What is Virtual DOM in React?

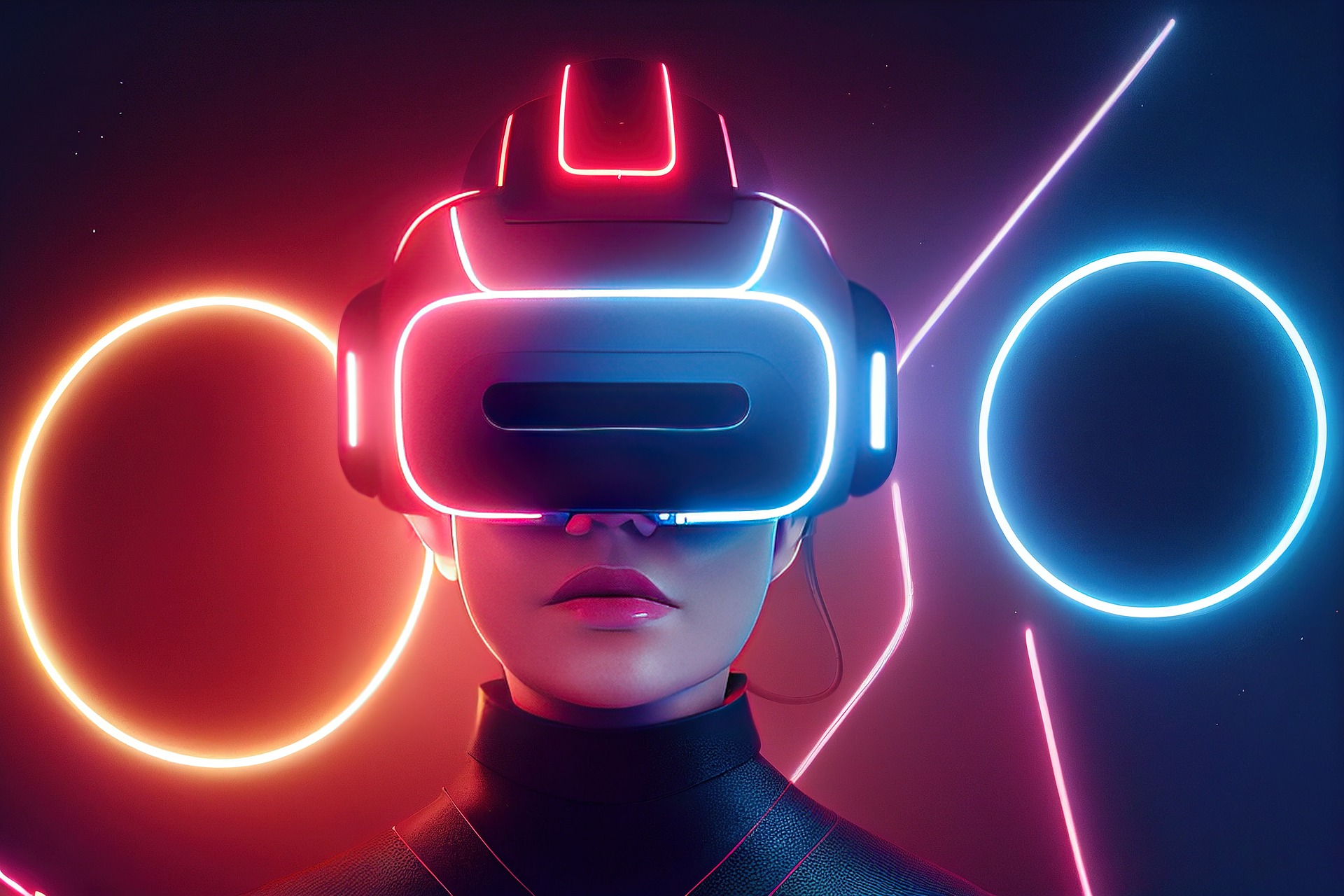
The Virtual DOM is a fundamental concept in React that significantly enhances the library's performance and efficiency. Understanding the Virtual DOM is crucial for both developing React applications and performing well in React-related interviews. Here's an in-depth explanation of what the Virtual DOM is, how it works, and why it's important:
What is the Virtual DOM?
The Virtual DOM (VDOM) is an in-memory representation of the actual Document Object Model (DOM) used by web browsers to render web pages. It's a lightweight copy of the real DOM maintained by React. Instead of directly manipulating the real DOM, React uses the Virtual DOM to manage changes and updates more efficiently. Now a question may arise that if React uses the Virtual DOM to manage changes, then how do the changes reflect in the real DOM?
How does the Virtual DOM reflect in the real DOM ?
step by step bullet points explains the process better. So, focus the following bullet points to understand the process:
Initial Render
When your React application first loads, React creates a Virtual DOM tree that mirrors the structure of the real DOM based on your components and state.
React then renders this Virtual DOM into the real DOM, which the browser displays.
State and Props Changes
When the state or props of a component change, React creates a new Virtual DOM tree reflecting these changes.
React compares this new Virtual DOM with the previous one to identify what has changed (using the diffing algorithm).
Updating the Real DOM
React calculates the minimal set of changes needed to update the real DOM to match the new Virtual DOM.
It then applies these changes to the real DOM.
The browser re-renders the affected parts of the UI accordingly.
What you see on the Browser: The browser only understands and renders the real DOM. The Virtual DOM is not rendered or visible in the browser; it's an internal mechanism used by React. All visual elements you see on the webpage are part of the real DOM.
Why Virtual DOM is Beneficial
Performance Optimization: Direct manipulation of the real DOM is relatively slow because the DOM is a complex and hierarchical structure. By using the Virtual DOM to batch and minimize updates, React improves performance and responsiveness, especially in applications with frequent UI updates.
Declarative UI: Developers describe what the UI should look like for a given state, and React takes care of updating the DOM to match that state. This declarative approach simplifies development and reduces the likelihood of bugs related to manual DOM manipulation.
Simplified Programming Model: Developers can focus on building components and managing state without worrying about the complexities of DOM manipulation, event handling, and browser compatibility issues.
How Virtual DOM Updates Work By Example
Consider a simple React component that renders a list of items:
import React, { useState } from 'react';
function ItemList() {
const [items, setItems] = useState(['Apple', 'Banana', 'Cherry']);
const addItem = () => {
setItems([...items, 'Date']);
};
return (
<div>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<button onClick={addItem}>Add Item</button>
</div>
);
}
export default ItemList;
React creates a Virtual DOM tree representing the <div>
, <ul>
, and <li>
elements based on the initial state ['Apple', 'Banana', 'Cherry']
.
When the "Add Item" button is clicked, the addItem
function updates the state to ['Apple', 'Banana', 'Cherry', 'Date']
. React generates a new Virtual DOM tree reflecting the updated list. The diffing algorithm compares the new Virtual DOM with the previous one, identifies that a new <li>
element for "Date" needs to be added. React updates the real DOM by only appending the new <li>
element, avoiding a full re-render of the entire list.
How the Virtual DOM Enhances Performance
Browsers perform reflows and repaints when the DOM is updated, which can be expensive operations. By minimizing the number of direct DOM manipulations, the Virtual DOM reduces these costly processes. React batches multiple state updates and applies them in a single operation, reducing the number of times the DOM needs to be updated. React's diffing algorithm ensures that only the necessary parts of the UI are updated, avoiding unnecessary re-renders and improving overall application performance.
React Fiber and the Virtual DOM
React Fiber is the underlying architecture that powers React's reconciliation process. It improves the Virtual DOM by allowing React to handle updates in small parts and manage tasks that happen at different times. This helps React focus on important updates first, handle large updates more smoothly, and make applications respond faster to user interactions.
Conclusion
The Virtual DOM is a pivotal aspect of React that enables efficient, high-performance updates to the user interface. By maintaining a lightweight copy of the real DOM and using smart algorithms to manage changes, React ensures that applications remain fast and responsive, even as they grow in complexity. Understanding the Virtual DOM is essential for React developers, as it not only helps in writing optimized code but also provides insights into how React manages rendering and updates under the hood.
Subscribe to my newsletter
Read articles from Abeer Abdul Ahad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abeer Abdul Ahad
Abeer Abdul Ahad
I am a Full stack developer. Currently focusing on Next.js and Backend.