Understanding the Difference Between IIFE and jQuery’s Ready Function
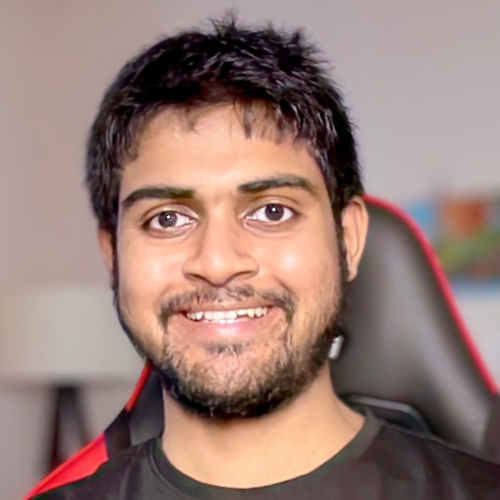
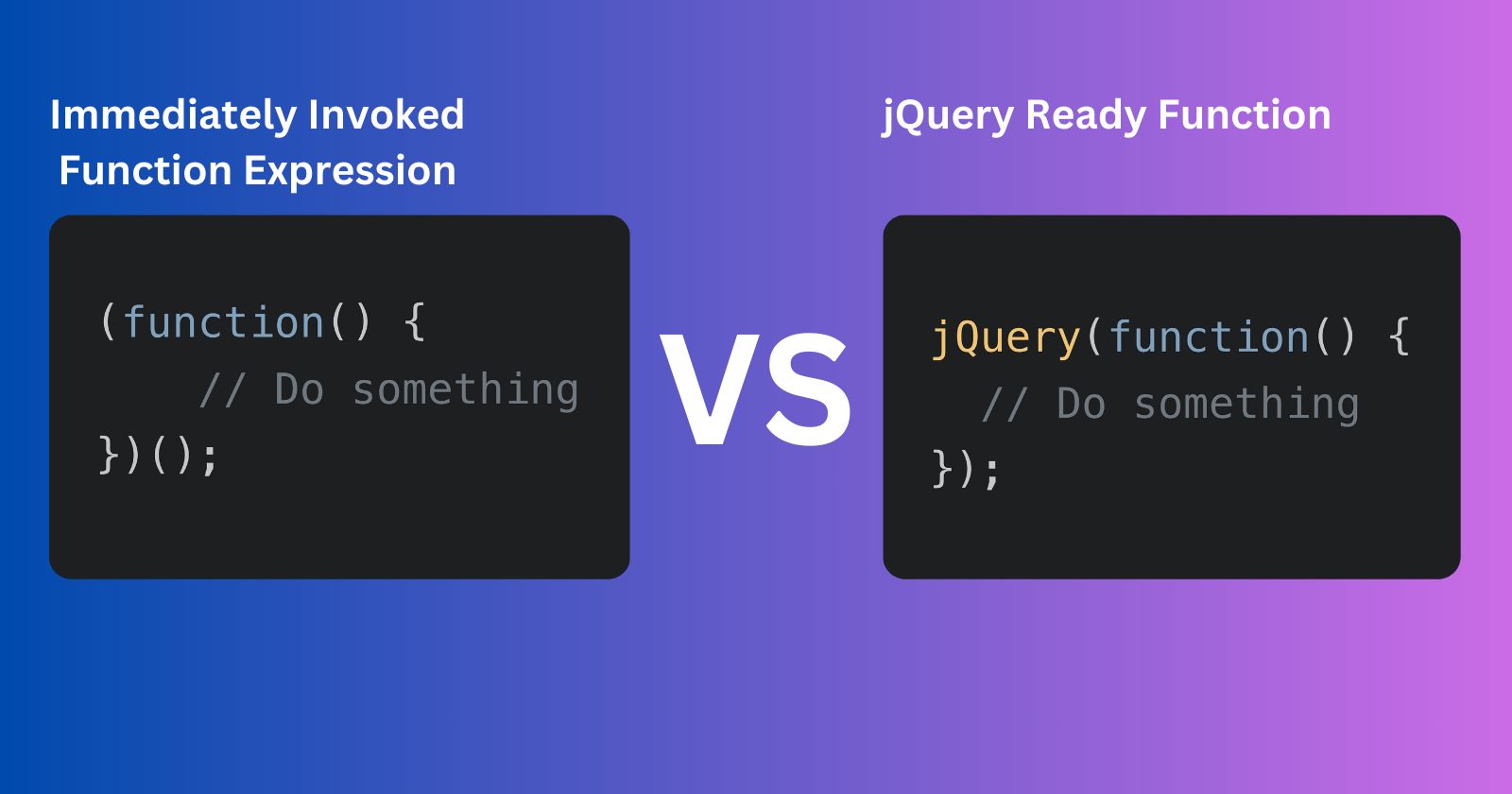
In the world of JavaScript and jQuery, it’s common to encounter different patterns for executing code. Two such patterns are the Immediately Invoked Function Expression (IIFE) and the jQuery ready function. While both may look similar in some cases, they have different purposes and are used in different contexts. In this article, we’ll explore the differences between the two, and also explain how jQuery(function(){})
is equivalent to jQuery(document).ready(function(){})
.
IIFE: The Immediately Invoked Function Expression
An IIFE is a self-executing JavaScript function. As soon as the function is defined, it is immediately invoked. The primary purpose of an IIFE is to create a private scope for your variables and avoid polluting the global scope.
Syntax of IIFE
(function() {
console.log("IIFE executed immediately");
})();
Here’s what’s happening:
The function is wrapped inside parentheses:
(function(){ ... })
.The second set of parentheses
()
at the end causes the function to be executed immediately.
This pattern is commonly used to avoid naming conflicts and to create private variables that won’t be accessible outside the function.
When to Use an IIFE
Scope isolation: IIFEs allow you to create variables and functions without polluting the global scope. This keeps your code organized and prevents unintended overwriting of global variables.
Modularity: In large applications, IIFEs are useful for keeping parts of your code modular and encapsulated.
Example:
(function() {
var message = "Hello from IIFE";
console.log(message); // Output: Hello from IIFE
})();
console.log(message); // Error: message is not defined
In this example, the variable message
is accessible only within the IIFE and cannot be accessed outside it.
jQuery’s Ready Function
On the other hand, jQuery’s ready function ensures that the code inside it runs only after the DOM is fully loaded. This is crucial when you want to manipulate HTML elements, as it ensures that the elements are available for JavaScript to interact with.
Syntax of jQuery’s Ready Function
jQuery(function() {
console.log("DOM is ready");
});
This shorthand is equivalent to:
jQuery(document).ready(function() {
console.log("DOM is ready");
});
Both of these versions do the same thing: they wait until the entire HTML document is fully loaded before running the enclosed code.
Why Use jQuery’s Ready Function?
DOM Manipulation: You often use the ready function when you need to manipulate elements (such as changing text, adding classes, or binding events) after the DOM is ready to be interacted with.
Prevent Errors: Running jQuery code too early (before the DOM is fully loaded) can lead to errors, as the elements you are trying to access may not yet exist.
Example:
jQuery(function() {
console.log("DOM is fully loaded and ready");
jQuery("h1").text("Hello, World!");
});
This code will run only after the DOM has fully loaded, ensuring that the <h1>
element exists before trying to manipulate it.
Key Differences Between IIFE and jQuery Ready Function
1. Purpose:
IIFE: Its primary purpose is to create a private scope and avoid polluting the global namespace. It executes immediately, regardless of the state of the DOM.
jQuery Ready Function: Its purpose is to ensure that the code runs only after the DOM has fully loaded. It is specific to scenarios where you want to manipulate the DOM.
2. Execution Timing:
IIFE: Runs immediately as soon as the JavaScript engine encounters it. It does not wait for the DOM to load.
jQuery Ready Function: Runs after the browser has completed loading the HTML document and the DOM is ready to be manipulated.
3. Global vs Local Scope:
IIFE: Encapsulates variables within a local scope, protecting them from being accessed globally.
jQuery Ready Function: Does not focus on scope isolation but ensures the code runs when the DOM is ready.
Understanding jQuery(function(){})
and jQuery(document).ready(function(){})
A common question arises when developers see both jQuery(function(){})
and jQuery(document).ready(function(){})
. Are they the same? The answer is yes.
jQuery(function() {
// This is the shorthand version
});
jQuery(document).ready(function() {
// This is the full version
});
Both ensure that the code inside them will only run when the DOM is fully loaded.
The shorthand
jQuery(function(){})
is just a more concise way of writingjQuery(document).ready(function(){})
.
Conclusion
The IIFE and jQuery’s ready function are both essential tools in JavaScript, but they serve different purposes:
IIFE is for scope isolation and immediate execution, making it useful for preventing global namespace pollution and encapsulating logic.
jQuery Ready Function (
jQuery(function(){})
orjQuery(document).ready(function(){})
) is used specifically for DOM manipulation after the document has fully loaded.
By understanding the differences between these patterns, you can apply them appropriately in your projects, ensuring both clean code and proper DOM manipulation timing.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
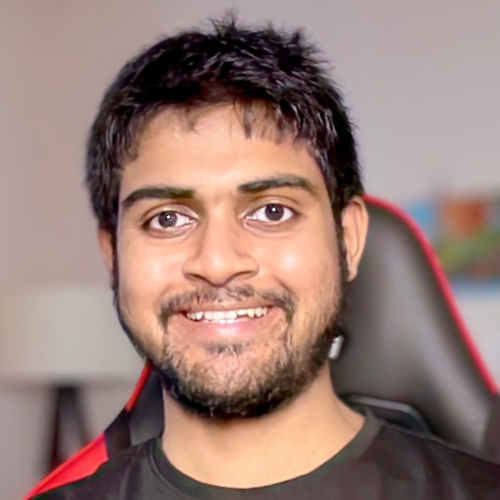
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.