Project 3: Write a MakeFile For Two-Tier-Flask-App
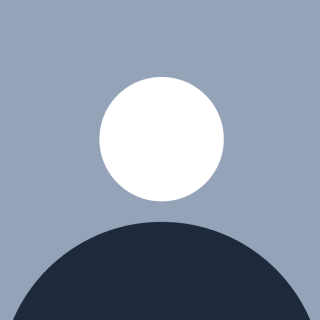
In this blog, I'll guide you through understanding and writing a Makefile that uses Docker Compose to build, run, and manage a two tier flask app across multiple operating systems. If you’re working on a project with Docker and need an easy way to streamline commands, this Makefile will come in handy.
Overview of the Makefile
A Makefile is a simple file that contains a set of instructions or rules for automating repetitive tasks. This specific Makefile is designed to manage a Dockerized service, with different commands tailored to run on Linux, macOS, and Windows. It provides four main functionalities:
Build the Docker image.
Run the service in the background.
Stop the service.
Clean up Docker resources.
Now, let's break down the Makefile and see how it works.
1. Determine the Operating System
OS := $(shell uname)
$(shell uname): This command is used to detect the operating system by running the
uname
command.On Linux,
uname
returnsLinux
.On macOS, it returns
Darwin
.On Windows,
uname
is not available, but the Makefile usesWindows_NT
to handle Windows systems.
This OS detection allows the Makefile to adjust commands based on which platform it is being executed on.
2. Define Docker Compose Command
DOCKER_COMPOSE := docker compose
This defines a variable
DOCKER_COMPOSE
which holds the commanddocker compose
.This is used later in the script to run Docker Compose commands without repeating the full
docker compose
command every time.
3. Define the Docker Service Name
SERVICE_NAME := web
SERVICE_NAME
is set to web, which is a placeholder for the actual name of the Docker service you are working with. In Docker Compose, services are defined in the docker-compose.yml
file.
4. Build the Docker Image
build:
ifeq ($(OS),Linux)
@echo "Building for Linux"
$(DOCKER_COMPOSE) build
endif
ifeq ($(OS),Darwin)
@echo "Building for macOS"
$(DOCKER_COMPOSE) build
endif
ifeq ($(OS),Windows_NT)
@echo "Building for Windows"
# Add Windows-specific build commands if you wish
endif
This section defines the build target, which builds the Docker image using Docker Compose. It does this differently depending on the detected OS:
Linux: If the OS is Linux, the script prints
Building for Linux
and runsdocker compose build
.macOS: If the OS is macOS, it prints
Building for macOS
and runsdocker compose build
.Windows: If it detects
Windows_NT
(for Windows), it printsBuilding for Windows
. You can add Windows-specific build commands here.
5. Run the Service
run:
ifeq ($(OS),Linux)
@echo "Running for Linux"
$(DOCKER_COMPOSE) up -d
endif
ifeq ($(OS),Darwin)
@echo "Running for macOS"
$(DOCKER_COMPOSE) up -d
endif
ifeq ($(OS),Windows_NT)
@echo "Running for Windows"
# Add Windows-specific run commands if you wish
endif
The run target starts the Docker service defined in the docker-compose.yml
file. Like the build section, it adjusts the commands depending on the OS:
Linux: Runs
docker compose up -d
, which starts the Docker containers in the background.macOS: Same as Linux, running the service in the background.
Windows: You can add custom run commands for Windows if needed.
6. Stop the Service
stop:
$(DOCKER_COMPOSE) down
The stop target stops and removes all running containers for the service. This target is the same for all operating systems, as the docker compose down
command works across platforms.
7. Clean Up Docker Resources
The clean target first runs the stop
target (i.e., stops the containers) and then removes any remaining containers and resources:
$(DOCKER_COMPOSE) rm -f
: Removes all stopped containers for the service.'docker system prune -f': Cleans up any dangling Docker images, networks, volumes, and containers that are no longer in use.
This ensures that you’re left with a clean Docker environment.
8. How to Use the Makefile
You can use this Makefile to simplify your Docker workflows. To run the tasks:
Build the Docker image:
make build
Run the Docker service:
make run
Stop the Docker service:
make stop
Clean up Docker resources:
make clean
The 'make' command automatically detects the correct task based on the OS you are running on.
Conclusion
This Makefile helps streamline Docker-related tasks across different operating systems. It detects the OS and runs appropriate Docker Compose commands, making your workflow more efficient. Whether you're using Linux, macOS, or Windows, this Makefile allows you to quickly build, run, stop, and clean up your Docker services with just a few commands.
By using a Makefile, you reduce manual errors and improve the efficiency of repetitive tasks, making it easier to manage Dockerized services in a cross-platform environment.
You can access the MAKEFILE and two tier flask app from here:
https://github.com/huzefaweb/Makefile_for_two_tier_flask_app
Subscribe to my newsletter
Read articles from Huzefa Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Huzefa Ahmed
Huzefa Ahmed
👋 Hi, I’m Huzefa Ahmed, and I’m starting my DevOps engineering journey! 🚀 I’m passionate about AWS DevOps and aim to land a full-time role in this field. As I dive into cloud technologies, automation, and continuous integration, I’ll share my progress, insights, and challenges. Let’s connect, learn, and grow as a DevOps community! Follow my journey on Hashnode and LinkedIn as I work towards securing a job in DevOps! LinkedIn: https://www.linkedin.com/in/huzefa-ahmed-15720b278/