"Java Basics Unveiled: A Comprehensive Guide to Variables, Data Types, and Basic Operations"?
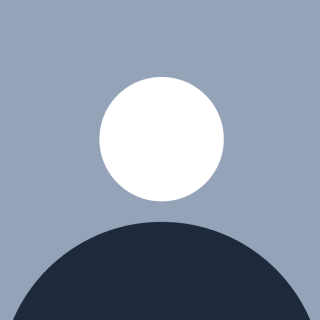
Chapter 1: Variables and Data Types in Java
Java is a powerful programming language widely used for building various applications. In this chapter, we will explore some fundamental concepts such as creating a Java file, understanding boilerplate code, and working with variables and data types. We'll also cover basic input/output operations, mathematical operations, and assignments.
1. Creating a Java File
To start coding in Java, the first step is to create a Java file. A Java file is simply a text file with the .java
extension. The file name should match the class name. For example, if your class is named Hello_World
, the file should be saved as Hello_
World.java
.
2. Boilerplate Code
Java programs require a specific structure or "boilerplate code" to run. This includes defining a class and the main method, which serves as the entry point of the program.
public class Hello_World {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
Class Declaration: The
public class Hello_World
line declares a class namedHello_World
.Main Method: The
public static void main(String[] args)
method is where the program execution begins. TheSystem.out.println("Hello World!");
statement prints "Hello World!" to the console.
3. Output in Java
The System.out.println()
function is used to print text to the console. For example:
System.out.println("Hello World!");
This will output Hello World!
on the console.
4. Print a Pattern
You can use the System.out.println()
function to print patterns. Here's an example of printing a triangle pattern:
public class PrintTriangle {
public static void main(String[] args) {
System.out.println("****");
System.out.println("***");
System.out.println("**");
System.out.println("*");
}
}
This code will output:
****
***
**
*
5. Variables in Java
Variables are used to store data in a Java program. You can declare and initialize variables like this:
public class Variable {
public static void main(String[] args) {
int a = 5;
int b = 9;
System.out.println(a); // Output: 5
String name = "Rohit Gawande";
System.out.print(name); // Output: Rohit Gawande
}
}
int: Stores integers (whole numbers) without decimals.
String: Stores a sequence of characters, like a word or sentence.
6. Data Types in Java
Java is a statically typed language, meaning each variable must have a data type.Java has various data types to store different kinds of data:
Primitive Data Types: These include
int
(integer),float
(floating-point number),char
(character),boolean
(true/false), etc.Non-Primitive Data Types: These include
String
, arrays, classes, etc.
Here's a summary of primitive data types:
Data Type | Description | Example |
int | Integer | 5 |
float | Floating-point number | 5.99f |
char | Character | 'A' |
boolean | True/False | true |
7. Sum of Two Numbers
Let's write a simple program to calculate the sum of two numbers:
public class Sum {
public static void main(String[] args) {
int a = 8;
int b = 7;
int sum = a + b;
System.out.println(sum); // Output: 15
}
}
8. Comments in Java
Comments are used to explain code and are ignored by the compiler. There are two types:
Single-line comments: Start with
//
Multi-line comments: Enclosed in
/* */
Example:
// This is a single-line comment
/* This is a
multi-line comment */
9. Input in Java
Java uses the Scanner
class to take input from the user. Here's how you can use it:
import java.util.Scanner;
public class InputSum {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a:");
int a = sc.nextInt();
System.out.println("Enter b:");
int b = sc.nextInt();
int sum = a + b;
System.out.println("The sum of a and b: " + sum);
}
}
Scanner sc = new Scanner(
System.in
);
initializes the scanner to take input from the user.nextInt()
reads an integer from the user.
10. Product of Two Numbers
Similarly, you can calculate the product of two numbers using input from the user:
import java.util.Scanner;
public class Prod {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a:");
int a = sc.nextInt();
System.out.println("Enter b:");
int b = sc.nextInt();
int mul = a * b;
System.out.println("The product of a and b: " + mul);
}
}
11. Area of a Circle
To calculate the area of a circle, you can use the formula ฯr^2
where r
is the radius:
import java.util.Scanner;
public class CircleArea {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter radius:");
float radius = sc.nextFloat();
float area = 3.14f * radius * radius;
System.out.println("Area of Circle: " + area);
}
}
12. Type Conversion
Type conversion allows converting one data type to another. For example:
int a = 10;
float b = a; // Automatic type conversion from int to float
13. Type Casting
Type casting is explicitly converting one data type to another, like converting a float to an int:
public class TypeCasting {
public static void main(String[] args) {
int a = 10;
float b = 5.0f;
int c = (int) b; // Narrowing conversion from float to int
System.out.println(c); // Output: 5
}
}
14. Type Promotion in Expressions
Java automatically promotes lower data types to higher ones in expressions. For example:
public class TypePromotion {
public static void main(String[] args) {
byte b = 42;
char c = 'a';
short s = 1024;
int i = 50000;
float f = 5.67f;
double d = .1234;
double result = (f * b) + (i / c) - (d * s);
System.out.println(result);
}
}
In this example, all smaller data types are promoted to the largest type in the expression, which is double
.
15. How Does Java Code Run?
Java code goes through several stages to execute:
Writing Code: Code is written in a
.java
file.Compilation: The Java compiler (
javac
) converts the code into bytecode, a platform-independent code stored in a.class
file.Execution: The Java Virtual Machine (JVM) executes the bytecode on the platform.
Assignments
- Calculate the Average of Three Numbers:
import java.util.Scanner;
public class Q1 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a:");
int a = sc.nextInt();
System.out.println("Enter b:");
int b = sc.nextInt();
System.out.println("Enter c:");
int c = sc.nextInt();
int average = (a + b + c) / 3;
System.out.println("The Average of 3 numbers is: " + average);
}
}
- Calculate the Area of a Square:
import java.util.Scanner;
public class Q2 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter side:");
float side = sc.nextFloat();
float area = side * side;
System.out.println("Area of Square is: " + area);
}
}
- Calculate the Total Cost of Items:
import java.util.Scanner;
public class Q3 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the price of Pen:");
float pen = sc.nextFloat();
System.out.println("Enter the price of Pencil:");
float pencil = sc.nextFloat();
System.out.println("Enter the price of Eraser:");
float eraser = sc.nextFloat();
float total = pen + pencil + eraser;
System.out.println("Bill: " + total);
float totalGST = total + (0.18f * total);
System.out.println("Bill with GST: " + totalGST);
}
}
Conclusion:
In this chapter, we covered the basics of Java, including creating Java files, understanding the boilerplate code, working with variables and data types, and solving basic problems using these concepts. Mastering these fundamentals is essential as you move forward in your journey of learning Java with DSA.
Stay tuned for the next chapter, where weโll dive deeper into more complex topics and continue building our Java programming skills. Happy coding!
Rohit Gawande
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
๐ Tech Enthusiast | Full Stack Developer | System Design Explorer ๐ป Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iโm Rohit Gawande! ๐I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iโm Currently Doing ๐น Writing an in-depth System Design Series to help developers master complex design concepts.๐น Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.๐น Exploring advanced Java concepts and modern web technologies. What You Can Expect Here โจ Detailed technical blogs with examples, diagrams, and real-world use cases.โจ Practical guides on Java, System Design, and Full Stack Development.โจ Community-driven discussions to learn and grow together. Letโs Connect! ๐ GitHub โ Explore my projects and contributions.๐ผ LinkedIn โ Connect for opportunities and collaborations.๐ LeetCode โ Check out my problem-solving journey. ๐ก "Learning is a journey, not a destination. Letโs grow together!" Feel free to customize or add more based on your preferences! ๐