Chapter 2: Operators in Java
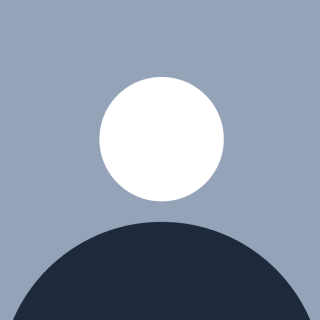
Operators are special symbols in Java that perform operations on variables and values. They are the foundation for performing tasks such as arithmetic, comparisons, and logical decisions in a program. Understanding how different operators work is essential for writing effective Java code.
i) Types of Operators
Java provides a variety of operators to perform different tasks. These include:
Arithmetic Operators
Unary Operators
Relational Operators
Logical Operators
Assignment Operators
Precedence of Operators
Each of these operator types is used in specific scenarios and performs specific operations. Let’s explore each of them in detail.
1. Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, division, and finding the remainder. These are some of the most common operations in any programming language.
Operator | Description | Example |
+ | Addition | a + b |
- | Subtraction | a - b |
* | Multiplication | a * b |
/ | Division | a / b |
% | Remainder (Modulus) | a % b |
Example:
import java.util.Scanner;
public class ArithmeticExample {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a:");
int a = sc.nextInt();
System.out.println("Enter b:");
int b = sc.nextInt();
System.out.println("The sum of a and b is: " + (a + b));
System.out.println("The difference of a and b is: " + (a - b));
System.out.println("The product of a and b is: " + (a * b));
System.out.println("The quotient of a and b is: " + (a / b));
System.out.println("The remainder of a and b is: " + (a % b));
}
}
2. Unary Operators
Unary operators work on a single operand. They are used to perform operations such as incrementing or decrementing a value, negating an expression, or inverting the value of a boolean.
Operator | Description | Example |
++ | Increment: increases value by 1 | ++a or a++ |
-- | Decrement: decreases value by 1 | --a or a-- |
+ | Positive: returns positive value | +a |
- | Negative: returns negative value | -a |
! | Logical NOT: inverts the boolean value | !true |
Example:
public class UnaryExample {
public static void main(String[] args) {
int a = 10;
int b = ++a; // Pre-increment
System.out.println("Pre-increment: " + a); // Output: 11
System.out.println("Value of b: " + b); // Output: 11
int c = 10;
int d = c++; // Post-increment
System.out.println("Post-increment: " + c); // Output: 11
System.out.println("Value of d: " + d); // Output: 10
}
}
3. Relational Operators
Relational operators are used to compare two values. These operators return a boolean result (true or false) depending on the comparison.
Operator | Description | Example |
== | Equal to | a == b |
!= | Not equal to | a != b |
> | Greater than | a > b |
< | Less than | a < b |
>= | Greater than or equal to | a >= b |
<= | Less than or equal to | a <= b |
Example:
public class RelationalExample {
public static void main(String[] args) {
int a = 10;
int b = 5;
System.out.println(a == b); // false
System.out.println(a != b); // true
System.out.println(a > b); // true
System.out.println(a < b); // false
System.out.println(a >= b); // true
System.out.println(a <= b); // false
}
}
4. Logical Operators
Logical operators are used to perform logical operations on boolean values. These operators are typically used in conditional statements.
Operator | Description | Example | |
&& | Logical AND | (a < b) && (b < c) | |
! | Logical NOT | !(a == b) |
Example:
public class LogicalExample {
public static void main(String[] args) {
int a = 5;
int b = 8;
// Logical AND
System.out.println((a < b) && (b < a)); // false
// Logical OR
System.out.println((a > b) || (a < b)); // true
// Logical NOT
System.out.println(!(a == b)); // true
}
}
5. Assignment Operators
Assignment operators are used to assign values to variables. They can also be used to perform operations like addition, subtraction, multiplication, etc., and assign the result back to the variable.
Operator | Description | Example |
= | Assign | a = b |
+= | Add and assign | a += b |
-= | Subtract and assign | a -= b |
*= | Multiply and assign | a *= b |
/= | Divide and assign | a /= b |
Example:
public class AssignmentExample {
public static void main(String[] args) {
int a = 6;
int b = 2;
a += 2; // a = a + 2
System.out.println(a); // Output: 8
a -= 3; // a = a - 3
System.out.println(a); // Output: 5
a /= b; // a = a / b
System.out.println(a); // Output: 2
a *= b; // a = a * b
System.out.println(a); // Output: 4
}
}
6. Precedence of Operators
Operator precedence determines the order in which operators are evaluated in an expression. For example:
int x = 3 * 4 - 1;
In this example, the multiplication operator *
has higher precedence than the subtraction operator -
, so the multiplication is performed first, and the value of x
will be 11
and not 9
.
Here’s a simplified precedence table:
Operators | Associativity |
Postfix increment and decrement | Left to Right |
Prefix increment and decrement | Right to Left |
Multiplicative (* , / , % ) | Left to Right |
Additive (+ , - ) | Left to Right |
Relational (< , <= , > , >= ) | Left to Right |
Equality (== , != ) | Left to Right |
Logical AND (&& ) | Left to Right |
Logical OR (` | |
Assignment (= , += , -= , etc.) | Right to Left |
Operators Practice Questions
Let's test your understanding of operators with some practice questions.
- What will be the output of the following program?
public class Test {
public static void main(String[] args) {
int x = 2, y = 5;
int exp1 = (x * y / x);
int exp2 = (x * (y / x));
System.out.print(exp1 + ", ");
System.out.print(exp2);
}
}
- What will be the output of the following program?
public class Test {
public static void main(String[] args) {
int x = 200, y = 50, z = 100;
if (x > y && y > z) {
System.out.println("Hello");
}
if (z > y && z < x) {
System.out.println("Java");
}
if ((y + 200) < x && (y + 150) < z) {
System.out.println("Hello Java");
}
}
}
- What will be the output of the following program?
public class Test {
public static void main(String[] args) {
int x, y, z;
x = y = z = 2;
x += y;
y -= z;
z /= (x + y);
System.out.println(x + " " + y + " " + z);
}
}
- What will be the output of the following program?
public class Test {
public static void main(String[] args) {
int x = 9, y = 12;
int a = 2, b = 4, c = 6;
int exp = 4 / 3 * (x + 34) + 9 * (a + b * c) + (3 + y * (2 + a)) / (a + b * y);
System.out.println(exp);
}
}
- What will be the output of the following program?
public class Test {
public static void main(String[] args) {
int x = 10, y = 5;
int exp1 = (y * (x / y + x / y));
int exp2 = (y * x / y + y * x / y);
System.out.println(exp1); // Output: 20
System.out.println(exp2); // Output: 20
}
}
Conclusion
Operators form the core of most programming tasks. Understanding how to use arithmetic, unary, relational, logical, and assignment operators effectively is crucial to building logic in Java programs. Additionally, knowing the precedence and associativity of operators ensures that your expressions are evaluated as expected, leading to correct results.
This chapter provided a detailed explanation of Java operators along with practical examples and questions to test your knowledge. Keep practicing, and soon, using operators in Java will become second nature!
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊