Day-47: Terraform Variables
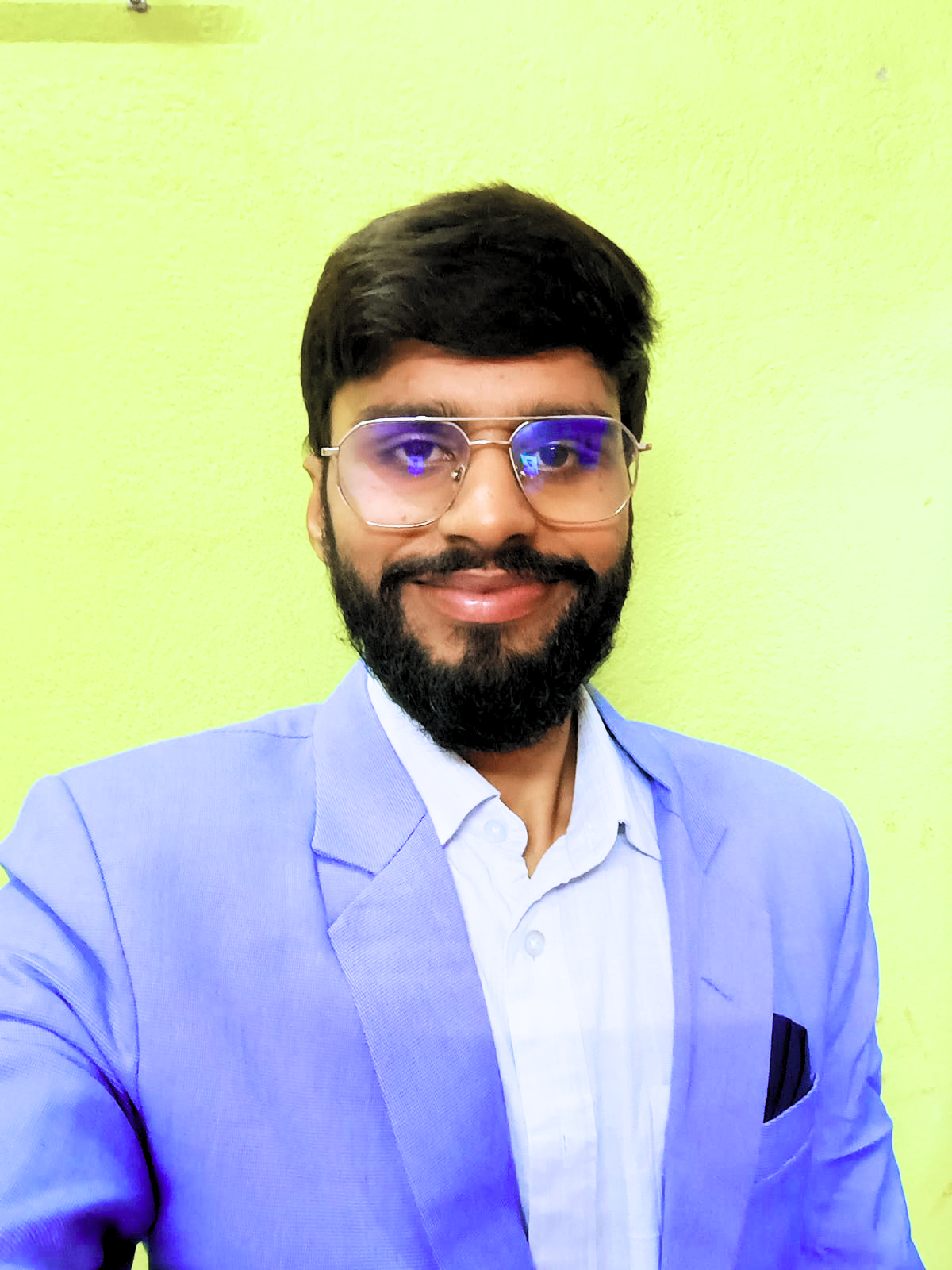
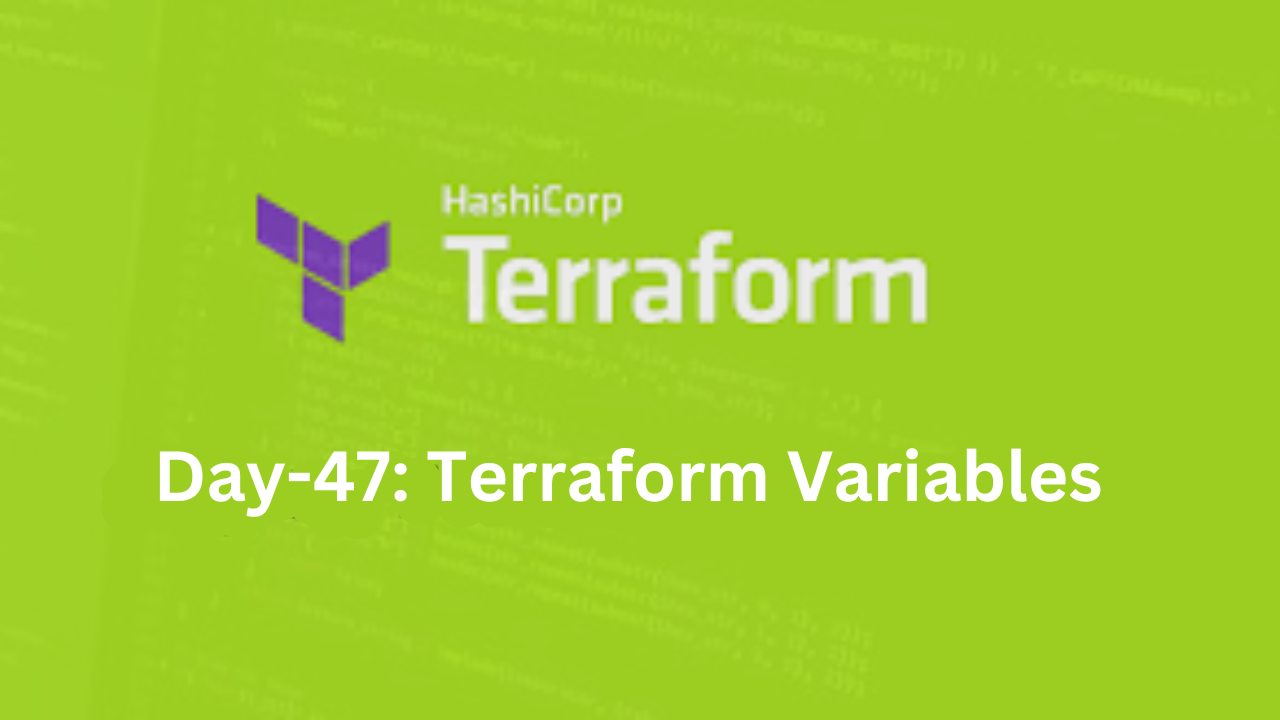
Terraform is a powerful tool for infrastructure as code (IaC) that allows you to define and manage cloud resources efficiently. One of the key concepts in Terraform is the use of variables. In this blog, we'll explore what variables are, the types of variables, and their importance. We'll also provide examples and compare how variables are used in different files.
What is a Variable?
A variable in Terraform is a placeholder for a value that you can define and reuse throughout your configuration. This allows you to customize your Terraform scripts without hardcoding values, making your code more flexible and easier to manage.
Real-Life Example:
Imagine you are setting up a web server. Instead of hardcoding the server type (e.g., t2.micro
), you can define it as a variable. This way, you can easily change it later without modifying your entire configuration.
Types of Variables
Terraform supports different types of variables. Here are the main types:
String Variables:
Used for text values.
Example:
variable "instance_type" { description = "Type of the EC2 instance" type = string default = "t2.micro" }
Number Variables:
Used for numerical values.
Example:
variable "instance_count" { description = "Number of EC2 instances" type = number default = 2 }
Boolean Variables:
Used for true/false values.
Example:
variable "enable_logging" { description = "Enable logging for the instance" type = bool default = true }
List Variables:
Used for a list of values.
Example:
variable "availability_zones" { description = "List of availability zones" type = list(string) default = ["us-east-1a", "us-east-1b"] }
Map Variables:
Used for key-value pairs.
Example:
variable "tags" { description = "Tags for the resources" type = map(string) default = { Name = "MyWebServer" Environment = "Development" } }
Creating a Simple File Locally
To demonstrate the use of variables, let's create a simple Terraform configuration. First, create two files: main.tf
and variables.tf
.
variables.tf
This file defines our variables.
variable "instance_type" {
description = "Type of the EC2 instance"
type = string
default = "t2.micro"
}
variable "instance_count" {
description = "Number of EC2 instances"
type = number
default = 2
}
main.tf
This file uses the defined variables.
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "web_server" {
count = var.instance_count
instance_type = var.instance_type
ami = "ami-12345678" # Replace with a valid AMI ID
}
How to Run
Initialize Terraform: Run the following command in your terminal:
terraform init
Plan the Deployment: Preview the changes:
terraform plan
Apply the Changes: Create the resources:
terraform apply
Why We Use Variables
Using variables in Terraform offers several advantages:
Flexibility: You can easily change configurations without modifying the main codebase.
Reusability: Variables can be reused in multiple resources, reducing duplication.
Clarity: Variables can make your code clearer by using descriptive names for resources and settings.
Parameterization: It allows you to parameterize your configuration, making it adaptable to different environments (e.g., development, staging, production).
Comparison: main.tf
vs. variables.tf
variables.tf
: Contains the definition of variables. It specifies what inputs the user can provide, along with default values.main.tf
: Contains the resource definitions that use the variables defined invariables.tf
. It describes how the infrastructure should be provisioned based on the values provided.
Example Comparison
In variables.tf
, you define what type of instance you want to create:
variable "instance_type" {
description = "Type of the EC2 instance"
type = string
default = "t2.micro"
}
In main.tf
, you use that variable to configure the resource:
resource "aws_instance" "web_server" {
instance_type = var.instance_type
}
Conclusion
Understanding variables is crucial for effective Terraform usage. By defining variables, you can create dynamic, reusable, and flexible configurations. Whether you are working on small projects or large-scale infrastructure, using variables can simplify your workflow and enhance the clarity of your code.
By using variables.tf
for definitions and main.tf
for resource deployment, you maintain a clean separation of concerns, making your Terraform code easier to manage and adapt to changing requirements.
Connect and Follow Me on Socials
Subscribe to my newsletter
Read articles from priyadarshi ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
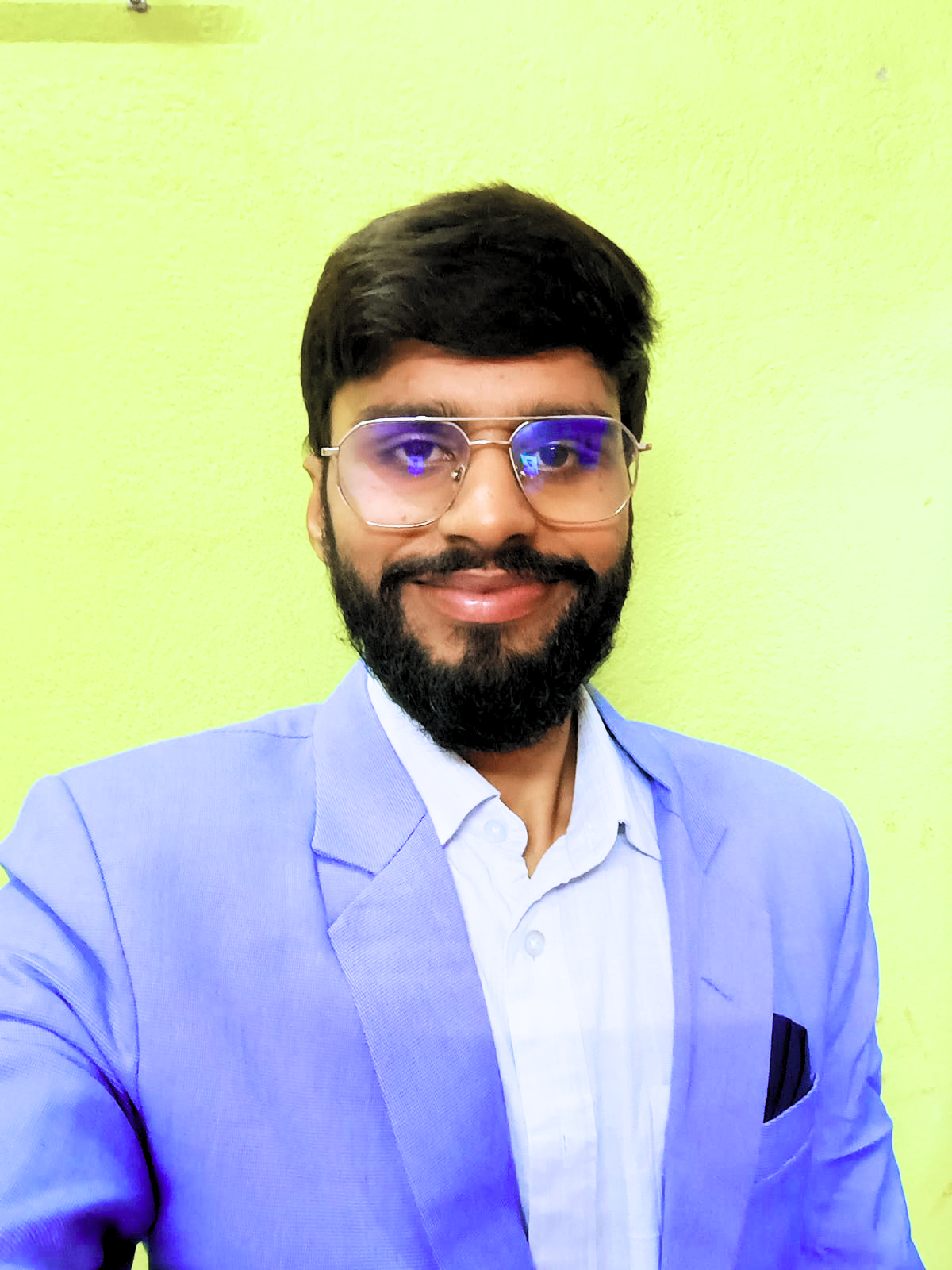
priyadarshi ranjan
priyadarshi ranjan
Greetings! ๐ I'm Priyadarshi Ranjan, a dedicated DevOps Engineer embarking on an enriching journey. Join me as I delve into the dynamic realms of cloud computing and DevOps through insightful blogs and updates. ๐ ๏ธ My focus? Harnessing AWS services, optimizing CI/CD pipelines, and mastering infrastructure as code. Whether you're peers, interns, or curious learners, let's thrive together in the vibrant DevOps ecosystem. ๐ Connect with me for engaging discussions, shared insights, and mutual growth opportunities. Let's embrace the learning curve and excel in the dynamic realm of AWS and DevOps technology!