How to Take a Screenshot of a Webpage in Java

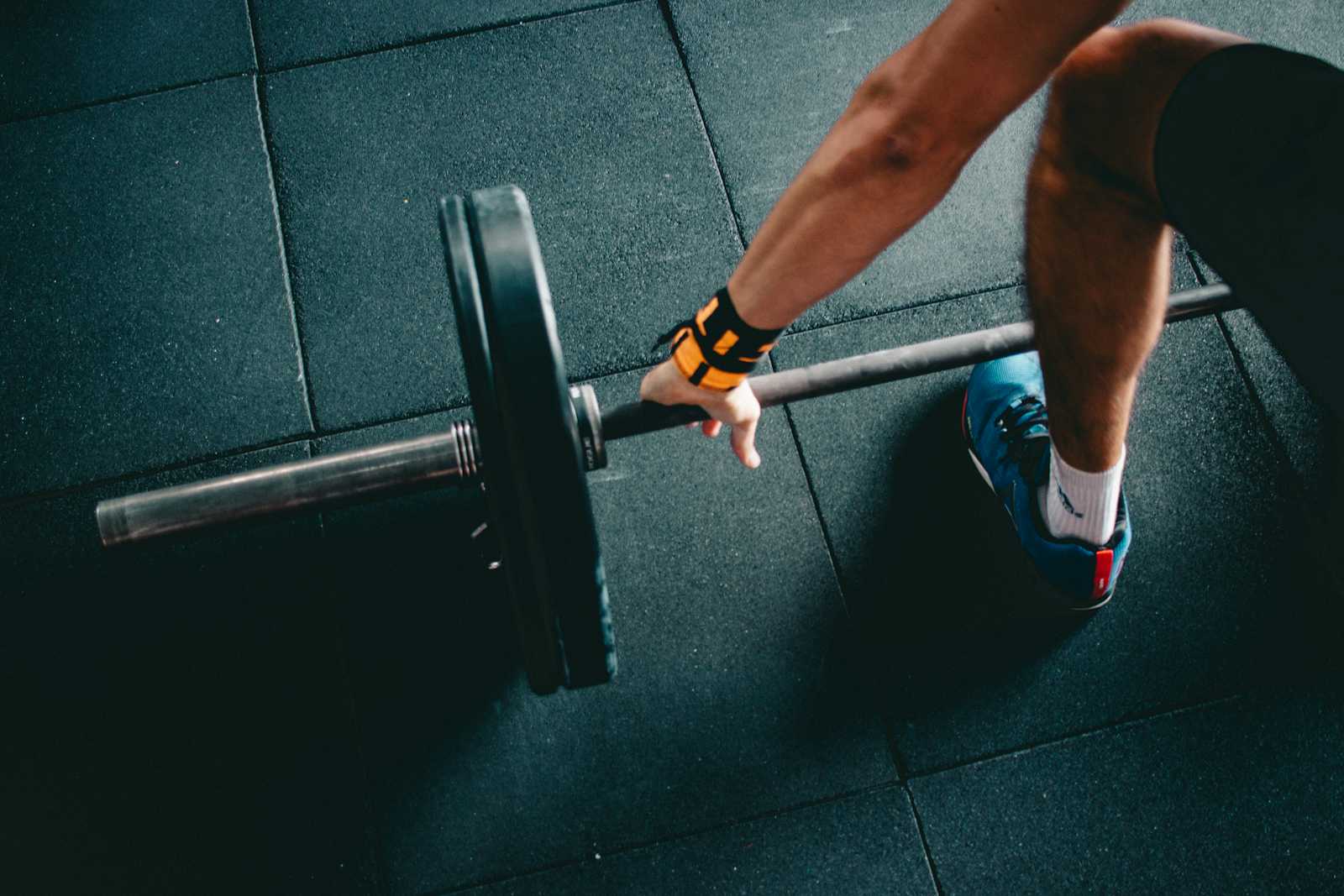
In today’s digital age, capturing screenshots is essential for various tasks like web development, testing, and automation. Often, developers need to programmatically capture webpages for reporting, monitoring, or archiving website states. In this article, we’ll explore how to take a screenshot of a webpage using Java, a popular and versatile programming language.
Prerequisites:
Before diving into the code, ensure you have the following prerequisites:
Java Development Kit (JDK) installed on your system.
A Java Integrated Development Environment (IDE) such as Eclipse, IntelliJ IDEA, or Visual Studio Code.
Selenium WebDriver library added to your Java project. You can include it using a build tool like Maven or Gradle.
Getting Started with Selenium
Selenium is a popular framework for automating web browsers, providing an efficient way to take webpage screenshots. Follow these steps to get started:
Set Up Your Java Project: Create a new Java project in your preferred IDE and add Selenium WebDriver as a dependency.
Configure WebDriver: Selenium supports browsers like Chrome, Firefox, and Edge. Here, we’ll use Chrome. You’ll need to download ChromeDriver for your Chrome browser version. Make sure ChromeDriver is in your system’s PATH or provide its path in the code.
Write Java Code: Let’s write the Java code to take a screenshot of a webpage. Here’s an example:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
public class WebpageScreenshot {
public static void main(String[] args) {
// Set the path to ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver.exe");
// Initialize the WebDriver
WebDriver driver = new ChromeDriver();
// Navigate to the webpage you want to capture
driver.get("https://www.genghisfitness.com");
try {
// Take the screenshot and store it as a file
File screenshotFile = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
// Define the destination path for the screenshot
File destinationFile = new File("screenshot.png");
// Copy the screenshot to the destination path
FileUtils.copyFile(screenshotFile, destinationFile);
System.out.println("Screenshot saved to: " + destinationFile.getAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
} finally {
// Close the browser
driver.quit();
}
}
}
Explanation:
We set the path to the ChromeDriver executable using
System.setProperty
.The WebDriver is initialized with ChromeDriver.
We navigate to the webpage that we want to capture.
The
TakesScreenshot
interface captures the screenshot and saves it as a file.Finally, we close the browser.
Real-World Use Case: Reversible Elbow Sleeves
This code can be extended for e-commerce websites, such as capturing product images or performance data for reversible elbow sleeves Whether for product development, quality assurance, or showcasing, automating screenshots in this way can streamline workflow.
Conclusion
Taking screenshots programmatically is a valuable skill for web developers and testers. Using Java and Selenium WebDriver, you can easily capture webpage screenshots and even extend this code for multiple pages, automation scripts, or testing frameworks. You can also integrate it with websites selling reversible elbow sleeves to monitor the display of products efficiently. Happy coding!
Subscribe to my newsletter
Read articles from Allan Potts directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
