Understanding Immediately Invoked Function Expressions (IIFE) in JavaScript
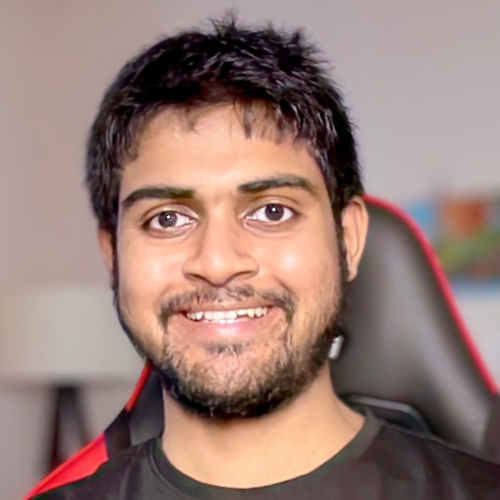
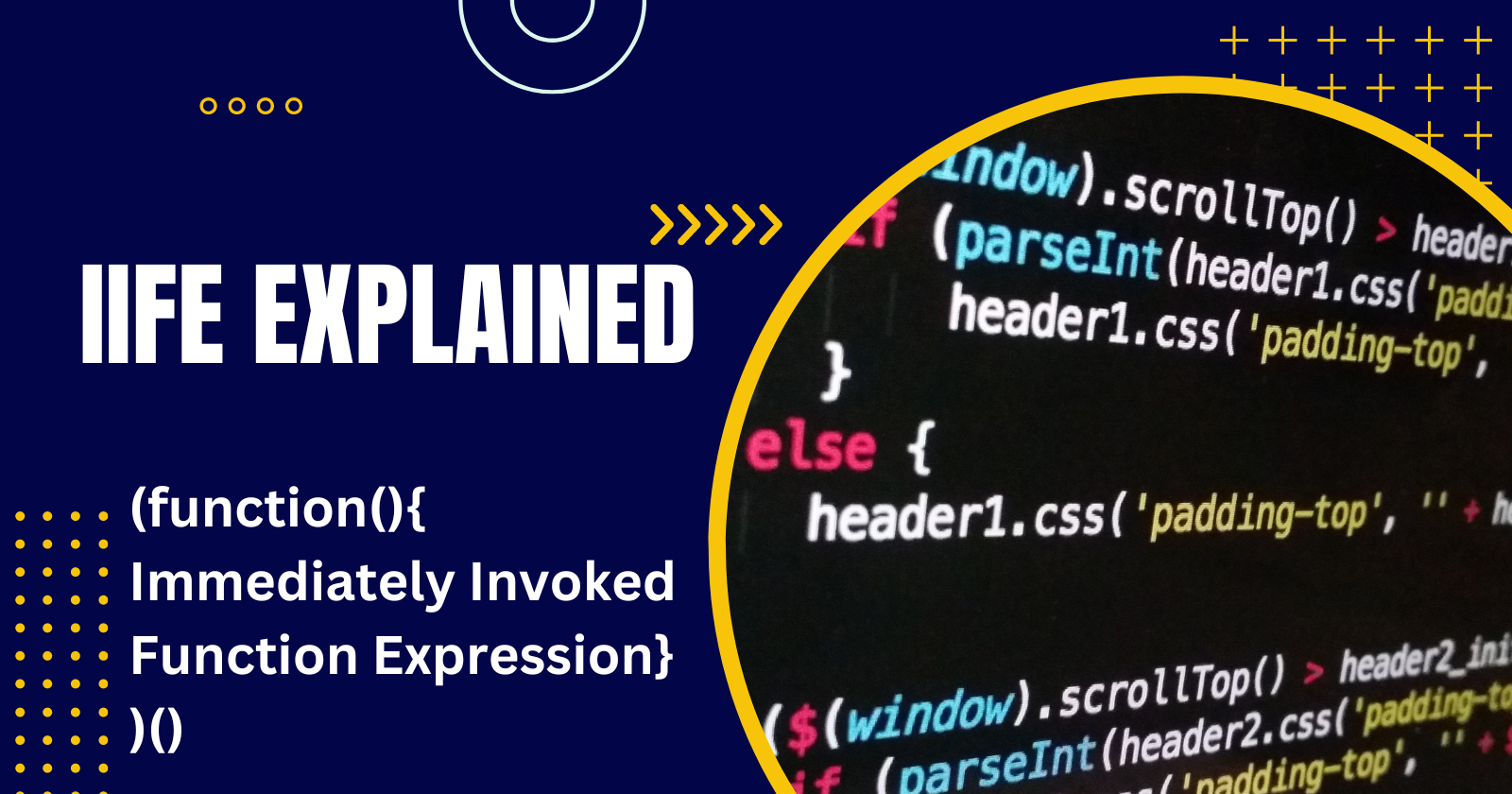
In JavaScript, one of the powerful features is the Immediately Invoked Function Expression, often abbreviated as IIFE. This pattern is widely used to maintain clean code, control variable scope, and prevent global namespace pollution. In this article, we’ll explore what IIFE is, how it works, and where you can use it in your projects.
What is an IIFE?
An Immediately Invoked Function Expression (IIFE) is a JavaScript function that runs as soon as it is defined. The function is wrapped in parentheses and is immediately executed. This technique is useful for creating local scopes and isolating code.
The basic structure of an IIFE looks like this:
(function() {
// Code here is executed immediately
console.log('This is an IIFE');
})();
How IIFE Works
Let’s break down what’s happening:
(function() {...})
: The function is defined inside parentheses. This is crucial because, in JavaScript, putting a function inside parentheses turns it into an expression (instead of a declaration).()
: This immediately invokes the function.
The result is that the function runs immediately after it’s defined, without needing to be called manually elsewhere in the code.
Why Use an IIFE?
There are several reasons why developers use IIFEs in their code:
Avoid Polluting the Global Scope: Variables and functions defined inside an IIFE are scoped to the IIFE itself and are not visible outside of it. This keeps the global scope clean.
Create Private Variables: You can use IIFEs to create private variables that are not accessible from outside the function, making your code more secure and organized.
Isolate Logic: IIFEs help in isolating pieces of logic that should not interfere with other parts of your code.
IIFE Example
Here’s a simple example that demonstrates how an IIFE works:
(function() {
let message = 'Hello from IIFE!';
console.log(message);
})();
// Trying to access 'message' outside the IIFE will result in an error
console.log(message); // ReferenceError: message is not defined
Practical Use Cases of IIFE
1. Encapsulating Variables
IIFEs are often used to encapsulate variables and prevent them from being accessible globally.
let counter = (function() {
let count = 0;
return function() {
count++;
console.log(count);
};
})();
counter(); // 1
counter(); // 2
counter(); // 3
In this example, the count
variable is encapsulated inside the IIFE and can only be modified by the returned function, ensuring it isn’t accidentally changed from outside.
2. Module Pattern
IIFEs can also be used to implement the Module Pattern, which allows us to create modules with private data and public methods.
const Module = (function() {
let privateVar = 'This is private';
return {
getPrivateVar: function() {
return privateVar;
}
};
})();
console.log(Module.getPrivateVar()); // "This is private"
console.log(Module.privateVar); // undefined
In this example, privateVar
is private within the IIFE, and only the public method getPrivateVar
allows access to it.
3. Using IIFE in Loops
IIFEs can be used inside loops to create new scopes for variables. This is particularly useful when dealing with closures in asynchronous operations.
for (var i = 1; i <= 3; i++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, 1000);
})(i);
}
// Output: 1, 2, 3
Without the IIFE, all the timeouts would log 3
, as the var i
is function-scoped and doesn’t retain its value for each iteration.
Arrow Functions and IIFE
With the introduction of ES6, you can also create IIFEs using arrow functions. Here’s an example:
(() => {
console.log('Arrow Function IIFE');
})();
It works exactly the same way, with a cleaner and more modern syntax.
Conclusion
The Immediately Invoked Function Expression (IIFE) is a valuable pattern for maintaining clean and efficient JavaScript code. It helps encapsulate variables, avoid polluting the global namespace, and create self-contained modules. Whether you’re working on a small project or a large-scale application, IIFEs can keep your code organized and free of conflicts.
Next time you need to control the scope of your variables or immediately execute some logic, give IIFE a try!
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
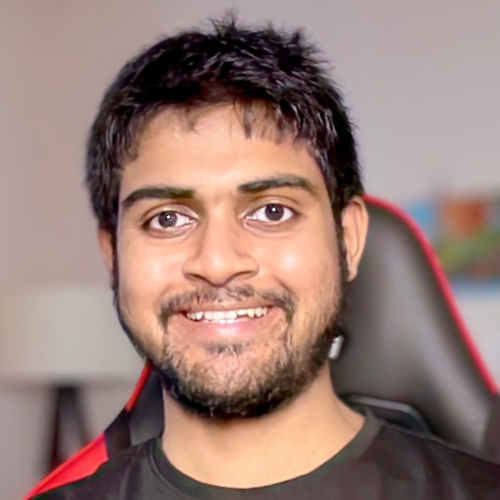
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.