Virtual DOM, Fiber and Reconciliation

Table of contents
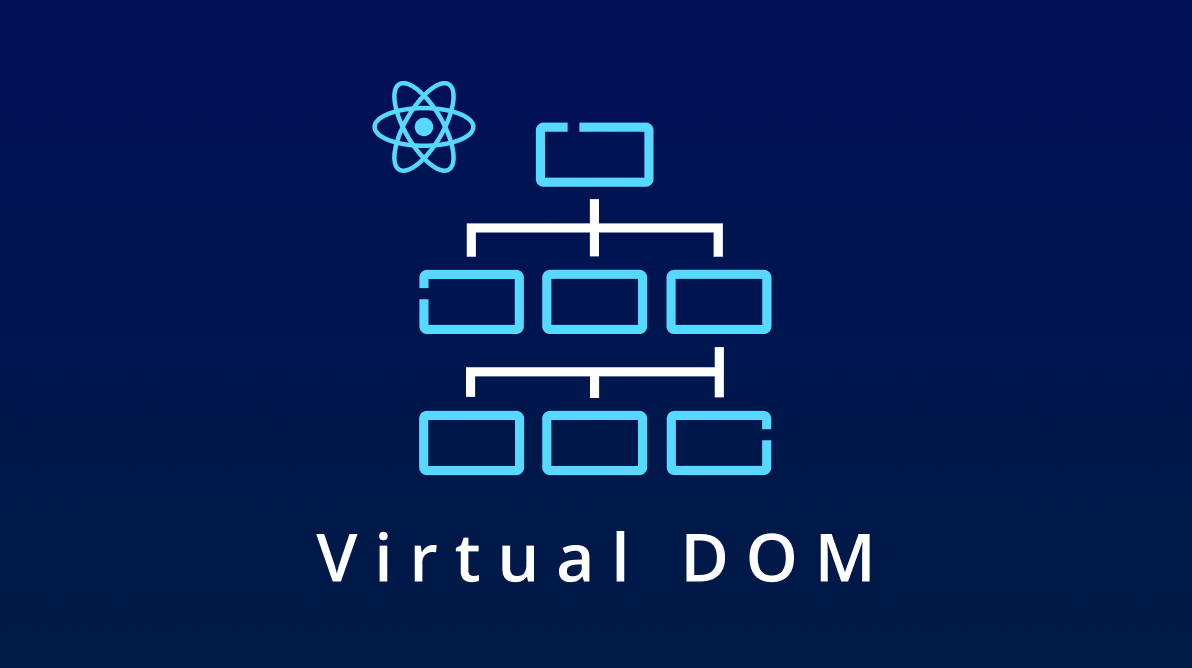
Get ready for some theoretical concepts and bear with me, it will be an interesting session.
Nowadays, Virtual Dom is not used that much in React but it does not mean we should not study about it because it is important from an interview perspective. Also, it is asked to check that you have knowledge of the basics of React. But in the practical world, it is not being implemented much because many internals have been changed.
Some major topics that we are covering in this blog are Virtual DOM, Fiber, and Reconciliation.
Prerequisites: Basic Knowledge of DOM(Document Object Model).
Virtual DOM
What is a Virtual DOM(Document Object Model) and How is it different than the Browser DOM?
Look at the following boilerplate code in the main.jsx
file :
import { StrictMode } from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
ReactDOM.createRoot(document.getElementById('root')).render(
<StrictMode>
<App />
</StrictMode>,
)
We are going to understand the working and behind-the-scenes of createRoot()
function :
Whenever we make some changes in code and then reload our page, the browser rewrites the DOM completely after removing everything written previously. This rewriting of DOM after completely removing the previous one is known as the reloading of a page.
This repetition of completely removing and writing DOM many times results in low efficiency. That is why React introduced Virtual DOM.
Virtual Dom is created by React which is somewhat similar to Browser-created Dom.
This DOM is created when we call the function createRoot()
using ReactDOM.
It is a tree-like structure that contains different elements of the web page.
Whenever we make some changes in code, Virtual Dom only removes that part of the code that is changed and adds the new element in place. This prevents complete reloading of the page again and again.
I hope you understand the basic idea of Virtual DOM.
But the only drawback of Virtual Dom was it updates the changes immediately. It does not look like a drawback I know, but in some cases, it is. Let us take a simple example of animations: In an animation, we see that the changes are coming one after the other, it is obvious, right? Whenever any update comes from the animation part of the code, Virtual Dom updates it immediately. Still, while this update is in process, another update comes from the animation part and then Virtual Dom has to update that change also, and this repetition of changes in Virtual DOM goes on until the updates stop coming. Why can't it just wait and update the whole thing at once after the updates stop coming? So, there are some scopes of optimization, right? This is where React Fibre comes into the picture.
React Fiber
React Fibre is an implementation of React's core algorithm.
We have already discussed the goal of React Fiber in the above paragraph. Its main feature is to take the rendering or loading process, break it into multiple parts, and update only those parts that have been changed, which is basically the concept we have understood previously.
The other feature that React Fibre provides is the ability to stop, cancel, or reuse previous updates whenever new updates come in to optimize the UI updation.
Also, React Fibre assigns priorities to different updates, For example, animations need to be updated more quickly than a variable.
This is the basic overview of React Fiber, we are not studying React Fibre Architecture because no one does actually, it is a really high-level topic.
But How are these optimizations done actually? What technique or algorithm is used to make these decisions?
Reconciliation
Reconciliation is an algorithm used by React to differentiate between two trees. Which two trees? The Virtual DOM tree that is created by React using createRoot()
function and the Browser DOM tree that is created by the browser. This algorithm differentiates these two trees and determines which part of the tree needs to be changed.
For example, in the case of a browser application, when the program is updated (usually via setState
), a new tree is generated. The new tree is differentiated or compared with the previous tree to compute which operations are needed to update the rendered app.
Actually, the Reconciliation algorithm is the so-called "behind-the-scene" of Virtual DOM.
Summary
The changes or updations in the UI/WebPage do not need to be immediate with every single change in the program. Sometimes it can be a bit less efficient and results in degrading the User experience.
Therefore, DOM can wait for a few moments before making the changes in the UI so that all the updates are handled at once.
Different types of updates have different priorities as we have discussed above with an example of animation and variable.
There are two types of approaches to make decisions for UI updation :
Push-based Approach: It is an approach in which the programmer itself makes the decisions. It is implemented when the programmer wants immediate change without the interference of the framework.
Pull-based Approach: It is an approach React itself makes the decision for us(programmers) and makes changes in the UI. An example of this approach is using the useState() hook (explained in the Previous Blog) where React itself handles the updation of UI when the variable is updated.
For more details on React Fiber and Reconciliation, please refer here.
Thank you.
Subscribe to my newsletter
Read articles from Umar Khursheed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Umar Khursheed
Umar Khursheed
On a journey to explore the exciting realms of Development. Pursuing Btech in Information Technology from GL Bajaj Institute of Technology and Management. Simultaneously, my journey includes the fascinating realm of Data Structures and Algorithms using C++. Solving complex problems and optimizing solutions is my forte, and I'm always eager to explore new algorithms and data structures that enhance my problem-solving skills.