Control Flow Statements in Java
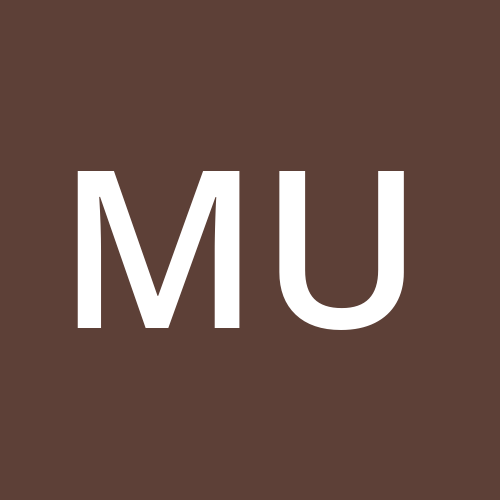
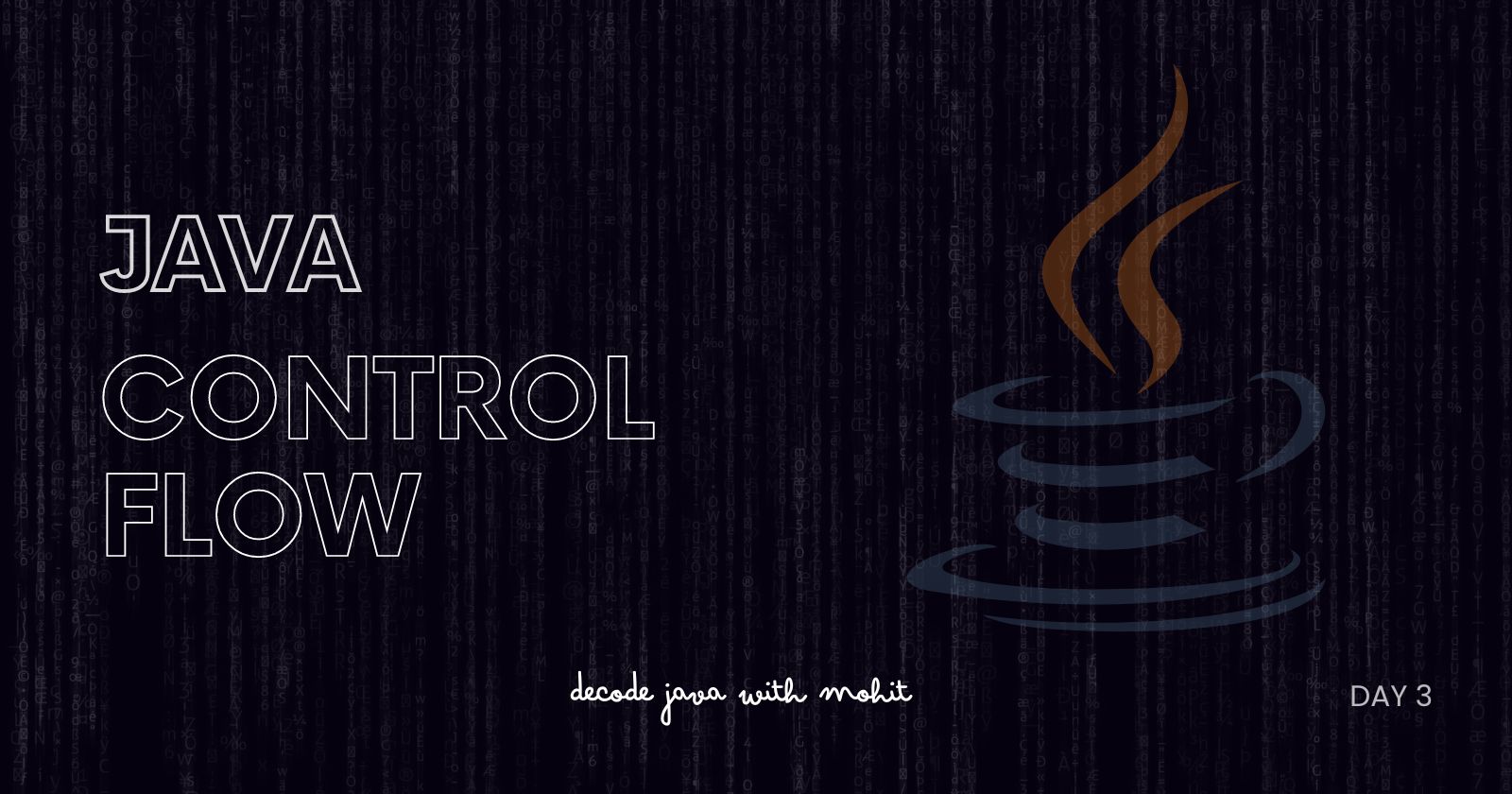
On Day 3, we will focus on understanding how Java programs make decisions and control the flow of execution based on different conditions and loops.
1. Introduction to Control Flow
Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated in a program. In Java, control flow can be managed using conditional statements and loops.
Conditional Statements allow the program to execute a block of code if a particular condition is true.
Loops allow repeated execution of a block of code as long as a certain condition is met.
2. if
, if-else
, and else-if
Statements
The if-else
statement is one of the most commonly used conditional control flow structures. It checks a condition and executes a block of code if the condition is true, and an alternative block if the condition is false.
Basic if
Statement:
int number = 10;
if (number > 5) {
System.out.println("Number is greater than 5");
}
if-else
Statement:
int number = 4;
if (number > 5) {
System.out.println("Number is greater than 5");
} else {
System.out.println("Number is not greater than 5");
}
else-if
Ladder: This structure is used when you need to test multiple conditions.
int score = 85;
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else if (score >= 70) {
System.out.println("Grade: C");
} else {
System.out.println("Grade: D or lower");
}
Points to Remember:
The condition in the
if
statement must be a boolean expression, i.e., it should evaluate totrue
orfalse
.You can have multiple
else-if
statements, but only oneelse
block.Once a condition is true, no further conditions in the
else-if
ladder are checked.
3. The switch
Statement
The switch
statement is used when you have multiple possible values for a variable, and you want to execute different code blocks depending on the value. It's an alternative to using multiple else-if
statements when comparing the same variable.
Syntax:
int day = 3;
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
default:
System.out.println("Invalid day");
}
Enhanced Switch Case Syntax :
int day = 3;
switch(day){
// here no need to specify break statement
case 1 -> System.out.println("Monday");
case 2 -> System.out.println("Tuesday");
case 3 -> System.out.println("Wednesday");
case 4 -> System.out.println("Thrusday");
case 5 -> System.out.println("Friday");
case 6 -> System.out.println("Saturday");
case 7 -> System.out.println("Sunday");
default -> System.out.println("Invalid Number of Day..");
}
Key Points:
Each
case
block compares the variable (day
) with a constant value.The
break
statement exits the switch block once a matching case is executed. Withoutbreak
, execution would continue to the next case.The
default
case is optional and executes if none of the cases match.
4. Loops in Java
Loops are used to repeatedly execute a block of code as long as the loop's condition remains true. Java provides three types of loops: for
loop, while
loop, and do-while
loop.
for
Loop: The for
loop is used when you know in advance how many times you want to repeat a block of code.
for (int i = 0; i < 5; i++) {
System.out.println("i: " + i);
}
for-each
Loop:
int ar[] = { 10, 50, 60, 80, 90 };
for (int element : ar)
System.out.print(element + " ");
}
The loop consists of three parts: initialization, condition, and increment/decrement.
In the example,
i
starts at 0, and the loop continues as long asi < 5
. After each iteration,i
is incremented by 1.
while
Loop: The while
loop executes as long as a given condition is true. It's used when the number of iterations is not known beforehand.
int i = 0;
while (i < 5) {
System.out.println("i: " + i);
i++;
}
- Here, the loop checks the condition before executing the block of code. If the condition is false at the beginning, the loop won’t run even once.
do-while
Loop: The do-while
loop is similar to the while
loop but guarantees that the code block is executed at least once, even if the condition is false initially.
int i = 0;
do {
System.out.println("i: " + i);
i++;
} while (i < 5);
Key Differences Between Loops:
for
loop: Used when the number of iterations is known.while
loop: Used when the condition needs to be checked before each iteration.do-while
loop: Used when the block of code needs to be executed at least once.
5. Break and Continue Statements
break
: Exits the loop or switch statement immediately.continue
: Skips the current iteration of the loop and moves to the next iteration.
Example of break
:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i is 5
}
System.out.println("i: " + i);
}
Example of continue
:
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue; // Skip the iteration when i is 5
}
System.out.println("i: " + i);
}
6. Nested Loops
A loop inside another loop is called a nested loop. They are often used in scenarios like printing patterns or handling multidimensional arrays.
Example of a Nested Loop:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
System.out.println("i: " + i + ", j: " + j);
}
}
Output:
i: 1, j: 1
i: 1, j: 2
i: 1, j: 3
i: 2, j: 1
i: 2, j: 2
i: 2, j: 3
i: 3, j: 1
i: 3, j: 2
i: 3, j: 3
7. Practical Example: Number Guessing Game
Here's a small practical project that uses control flow and loops to create a number guessing game:
import java.util.Scanner;
public class GuessingGame {
public static void main(String[] args) {
int targetNumber = (int) (Math.random() * 100) + 1; // Generate a random number between 1 and 100
Scanner scanner = new Scanner(System.in);
int guess;
int attempts = 0;
do {
System.out.print("Guess a number between 1 and 100: ");
guess = scanner.nextInt();
attempts++;
if (guess > targetNumber) {
System.out.println("Too high!");
} else if (guess < targetNumber) {
System.out.println("Too low!");
} else {
System.out.println("Correct! You guessed it in " + attempts + " attempts.");
}
} while (guess != targetNumber);
scanner.close();
}
}
How it Works:
The game generates a random number between 1 and 100.
The player guesses the number, and the program provides feedback (
Too high
orToo low
) until the player guesses correctly.
Summary of Day 4
By the end of Day 3, we will have a solid understanding of:
Conditional statements like
if
,if-else
, andswitch
.Different types of loops (
for
,while
,do-while
) and their use cases.The purpose and use of
break
andcontinue
.Practical applications of control flow in Java.
This knowledge will equip readers with the skills to write more dynamic, interactive programs that can make decisions and handle repetitive tasks effectively. So stay tuned!! for more advanced topics.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
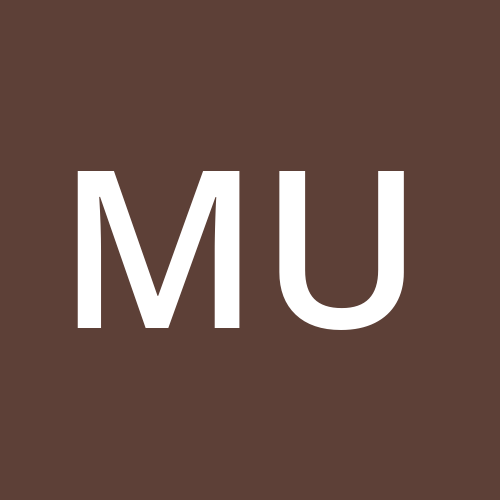