Remix js
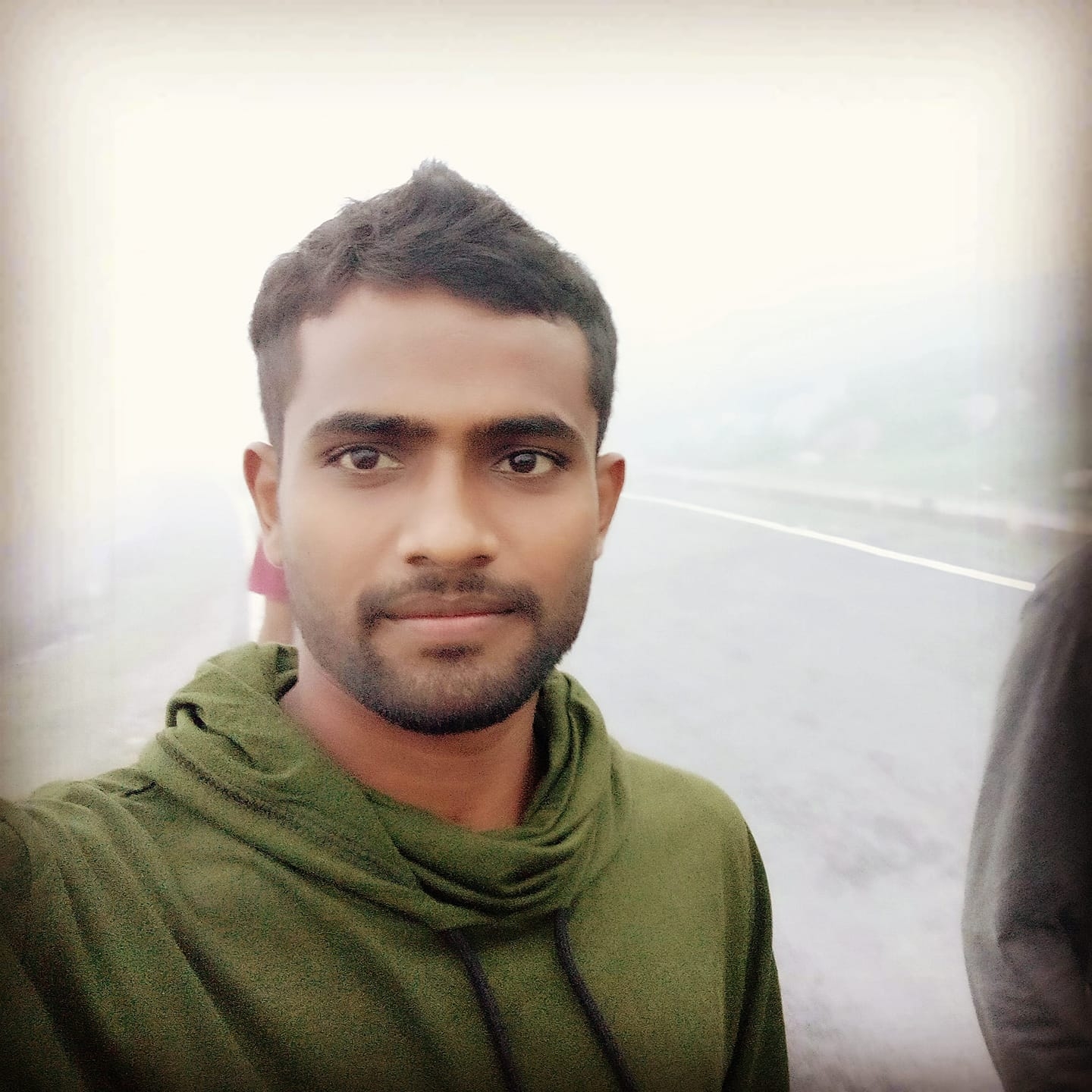
Remix.js Overview
Remix.js is a robust web framework designed to simplify the development of modern web applications. It offers a flexible and efficient approach to building interactive user interfaces. Remix.js prioritizes performance, developer experience, and scalability, making it a popular choice among web developers.
Key Features of Remix.js
Server-Side Rendering: Remix.js emphasizes server-side rendering to enhance SEO and initial load times by generating HTML on the server before sending it to the client. This approach improves user experience by delivering content quickly and efficiently.
Advanced Routing: Remix.js provides advanced routing capabilities, allowing developers to define custom routes easily and efficiently navigate between different parts of the application.
Data Loading Strategies: Remix.js offers flexible data loading strategies, enabling developers to fetch data from various sources and integrate it seamlessly into their applications.
Plugin System: Remix.js supports a plugin system that allows developers to extend and customize their applications with ease, enhancing functionality and scalability.
Example
Here's a simple example of how routing works in Remix.js:
// app/routes/index.js
import { json } from 'remix';
export const loader = async () => {
const data = await fetch('https://api.example.com/data');
const jsonData = await data.json();
return json({ data: jsonData });
};
export default function Index({ data }) {
return (
<div>
<h1>Welcome to Remix.js</h1>
<p>{data}</p>
</div>
);
}
In this example, we define a route for the homepage (/
) that loads data from an external API and renders it on the page. This demonstrates how Remix.js simplifies data loading and routing to create dynamic web applications efficiently
Here are some examples of working with APIs in Remix.js:
Example 1: Fetching Data from an API
// app/routes/api/posts.js
import { json } from 'remix';
export const loader = async () => {
const response = await fetch('https://api.example.com/posts');
const posts = await response.json();
return json({ posts });
};
In this example, we define a route for fetching posts from an API and returning the data as JSON.
Example 2: Posting Data to an API
// app/routes/api/createPost.js
import { redirect } from 'remix';
export const loader = async ({ request }) => {
const formData = await request.json();
// Code to post data to an API
// Example: await fetch('https://api.example.com/posts', { method: 'POST', body: JSON.stringify(formData) });
return redirect('/success');
};
In this example, we define a route for posting data to an API. The route receives data from the request, processes it, and then posts it to the API.
Example 3: Updating Data via an API
// app/routes/api/updatePost.js
import { redirect } from 'remix';
export const loader = async ({ request }) => {
const { postId, newData } = await request.json();
// Code to update data via an API
// Example: await fetch(`https://api.example.com/posts/${postId}`, { method: 'PUT', body: JSON.stringify(newData) });
return redirect('/success');
};
In this example, we define a route for updating data via an API. The route receives the post ID and new data, updates the data on the API, and then redirects to a success page.
These examples demonstrate how Remix.js can interact with APIs to fetch, post, and update data, providing a seamless integration with external services. Feel free to customize and expand upon these examples to suit your specific API integration needs!
Feel free to explore Remix.js further to discover its full potential in building modern web applications!
Subscribe to my newsletter
Read articles from venkata ravindra pv directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
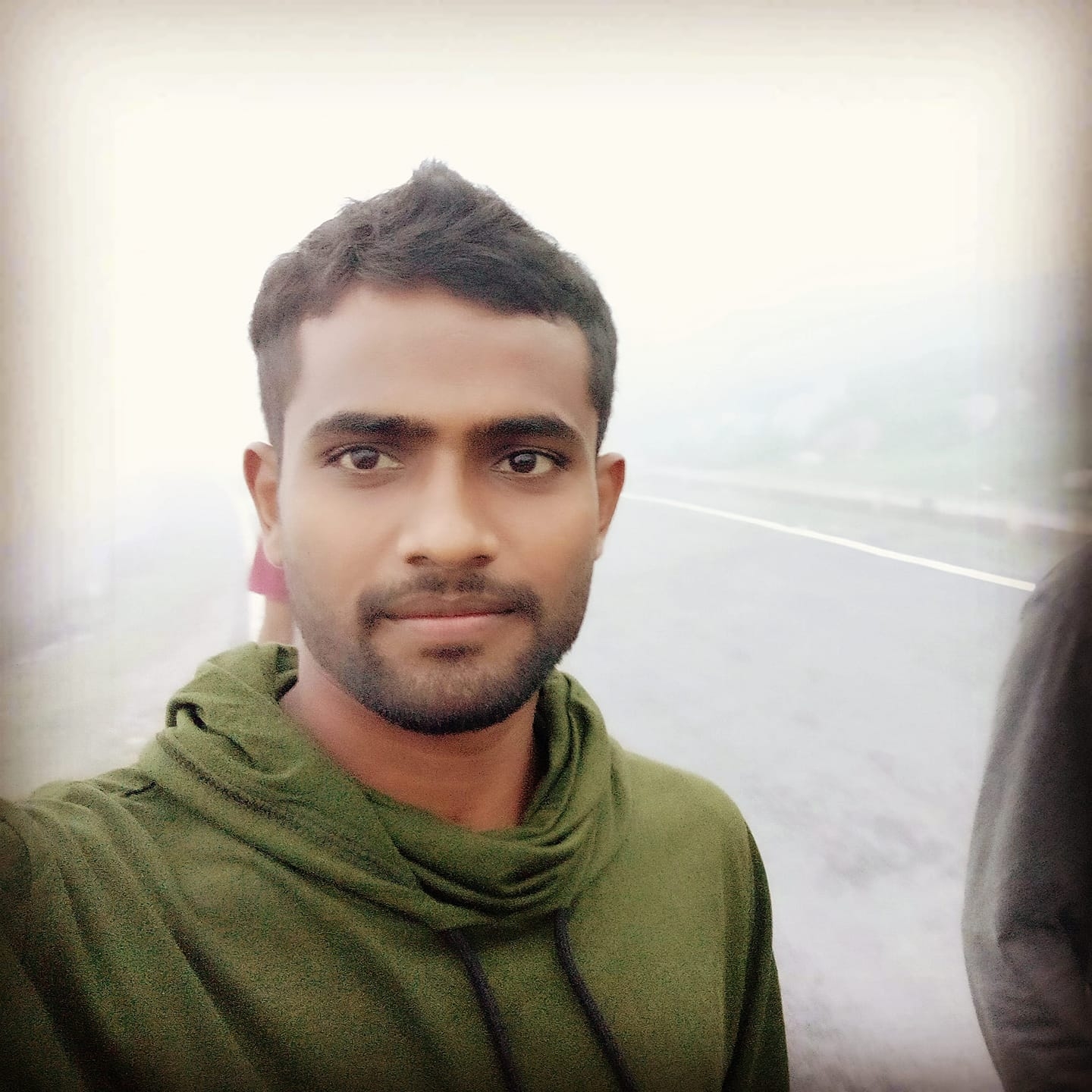