Decentralized Orchestration with Smart Contracts in DevSecOps: Automating and Securing the Deployment Pipeline
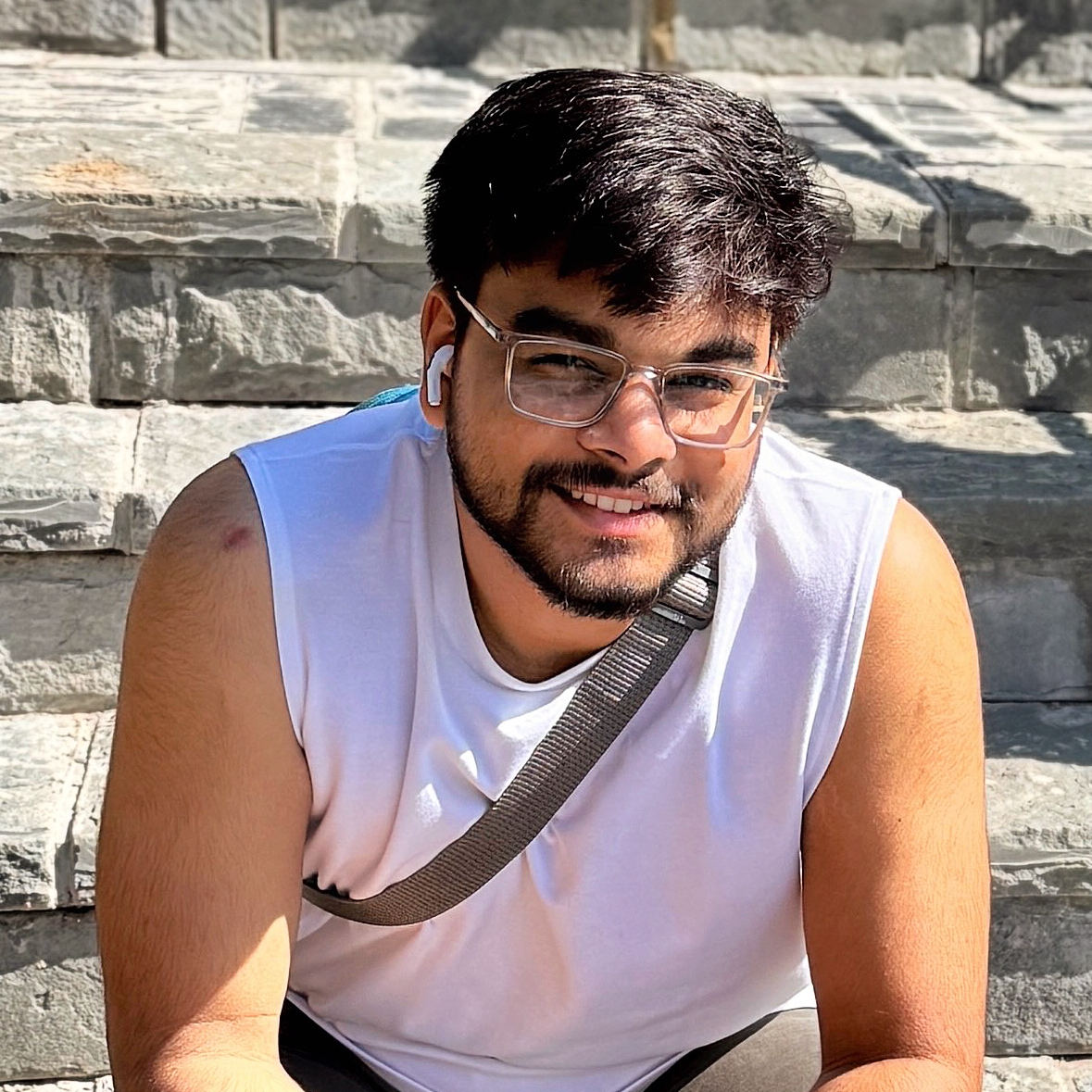
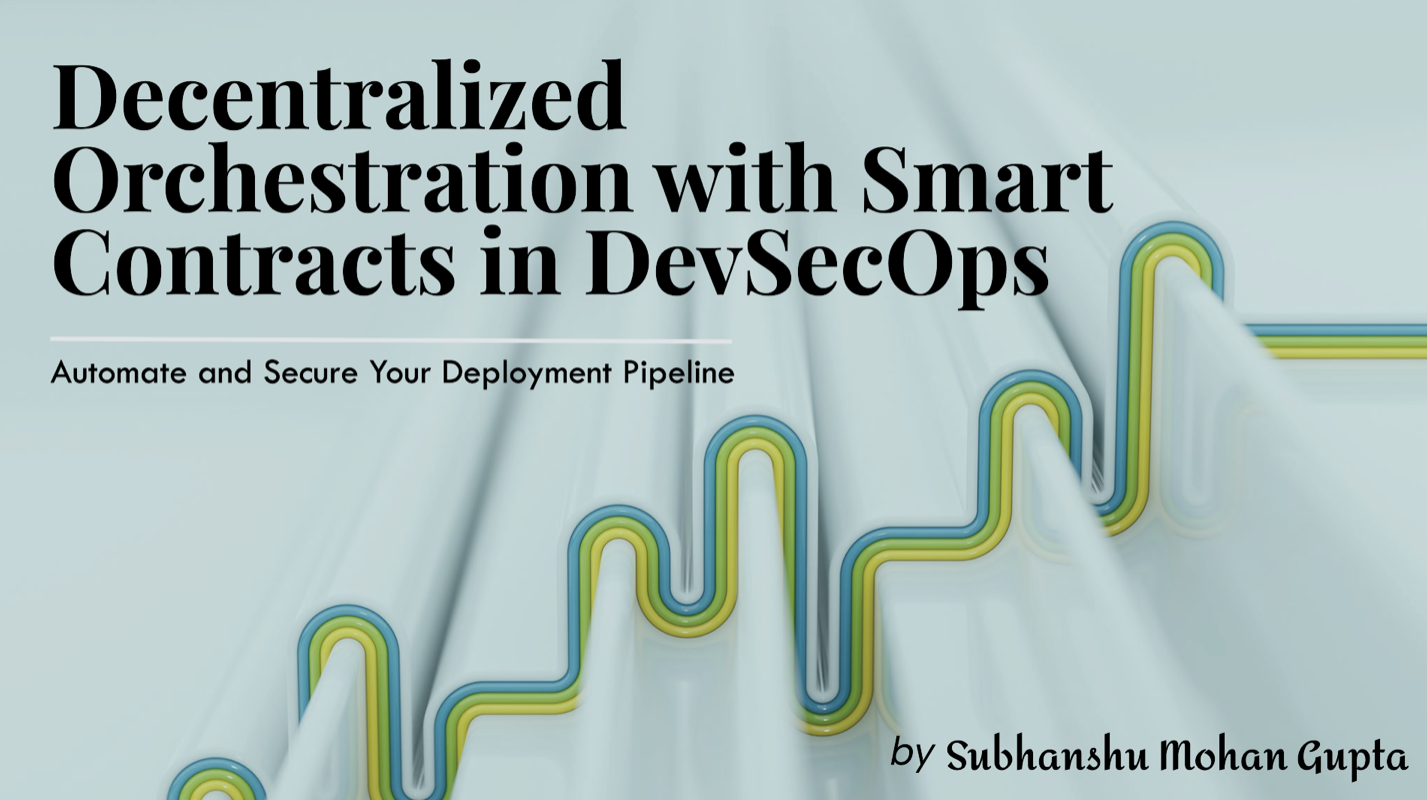
Introduction
As DevSecOps evolves, the need for secure, automated, and efficient deployment pipelines has become critical. Traditional orchestration methods rely on centralized controllers, which are prone to single points of failure and potential security vulnerabilities.
Enter decentralized orchestration using smart contracts - an innovative approach that leverages blockchain technology to automate and secure the deployment pipeline, ensuring tamper-proof, transparent, and reliable orchestration. This blog post explores how smart contracts can revolutionize DevSecOps pipelines by making them more resilient and secure while providing a step-by-step guide to implementing this solution.
The Problem: Centralized Orchestration Bottlenecks
In a typical DevSecOps pipeline, orchestration is managed by centralized systems like Jenkins, CircleCI, or GitLab CI/CD. While these tools are highly effective, they come with several drawbacks:
Single Point of Failure: If the centralized server is compromised, the entire pipeline is at risk.
Security Vulnerabilities: Attackers can exploit centralized controllers to manipulate deployments.
Lack of Transparency: Deployment processes and their logs may not be fully transparent, making auditing and compliance difficult.
In highly sensitive environments, these issues can compromise both security and reliability. A decentralized orchestration model, powered by smart contracts, addresses these challenges.
Real-World Example: Securing Multi-Cloud Deployments
Imagine a scenario where a financial institution needs to deploy its services across multiple cloud providers (AWS, GCP, Azure). The complexity of managing the orchestration securely becomes exponentially challenging, especially with various compliance regulations, data privacy concerns, and potential points of attack. A centralized orchestration controller may introduce risks, like unauthorized access or tampered configurations, which can jeopardize the entire deployment.
By using smart contracts as the orchestrators, this financial institution can ensure that each deployment step is verified on-chain. The smart contract would define rules like:
Validate that the deployment meets security requirements (e.g., vulnerability scanning).
Ensure that the right cryptographic signatures authorize the deployment.
Record every action on the blockchain for transparency and auditing.
In this decentralized setup, even if a malicious actor gains access to one cloud provider, they cannot interfere with the deployment to others without breaching the entire blockchain-based system.
How Decentralized Orchestration Works
Decentralized orchestration involves using smart contracts to automate the tasks typically handled by a centralized CI/CD tool. Smart contracts, which run on a blockchain, can trigger various stages of a deployment pipeline such as building, testing, and deploying based on predefined conditions. Here’s a simplified architecture and end-to-end implementation:
Architecture Diagram
Smart Contract: Acts as the decentralized orchestrator. It governs deployment triggers, security checks, and authorization flows.
Developer/DevOps Team: Interacts with the smart contract to push new code or updates. They trigger deployments via blockchain transactions.
Off-Chain Build Systems: These are external CI/CD tools (like Jenkins or GitLab) that execute build and test steps. However, they are controlled by the smart contract.
Deployment Nodes (AWS, GCP, Azure): The smart contract triggers deployment commands to these nodes, ensuring they follow the rules encoded in the contract.
Blockchain Network: Records every transaction, ensuring full transparency and immutability.
Implementation
1. Define the Smart Contract
The smart contract will manage the deployment process and enforce security policies. Here’s a basic template for such smart contract:
pragma solidity ^0.8.0;
contract DeploymentOrchestrator {
address public owner;
event BuildTriggered(uint256 buildId);
event DeploymentTriggered(uint256 deploymentId);
modifier onlyOwner() {
require(msg.sender == owner, "Not authorized");
_;
}
constructor() {
owner = msg.sender;
}
function triggerBuild(uint256 buildId) public onlyOwner {
// Code to trigger the build process
emit BuildTriggered(buildId);
}
function triggerDeployment(uint256 deploymentId) public onlyOwner {
// Code to trigger the deployment
emit DeploymentTriggered(deploymentId);
}
}
This contract ensures that only authorized users can trigger builds or deployments, providing a secure, decentralized control point.
2. Integrate CI/CD with Smart Contracts
Your CI/CD tools will now be triggered by smart contracts rather than a centralized controller. For example, you can use web3.js to allow Jenkins to interact with the blockchain:
const Web3 = require('web3');
const web3 = new Web3('https://infura.io/v3/YOUR_INFURA_PROJECT_ID');
const contractAddress = '0xYourSmartContractAddress';
const contractABI = [...] // Smart contract ABI
const contract = new web3.eth.Contract(contractABI, contractAddress);
async function triggerBuild(buildId) {
await contract.methods.triggerBuild(buildId).send({ from: '0xYourAddress' });
}
This integration ensures that every build or deployment is cryptographically signed and logged on-chain, making it tamper-proof.
3. Deploy and Test
To test this system:
Deployment: Deploy the smart contract to a blockchain network (e.g., Ethereum, Polygon).
Testing: Push a new update from your code repository. This should automatically trigger a build via the smart contract.
Audit: Verify that every step—build, test, deploy—is logged on the blockchain, ensuring transparency and traceability.
Benefits of Decentralized Orchestration in DevSecOps
Immutability and Transparency: All actions are recorded on-chain, ensuring a tamper-proof audit trail.
Enhanced Security: By decentralizing control, you eliminate single points of failure, making your pipeline more resilient to attacks.
Automated Compliance: Smart contracts can enforce compliance rules automatically, reducing human error.
Conclusion
By integrating smart contracts into your DevSecOps pipeline, you can create a decentralized, secure, and transparent orchestration system. This approach not only strengthens security but also enhances the resilience and automation of your deployment pipeline. The decentralized nature of blockchain ensures that malicious actors cannot tamper with the pipeline, and the transparency it provides can be invaluable for audits and compliance. As organizations continue to move toward multi-cloud, highly regulated environments, decentralized orchestration will become a critical tool in the DevSecOps toolkit.
Call to Action: If you're looking to future-proof your deployment pipelines while enhancing security, consider adopting decentralized orchestration. The combination of blockchain and DevSecOps is not just innovative, it’s essential for the next generation of secure and scalable operations.
Subscribe to my newsletter
Read articles from Subhanshu Mohan Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
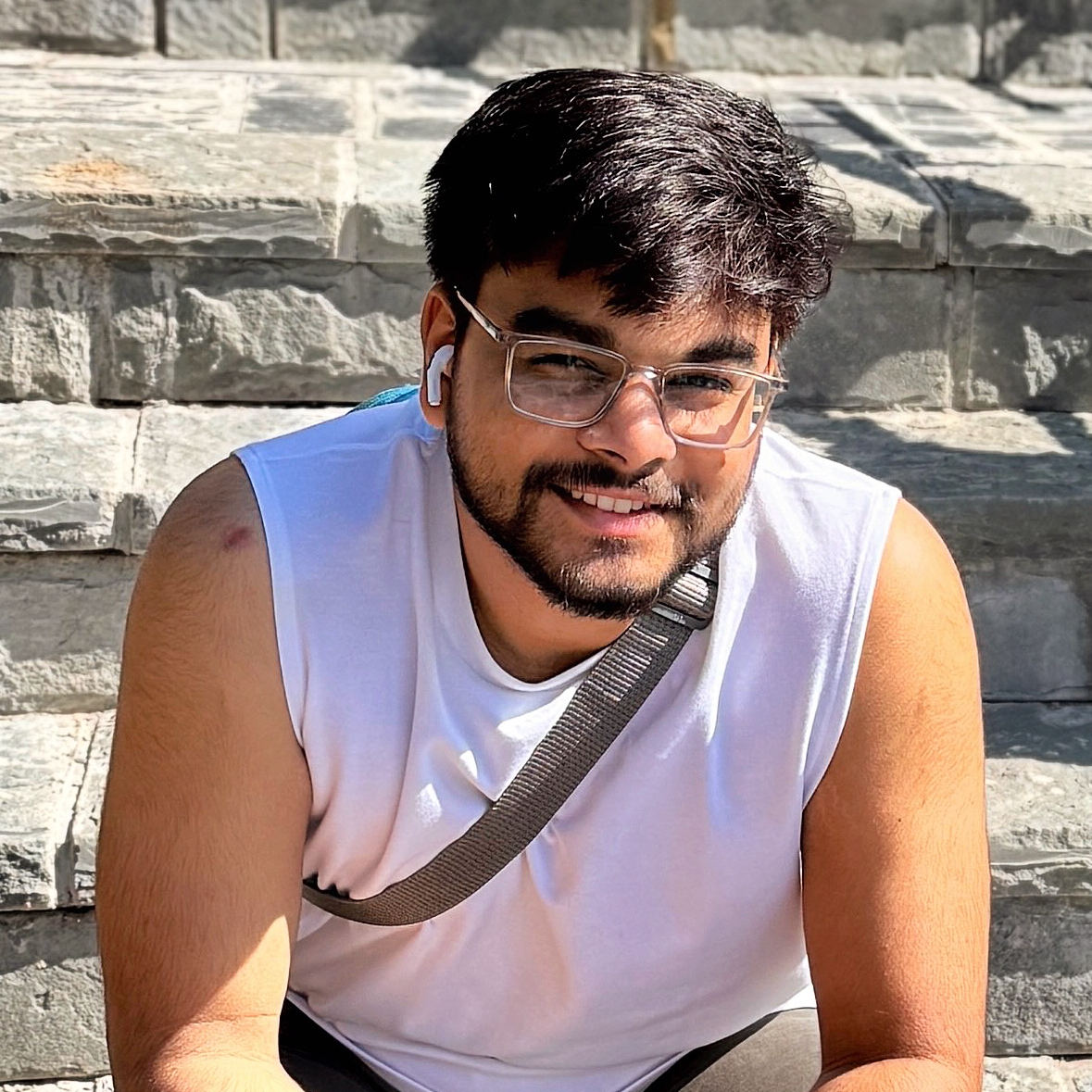
Subhanshu Mohan Gupta
Subhanshu Mohan Gupta
A passionate AI DevOps Engineer specialized in creating secure, scalable, and efficient systems that bridge development and operations. My expertise lies in automating complex processes, integrating AI-driven solutions, and ensuring seamless, secure delivery pipelines. With a deep understanding of cloud infrastructure, CI/CD, and cybersecurity, I thrive on solving challenges at the intersection of innovation and security, driving continuous improvement in both technology and team dynamics.