Understanding var, let, and const in JavaScript

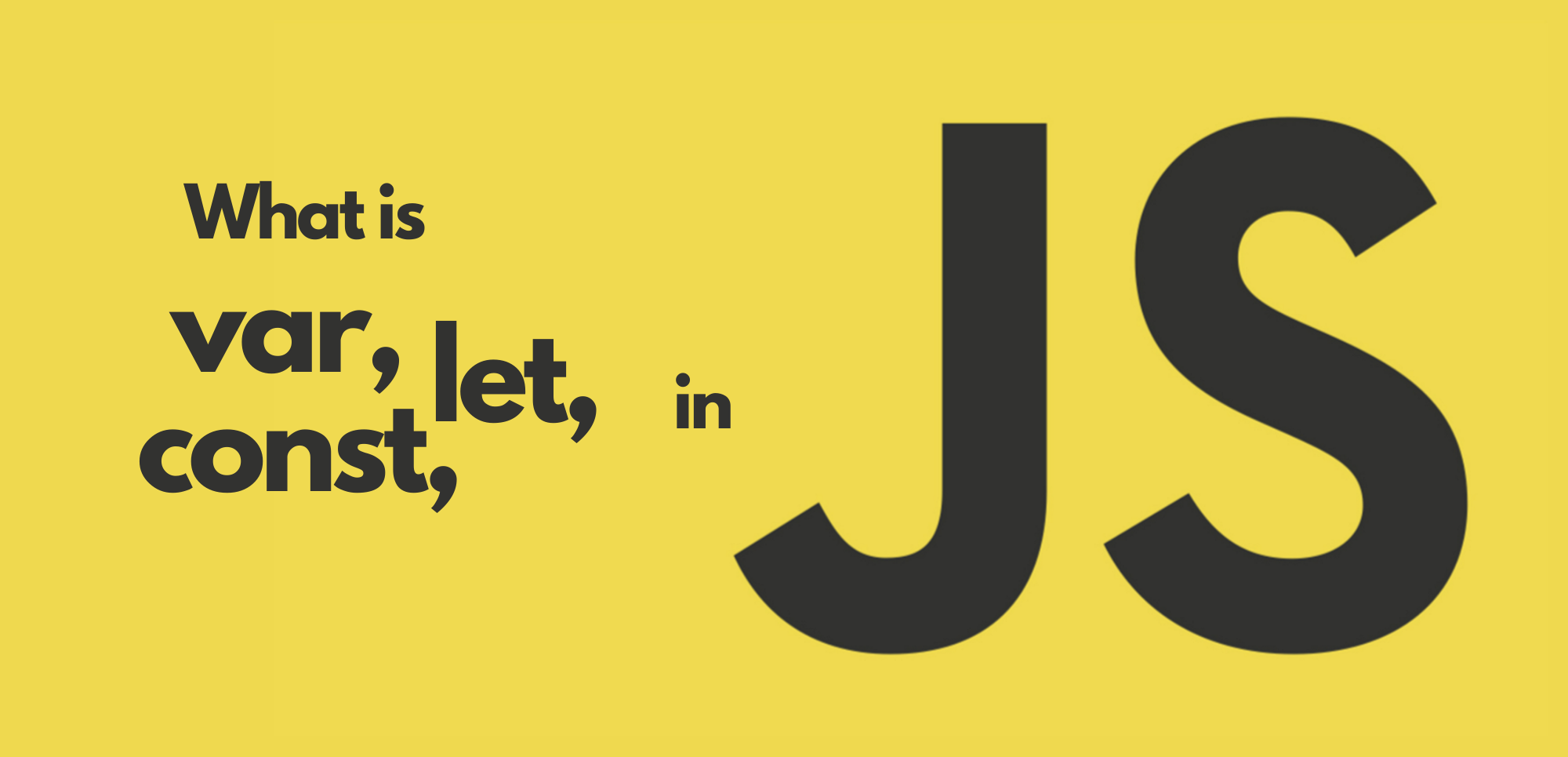
Introduction
JavaScript is one of the most popular programming languages today, and it has changed a lot over time. When JavaScript was first introduced, var
was the only way to declare variables. While it served its purpose, var
had some tricky parts that often led to confusing bugs, especially in larger codebases. To fix these issues, let
and const
is introduced in ES6. These newer keywords brought significant improvements, particularly in terms of scope management and immutability. In this blog post, we’ll explore what makes each of these keywords different, when to use them, and the best ways to use them.
var
: The Original Variable Declaration
var
was the original way to declare variables in JavaScript, and it’s still in use today. However, it comes with a few important characteristics that can sometimes lead to unexpected behavior.
- Function Scope
Variables declared with var
are function-scoped, meaning they are only accessible within the function they are declared in. If declared outside of a function, they are globally scoped.
function example() {
var x = 10;
if (true) {
var x = 20; // same variable
console.log(x); // 20
}
console.log(x); // 20
}
example();
- Hoisting
var
variables are hoisted to the top of their scope, meaning they are accessible even before their declaration in the code. However, the value is not hoisted—only the declaration.
console.log(y); // Output: undefined
var y = 5;
- Redeclaration
A variable declared with var
can be redeclared within the same scope without throwing an error. This can sometimes lead to unintentional overwriting of variables.
var z = 20;
var z = 30; // No error, z is now 30
let
: Block-Scoped Variables
let
was introduced to address some of the issues associated with var
. It is now the preferred way to declare variables that might need to change.
- Block Scope
Unlike var
, variables declared with let
are block-scoped. This means they are only accessible within the block (denoted by {}
) in which they are declared.
if (true) {
let a = 10;
console.log(a); // Output: 10
}
console.log(a); // Error: a is not defined
- No Hoisting
While let
is technically hoisted, it’s not initialized until the variable’s declaration is encountered in the code. This means accessing a let
variable before its declaration will result in a ReferenceError
.
console.log(b); // Error: Cannot access 'b' before initialization
let b = 5;
- No Redeclaration
Variables declared with let
cannot be redeclared in the same scope, which helps prevent accidental overwriting of variables.
let c = 15;
let c = 25; // Error: Identifier 'c' has already been declared
const
: Immutable Variable Declaration
const
is another block-scoped variable declaration introduced in ES6, designed for variables that should not be reassigned after their initial declaration.
- Block Scope
Like let
, const
is block-scoped and is only accessible within the block it is declared in.
if (true) {
const d = 10;
console.log(d); // Outputs: 10
}
console.log(d); // Error: d is not defined
- Immutability
Variables declared with const
cannot be reassigned. However, it’s important to note that while the variable identifier itself is immutable, if the const
variable refers to an object or array, the contents of the object or array can still be changed.
const e = 10;
e = 20; // Error: Assignment to constant variable
const f = [1, 2, 3];
f.push(4); // This is allowed
console.log(f); // Outputs: [1, 2, 3, 4]
- No Redeclaration and No Hoisting
Just like let
, const
variables cannot be redeclared in the same scope, and accessing them before their declaration will result in a ReferenceError
.
const g = 50;
const g = 60; // Error: Identifier 'g' has already been declared
console.log(h); // Error: Cannot access 'h' before initialization
const h = 5;
When to Use var
, let
, or const
Use
var
if you are working on legacy code or if you require function-scoped variables (though this is rare in modern JavaScript).Use
let
when you need a variable that might need to be reassigned.let
is ideal for loop counters, variables that will be updated, or situations where block scope is required.Use
const
when you want to declare a variable that should not be reassigned. This is the best choice for constants, fixed configurations, or values that should remain unchanged throughout the program.
Best Practices
Use
const
by default. It's a signal that the variable won't be reassigned.Use
let
when you know the variable will be reassigned.Avoid
var
in modern JavaScript, unless you're working with older codebases or need function-scoped variables.
Conclusion
By understanding and properly using var
, let
, and const
, you can write cleaner, more predictable JavaScript code. Remember, choosing the right variable declaration is not just about functionality, but also about communicating your intentions to other developers who might read your code.
Subscribe to my newsletter
Read articles from Fahimul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Fahimul Islam
Fahimul Islam
Hey, I'm a fresh CS graduate from a public university from Bangladesh named BSMRSTU. I love to write code in Javascript and Go.