Day 21 Task: Important Docker Interview Questions

Table of contents
- Questions:
- What is the difference between an Image, Container, and Docker Engine?
- What is the difference between the Docker command COPY vs ADD?
- What is the difference between the Docker command CMD vs RUN?
- How will you reduce the size of a Docker image?
- Why and when should you use Docker?
- Explain the Docker Components and How They Interact with Each Other
- Explain the terminology: Docker Compose, Dockerfile, Docker Image, Docker Container.
- In what real scenarios have you used Docker?
- Docker vs Hypervisor?
- What are the advantages and disadvantages of using Docker?
- What is a Docker namespace?
- What is a Docker registry?
- What is an entry point?
- How to implement CI/CD in Docker?
- Will data on the container be lost when the Docker container exits?
- What is a Docker swarm?
- What are the Docker commands for the following:
- What are the common Docker practices to reduce the size of Docker images?
- How do you troubleshoot a Docker container that is not starting?
- Can you explain the Docker networking model?
- Types of Network Drivers
- Two options for persistent data storage available with Docker are volumes and bind mounts.
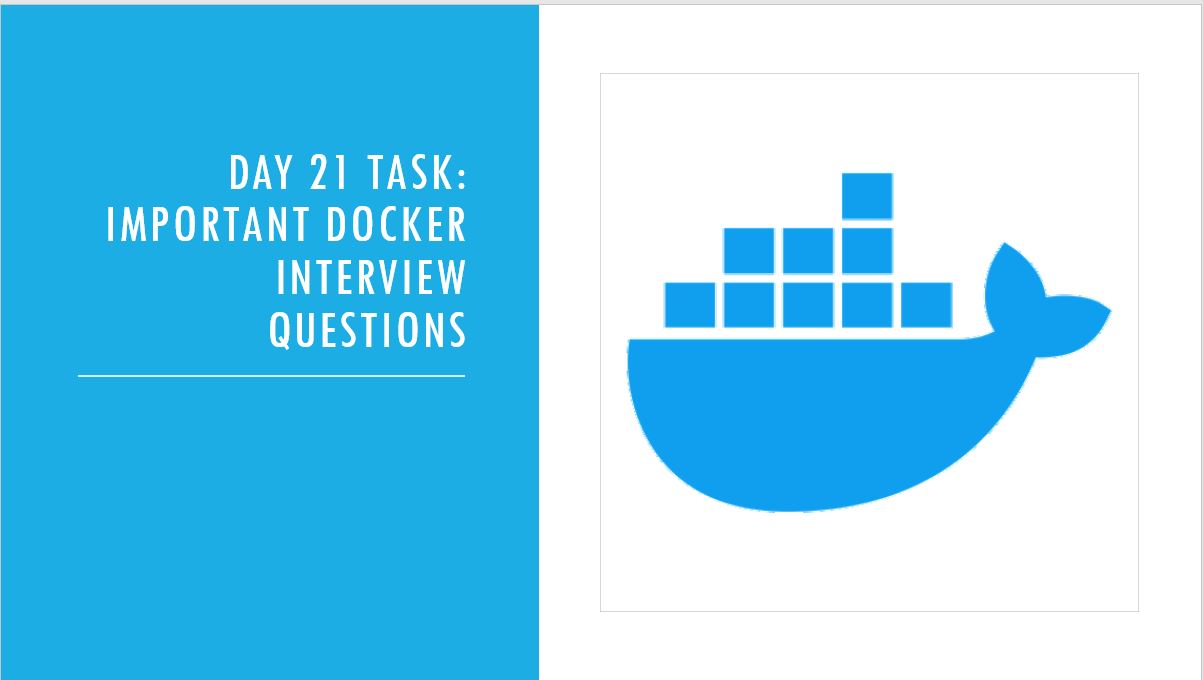
Questions:
What is the difference between an Image, Container, and Docker Engine?
Docker Image: A Docker Image is a read-only, static template that contains all the instructions necessary to create a container. It includes the application code, dependencies, environment variables, and configuration files required to run a piece of software.
Immutable: Once created, an image does not change. Any modifications require building a new image.
Layered Structure: Docker images are made up of a series of layers, where each layer represents a change or instruction (e.g., installing software, copying files). These layers are cached, making subsequent builds faster.
Registry: Images are stored in repositories (e.g., Docker Hub), from where they can be pulled and used to create containers.
Docker Container: A Docker Container is a runnable instance of a Docker Image. When you run an image, it becomes a container, which can be started, stopped, moved, or deleted. Containers encapsulate everything needed to run an application, ensuring consistency across different environments.
Isolated: Containers run in their own isolated environments, including their own file system, network interface, and process tree. However, they share the kernel of the host operating system.
Ephemeral: By default, containers are ephemeral. Once a container is stopped, it can be restarted, but any changes made to the container's filesystem during its runtime are lost unless they are committed to a new image or stored in a volume.
Lightweight: Containers are more lightweight than virtual machines because they share the host system's OS kernel, rather than requiring a full operating system for each instance.
Docker Engine: Docker Engine is the underlying client-server technology that creates and manages Docker images, containers, networks, and storage volumes. It’s the core component that allows the entire Docker ecosystem to function.
Components:
Docker Daemon (
dockerd
): The Docker Daemon is the server-side process that listens for Docker API requests and manages Docker objects (containers, images, networks, etc.). It does the heavy lifting of building, running, and managing Docker containers.Docker Client (
docker
): The Docker Client is the command-line tool that users interact with to send commands to the Docker Daemon, such as building images, running containers, or managing resources.REST API: The Docker REST API allows developers and tools to interact programmatically with the Docker Daemon, enabling automation and integration into CI/CD pipelines.
What is the difference between the Docker command COPY vs ADD?
COPY Command: The COPY
command is used to copy files and directories from the host filesystem to the filesystem of the Docker image.
COPY <source-path> <destination-path>
Usage:
COPY
is a straightforward command that only supports copying files and directories.It does not perform any additional processing on the files, such as extraction or downloading.
It’s generally preferred when you just need to copy local files or directories into the image.
ADD Command: The ADD
command is more powerful than COPY
and is capable of additional functionalities.
Use ADD
when you need its extra features, like downloading files from URLs or automatically extracting archives. However, if you don't need these features, it is better to use COPY
to avoid unintentional side effects.
Usage:
Copying Files: Like
COPY
, it can copy files and directories from the host to the image.Remote URLs:
ADD
can also handle URLs as sources. If the source is a URL,ADD
will download the file from the internet and place it in the destination directory.Archive Extraction: If the source is a compressed archive file (e.g.,
.tar
,.gz
,.zip
),ADD
will automatically extract its contents into the destination directory.
ADD <source-path> <destination-path>
What is the difference between the Docker command CMD vs RUN?
RUN Command: The RUN
command is used to execute commands in a new layer on top of the current image and commit the results. It is commonly used to install software packages, set up the environment, or perform any other actions that are necessary for building the image.
RUN <command>
Usage:
RUN
is used during the build process of the Docker image.Every
RUN
command creates a new layer in the Docker image.It’s typically used to install dependencies, download files, and perform other tasks that need to be done before the image is run.
CMD Command: The CMD
command is used to specify the default command that will be run when a container is started from the image. It sets the command that gets executed by default when the container runs, but this can be overridden by specifying a different command when running the container.
CMD ["executable","param1","param2"]
Usage:
CMD
is used to provide defaults for an executing container.There can only be one
CMD
in a Dockerfile. If you include multipleCMD
commands, only the last one will be used.CMD
can be overridden by passing a command in thedocker run
command.
How will you reduce the size of a Docker image?
Use a Smaller Base Image.
Minimize the Number of Layers
Use Multi-Stage Builds
Remove Unnecessary Files
Use
.dockerignore
File
Why and when should you use Docker?
Docker is a powerful tool that allows you to create, deploy, and run applications in isolated environments called containers. Here's why and when you should use Docker:
Why Use Docker?
Isolation:
- Docker containers run in isolation from one another and from the host system. This means that dependencies, libraries, and configurations in one container do not interfere with those in another container.
Portability:
- Docker containers can run on any platform that supports Docker, whether it's a developer’s laptop, a test server, or a production cloud environment. This makes Docker extremely portable and versatile.
Efficiency:
- Containers are lightweight and share the host OS's kernel, making them more efficient than traditional virtual machines (VMs) that require their own OS. This leads to faster startup times and less overhead.
Simplified Deployment:
- Docker allows you to package an application and its dependencies into a single image. This image can be deployed and run on any machine with Docker, making the deployment process simple and reliable.
Explain the Docker Components and How They Interact with Each Other
Docker Daemon: This is the heart of Docker. It runs on the host machine and is responsible for building, running, and managing containers. It listens for API requests and handles container orchestration.
Docker CLI: The command-line interface is used to interact with the Docker Daemon. Commands like
docker run
,docker build
, anddocker ps
are executed through the CLI.Docker Images: Images are read-only templates used to create containers. They include everything needed to run an application. Images are built using Dockerfiles and stored in Docker registries.
Docker Containers: Containers are instances of Docker images. They provide an isolated environment for applications to run, using the resources and libraries defined in the image.
Docker Registry: A Docker registry stores Docker images. Docker Hub is a public registry, but you can also use private registries to store your images securely.
Docker Compose: Docker Compose is a tool for defining and running multi-container applications. You use a
docker-compose.yml
file to configure your application’s services, networks, and volumes.
Explain the terminology: Docker Compose, Dockerfile, Docker Image, Docker Container.
Docker Compose A tool that simplifies the management of multi-container Docker applications. You define your application’s services, networks, and volumes in a
docker-compose.yml
file. It helps you start and manage all components of your application with a single command.Dockerfile: A text file with a set of instructions used to build a Docker image. Each instruction in the Dockerfile creates a layer in the image, which is then used to create containers.
Docker Image: A lightweight, standalone package that includes everything needed to run an application—code, runtime, libraries, and environment variables. Images are used to create containers.
Docker Container: A runnable instance of a Docker image. Containers encapsulate the application and its dependencies in an isolated environment, making it easy to deploy and manage applications.
In what real scenarios have you used Docker?
Development Environment: Developers use Docker to create consistent development environments that mirror production systems, eliminating discrepancies between environments.
Microservices Architecture: Docker is ideal for deploying microservices, where each service runs in its own container. This makes it easier to manage, scale, and deploy each microservice independently.
Testing: Docker containers provide isolated environments for running tests, ensuring that tests run consistently and independently of the host system.
Continuous Deployment: Docker is used in CI/CD pipelines to automate the build, test, and deployment processes, ensuring faster and more reliable releases.
Docker vs Hypervisor?
Docker: The code doesn’t work on the other system due to the difference in computer Environments. Docker is the world’s leading software container platform.
Docker is a tool designed to make it easier to create, deploy, and run applications by using containers.
Docker containers are lightweight alternatives to Virtual Machines and it uses the host OS
You don’t have to pre-allocate any RAM in containers.
Virtualization : A virtual version of something, such as an operating system (OS), server, storage device, or network resources, is created instead of the real-life counterpart. A virtual system is created by virtualization using software that mimics hardware capabilities. By using this technique, IT companies may run several operating systems, numerous virtual systems, and a variety of applications on a single server.
What are the advantages and disadvantages of using Docker?
Advantages of Docker:
Portability:
Docker containers can run consistently across different environments (development, staging, production) on various operating systems and cloud providers, eliminating environment-specific configuration issues.
Scalability:
Easily scale applications by deploying multiple containers on a single host, allowing for efficient resource utilization and rapid scaling based on demand.
Rapid Deployment:
Docker enables fast deployment of applications due to its lightweight containerization, significantly reducing deployment time.
Isolation:
Each container is isolated from other containers and the host system, enhancing security by preventing conflicts and minimizing the impact of vulnerabilities.
Consistency:
Docker ensures consistent application behavior across different environments by packaging all dependencies within the container.
Disadvantages of Docker:
Learning Curve:
Understanding Docker concepts and best practices can initially be challenging for new users.
Resource Overhead:
Managing a large number of containers can lead to increased resource consumption on the host machine, especially when dealing with CPU, memory, and network usage.
Security Concerns:
Improper configuration or usage of Docker containers can expose security vulnerabilities if not handled carefully.
Limited Orchestration Capabilities (without additional tools):
Docker alone might not be sufficient for managing large-scale container deployments without utilizing tools like Kubernetes.
What is a Docker namespace?
A Docker namespace is a fundamental feature in Docker that provides isolation to containers. Namespaces create an isolated environment for each container, ensuring that the processes running inside a container do not interfere with processes in other containers or on the host system.
PID Namespace: Isolates process IDs, so processes in one container cannot see or interact with processes in another.
Network Namespace: Provides isolated network interfaces and configurations, so containers have their own IP addresses and network settings.
Mount Namespace: Isolates filesystem mounts, allowing containers to have their view of the filesystem.
UTS Namespace: Allows containers to have their own hostname and domain name, which is useful for ensuring unique network identities.
What is a Docker registry?
A Docker registry is a system for storing and distributing Docker images with specific names. There may be several versions of the same image, each with its own set of tags. A Docker registry is separated into Docker repositories, each of which holds all image modifications. The registry may be used by Docker users to fetch images locally and to push new images to the registry (given adequate access permissions when applicable). The registry is a server-side application that stores and distributes Docker images. It is stateless and extremely scalable.
What is an entry point?
ENTRYPOINT is one of the many instructions you can write in a dockerfile. The ENTRYPOINT instruction is used to configure the executables that will always run after the container is initiated. For example, you can mention a script to run as soon as the container is started.
ENTRYPOINT ["nginx", "-g", "daemon off;"]
How to implement CI/CD in Docker?
Continuous Integration: Set up Docker to build images and run tests automatically when code changes are pushed. Use CI tools like Jenkins, GitLab CI/CD, or GitHub Actions to automate the process.
Continuous Deployment: Automate the deployment of Docker containers to staging and production environments. Use orchestration tools like Kubernetes or Docker Swarm to manage and scale deployments.
Pipeline Example: In a CI/CD pipeline, you might use Docker to build a new image, run tests inside containers, and then deploy the image to a staging environment for further testing before promoting it to production.
Will data on the container be lost when the Docker container exits?
Yes, by default, any data stored in a container’s filesystem will be lost when the container exits. To persist data across container restarts or to share data between containers, you should use Docker volumes or bind mounts. Volumes are stored on the host filesystem but managed by Docker, while bind mounts allow you to mount host directories into containers.
What is a Docker swarm?
Docker Swarm is a native clustering and orchestrating tool that helps in managing the a docker engines. In this the group of docker engines turned into as single virtual docker host. It facilitates the users to deploy, manage and scale the applications seamlessly across multiple docker nodes. It comes with providing the features such as service discovery, load balancing, scaling, and rolling updates. It provides an easy and integrated way to manage the containerized applications in a cluster.
What are the Docker commands for the following:
- Viewing running containers
docker ps -a
- Running a container under a specific name
docker run --name <name> <image>
- Exporting a Docker image
docker save -o <filename>.tar <image>
- Importing an existing Docker image
docker load -i <filename>.tar
- Deleting a container
docker remove <container_name or container id>
- Removing all stopped containers, unused networks, build caches, and dangling images?
docker system prune -a
What are the common Docker practices to reduce the size of Docker images?
Multi-Stage Builds: Use multi-stage builds to separate the build environment from the runtime environment, ensuring only the final artifacts are included in the image.
Minimize Layers: Combine commands into single
RUN
instructions to reduce the number of layers in the image.Smaller Base Images: Use minimal base images like
alpine
to keep your images lean.Clean Up: Remove unnecessary files and package manager caches in the same
RUN
instruction to avoid leaving temporary files in the image.Efficient Dependencies: Include only the necessary dependencies and libraries in your image to reduce its size.
How do you troubleshoot a Docker container that is not starting?
docker logs <container_name>
Can you explain the Docker networking model?
A network is a group of two or more devices that can communicate with each other either physically or virtually. The Docker network is a virtual network created by Docker to enable communication between Docker containers. If two containers are running on the same host they can communicate with each other without the need for ports to be exposed to the host machine.
Types of Network Drivers
bridge: If you build a container without specifying the kind of driver, the container will only be created in the bridge network, which is the default network.
host: Containers will not have any IP address they will be directly created in the system network which will remove isolation between the docker host and containers.
none: IP addresses won’t be assigned to containers. These containments are not accessible to us from the outside or from any other container.How do you manage persistent storage in Docker?
Two options for persistent data storage available with Docker are volumes and bind mounts.
Volumes are preferred to manage persistent data as they are created and managed by Docker, thus isolated from the core functionality of the host machine. Volumes can be stored in a part of the host filesystem managed by Docker (/var/lib/docker/volumes/), on remote hosts, or on cloud providers, and offer better performance than bind mounts.
With bind mounts, data on the host machine is mounted into a container. This process relies heavily on the host machine having a specific file structure, thus offering limited functionality when compared to volumes.
Follow for more updates:)
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.