Unlocking the Power of Integration with Node.js and Salesforce

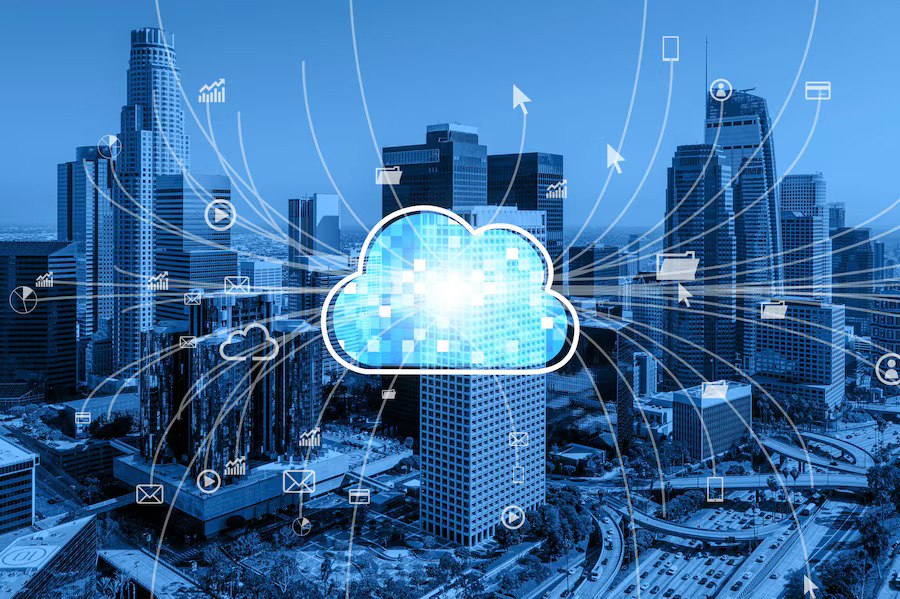
Introduction
In today’s interconnected digital landscape, businesses are constantly seeking ways to streamline their operations, improve efficiency, and enhance customer experiences. One powerful combination that achieves these goals is the integration of Node.js with Salesforce. By leveraging the strengths of both technologies, organizations can unlock new levels of productivity and innovation.
Node.js is a versatile JavaScript runtime built on Chrome's V8 JavaScript engine, known for its event-driven architecture and non-blocking I/O operations. It allows developers to build scalable network applications efficiently. Salesforce, on the other hand, is a leading Customer Relationship Management (CRM) platform that helps businesses manage customer interactions, sales processes, and data.
Integrating Node.js with Salesforce offers a multitude of benefits, including real-time data synchronization, enhanced automation, and the ability to build custom applications tailored to specific business needs. This comprehensive guide will explore the intricities of integrating Node.js with Salesforce, providing in-depth insights, practical examples, and best practices.
1. Understanding the Need for Integration
1.1. The Role of Salesforce
Hire Salesforce Developers is a powerful CRM platform that provides a range of tools to manage customer relationships, track sales, and analyze data. Businesses use Salesforce to gain insights into customer behavior, manage leads and opportunities, and automate various processes. The platform offers various modules such as Sales Cloud, Service Cloud, Marketing Cloud, and more, each designed to cater to different business needs.
1.2. The Role of Node.js
Node.js is a JavaScript runtime that enables developers to build fast and scalable server-side applications. It’s particularly well-suited for building real-time applications, APIs, and microservices. With its non-blocking I/O model, Node.js can handle a large number of simultaneous connections efficiently, making it ideal for applications that require high performance and responsiveness.
1.3. Why Integrate Node.js with Salesforce?
Integrating Node.js with Salesforce provides several advantages:
Real-Time Data Synchronization: Ensure that your Salesforce data is consistently updated across applications and systems.
Enhanced Automation: Automate repetitive tasks and workflows, reducing manual effort and improving efficiency.
Custom Applications: Build custom applications that leverage Salesforce data and functionality, tailored to your specific business needs.
Scalability: Scale your applications efficiently to handle growing amounts of data and user interactions.
2. What is JSforce?
2.1. Introduction to JSforce
JSforce is a popular open-source JavaScript library designed to facilitate integration between Node.js and Salesforce. It simplifies the process of interacting with Salesforce APIs, making it easier for developers to perform various operations such as querying data, updating records, and managing metadata.
2.2. Key Features of JSforce
Ease of Use: Provides a straightforward API for interacting with Salesforce.
Support for Multiple APIs: Includes support for Salesforce REST API, SOAP API, Bulk API, and Streaming API.
Real-Time Updates: Allows for real-time updates and notifications using the Streaming API.
Data Management: Facilitates bulk data operations and file handling.
2.3. Why Use JSforce?
Simplified Integration: Reduces the complexity of working with Salesforce APIs directly.
Flexibility: Can be used in various environments, including server-side and client-side applications.
Active Community: Supported by an active community and regular updates.
3. Prerequisites for Integration
3.1. Salesforce Developer Account To integrate Node.js with Salesforce, you need a Salesforce Developer Account. This account provides access to Salesforce’s development environment, including API access and tools for creating Connected Apps.
3.2. Node.js and NPM Ensure that Node.js and NPM (Node Package Manager) are installed on your system. Node.js is essential for running your JavaScript code on the server side, while NPM is used to manage your project’s dependencies.
3.3. Salesforce API Access Your Salesforce instance must have API access enabled. This is typically available in Enterprise, Unlimited, and Developer editions. You will also need to create a Connected App within Salesforce to obtain the necessary credentials for authentication.
3.4. Basic Understanding of JavaScript and Node.js Familiarity with JavaScript and Node.js is crucial, as you’ll be writing server-side code to interact with Salesforce Development Services.
4. Setting Up Your Salesforce Connected App
4.1. Create a Connected App To authenticate your Node.js application with Salesforce, you need to create a Connected App. Follow these steps:
Log in to Salesforce: Access your Salesforce Developer account.
Navigate to Setup: Go to Setup by clicking the gear icon in the top right corner.
Find App Manager: In the Quick Find box, search for App Manager and select it.
Create New Connected App: Click New Connected App.
Fill in App Details:
Connected App Name: Enter a name for your app.
API Name: This is auto-filled based on the Connected App Name.
Contact Email: Provide a contact email address.
Enable OAuth Settings:
Check the box Enable OAuth Settings.
Callback URL: Enter
http://localhost:3000/oauth/callback
(this can be changed based on your setup).OAuth Scopes: Add the necessary OAuth scopes such as Full access (full) or Access and manage your data (api).
Save: Save your Connected App settings and take note of the Consumer Key and Consumer Secret.
4.2. Obtain OAuth Credentials Once your Connected App is created, you will get a Consumer Key and Consumer Secret. These credentials are required for authenticating your Node.js Development Company with Salesforce.
5. Integrating Node.js with Salesforce Using JSforce
5.1. Install JSforce
First, install JSforce in your Node.js project by running the following command in your project directory:
bashCopy codenpm install jsforce
5.2. Set Up Your Node.js Application
Create a simple Node.js application to connect to Salesforce. Create a file named app.js
and add the following code:
javascriptCopy codeconst jsforce = require('jsforce');
const express = require('express');
const app = express();
// Salesforce connection details
const oauth2 = new jsforce.OAuth2({
loginUrl: 'https://login.salesforce.com',
clientId: 'YOUR_CONSUMER_KEY',
clientSecret: 'YOUR_CONSUMER_SECRET',
redirectUri: 'http://localhost:3000/oauth/callback'
});
app.get('/oauth/callback', (req, res) => {
const conn = new jsforce.Connection({ oauth2 });
const code = req.query.code;
conn.authorize(code, (err, userInfo) => {
if (err) {
return res.send('Authentication error: ' + err.message);
}
res.send('Successfully connected to Salesforce');
});
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In this example:
We use Express to create a basic web server.
JSforce is used to handle OAuth2 authentication with Salesforce.
When a user visits
http://localhost:3000/oauth/callback
, it triggers the OAuth callback and attempts to authenticate with Salesforce.
5.3. Authenticate and Connect
To authenticate, open the following URL in your browser:
bashCopy codehttp://localhost:3000/oauth/callback
Log in with your Salesforce credentials and authorize the app. Once authenticated, you’ll be redirected back to your Node.js app with a confirmation message.
5.4. Querying Salesforce Data
Once connected, you can query Salesforce data using SOQL (Salesforce Object Query Language). For example, to fetch data from the Account object, you can add the following code:
javascriptCopy codeapp.get('/accounts', (req, res) => {
const conn = new jsforce.Connection({ oauth2 });
conn.query('SELECT Id, Name FROM Account', (err, result) => {
if (err) {
return res.send('Error querying Salesforce: ' + err.message);
}
res.json(result.records);
});
});
In this code:
We use
conn.query
to execute a SOQL query to fetch Account records.The results are returned in JSON format.
5.5. Handling Bulk Data Operations
For bulk data operations, you can use the Bulk API provided by Salesforce. Here’s an example of how to use JSforce for bulk data uploads:
javascriptCopy codeapp.post('/bulk-upload', (req, res) => {
const conn = new jsforce.Connection({ oauth2 });
const records = [
{ Name: 'Account1', Industry: 'Technology' },
{ Name: 'Account2', Industry: 'Finance' }
];
conn.bulk.load('Account', 'insert', records, (err, rets) => {
if (err) {
return res.send('Error uploading data: ' + err.message);
}
res.send('Bulk upload complete: ' + JSON.stringify(rets));
});
});
In this example:
- We use
conn.bulk.load
to perform a bulk insert operation for Account records.
5.6. Real-Time Data Updates with Streaming API
JSforce supports Salesforce’s Streaming API, which allows you to receive real-time updates. Here’s an example of how to use the Streaming
Subscribe to my newsletter
Read articles from Saurabh Dhariwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Dhariwal
Saurabh Dhariwal
Saurabh is a qualified engineer with more than a decade of proven experience in solving complex problems with scalable solutions, harnessing the power of PHP frameworks/CMS with a keen eye on UX.