How to Trigger a Function When an Elementor Popup Hides with jQuery
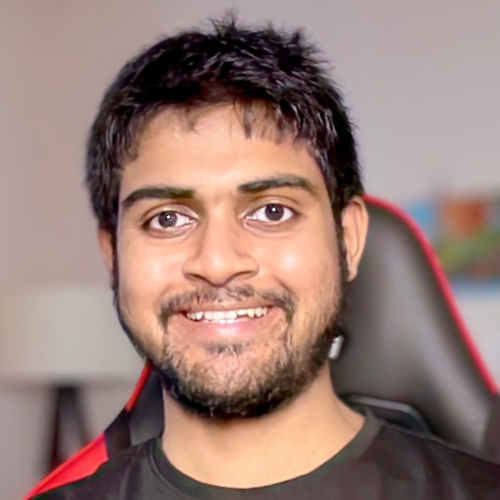
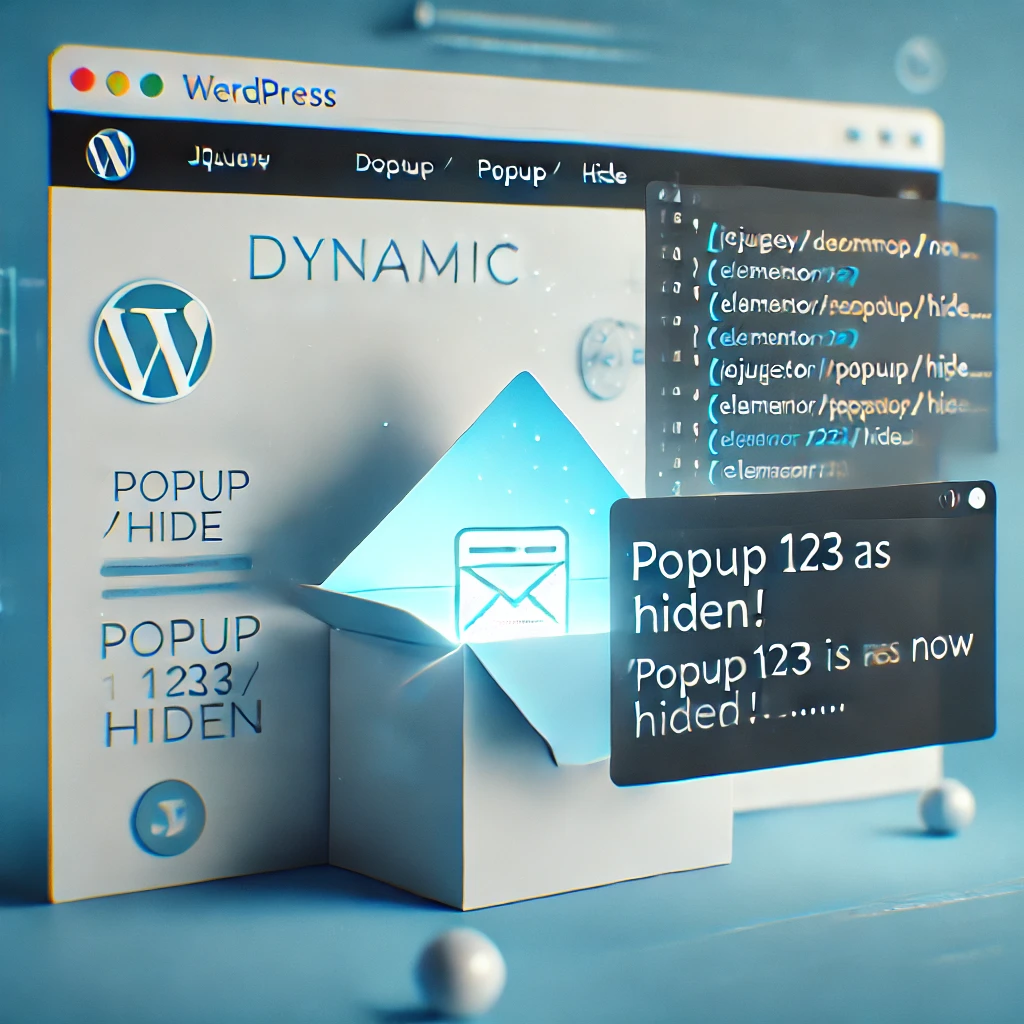
If you're building a website with Elementor and need to perform actions when an Elementor popup hides, you can easily do this using jQuery. Elementor provides useful event hooks for popups, such as elementor/popup/show
and elementor/popup/hide
. In this blog, we will focus on how to trigger an action when a specific popup hides.
Why Use Elementor Popup Events?
When you're developing custom functionality for your WordPress website, there are times when you'll want to track user interactions with popups. For example, you may want to:
Trigger analytics tracking when a popup closes.
Automatically hide certain page elements when the popup is dismissed.
Save user data, such as form submissions, after the popup closes.
Elementor’s elementor/popup/hide
event helps you achieve this by providing a way to detect when a popup is hidden, allowing you to perform your custom actions seamlessly.
How to Trigger an Action When a Popup Hides
The Code
Here’s a simple jQuery snippet that listens for the elementor/popup/hide
event and triggers a function when a specific popup (with ID 123) hides.
jQuery(document).on('elementor/popup/hide', (event, id, instance) => {
if (id === 123) {
// Your custom action here
console.log("Popup 123 is now hidden!");
}
});
Explanation
Let’s break down the code:
jQuery(document).on('elementor/popup/hide', (event, id, instance) => { ... });
This line listens for theelementor/popup/hide
event. It triggers a callback function whenever any Elementor popup is hidden.event
: The event object.id
: The ID of the popup that is being hidden.instance
: The popup instance object.Inside the callback function, we check if the
id
is equal to123
(you should replace this with your actual popup ID). If it matches, you can run your custom action, such as tracking user behavior, displaying a message, or logging the popup closure in your console.
How to Find the Popup ID
Before using the above code, you'll need to know the ID of your popup. Here’s how you can find it:
Open the Elementor editor for your page.
Click on the popup settings.
You’ll see the popup ID in the URL bar of your browser, or you can inspect the popup in the page’s source code by searching for
data-elementor-id
.
Example Use Cases
Here are some common use cases for triggering functions when the popup hides:
1. Track Analytics
If you want to track how users interact with popups on your site, you can insert tracking code inside the if
block:
if (id === 123) {
// Trigger Google Analytics event
gtag('event', 'PopupClosed', {
'event_category': 'Popups',
'event_label': 'Popup 123 Closed'
});
}
2. Redirect Users After Popup Close
You can automatically redirect users to another page after the popup closes:
if (id === 123) {
// Redirect to a thank-you page
window.location.href = 'https://yourwebsite.com/thank-you';
}
3. Show a Notification After Closing
You might want to display a success message or another notification after the user dismisses the popup:
if (id === 123) {
// Show a custom message
alert("Thank you for checking out the popup!");
}
Conclusion
With Elementor's elementor/popup/hide
event and a little bit of jQuery, you can easily extend the functionality of your WordPress website to react when popups are hidden. This simple yet powerful feature allows you to improve user interaction and make your website more dynamic.
I hope this tutorial helps you in creating amazing user experiences on your website. If you have any questions, feel free to leave a comment below!
Happy coding!
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
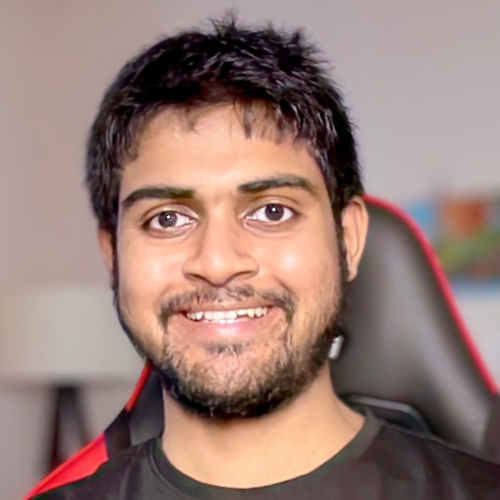
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.