Step-by-Step Guide to Setting Up API Gateway with AWS Lambda Using Python FastAPI
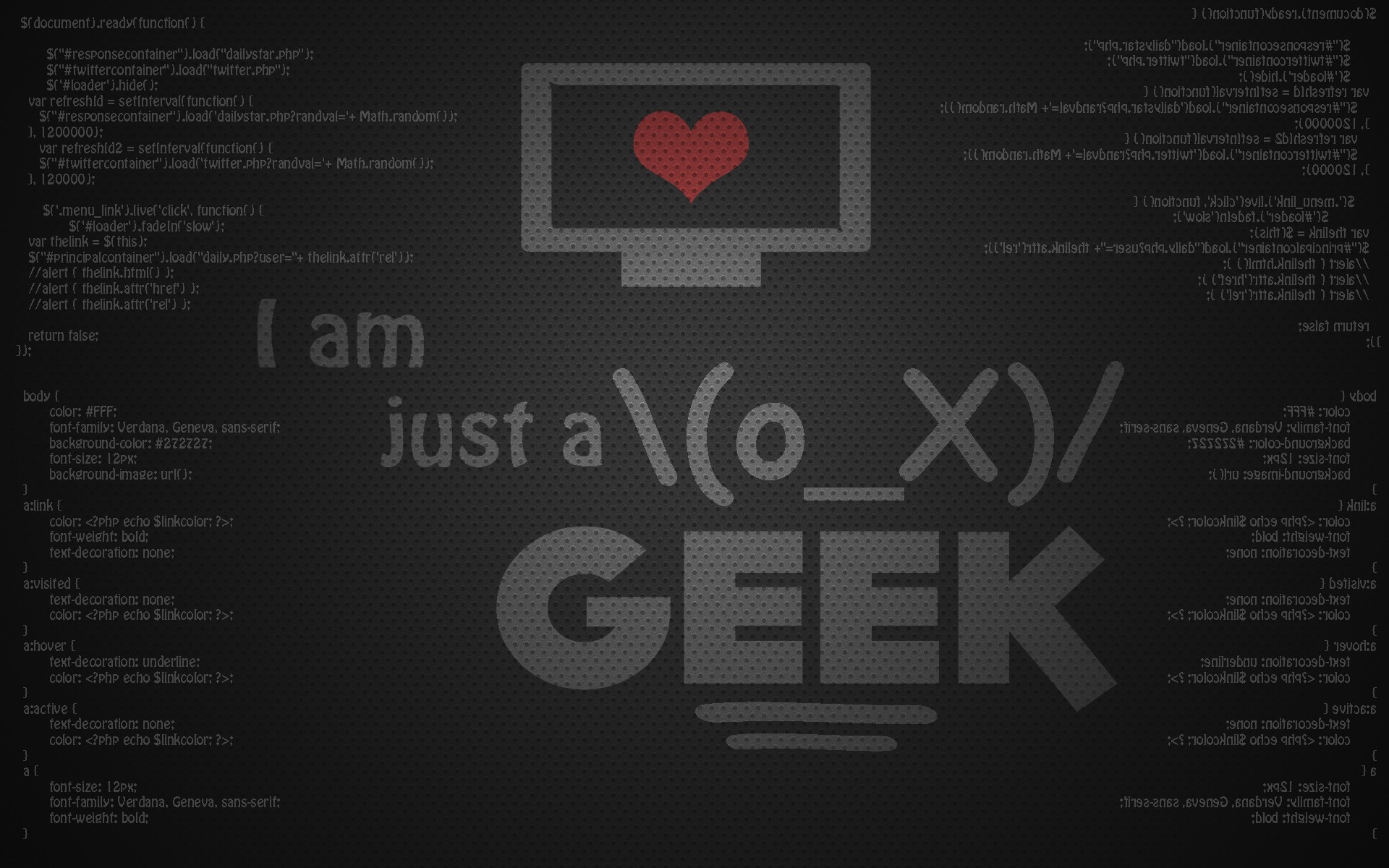
When setting up API Gateway with AWS Lambda and FastAPI, understanding how to properly configure API Gateway is crucial. This section will give you a more detailed breakdown of setting up API Gateway, including how to map requests and responses between API Gateway and Lambda, and how to configure authentication.
Let’s dive deeper into the nuts and bolts of the setup.
Detailed Breakdown of API Gateway Setup and Integration
After setting up your FastAPI app and preparing it for deployment using the Serverless Framework, you need to configure API Gateway to properly route incoming HTTP requests to AWS Lambda and FastAPI.
1. Creating API Gateway in the AWS Console (Manually)
While we’ll automate most of the process using the Serverless Framework, it’s important to understand the manual setup steps in API Gateway. You can use the AWS Console to create and configure API Gateway directly.
Steps:
Open API Gateway in the AWS Management Console.
Click Create API and choose REST API. You’ll want to select the “REST API” option to handle standard HTTP requests.
API Name: Name your API something memorable, like “FastAPI-Lambda-App”.
Create Resources:
In API Gateway, resources are paths within your API (like
/hello
).Click Actions > Create Resource.
Enter a name and resource path (e.g.,
/hello
) for your resource.
Create Methods:
With your resource (
/hello
) selected, click Actions > Create Method.Choose GET (since we’ll be using this method for a simple FastAPI endpoint).
Integration Type: Select Lambda Function and choose the Lambda function you’ve already created for your FastAPI app.
Ensure you check Use Lambda Proxy Integration, which simplifies passing the request and response data between API Gateway and Lambda.
2. Request and Response Mapping in API Gateway
Mapping templates in API Gateway allow you to transform incoming requests and outgoing responses between API Gateway and your backend (AWS Lambda in this case). Let’s walk through some common scenarios.
Request Mapping Example:
In some cases, you might want to manipulate or validate the incoming request data before it reaches Lambda. For example, converting HTTP request body data into a format your FastAPI function expects.
Select your GET method under your resource (
/hello
).Under Integration Request, click on Mapping Templates.
Choose Add mapping template and set the Content-Type to
application/json
.Now you can define a mapping template using Velocity Template Language (VTL). Here’s a simple example that extracts data from the request:
{
"queryStringParameters": {
"name": "$input.params('name')"
},
"headers": {
"user-agent": "$input.params('User-Agent')"
}
}
In this example:
The query parameter
name
is passed to the Lambda function.The
User-Agent
header is also sent along.
Response Mapping Example:
You may also want to map the Lambda function’s response back to the client in a more structured way.
Under the Integration Response tab, click Mapping Templates.
Select the Content-Type (
application/json
) and provide a template to format the response:
{
"statusCode": 200,
"body": {
"message": "$input.path('$.message')",
"details": "Processed successfully"
}
}
This example assumes your FastAPI app returns a response with a message
field, and you are adding extra details to the response like "Processed successfully."
3. Adding Authentication to Your API Gateway
Securing your API is critical, especially in production environments. API Gateway offers several ways to secure your API, including API keys, AWS IAM roles, and Amazon Cognito. For this example, we’ll focus on setting up API Gateway with Cognito User Pools for authentication.
Steps to Set Up Cognito Authentication:
Create a Cognito User Pool:
Go to the Amazon Cognito Console.
Click Create a user pool and configure it with your desired settings.
Name your user pool (e.g.,
FastAPIUserPool
), and set up any required attributes (like email or username).Configure a client for your user pool that will handle authentication requests (ensure you select “Enable sign-in API for server-based authentication”).
Integrate Cognito with API Gateway:
Go to your API in API Gateway.
Select your GET method under the /hello resource.
Under Method Request, click Authorization.
Choose Cognito User Pool Authorizer and select the user pool you just created.
Enforcing Authorization in FastAPI: In your FastAPI app, you can add logic to validate the incoming requests based on tokens received from Cognito. For example, verifying the JWT token.
Here’s an example of a FastAPI route that requires JWT authentication:
from fastapi import Depends, HTTPException, Security
from fastapi.security import OAuth2PasswordBearer
from jose import JWTError, jwt
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.get("/secure")
async def read_secure_data(token: str = Depends(oauth2_scheme)):
try:
payload = jwt.decode(token, "your-secret-key", algorithms=["HS256"])
user_id = payload.get("sub")
if user_id is None:
raise HTTPException(status_code=401, detail="Invalid token")
return {"message": "Secure data", "user_id": user_id}
except JWTError:
raise HTTPException(status_code=403, detail="Token validation failed")
Explanation:
OAuth2PasswordBearer: Used to handle the Bearer token received from Cognito.
JWT decode: The JWT is decoded and the user information is extracted. If the token is invalid or missing, the request will be rejected.
4. Deploying Your API with Authentication
Once your API Gateway is configured with Cognito and Lambda, and your FastAPI app has authentication in place, it’s time to deploy everything.
In API Gateway, go to Actions > Deploy API.
Choose the stage (for example, “prod” or “dev”) and hit Deploy.
Once deployed, any request to your API Gateway endpoint for the /secure
route will require an authorization token from Cognito. Users without the token will be denied access.
5. Testing Authentication with Postman
Let’s test the secure endpoint using Postman:
First, retrieve an access token by logging into the Cognito user pool.
Use this access token to authenticate requests in Postman. Add the token under Authorization with Bearer Token:
Bearer <your-access-token>
Send a GET request to the
/secure
endpoint:https://{api-id}.execute-api.us-east-1.amazonaws.com/prod/secure
If the token is valid, you should get a response with the secured message and the user’s ID.
Conclusion
You’ve successfully configured API Gateway to work with FastAPI and AWS Lambda, added request and response mapping for flexible integration, and implemented authentication using Cognito. By combining FastAPI’s speed and ease of development with AWS Lambda’s serverless power, you can build secure, scalable APIs that are cost-efficient and easy to maintain.
What’s next? You can extend this setup by:
Adding additional routes with more complex logic.
Implementing role-based access control (RBAC) using Cognito Groups.
Monitoring and analyzing API performance with CloudWatch.
Serverless FastAPI is a powerful way to build robust APIs without managing infrastructure, so take advantage of the flexibility and scalability AWS provides!
Subscribe to my newsletter
Read articles from Tech Shots(RoamPals) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
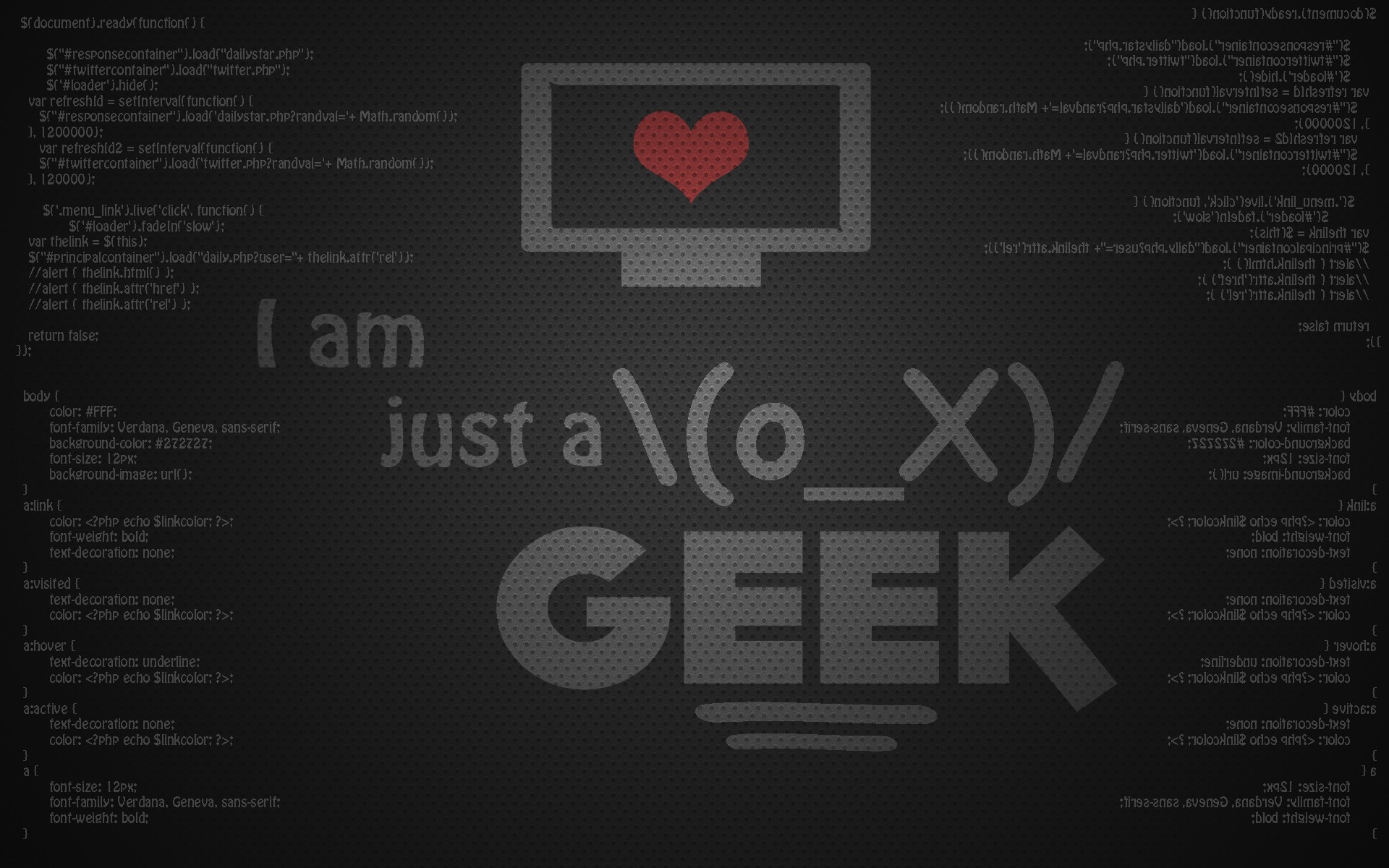