Find Middle of a Linked List - Leet code 876 - two pointers approach
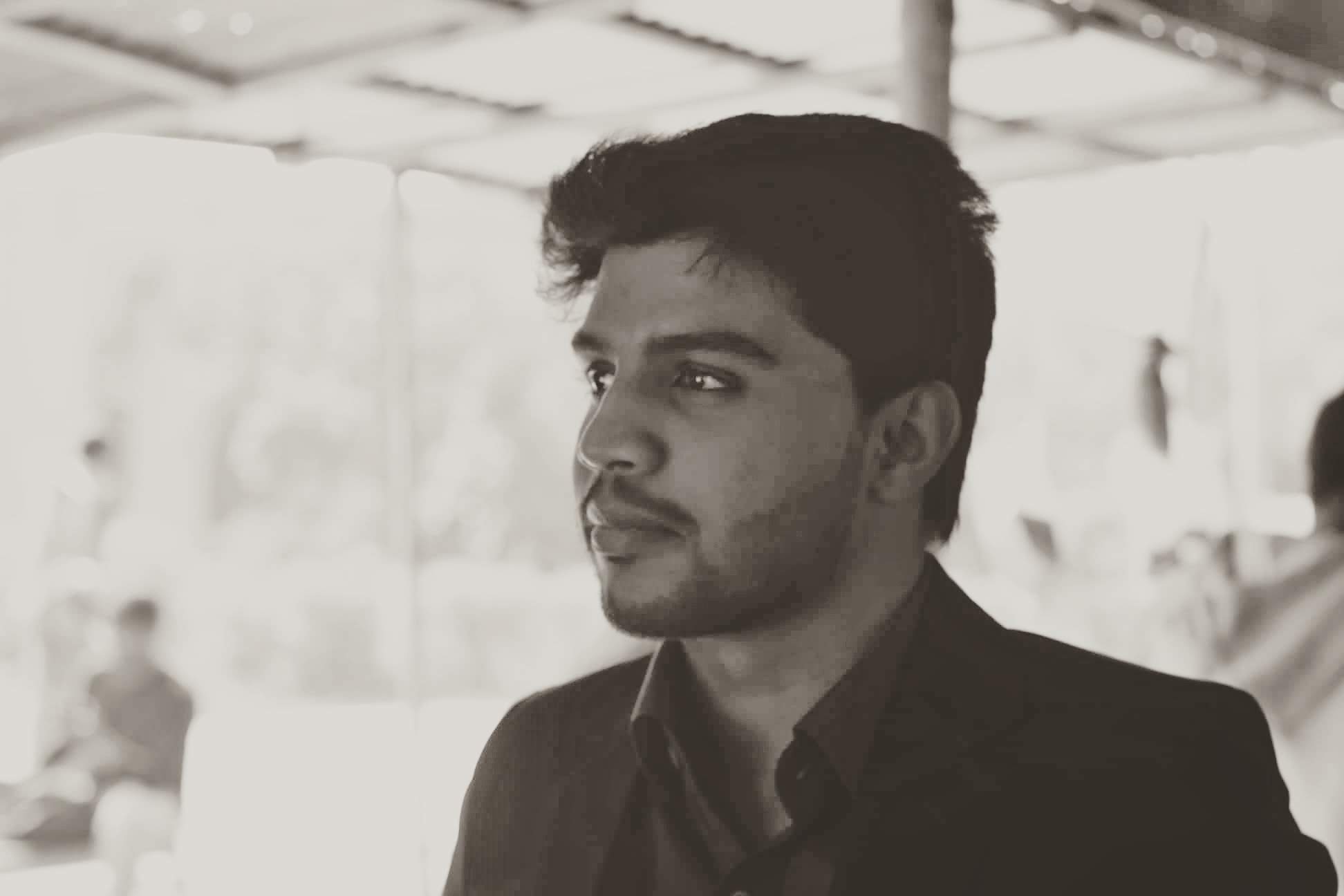
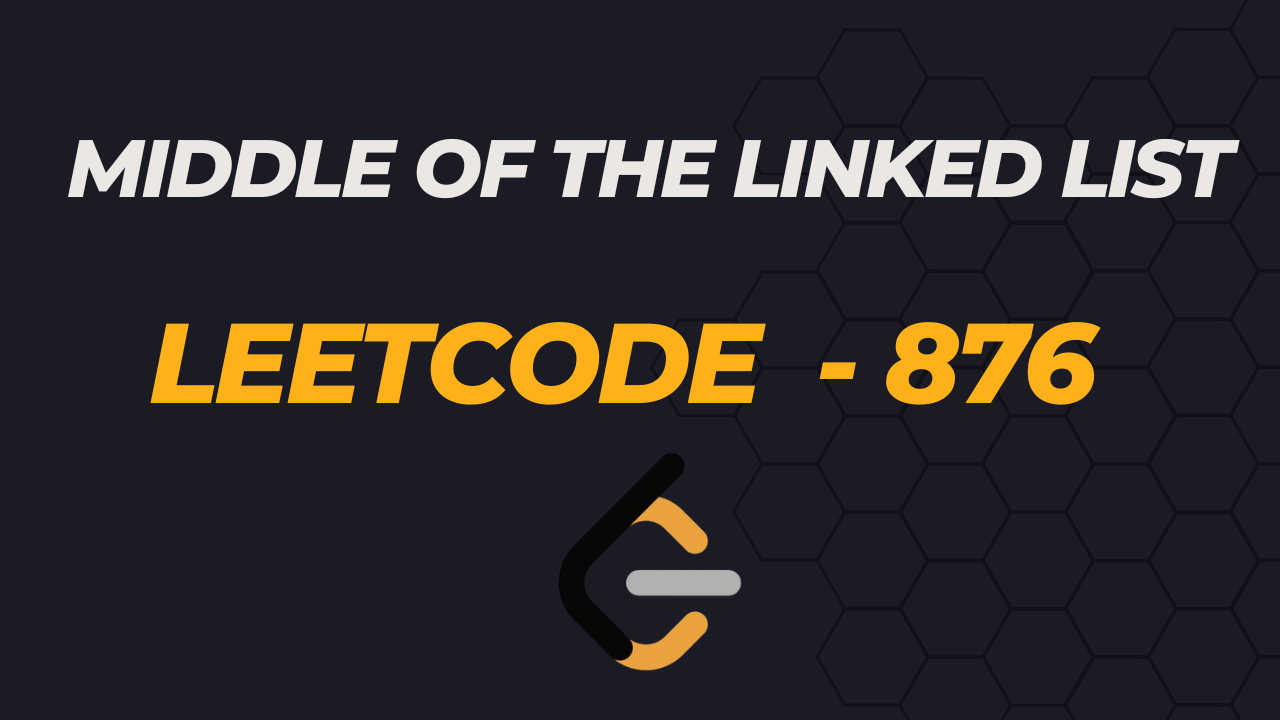
Hey everyone, we will solve how we can find the Middle of the Linked List and solve this problem using a pointers approach.
Step 1: Initialize Pointers
We are going to initialize the left
and right
pointers at the beginning of our beginning of our Linked List.
left = head # Start both pointers at the head
right = head
Step 3: Traverse through the linked list
In this step, we will traverse through the list by moving the right
pointer by two steps and the left
pointer by one step. When the right
pointer reaches the end (null), the left
the pointer will be in the middle.
Step 3: Once the loop terminates, the left
the pointer will be at the middle node.
def find_middle(head):
# Step 1: Initialize left and right pointers at the head
left = head
right = head
# Step 2: Traverse the linked list, moving left by 1 step and right by 2 steps
while right and right.next:
left = left.next # Move left one step
right = right.next.next # Move right two steps
# Step 3: Left is now pointing to the middle of the linked list
return left
Time and Space Complexity:
Time Complexity: O(n)
Space Complexity: O(1)
Video Explanation:
Thanks, everyone hanging in there till the last ❤️ and I'll see you in the next one!!
Subscribe to my newsletter
Read articles from Shojib directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
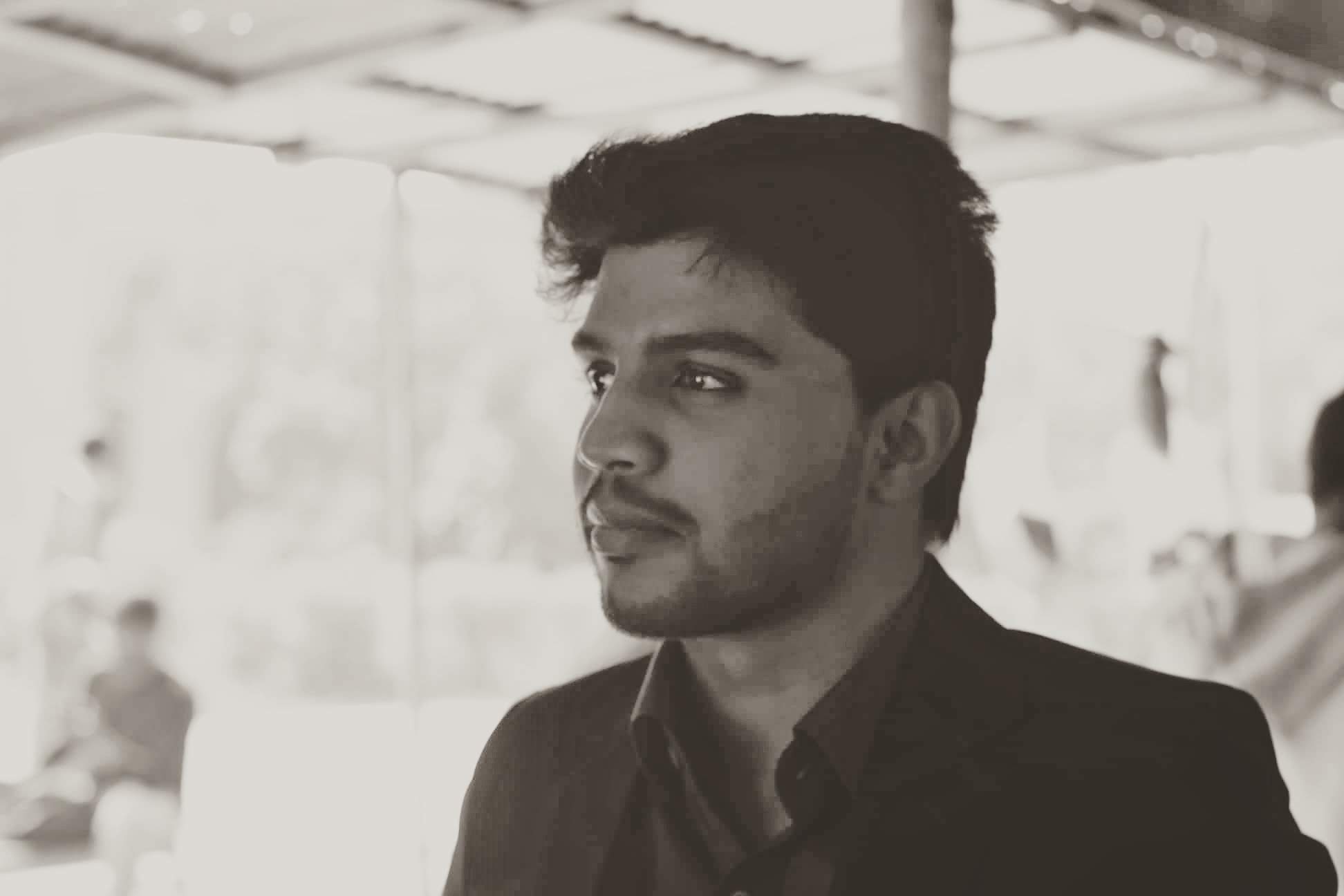
Shojib
Shojib
Hey, I'm Kamruzaman Shojib and I'm a front-end developer. I love to talk about tech mostly I'll be writing about JavaScript and trying to help the community.