Strings in Java
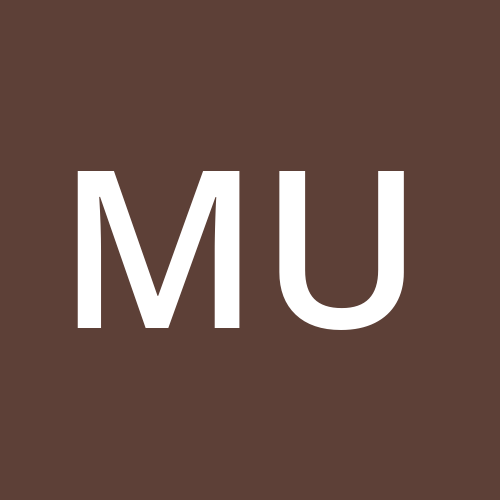
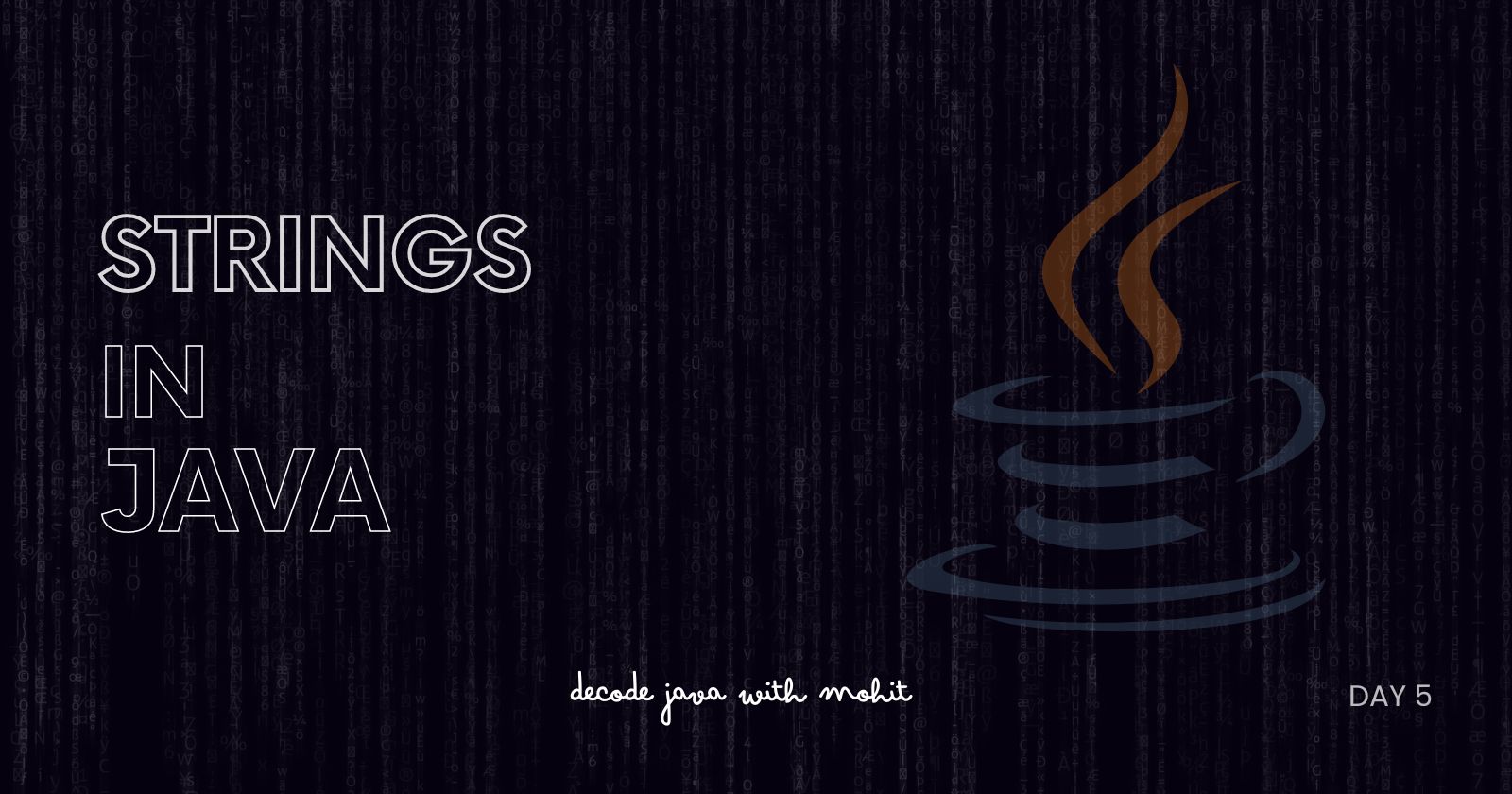
On Day 5, the focus will be on Strings in Java, Strings are objects in Java, which makes them both powerful and complex, but they are also easy to use in most basic operations.
1. Introduction to Strings
In Java, a String is a sequence of characters.
Unlike in some programming languages where a string is just an array of characters, Java strings are objects of the
String
class.Strings are immutable, meaning once a string is created, it cannot be changed.
Basic Declaration:
String name = "John"; // String literal
String Object:
String name = new String("John"); // String object using the new keyword
Important Note:
- The difference between using a string literal and creating a string with the
new
keyword is that literals are stored in the String pool, which helps save memory, while objects created withnew
are stored in the heap memory.
2. String Methods
Java provides many built-in methods for manipulating strings.
Important Methods:
length()
: Returns the length of the string (number of characters).String str = "Hello World"; System.out.println(str.length()); // Output: 11
charAt()
: Returns the character at a specified index.System.out.println(str.charAt(0)); // Output: H
substring()
: Returns a substring of the original string, starting and ending at specified indices.System.out.println(str.substring(0, 5)); // Output: Hello
toUpperCase()
andtoLowerCase()
: Converts the string to uppercase or lowercase.//Strings in Java are immutable , these will create new Object System.out.println(str.toUpperCase()); // Output: HELLO WORLD System.out.println(str.toLowerCase()); // Output: hello world
contains()
: Checks whether a string contains a specified sequence of characters.System.out.println(str.contains("World")); // Output: true
equals()
andequalsIgnoreCase()
: Compares two strings for equality.equalsIgnoreCase()
ignores case differences.String str1 = "Hello"; String str2 = "hello"; System.out.println(str1.equals(str2)); // Output: false System.out.println(str1.equalsIgnoreCase(str2)); // Output: true
replace()
: Replaces characters or substrings within a string.System.out.println(str.replace("World", "Java")); // Output: Hello Java
3. String Concatenation
- You can join (concatenate) two or more strings using the
+
operator or theconcat()
method.
Using +
operator:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
System.out.println(fullName); // Output: John Doe
Using concat()
method:
String fullName = firstName.concat(" ").concat(lastName);
System.out.println(fullName); // Output: John Doe
4. String Immutability
- Strings in Java are immutable. Once a string is created, it cannot be changed. Any method that appears to modify a string actually creates a new string object.
Example:
String original = "Hello";
String modified = original.concat(" World");
System.out.println(original); // Output: Hello
System.out.println(modified); // Output: Hello World
Explanation:
- The
concat()
method does not change the original string but returns a new string with the added value.
5. String Comparison
- Comparing strings is a common task in programming. In Java, there are two main ways to compare strings: using
==
andequals()
.
Using ==
:
- The
==
operator checks whether two string variables refer to the same object in memory (i.e., checks for reference equality).
String str1 = "Hello";
String str2 = "Hello";
// Output: true (because both refer to the same object in the string pool)
System.out.println(str1 == str2);
Using equals()
:
- The
equals()
method checks whether two strings have the same sequence of characters (i.e., checks for value equality).
String str1 = new String("Hello");
String str2 = new String("Hello");
// Output: true (same value, different objects)
System.out.println(str1.equals(str2));
Conclusion:
- Use
==
to check reference equality, andequals()
to compare string content.
6. StringBuilder and StringBuffer
- Since strings are immutable, performing multiple string manipulations can be inefficient because each change creates a new string object. To overcome this, Java provides StringBuilder and StringBuffer classes, which allow mutable sequences of characters.
StringBuilder:
Used for creating and modifying strings without the overhead of creating new objects.
It is not thread-safe (i.e., it does not handle concurrent modifications).
Example:
StringBuilder sb = new StringBuilder("Hello");
sb.append(" World");
System.out.println(sb.toString()); // Output: Hello World
StringBuffer:
- Similar to StringBuilder, but it is thread-safe (i.e., it can be safely used in a multithreaded environment).
Example:
StringBuffer sb = new StringBuffer("Hello");
sb.append(" World");
System.out.println(sb.toString()); // Output: Hello World
When to Use:
Use StringBuilder when performance is a concern and thread safety is not needed.
Use StringBuffer in multithreaded applications where thread safety is required.
7. Practical Example: Palindrome Check
Let’s take a practical example of checking if a given string is a palindrome (a string that reads the same backward as forward).
Example:
public class PalindromeCheck {
public static void main(String[] args) {
String original = "madam";
String reversed = new StringBuilder(original).reverse().toString();
if (original.equals(reversed)) {
System.out.println(original + " is a palindrome.");
} else {
System.out.println(original + " is not a palindrome.");
}
}
}
Explanation:
We use
StringBuilder
to reverse the original string.We then compare the reversed string with the original using the
equals()
method.
8. Practical Example: Word Count
Another common string manipulation task is counting the number of words in a sentence.
Example:
public class WordCount {
public static void main(String[] args) {
String sentence = "Hello world this is Java";
String[] words = sentence.split(" ");
System.out.println("Word count: " + words.length);
}
}
Explanation:
- The
split()
method splits the string into an array of words based on spaces, and then we use thelength
property of the array to count the words.
Summary
By the end of Day 5, we will have a deep understanding of:
Declaring and manipulating strings.
Various methods provided by the
String
class for common operations.The concept of string immutability and why it matters.
How to use
StringBuilder
andStringBuffer
for efficient string manipulation.Practical examples like palindrome checks and word counting using strings.
Strings are an integral part of Java programming, and mastering them will enable you to handle text processing, input validation, and other key tasks in building applications. Stay tuned! for further topics.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
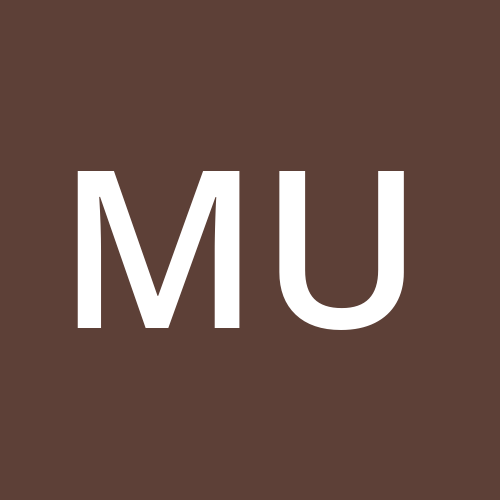