use when performance is critical, and you will immediately fill the buffer, making initialization unnecessary.
3. Buffer.from(data, [encoding])
Creates a buffer from existing data such as a string, array, or another buffer.
const buf = Buffer.from('Hello'); // From a string
console.log(buf);
// Outputs: <Buffer 48 65 6c 6c 6f>
const arrBuf = Buffer.from([0x48, 0x65, 0x6c, 0x6c, 0x6f]); // From an array of bytes
console.log(arrBuf.toString());
// Outputs: Hello
Usage: When converting data (e.g., strings or arrays) into a buffer for low-level manipulation.
4. buf.write(string, [offset], [length], [encoding])
Writes a string to the buffer starting at a given offset.
const buf = Buffer.alloc(10);
buf.write('Hello', 0, 'utf8');
console.log(buf);
// Outputs: <Buffer 48 65 6c 6c 6f 00 00 00 00 00>
Usage: When you need to write a string into an existing buffer at a specific position.
5. buf.slice([start], [end])
Returns a new buffer that references the same memory but is sliced from the specified start and end indices.
const buf = Buffer.from('Hello, World!');
const slicedBuf = buf.slice(0, 5);
console.log(slicedBuf.toString());
// Outputs: Hello
Usage: Useful for extracting a portion of a buffer without copying data, improving performance.
6. buf.readUInt32BE(offset)
Reads a 32-bit unsigned integer from the buffer using Big Endian byte order.
const buf = Buffer.alloc(4);
buf.writeUInt32BE(12345678, 0);
console.log(buf.readUInt32BE(0));
// Outputs: 12345678
Usage: When reading large integers from buffers that follow network byte order (Big Endian).
7. buf.writeUInt32BE(value, offset)
Writes a 32-bit unsigned integer into the buffer using Big Endian byte order.
const buf = Buffer.alloc(4);
buf.writeUInt32BE(987654321, 0);
console.log(buf);
// Outputs: <Buffer 3a de 68 b1>
Usage: When you need to write integers in a format compatible with network protocols or other systems using Big Endian.
8. buf.copy(targetBuffer, targetStart, sourceStart, sourceEnd)
Copies data from one buffer to another.
const source = Buffer.from('Hello');
const target = Buffer.alloc(5);
source.copy(target, 0, 0, 5); // Copy 'Hello' into target buffer
console.log(target.toString());
// Outputs: Hello
Usage: When you need to copy data between buffers, such as concatenating or reusing parts of a buffer.
Alex’s Problem: Solving Real-World Binary Data Issues
Alex, a developer working with network protocols, had to process binary data that included text and numeric fields encoded using Big Endian byte order. Reading this data as strings led to corrupted results, and traditional JavaScript types weren’t cutting it.
Using Node.js Buffers, Alex was able to read and write the exact bytes needed, solving the issue.
Step 1: Reading Data Using Buffers
const fs = require('fs');
fs.readFile('data.bin', (err, data) => {
if (err) throw err;
// First 10 bytes as UTF-8 string
const text = data.slice(0, 10).toString('utf8');
// Next 4 bytes as a 32-bit unsigned integer (Big Endian)
const number = data.readUInt32BE(10);
console.log('Text:', text); // Outputs: 'SomeText'
console.log('Number:', number); // Outputs: 123456
});
Step 2: Writing Data Back Using Buffers
const buffer = Buffer.alloc(14);
// 10 bytes for text, 4 bytes for integer
buffer.write('Processed', 0, 'utf8'); // Write string at the start
buffer.writeUInt32BE(654321, 10);
// Write 32-bit number starting at byte 10
fs.writeFile('output.bin', buffer, (err) => {
if (err) throw err;
console.log('Data written successfully!');
});
Real-World Usage of Buffers
Buffers are essential in many real-world scenarios, including:
1. File I/O Operations
When reading or writing files in binary format, such as images, videos, or raw data, buffers allow you to handle byte-level manipulation.
2. Network Communication
In network protocols (TCP/UDP), data is often transmitted as raw bytes. Buffers allow developers to encode and decode data packets in the correct format.
3. Streaming Media
When working with media streams (audio/video), buffers are used to temporarily store chunks of data before it is processed.
4. Interfacing with Hardware
In IoT and embedded systems, buffers are used to communicate with hardware devices that require low-level byte streams.
5. Cryptography
Buffers are often used to store and manipulate binary data for encryption and decryption algorithms.
Subscribe to my newsletter
Read articles from Zeeshan Ashraf directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zeeshan Ashraf
Zeeshan Ashraf
I’m an aspiring engineer, tech-savvy, and a pragmatic developer wandering around the tech fields to bring creativity to life. My name is Zeeshan Ashraf, and this is my inspiration for technology. My Aim is to be a one-of-a-kind leader who can inspire others, promote innovation and creativity, and develop leading technology. To do this, I constantly ask questions, challenge traditional thinking, imagine endless possibilities and never stop learning from the greats!