Mastering Regular Expressions (Regex) and Using Them Effectively in PHP
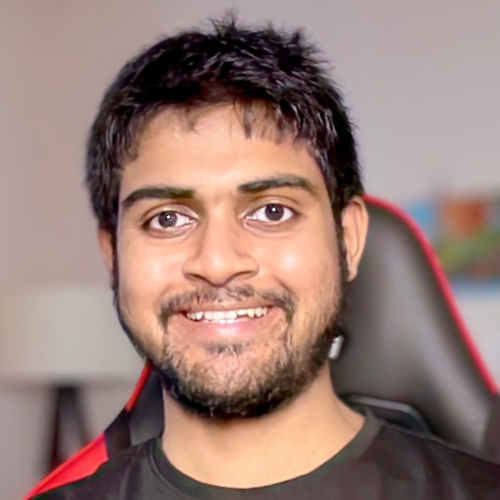
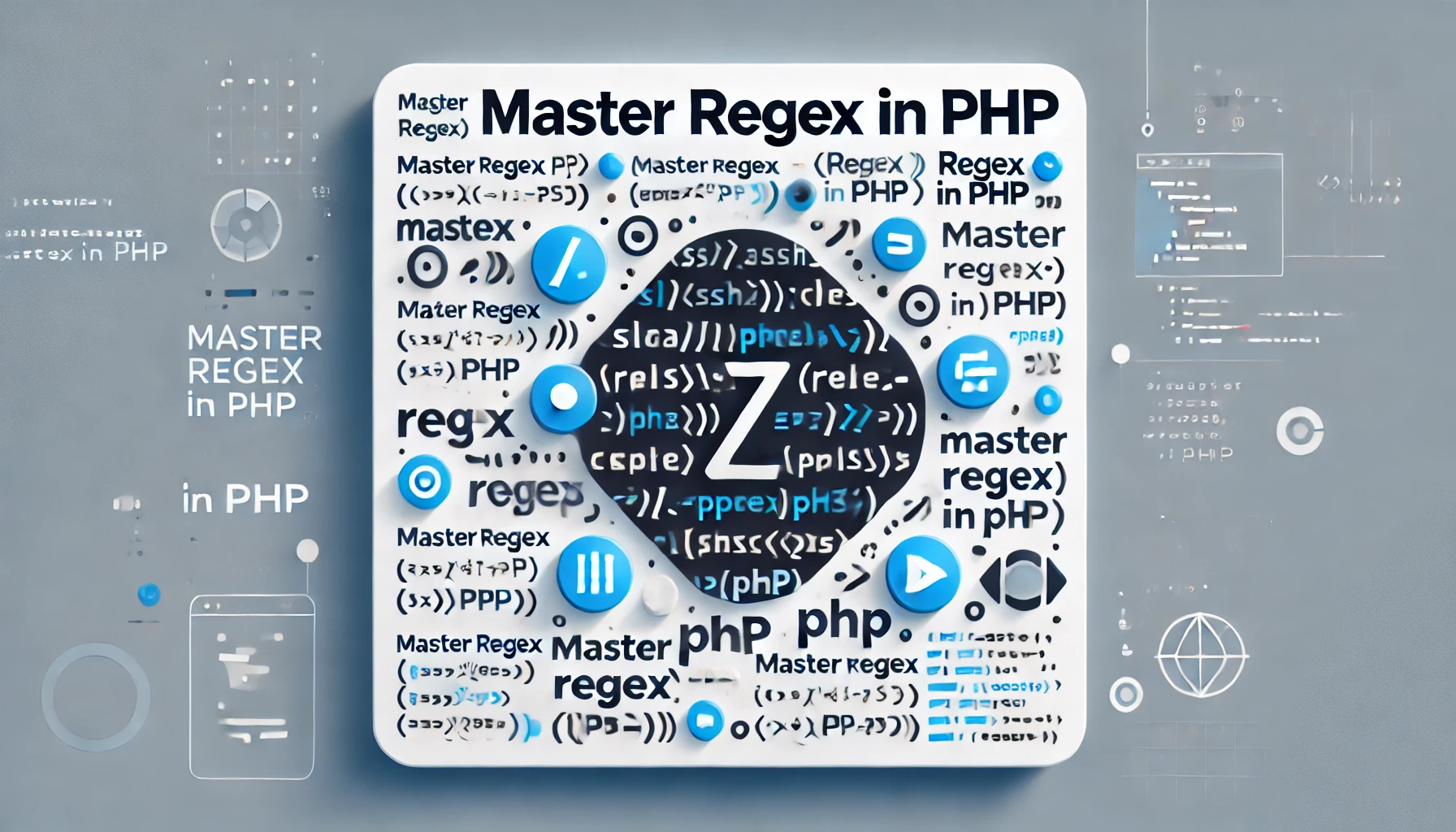
Introduction
Regular expressions (Regex) are essential tools in programming that enable developers to search for patterns within strings efficiently. Whether you’re working on form validations, search functionalities, or data extraction, regex offers unmatched versatility in handling text-based data.
In this comprehensive guide, we'll start by exploring the basic concepts of regular expressions and then dive into using them effectively in PHP with real-world examples. By the end, you’ll be well-equipped to master regex in your PHP applications.
Table of Contents
What is Regex?
Common Regex Syntax and Concepts
Anchors
Character Classes
Predefined Character Classes
Quantifiers
Grouping and Alternation
Escape Characters
Advanced Regex Concepts
Lookaheads and Lookbehinds
Greedy vs. Lazy Matching
Backreferences
Using Regex in PHP
Introduction to PHP Regex Functions
preg_match()
preg_match_all()
preg_replace()
preg_split()
Real-world Examples of Regex in PHP
Email Validation
Extracting Numbers
Phone Number Validation
URL Validation
Removing Whitespace
Optimizing Regex for Performance
Debugging and Testing Regex Patterns
Conclusion
1. What is Regex?
A regular expression (regex) is a string of characters that forms a search pattern. This pattern can be used to perform all types of text searches and text replacements. Regular expressions are often used for input validation, data extraction, and text parsing.
Think of regex as a powerful search tool. Instead of looking for specific words or characters, you can define patterns that represent those words or characters and search for them in large strings of text.
Regex in a Nutshell:
Literal characters are characters that match themselves. For example,
hello
will match the exact string "hello" wherever it appears.Metacharacters are characters that hold special meanings, such as
.
or*
, which help you define complex search patterns.
Let’s break down some key components of regex.
2. Common Regex Syntax and Concepts
Anchors:
Anchors are used to match positions within a string rather than specific characters.
^
– Matches the beginning of a string.- Example:
^hello
will match the word "hello" only if it appears at the beginning of the string.
- Example:
if (preg_match("/^hello/", "hello world")) {
echo "Match!";
}
$
– Matches the end of a string.- Example:
world$
will match the word "world" only if it appears at the end of the string.
- Example:
if (preg_match("/world$/", "hello world")) {
echo "Match!";
}
Character Classes:
Character classes allow you to define a set of characters to match.
.
– Matches any single character except for newline characters.- Example:
h.llo
will match "hello", "hallo", "hullo", etc.
- Example:
[abc]
– Matches any single character inside the brackets.- Example:
[aeiou]
will match any vowel in a string.
- Example:
if (preg_match("/h[aeiou]llo/", "hello")) {
echo "Match!";
}
[^abc]
– Matches any character that is not inside the brackets.- Example:
[^aeiou]
will match any consonant.
- Example:
if (preg_match("/[^aeiou]/", "hello")) {
echo "Match!";
}
Predefined Character Classes:
Some character classes are predefined for convenience. Here are the most common ones:
\d
– Matches any digit (equivalent to[0-9]
).- Example:
\d{3}
will match exactly three digits.
- Example:
\D
– Matches any non-digit character.\w
– Matches any word character (letters, digits, or underscores).\W
– Matches any non-word character.\s
– Matches any whitespace character (spaces, tabs, line breaks).\S
– Matches any non-whitespace character.
Quantifiers:
Quantifiers allow you to specify how many times a character or group should be matched.
*
– Matches 0 or more occurrences of the preceding element.Example:
a*
matches "a", "aa", "aaa", or "" (empty string).+
– Matches 1 or more occurrences.Example:
a+
matches "a", "aa", "aaa", but not "" (empty string).?
– Matches 0 or 1 occurrence.Example:
a?
matches either "a" or "".{n}
– Matches exactlyn
occurrences.Example:
\d{3}
matches exactly three digits.{n,}
– Matchesn
or more occurrences.Example:
a{2,}
matches "aa", "aaa", "aaaa", etc.{n,m}
– Matches betweenn
andm
occurrences.Example:
\d{2,4}
will match between two and four digits.
Grouping and Alternation:
Grouping is used to group parts of a regex pattern together.
(abc)
– Groups characters into a single unit.Example:
(abc){2}
will match "abcabc".|
– Acts as an OR operator.Example:
hello|world
will match either "hello" or "world".
Escape Characters:
Some characters have special meanings in regex. To match these characters literally, you need to escape them with a backslash (\
).
- Example:
\.
matches a literal period instead of the "any character" pattern.
3. Advanced Regex Concepts
Lookaheads and Lookbehinds:
Lookaheads and lookbehinds allow you to search for patterns that are preceded or followed by another pattern without including the surrounding characters in the match.
Positive Lookahead (
?=
): Matches a group only if it is followed by another group.Example:
foo(?=bar)
matches "foo" only if it is followed by "bar".Negative Lookahead (
?!
): Matches a group only if it is not followed by another group.Example:
foo(?!bar)
matches "foo" only if it is not followed by "bar".
Greedy vs. Lazy Matching:
Greedy Matching: By default, quantifiers are greedy, meaning they match as many characters as possible.
Example: In the pattern
a.*b
, the.*
will match as many characters as possible, resulting in the entire string being matched.Lazy Matching: To make a quantifier lazy (i.e., match as few characters as possible), append a
?
to it.Example: In the pattern
a.*?b
, the.*?
will match as few characters as possible, stopping as soon as the first "b" is found.
Backreferences:
Backreferences allow you to reuse part of the regex pattern.
- Example:
(abc)\1
will match "abcabc", because\1
refers to the content of the first capturing group.
4. Using Regex in PHP
PHP provides several functions for working with regex, which are based on Perl-Compatible Regular Expressions (PCRE). Let's explore these in detail.
preg_match()
The preg_match()
function is used to search a string for a pattern and returns 1
if a match is found, or 0
if no match is found.
$string = "hello world";
if (preg_match("/hello/", $string)) {
echo "Match found!";
} else {
echo "No match found.";
}
preg_match_all()
This function works similarly to preg_match()
, but it returns all matches rather than stopping at the first match.
$string = "apple, banana, orange";
preg_match_all("/\w+/", $string, $matches);
print_r($matches);
preg_replace()
preg_replace()
is used to replace matches of a regex pattern within a string with a replacement string.
$string = "hello world";
$new_string = preg_replace("/world/", "PHP", $string);
echo $new_string; // Output: hello PHP
preg_split()
The preg_split()
function splits a string into an array based on a regex pattern.
$string = "one,two,three";
$array = preg_split("/,/", $string);
print_r($array);
5. Real-world Examples of Regex in PHP
Email Validation
Validating email addresses is one of the most common uses of regex.
$email = "test
6. Optimizing Regex for Performance
While regex is incredibly powerful, poorly written regular expressions can lead to performance issues, especially when working with large datasets or complex patterns. Here are some tips for optimizing regex performance in PHP:
1. Use Lazy Quantifiers When Appropriate
As mentioned earlier, quantifiers like *
and +
are greedy by default, meaning they match as much of the string as possible. In certain cases, this behavior can slow down your regex processing. Instead, use lazy quantifiers (*?
, +?
) to match only as much as needed.
Example:
// Greedy quantifier
preg_match("/<.*>/", $string); // Matches the longest possible string within the tags.
// Lazy quantifier
preg_match("/<.*?>/", $string); // Matches the shortest string within the tags.
2. Avoid Excessive Backtracking
Backtracking occurs when the regex engine tries multiple combinations of characters to find a match. Patterns with too much backtracking can slow down the matching process. Using explicit quantifiers and lazy matching can reduce the amount of backtracking.
Example of potential backtracking issues:
// This regex pattern can cause excessive backtracking
preg_match("/(a+)+b/", $string); // Matching "aaaaa" before "b" leads to heavy backtracking.
3. Anchor Your Patterns
If you know where a match should occur within the string (beginning or end), use the ^
(start of string) and $
(end of string) anchors. Anchoring your patterns can reduce the number of unnecessary comparisons the regex engine makes, improving overall performance.
Example:
// Anchored pattern for a better performance
preg_match("/^hello/", $string); // Only checks for "hello" at the start of the string.
4. Precompile Your Regex Patterns
If you're using the same regular expression repeatedly, it's a good idea to precompile the pattern. In PHP, the regular expressions are automatically compiled into a pattern tree, but you can further optimize by avoiding dynamic pattern generation during runtime.
5. Limit Your Use of Alternation (|
)
While alternation (|
) is a powerful feature of regex, it can slow down the matching process, especially when used with complex expressions. Instead, try to refactor your pattern to avoid excessive alternation.
Example of inefficient alternation:
// Multiple alternations can be costly
preg_match("/apple|orange|banana|grape/", $string);
A more efficient way might be to group common patterns:
preg_match("/(apple|orange|banana|grape)/", $string);
6. Use Atomic Groups for Complex Patterns
Atomic groups prevent backtracking inside the group once a match is made, improving performance in certain cases where backtracking would otherwise occur.
// Use atomic groups to prevent backtracking
preg_match("/(?>a+)\w/", $string);
Atomic groups are a great way to optimize complex regex patterns where unwanted backtracking may occur.
7. Debugging and Testing Regex Patterns
Creating regular expressions can sometimes be tricky, and debugging them can take time. Here are several best practices and tools to help you debug and test your regex patterns effectively.
1. Use Online Regex Testers
There are several online tools available that allow you to test and debug your regular expressions interactively. These tools provide instant feedback and allow you to see how your pattern behaves with different inputs. Some popular ones include:
regex101 – This tool provides real-time explanations, match highlighting, and pattern analysis for PCRE, JavaScript, and Python regex.
RegExr – A user-friendly tool with helpful documentation, a library of patterns, and real-time testing.
2. Use PHP Debugging Functions
In PHP, the preg_last_error()
function can be used to check if a regular expression has caused an error during the matching process. The function returns error constants like PREG_NO_ERROR
, PREG_INTERNAL_ERROR
, or PREG_BACKTRACK_LIMIT_ERROR
.
Example:
if (preg_match("/(abc/", $string) === false) {
$error = preg_last_error();
echo "Regex Error: " . $error;
}
3. Break Down Complex Patterns
When working with complex patterns, it’s helpful to break them down into smaller components. You can test individual parts of the pattern separately before combining them into a larger expression.
Example: If you’re writing a complex email validation pattern, start by testing the domain-matching part first, then add the local part and other elements incrementally.
// Test domain pattern first
preg_match("/@[a-zA-Z]+\.[a-zA-Z]{2,}/", $email);
4. Use Comments for Readability
You can use inline comments in your regular expressions to make them easier to understand, especially for complex patterns. PHP allows the use of x
mode (extended) which allows whitespace and comments inside the pattern.
Example:
// Adding comments and spaces for better readability
$pattern = '/
^[a-zA-Z0-9._%+-]+ # Local part of the email
@ # At symbol
[a-zA-Z0-9.-]+ # Domain name
\.[a-zA-Z]{2,6}$ # Top-level domain
/x';
preg_match($pattern, $email);
5. Test with a Variety of Inputs
To ensure your regex works as expected, test it with a wide variety of inputs, including edge cases. For example, if you're writing a regex to validate phone numbers, test different formats like:
Valid inputs:
123-456-7890
,(123) 456-7890
,123 456 7890
Invalid inputs:
12-3456-7890
,1234567890
,abc-123-defg
6. Debugging with preg_replace()
One handy trick for debugging is using preg_replace()
to highlight the matches. Instead of just checking if something matches, replace the matched portions with a visual marker, such as [MATCH]
. This lets you see exactly what parts of your string are being matched.
Example:
$string = "The quick brown fox jumps over 12 lazy dogs.";
$result = preg_replace("/\w+/", "[MATCH]", $string);
echo $result; // Outputs: [MATCH] [MATCH] [MATCH] [MATCH] [MATCH] [MATCH] [MATCH] [MATCH] [MATCH]
Conclusion
Understanding and mastering regular expressions is a crucial skill for any PHP developer. This guide provided you with a deep dive into regex, from basic syntax to advanced concepts, along with their practical applications in PHP. Regex can seem daunting at first, but with practice, you'll be able to write efficient, optimized patterns that handle complex string manipulation with ease.
By following best practices such as optimizing for performance, avoiding unnecessary backtracking, and using tools for debugging, you can create efficient, maintainable regular expressions. Whether you're validating form inputs, parsing text, or cleaning data, regex will be a valuable tool in your development toolkit.
With a solid understanding of both basic and advanced regex concepts, you're now prepared to tackle a wide range of real-world problems in PHP. Keep experimenting, testing, and refining your patterns to master regex for even the most complex text processing tasks.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
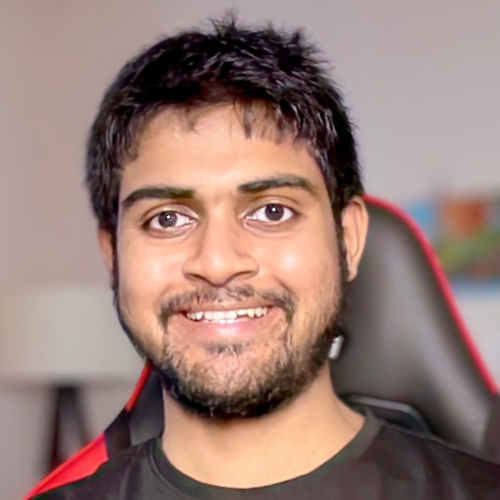
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.