Tackling Real-World Problems with Divide and Conquer: An Interactive Guide
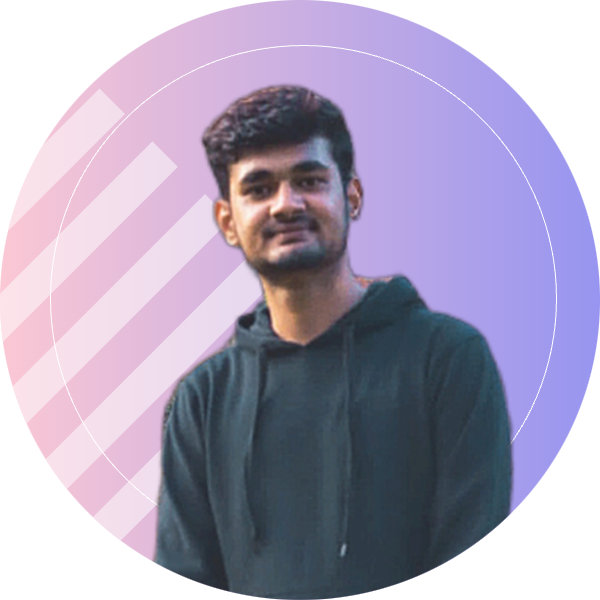
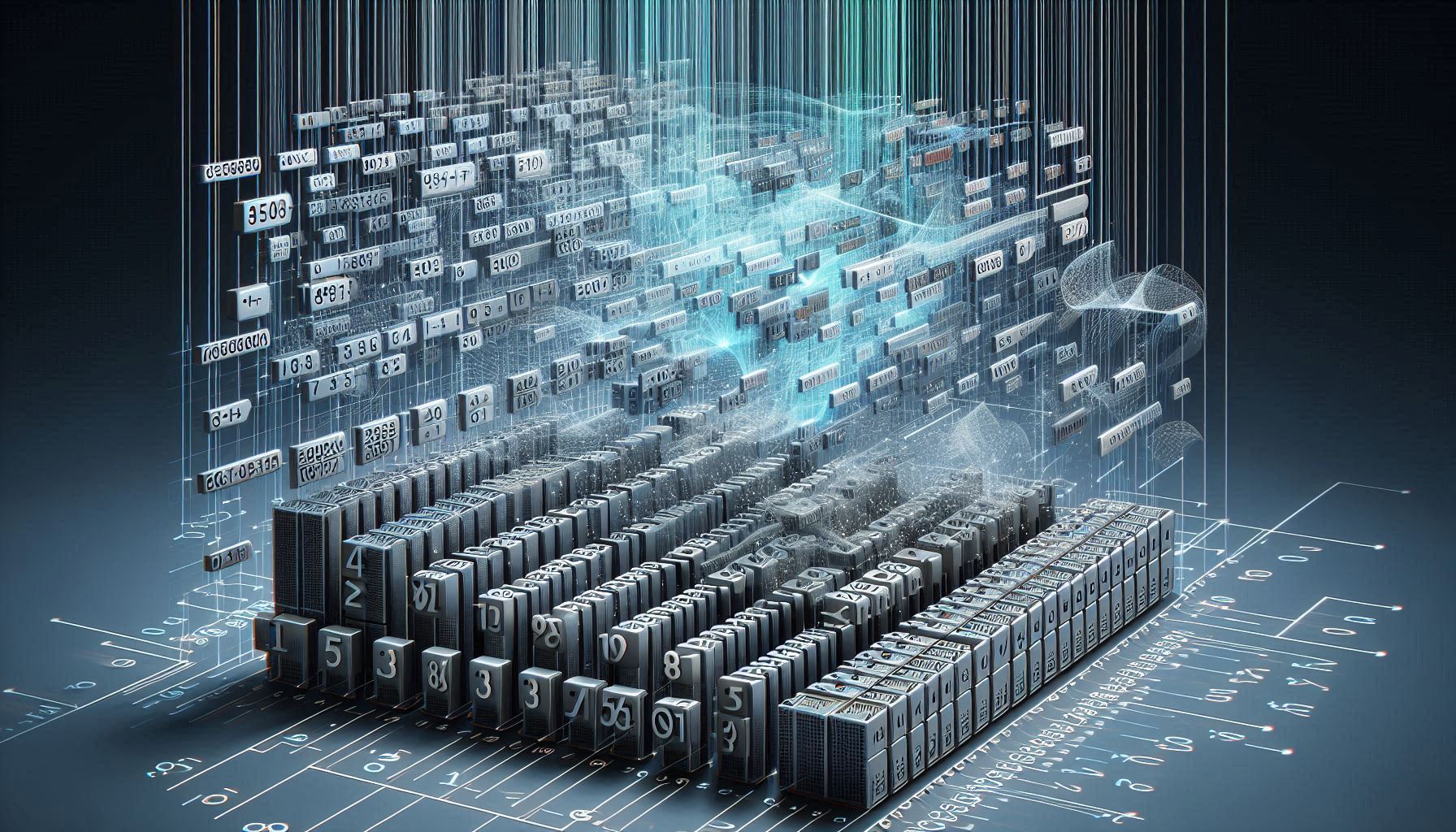
Have you ever wondered how to solve large-scale problems by breaking them down into smaller, more manageable pieces? That’s what Divide and Conquer is all about! In this blog, you’ll not only learn how Divide and Conquer works, but you’ll also apply it yourself using two famous algorithms: Mergesort and Quicksort. Let’s dive in!
What is Divide and Conquer? ⚔
Divide and Conquer is a strategy used to solve complex problems efficiently by breaking them into smaller subproblems, solving each one independently, and then combining the results. Sounds simple, right?
Here’s how it works:
Divide: Break the problem into smaller subproblems.
Conquer: Solve the subproblems recursively (or iteratively).
Combine: Merge the solutions of the subproblems to solve the original problem.
Real-World Application #1: Sorting Massive Data with Mergesort 📊
Sorting is one of the most common tasks in computer science, especially with large datasets. Imagine you have to sort billions of records, like user profiles or product reviews. How can you efficiently handle such massive data?
That’s where Mergesort shines. Here’s why:
Time Complexity: Mergesort has a time complexity of O(n log n), which means it can handle even large datasets in a scalable manner.
Stable Sort: It maintains the relative order of records with equal keys, making it useful for sorting complex data structures.
How Mergesort Works:
Divide: Split the array into two halves.
Conquer: Recursively sort each half.
Combine: Merge the two sorted halves into a single sorted array.
Code snippet to try merge sort on your own:
void merge(int arr[], int left, int mid, int right) {
int n1 = mid - left + 1;
int n2 = right - mid;
int L[n1], R[n2];
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
Real-World Application #2: Efficient Sorting with Quicksort ⚡
If speed is your priority, Quicksort might be the go-to algorithm. Quicksort uses the Divide and Conquer technique but in a slightly different way from Mergesort. It works by choosing a "pivot" element and rearranging the array such that elements smaller than the pivot go to one side and larger elements to the other.
Here’s why Quicksort is often preferred:
In-Place Sorting: Unlike Mergesort, which requires extra space for merging, Quicksort sorts the array in place.
Average Time Complexity: O(n log n), but with a much smaller constant factor, making it faster in practice for many datasets.
How Quicksort Works:
Divide: Pick a pivot and partition the array around it.
Conquer: Recursively apply Quicksort to the subarrays.
Combine: Since the array is already sorted, no actual merging is required.
Code snippet to try quick sort on your own:
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Scalability in the Real World 🌍
Divide and Conquer is not just limited to sorting algorithms. It’s used in various real-world applications where scalability is crucial:
Image Processing: Large images are split into smaller sections, processed individually, and then merged back.
Parallel Computing: Tasks are divided across multiple processors to reduce computational time.
Binary Search: A simpler form of Divide and Conquer used in searching sorted datasets with O(log n) time complexity.
Imagine This:
You’re working at a tech company that handles millions of transactions every day. Sorting, searching, and processing this massive data efficiently is key. Which algorithm would you use—Mergesort or Quicksort? Why?
Hint: Consider whether stability, space, or speed is more important!
A Quick Recap 📝
By now, you should have a good understanding of how Divide and Conquer works, especially through Mergesort and Quicksort. Here’s a quick summary of when to use them:
Mergesort: When stability and guaranteed performance (O(n log n)) are essential, especially for large datasets.
Quicksort: When in-place sorting and speed are more critical, with an average time complexity of O(n log n).
If you enjoyed this post, feel free to like and leave a comment!
Happy Coding! ✨
Subscribe to my newsletter
Read articles from Musab Rayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
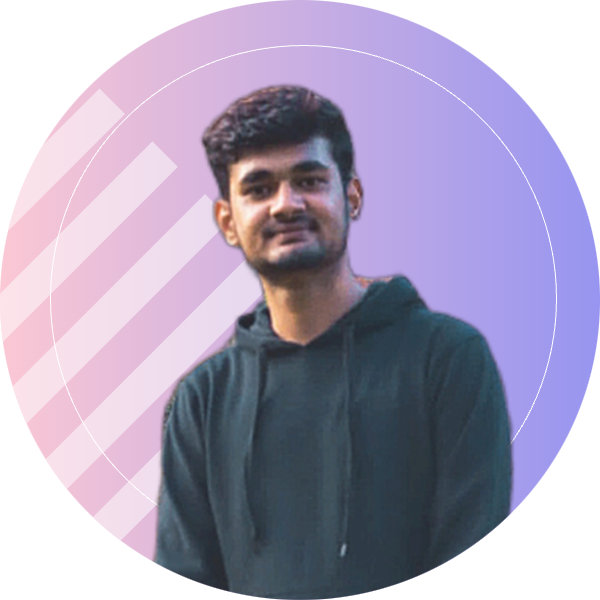
Musab Rayan
Musab Rayan
Hello, I'm Musab Rayan, a third-year CSE student at BSA Crescent Institute of Science and Technology. I love building websites! Since I was a kid, I've been curious about how they work, and now that curiosity drives me into the exciting world of tech. Currently honing my skills in DSA, I aspire to carve a successful career as a software engineer with a focus on innovation.