How to Automate Social Media Content Creation Using Python and Deep Learning
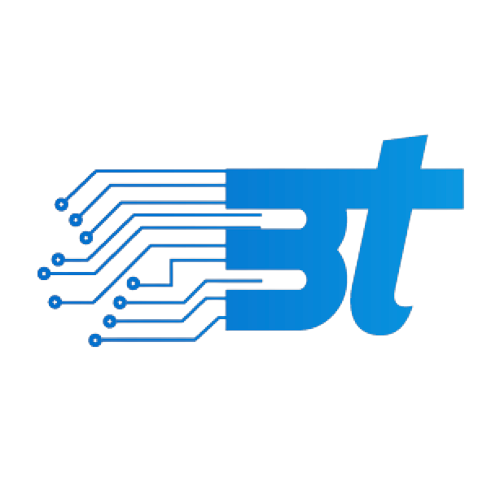
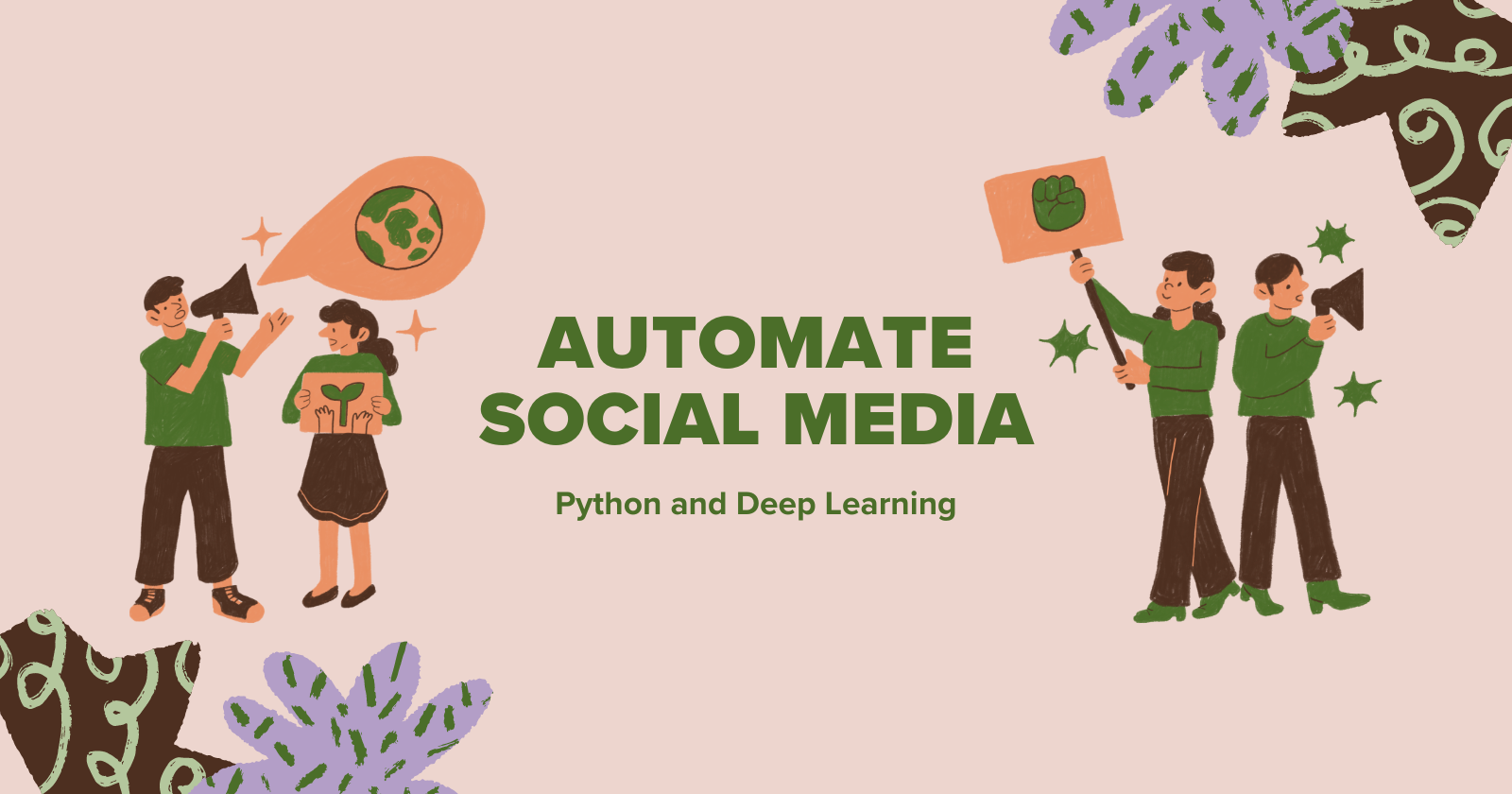
In the fast-paced world of social media, generating engaging content consistently can be challenging for individuals and businesses. Automation can help streamline content creation and allow you to stay ahead of the curve. By leveraging Python and deep learning techniques, you can create an automated pipeline to generate captivating social media posts, captions, and even images.
In this article, we’ll walk you through how to use Python and deep learning models to automate social media content creation.
1. Introduction to Social Media Automation
Social media content creation involves writing compelling posts, generating hashtags, and designing appealing images. By automating some of these processes using AI-powered tools, you can:
Save time by automating repetitive tasks.
Maintain consistent branding and tone.
Increase engagement by quickly reacting to trends.
Python, paired with deep learning, can handle many aspects of content creation—from generating text to producing images.
2. Using Python for Automation
Python is an excellent tool for automating repetitive social media tasks due to its vast ecosystem of libraries and its simplicity. Some tasks that can be automated with Python include:
Post Scheduling: Using Python's integration with APIs like Twitter or Facebook Graph API.
Hashtag Generation: Using natural language processing (NLP) to create relevant hashtags based on the content.
Analytics: Automatically fetching data on likes, shares, and comments for evaluation.
Here's an example of using Python for basic Twitter automation:
import tweepy
# Authenticate to Twitter
auth = tweepy.OAuthHandler("API_KEY", "API_SECRET_KEY")
auth.set_access_token("ACCESS_TOKEN", "ACCESS_TOKEN_SECRET")
api = tweepy.API(auth)
# Create a tweet
api.update_status("Hello world! This is an automated post created with Python.")
3. Deep Learning for Content Creation
Deep learning models are transforming content creation by enabling machines to understand context, generate text, and even create images. Two important deep learning concepts for social media automation are:
NLP for Text Generation: This involves models like GPT (Generative Pretrained Transformer) that can generate human-like text.
Generative Adversarial Networks (GANs): These are deep learning models that generate images, which can be used for creating unique visual content for posts.
4. Generating Social Media Posts with GPT Models
GPT models are highly effective in creating natural language text. They can be fine-tuned to generate social media posts, captions, and even responses to comments. OpenAI's GPT-3 and GPT-4 models are widely used for this purpose.
Here’s how you can use openai
library to generate posts:
import openai
# Set your OpenAI API key
openai.api_key = 'your-api-key'
# Generate a social media post based on a given topic
response = openai.Completion.create(
engine="text-davinci-003",
prompt="Write an engaging social media post about the benefits of meditation.",
max_tokens=50
)
# Extract the generated text
generated_post = response['choices'][0]['text'].strip()
print(generated_post)
The model can generate several variations of posts on different topics, making it easy to automate and diversify content.
5. Automating Image Creation with GANs
Images are an integral part of social media. GANs (Generative Adversarial Networks) are used to create new images by learning from a set of training images. A popular application is StyleGAN, which generates images with various artistic styles.
Using TensorFlow
or PyTorch
, you can create and fine-tune a GAN to generate custom visuals. Pre-trained GANs like Artbreeder or DeepArt can be integrated into your pipeline.
For example, using the keras
library to load a pre-trained GAN:
from keras.preprocessing import image
from keras.applications.vgg19 import VGG19
# Load a pre-trained model (GAN can be used similarly)
model = VGG19(weights='imagenet', include_top=False)
# Preprocess an input image
img = image.load_img('input.jpg', target_size=(224, 224))
img_array = image.img_to_array(img)
img_array = np.expand_dims(img_array, axis=0)
# Predict and generate features
features = model.predict(img_array)
You can use this to generate backgrounds, textures, or custom visuals for your social media posts.
6. Scheduling and Publishing Content
Once your content is generated, Python libraries like schedule
or APScheduler
can help automate the posting process. This ensures that your posts go live at the most optimal times for engagement.
Here’s a simple Python script to schedule posting a tweet:
import schedule
import time
def post_tweet():
api.update_status("This is a scheduled post!")
# Schedule the tweet to post every day at a certain time
schedule.every().day.at("12:00").do(post_tweet)
while True:
schedule.run_pending()
time.sleep(1)
With more complex APIs like Facebook Graph API or Instagram’s Business API, you can automate cross-platform content posting.
Conclusion
As AI technology continues to evolve, the potential for automating content creation will grow, allowing for more personalized and creative automation.
Key Takeaways:
Python, with its powerful libraries, can automate various social media tasks.
GPT models can generate human-like posts and captions, while GANs can create unique images.
By automating both content generation and scheduling, you can maintain a consistent social media presence without constant manual effort.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
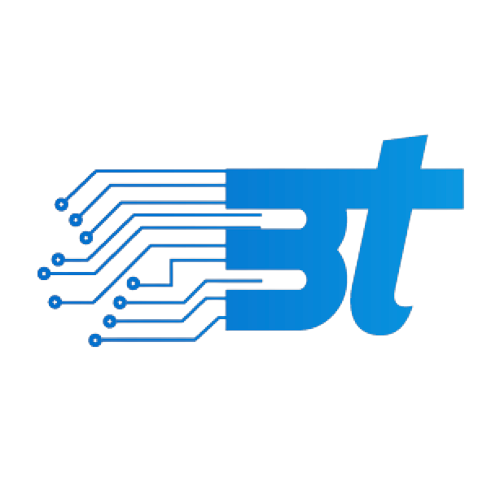
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.