Unlock the Power of JavaScript Closures: A Comprehensive Guide

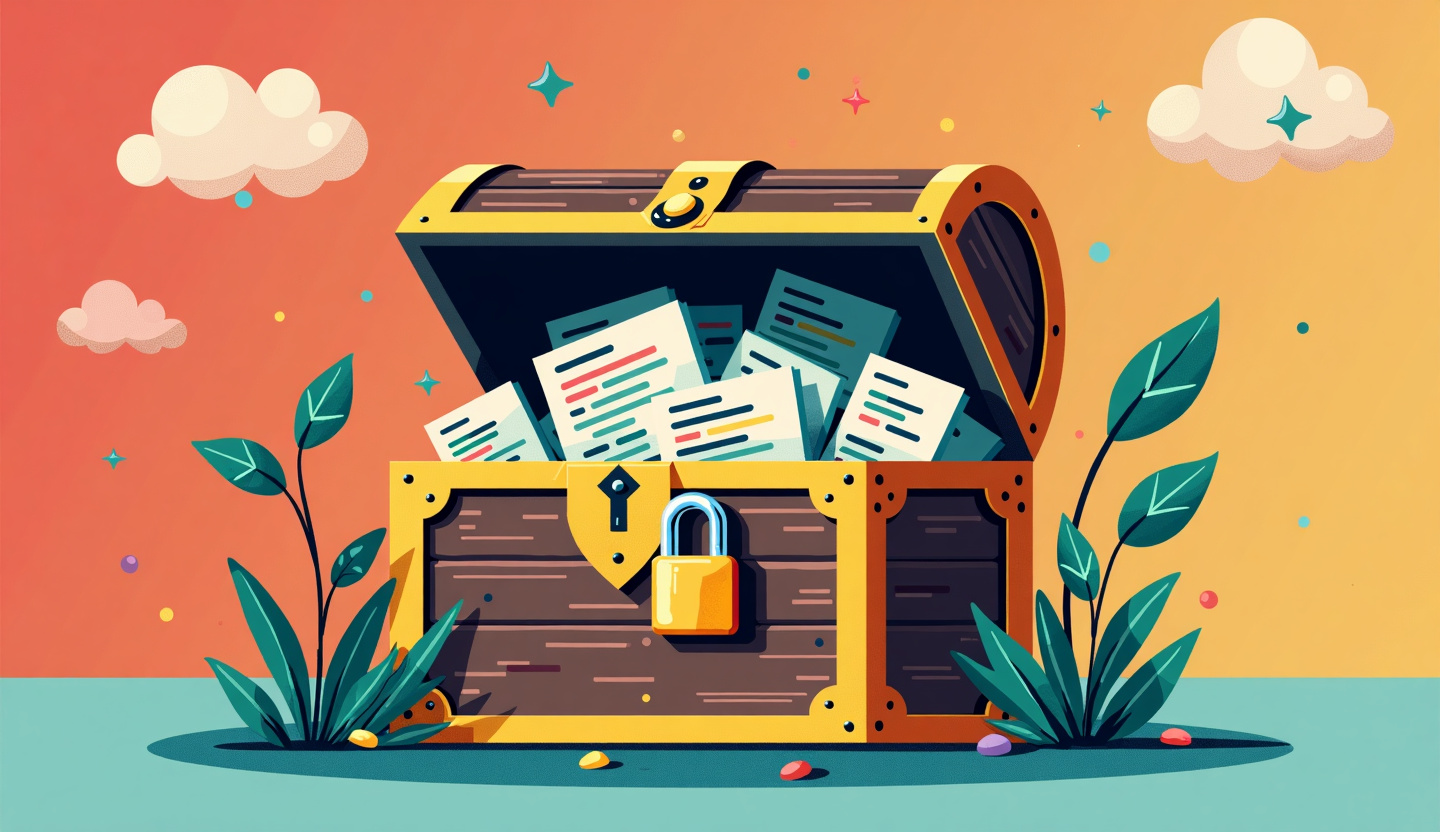
Introduction JavaScript closures are a core concept in modern web development. Mastering them is key to writing efficient, maintainable, and secure code. Whether you're handling event listeners, managing data privacy, or optimizing performance, closures can make your JavaScript code more powerful. In this guide, we’ll break down what closures are, how they work, and showcase practical examples to help you understand and leverage them effectively.
1. What Are JavaScript Closures?
A closure is a function that remembers the variables from its outer scope, even when the function is executed outside that scope. It’s a way for functions to "close over" their lexical environment, making variables available even after they’ve been "closed" off from their original scope.
Example:
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log(`Outer: ${outerVariable}`);
console.log(`Inner: ${innerVariable}`);
};
}
const newFunction = outerFunction('outside');
newFunction('inside');
// Output:
// Outer: outside
// Inner: inside
In the example above, the inner function retains access to the variable outerVariable
, even after outerFunction
has finished executing. This is the essence of a closure.
2. Understanding Scope in JavaScript
Before diving deeper into closures, it’s essential to understand scope. Scope in JavaScript refers to the context in which variables are declared and determines their visibility and lifetime.
Global Scope: Variables declared outside any function are in the global scope.
Local/Function Scope: Variables declared inside a function are in the local scope.
Block Scope: Variables declared using
let
orconst
inside a block ({}
) are limited to that block.
Closures capture variables from these scopes and keep them alive, even when the function that created them is no longer in execution.
3. How Closures Work: A Deep Dive
Closures work by preserving the lexical environment of a function. This means a function can access variables from the outer scope, even after that outer function has returned.
Step-by-step Breakdown:
A function creates another function within its body.
The inner function references variables from the outer function.
Even after the outer function is called, the inner function retains access to those variables.
Here’s a more in-depth example:
function counter() {
let count = 0;
return function increment() {
count++;
return count;
};
}
const myCounter = counter();
console.log(myCounter()); // 1
console.log(myCounter()); // 2
console.log(myCounter()); // 3
In this example, myCounter
retains access to the count
variable, even after counter()
has executed, because increment()
forms a closure around the count
variable.
4. Practical Use Cases of Closures
Event Handlers
Closures are often used in event handlers to maintain a reference to variables even after the event has been triggered:
function addClickEvent(buttonId) {
let count = 0;
document.getElementById(buttonId).addEventListener('click', function() {
count++;
console.log(`Button clicked ${count} times`);
});
}
addClickEvent('myButton');
Here, the event listener holds a closure over the count
variable, even though the addClickEvent
function has already completed execution.
Data Privacy & Encapsulation
Closures can be used to hide variables from the global scope, providing data privacy:
function privateCounter() {
let count = 0;
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
}
};
}
const counterObj = privateCounter();
counterObj.increment(); // 1
counterObj.increment(); // 2
counterObj.decrement(); // 1
In this case, count
is private and can only be modified by increment
and decrement
methods. This is a common pattern for encapsulation.
Asynchronous Programming
Closures are incredibly useful in asynchronous programming, especially when dealing with callbacks or promises:
function delayedLogger(message) {
setTimeout(function() {
console.log(message);
}, 1000);
}
delayedLogger("Hello after 1 second!");
Even though delayedLogger
finishes execution, the message is logged after a second because the closure retains access to the message
variable.
5. Common Pitfalls of Closures and How to Avoid Them
While closures are powerful, they can also lead to unintended consequences, such as memory leaks or unexpected behavior in loops. Let’s discuss some common pitfalls and how to avoid them.
Memory Leaks
Closures can inadvertently cause memory leaks if they keep unnecessary variables alive. To avoid this, ensure you’re not retaining references to variables you no longer need.
Loop Issues
Closures in loops can cause unexpected behavior due to how they capture variables. Here’s a typical problem:
for (var i = 1; i <= 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// Output: 6, 6, 6, 6, 6
To fix this, use let
instead of var
, as let
is block-scoped:
for (let i = 1; i <= 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// Output: 1, 2, 3, 4, 5
6. Hands-On Coding Exercises
Exercise 1: Create a Counter
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter1 = createCounter();
console.log(counter1()); // 1
console.log(counter1()); // 2
const counter2 = createCounter();
console.log(counter2()); // 1
Exercise 2: Button Click Tracker
Modify the following code to track how many times a button is clicked:
function clickTracker(buttonId) {
let count = 0;
document.getElementById(buttonId).addEventListener('click', function() {
count++;
console.log(`Clicked ${count} times`);
});
}
clickTracker('myButton');
7. Conclusion: Mastering JavaScript Closures
Closures are an essential part of JavaScript that help you manage scope, preserve state, and build more maintainable code. From event handlers to private variables, closures offer flexibility in your coding logic. Practice and understanding will allow you to harness their power effectively, avoiding common pitfalls while taking full advantage of their capabilities.
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
