Understanding Vue.js ( v-model ) A Complete Guide

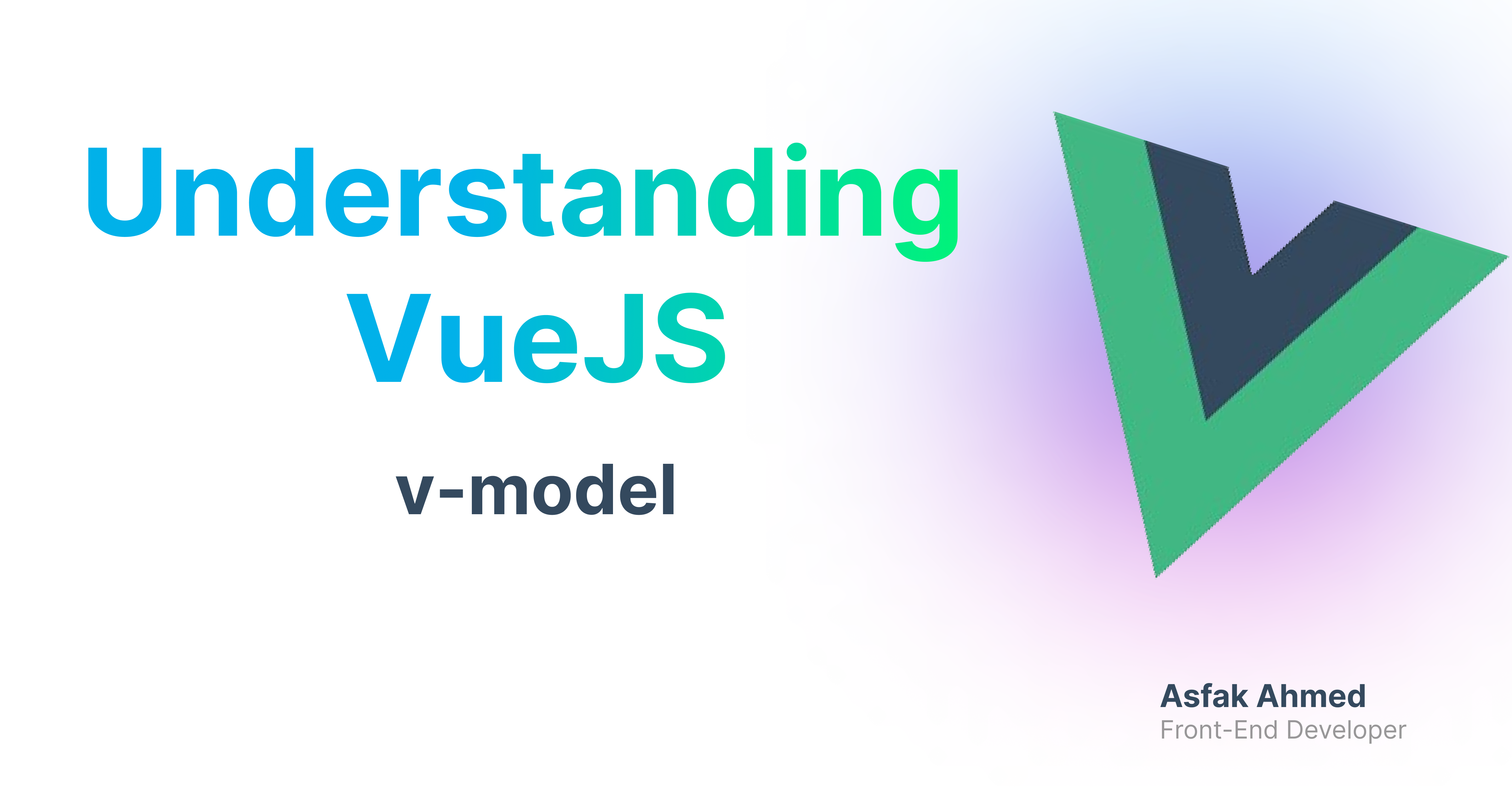
Vue.js provides a robust system for building dynamic, reactive interfaces, and one of the most frequently used directives is v-model
. This directive allows for seamless two-way data binding between form inputs and the application's state, making it easier to handle user input and state synchronization.
In this article, we will take a deep dive into v-model
, covering how it works, when and why to use it, and how it simplifies common tasks in Vue applications. We’ll also look at some code examples to illustrate the power and flexibility of v-model
.
What is v-model
?
v-model
is a directive in Vue.js that provides two-way data binding between the value of form input elements (like <input>
, <textarea>
, and <select>
) and Vue's application state (data or component state).
This means that when the user updates the form input, the bound data in the Vue instance is automatically updated, and when the data changes in Vue, the form input value is updated as well.
Key Points:
Two-way data binding: Changes in the input are reflected in the data, and changes in the data are reflected in the input.
Simplifies state management for form inputs in Vue.js applications.
Works with various input types including text, checkboxes, radio buttons, selects, and even custom components.
Why Use v-model
?
Ease of use:
v-model
eliminates the need to manually listen for input events and update the data. You don’t need to write separate event listeners or watchers for input fields—v-model
handles everything.Synchronization: It ensures automatic synchronization between the form inputs and the data model. This is particularly useful in forms where you need to keep your state in sync with user input.
Less boilerplate code: With
v-model
, you can avoid writing repetitive event handling code, reducing the overall complexity of your Vue.js applications.
When to Use v-model
?
Forms: Whenever you’re dealing with forms, such as login forms, registration forms, or any interface where users input data,
v-model
is extremely handy.Custom Components: You can even use
v-model
to bind data in custom Vue components, making them more reusable and flexible.
How v-model
Works
v-model
is essentially syntactic sugar for binding a value and listening to input events. Under the hood, it works like this:
Binding the
value
property of the input to a data property.Listening for input events to update the bound data property.
Here’s a simple example of v-model
usage:
Example 1: Text Input
<template>
<div>
<label for="name">Enter your name:</label>
<input type="text" id="name" v-model="name" />
<p>Your name is: {{ name }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const name = ref('');
</script>
Explanation:
v-model="name"
creates a two-way data binding between the input field and thename
property in the data object.As the user types into the input field, the
name
property is updated, and the<p>
element reflects the current value ofname
.
Example 2: Checkbox Input
v-model
also works with checkboxes, keeping track of whether they are checked or unchecked:
<template>
<div>
<label>
<input type="checkbox" v-model="isSubscribed" /> Subscribe to newsletter
</label>
<p>{{ isSubscribed ? 'Thank you for subscribing!' : 'Please subscribe.' }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const isSubscribed = ref(false);
</script>
Explanation:
The
v-model="isSubscribed"
binds the checkbox’s checked state to theisSubscribed
variable.When the checkbox is checked or unchecked, the value of
isSubscribed
is updated automatically, and the message displayed changes accordingly.
Example 3: Radio Buttons
Radio buttons are also supported by v-model
. Here's an example where the selected gender is updated:
<template>
<div>
<label>
<input type="radio" value="male" v-model="gender" /> Male
</label>
<label>
<input type="radio" value="female" v-model="gender" /> Female
</label>
<p>Selected gender: {{ gender }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const gender = ref('');
</script>
Explanation:
v-model="gender"
binds the selected radio button’s value to thegender
property.As the user selects a gender, the
gender
variable is updated to either "male" or "female".
Example 4: Select Dropdown
You can bind a v-model
to a select element to track the selected option:
<template>
<div>
<label for="fruit">Choose a fruit:</label>
<select id="fruit" v-model="selectedFruit">
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
</select>
<p>You selected: {{ selectedFruit }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const selectedFruit = ref('');
</script>
Explanation:
The
v-model="selectedFruit"
binds the selected value of the dropdown to theselectedFruit
variable.When the user selects an option, the
selectedFruit
is updated accordingly.
Using v-model
with Custom Components
One of the most powerful features of v-model
is that it can be used with custom Vue components. This allows you to create reusable form components that can still take advantage of two-way data binding.
Example 5: v-model
in a Custom Component
Here’s how you can implement v-model
in a custom component:
<!-- Parent Component -->
<template>
<div>
<custom-input v-model="message" />
<p>Message: {{ message }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
import CustomInput from './CustomInput.vue';
const message = ref('');
</script>
<!-- CustomInput.vue -->
<template>
<input type="text" :value="modelValue" @input="$emit('update:modelValue', $event.target.value)" />
</template>
<script setup>
const props = defineProps({
modelValue: String
});
</script>
Explanation:
In the custom component (
CustomInput.vue
), we use:value="modelValue"
to bind the input’s value to themodelValue
prop.We listen for the
input
event and emit anupdate:modelValue
event to notify the parent component of changes. This enables two-way binding in the parent component usingv-model
.
Modifiers in v-model
Vue provides modifiers that can be used with v-model
to handle specific cases. Some common modifiers include:
.lazy
: Updates the model only when the input loses focus, rather than on each keystroke..number
: Automatically converts the input value to a number..trim
: Automatically trims any leading or trailing whitespace from the input.
Example 6: v-model
Modifiers
<template>
<div>
<label for="age">Enter your age:</label>
<input id="age" v-model.number="age" type="text" />
<p>Your age is: {{ age }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const age = ref('');
</script>
Explanation:
- The
.number
modifier ensures that the input is always treated as a number, converting the string input into a number.
Benefits of v-model
Simplifies Form Handling: With
v-model
, managing user input in forms becomes effortless. You don’t need to manually bind and track the state of each form field.Automatic Synchronization: It provides automatic synchronization between the DOM and the Vue component state, making the UI responsive to user input.
Less Boilerplate Code: You can avoid writing additional code for event handling and state updates, as
v-model
takes care of it for you.
The v-model
directive is one of the most powerful and commonly used features in Vue.js for managing user input. It simplifies two-way data binding, making form handling much easier and more intuitive. By automatically syncing the form fields with the Vue instance’s data, v-model
significantly reduces boilerplate code, allowing you to focus on building more complex and interactive features. Whether you're working with simple form fields or custom components, v-model
offers an elegant solution for handling input and keeping your UI and data in sync.
Subscribe to my newsletter
Read articles from Asfak Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfak Ahmed
Asfak Ahmed
I'm Asfak Ahmed, a Front-End Developer with 2+ years of experience, currently enhancing user experiences at Doplac CRM. As the founder of ZenUI Library, I focus on creating intuitive, user-centric interfaces. My passion lies in blending creativity with technology to craft impactful digital solutions.