Components and Props in ReactJS

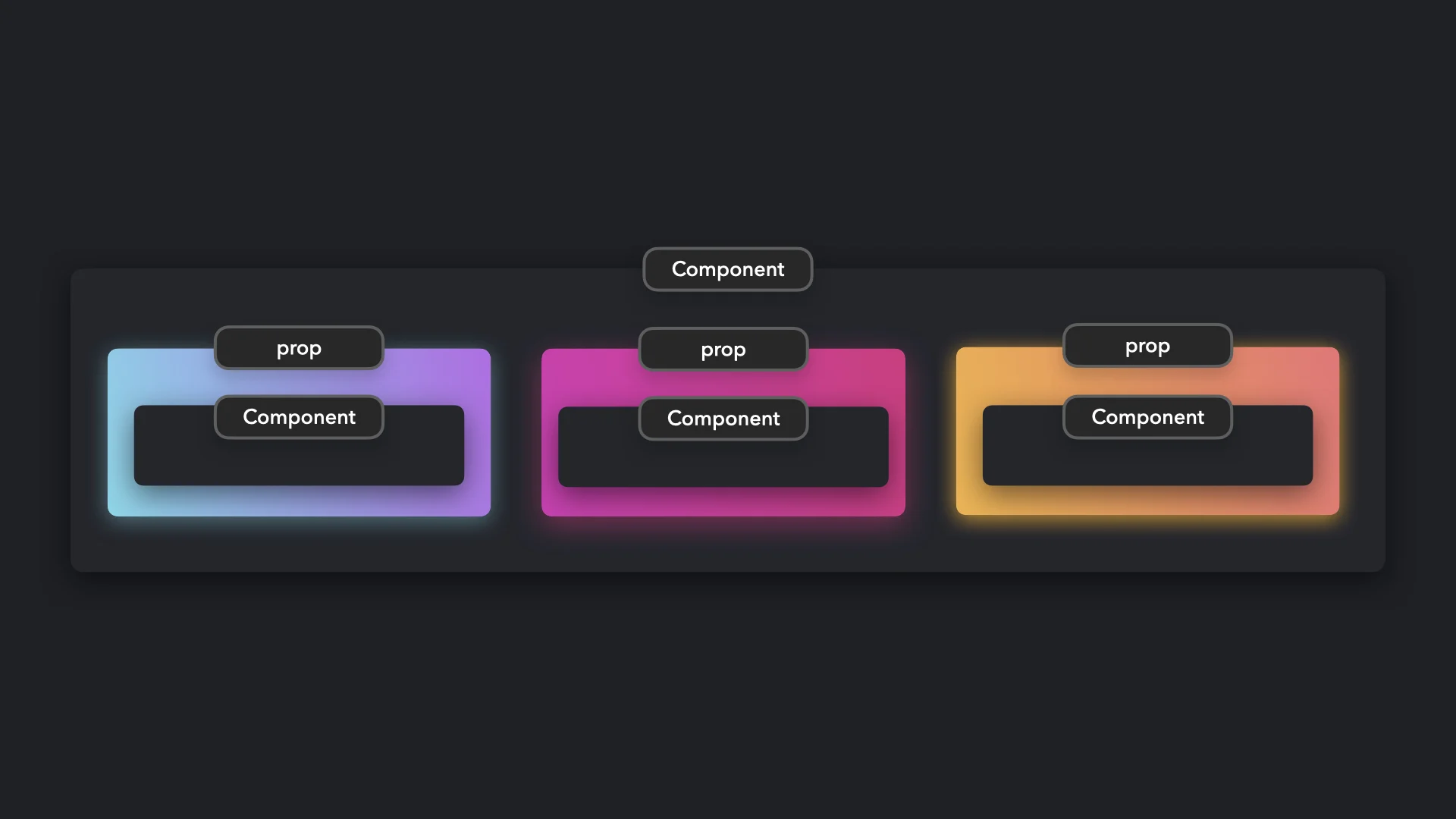
The word Props is not a keyword or something. It is a term used to refer to the properties of a JSX component which makes the component unique.
It is a feature in React that allows us to make the content of a component dynamic.
What is a JSX?
It is nothing but Javascript + HTML combined. Its full form is Javascript XML.
It makes it easy to handle both HTML and Javascript in one place.
JSX code cannot be interpreted by the browser directly, it needs a transpiler known as Babel.
Transpiler?
(Transform + Compile)
Because it transforms the JSX code into regular Javascript functions that work across all browsers.
It basically converts JSX into an object or tree that contains properties like the name of the element, attributes of the element, inner HTML of the element, etc.
It looks something like this :
const element = {
type: 'img',
attributes: {
src: "/source.jpg"
alt: "image"
},
inner: "This is an Image"
}
The above code is just an idea of how it transpiles the JSX code, it has some rules for doing it. The above code is really different from what Babel does.
Now, What do props have to do with JSX?
So the idea is, that React never discriminates the code on the basis of their language whether it is HTML, CSS, or Javascript. It uses JSX format to combine them.
React only discriminates the code or page on the basis of the work of the Component.
Here comes an important part Components.
Components are basically different parts of a page written separately as functions in different files and then joined together using JSX in one place.
It uses functional programming to enhance coding experience, reusability, and readability.
Still have no idea what Props have to do with all these!!!
Props are used to differentiate each similar component.
If we are using the same component more than one time in the final code, and we want to change some content in each copy of the component, we assign that change in the form of props or properties and we use that property name in the function parameter of the component in the form of function Card({name})
.
This makes each of the same type of Components differ from each other if needed.
Now look at the below code :
NOTE: TailwindCSS is also used in the code, but don't worry if you don't understand it, just focus on the concept.
//App.jsx//
import Card from './Components/Cards'
function App() {
return (
<>
<Card />
</>
)
}
export default App
//Card.jsx//
import React from "react";
function Card(){
return(
<div className="w-[300px] rounded-md border">
<img
src="https://images.unsplash.com/photo-1522199755839-a2bacb67c546?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxzZWFyY2h8MTF8fGJsb2d8ZW58MHx8MHx8&auto=format&fit=crop&w=800&q=60"
alt="Laptop"
className="h-[200px] w-full rounded-md object-cover"
/>
<div className="p-4">
<h1 className="text-lg font-semibold text-yellow-100">About Macbook</h1>
<p className="mt-3 text-sm text-white">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Excepturi, debitis?
</p>
<button
type="button"
className="mt-4 rounded-sm bg-black px-2.5 py-1 text-[10px] font-semibold text-white shadow-sm hover:bg-black/80 focus-visible:outline focus-visible:outline-2 focus-visible:outline-offset-2 focus-visible:outline-black"
>
Read
</button>
</div>
</div>
)
}
export default Card
Don't worry about the code, it is just a code for the Card.
Output :
Now if we want 3 cards but one for Macbook, one for Hp, and one for Lenovo, we are not going to write that big code (you were scared of) two more times, right?
So we use the component two more times.
//App.jsx//
import Card from './Components/Cards'
function App() {
return (
<>
<Card />
<Card />
<Card />
</>
)
}
export default App
Output :
But Umar, how are we going to change the details of each copy? Hmmm....
We will use Props for this task.
Look at the changes in the code below in Cards.jsx
:
//Card.jsx//
import React from "react";
function Card( { brand_name } ){
return(
// SOME CODE ABOVE
<h1 className="text-lg font-semibold text-yellow-100">About {brand_name}</h1>
// SOME CODE BELOW
)
}
export default Card
Now, in App.jsx
:
//App.jsx//
import Card from './Components/Cards'
function App() {
return (
<>
<Card brand_name = "Macbook"/>
<Card brand_name = "HP"/>
<Card brand_name = "Lenovo"/>
</>
)
}
export default App
Output :
See, how easily we can do magic on the page using Components and Props.
Summary
Components are used to divide the web page into different parts, code them separately, and then join them together. It enhances the readability and reusability of code.
Props allows us to send data from one component to another. It defines the properties of each component (which is used to differentiate one copy of a component from another). It is a way to make UNIQUE REUSABLE components in React.
Thank you.
Subscribe to my newsletter
Read articles from Umar Khursheed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Umar Khursheed
Umar Khursheed
On a journey to explore the exciting realms of Development. Pursuing Btech in Information Technology from GL Bajaj Institute of Technology and Management. Simultaneously, my journey includes the fascinating realm of Data Structures and Algorithms using C++. Solving complex problems and optimizing solutions is my forte, and I'm always eager to explore new algorithms and data structures that enhance my problem-solving skills.