How to Implement Winston for Logging in Node.js
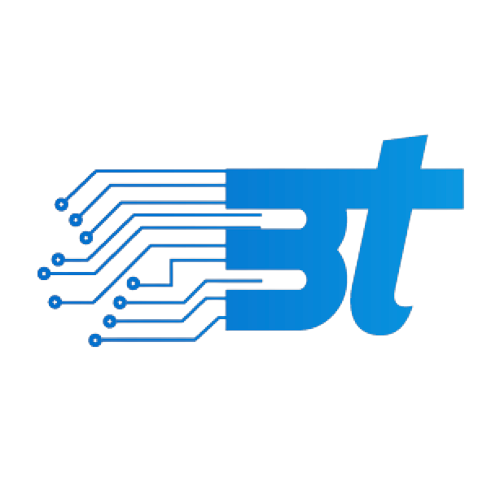
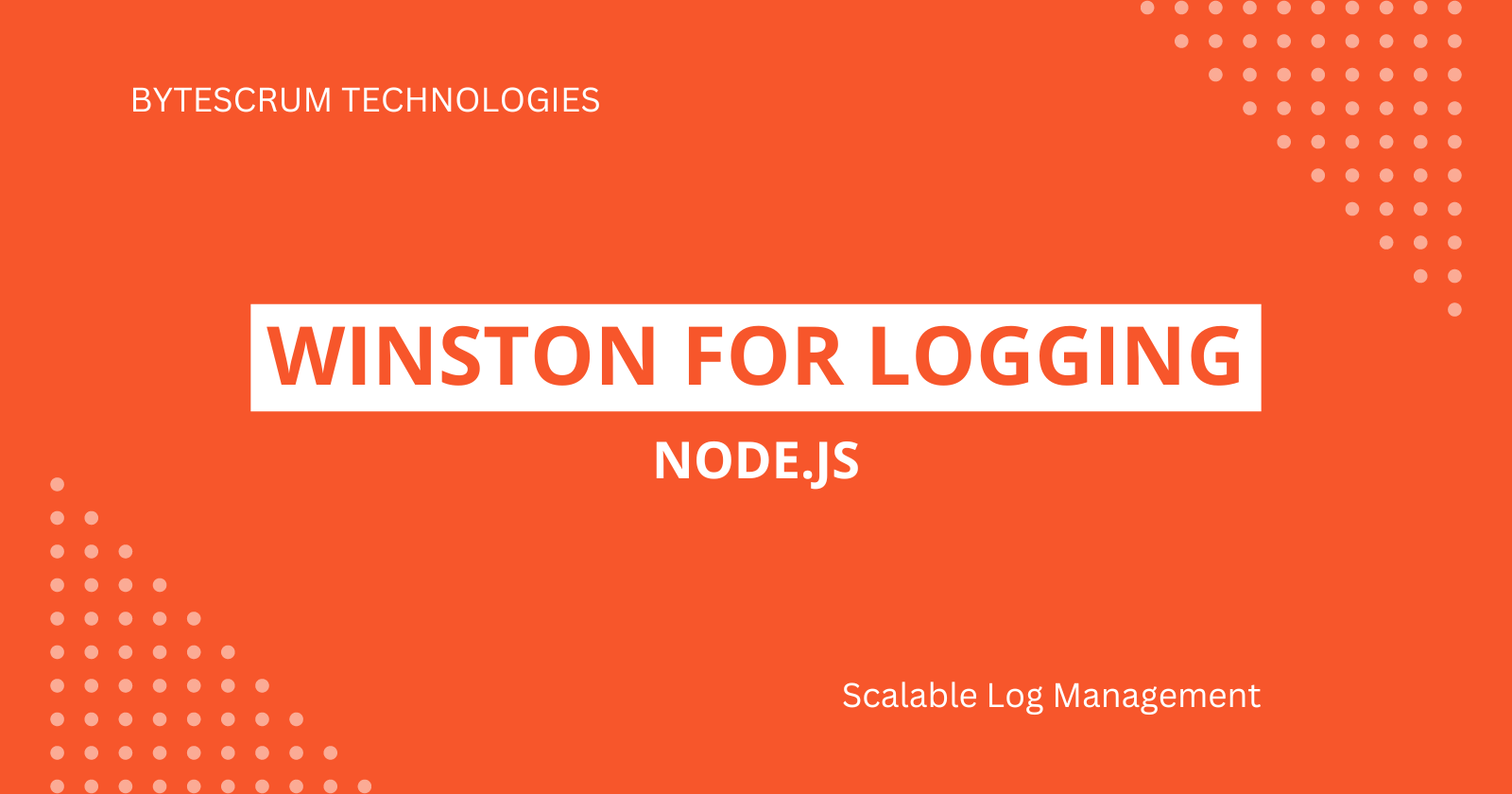
Logging is a crucial aspect of software development, especially in production environments. It helps developers track events, debug issues, and gain insights into their application's performance. In the world of Node.js, one of the most popular logging libraries is Winston.
In this blog, we'll explore Winston's features, usage, and benefits, helping you set up a powerful logging system for your Node.js applications.
What is Winston?
Winston is a versatile, widely used logging library for Node.js that supports multiple logging levels, transports, formats, and customization options. Its flexibility and rich feature set make it a go-to tool for both small applications and large-scale production systems.
Key features include:
Multiple transports: Write logs to different destinations, including files, databases, or third-party services.
Custom formats: Customize how your logs are formatted using simple or complex formats (JSON, strings, etc.).
Log levels: Use different log levels (e.g., info, error, warn, debug) to categorize logs.
Asynchronous logging: Handle large-scale logging efficiently without blocking your application.
Extensibility: Create custom transports and formats for specific use cases.
Why Use Winston for Logging in Node.js?
Node.js has built-in logging with console.log()
, but it's basic and not suitable for advanced use cases like logging to files or third-party services. Here's why Winston is a better choice:
Scalability: Winston handles logs in a structured manner, making it easier to scale as your application grows.
Multiple Output Channels: You can direct logs to different outputs, such as files, databases, or even cloud logging platforms, ensuring that your logs are stored securely and are easy to retrieve.
Flexible Logging Levels: With Winston, you can categorize logs based on severity. This allows you to filter logs based on their importance in production environments.
Customizable Formats: Whether you want your logs in JSON format for easy parsing or in human-readable format for developers, Winston can handle both.
Error Handling: Winston provides options to log uncaught exceptions and unhandled promise rejections, ensuring you don't miss out on crucial error information.
Installation and Basic Setup
Before we dive into Winston's advanced features, let's set it up in a Node.js project. First, install Winston via npm:
npm install winston
Once installed, you can create a basic logger:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'combined.log' })
]
});
// Log an example message
logger.info('Hello, Winston!');
In this example:
We set up two transports: one to log to the console and another to log to a file named
combined.log
.The log level is set to
info
, meaning messages of levelinfo
and higher (likewarn
orerror
) will be logged.
Available Log Levels
Winston supports a default set of log levels, which include:
error
: Logs only critical errors.warn
: Logs potential issues.info
: Logs general information.http
: Logs HTTP requests.verbose
: Logs detailed application-level information.debug
: Logs debugging information.silly
: Logs everything, including very detailed debugging info.
By default, Winston logs messages of the info
level and higher. You can adjust this behavior by setting a different level
value when creating the logger.
Transports: How Winston Handles Output
Winston's transports are what make it so flexible. A transport is essentially a storage device for your logs. You can add multiple transports to log in different formats and locations. Common transports include:
Console: Logs to the terminal or console.
File: Logs to a file (e.g.,
app.log
).HTTP: Sends logs to a remote server over HTTP.
Stream: Logs to a writable stream (like a network socket).
Example: Logging to Console and File Simultaneously
const logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.colorize(),
winston.format.simple()
),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
In this setup:
The
Console
transport prints logs to the terminal.The
File
transports store logs in two files: one for errors (error.log
) and another for all logs (combined.log
).
Formats: Customizing Log Output
Winston allows you to customize the output of your logs with its format option. You can combine multiple formats to tailor the output to your needs. For example, you might want to format logs as JSON in production but use colored, human-readable logs during development.
Simple Example: JSON vs. Pretty Print
const logger = winston.createLogger({
format: winston.format.json(), // JSON format
transports: [
new winston.transports.Console()
]
});
logger.info('This is a JSON formatted log');
To use a simple human-readable format, you can switch to winston.format.simple()
:
const logger = winston.createLogger({
format: winston.format.combine(
winston.format.colorize(),
winston.format.simple()
),
transports: [
new winston.transports.Console()
]
});
logger.info('This is a pretty-printed log');
In this case, colorize()
adds color coding to log levels, and simple()
ensures the logs are printed as plain text.
Timestamp and Label in Logs
Adding timestamps or labels to your logs can make it easier to debug issues or correlate logs across systems.
const logger = winston.createLogger({
format: winston.format.combine(
winston.format.label({ label: 'My App' }),
winston.format.timestamp(),
winston.format.printf(({ level, message, label, timestamp }) => {
return `${timestamp} [${label}] ${level}: ${message}`;
})
),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'app.log' })
]
});
logger.info('This log message includes a timestamp and label');
Here, the timestamp()
format adds the current time, and label()
lets you tag logs with a custom label. printf()
is used to define the output format.
Handling Uncaught Exceptions
Winston can handle uncaught exceptions and unhandled promise rejections, making sure critical errors are logged before the process exits.
const logger = winston.createLogger({
transports: [
new winston.transports.File({ filename: 'uncaughtExceptions.log' })
],
exceptionHandlers: [
new winston.transports.File({ filename: 'exceptions.log' })
]
});
In this setup, if an uncaught exception occurs, it will be logged to the exceptions.log
file.
Profiling with Winston
Winston also allows you to measure the duration of an operation using its built-in profiling feature.
logger.profile('slow operation');
setTimeout(() => {
logger.profile('slow operation'); // End profiling and log duration
}, 1000);
This will log the time taken by the operation and display it in the logs.
Custom Transports
You can create custom transports to extend Winston's functionality. For example, you might want to log messages to a custom API or store them in a database.
const { Transport } = require('winston-transport');
class CustomTransport extends Transport {
log(info, callback) {
// Send the log message to a custom API or storage
console.log('Logging to custom transport:', info.message);
callback();
}
}
const logger = winston.createLogger({
transports: [
new CustomTransport()
]
});
logger.info('Log message to custom transport');
This custom transport logs messages to the console, but you can easily extend it to send logs to an external service.
Conclusion
By combining various transports, formats, and logging levels, you can build a highly customized and reliable logging system tailored to your specific needs. Happy logging!
If you have further questions or want to explore more advanced configurations of Winston, feel free to reach out in the comments or explore the Winston documentation.
Hire us for software development work!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
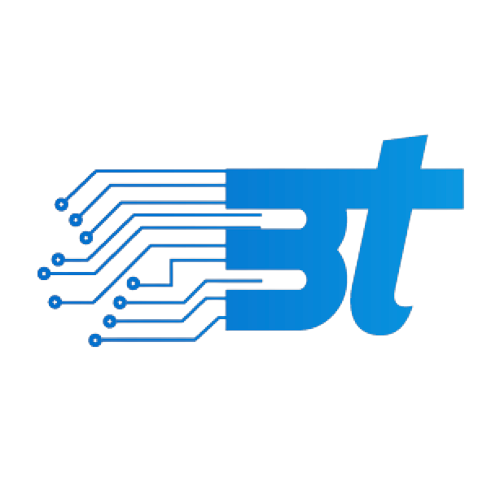
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.