Master Prototypical Inheritance: The Secret to Efficient JavaScript

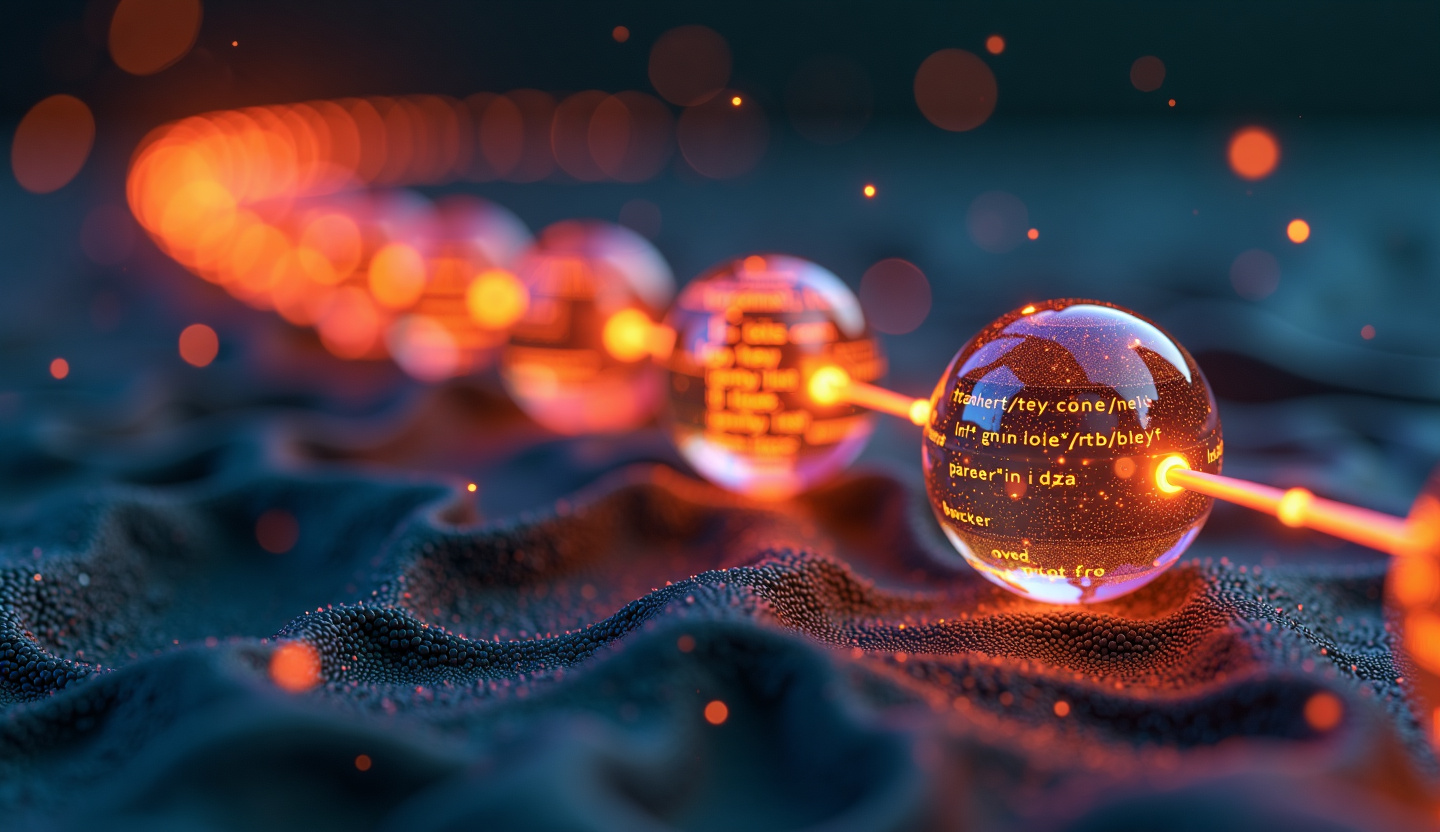
Introduction
Prototypical inheritance is a core feature of JavaScript, often misunderstood but highly powerful when it comes to optimizing your code. Unlike classical inheritance, which you might be familiar with in other programming languages, JavaScript's inheritance is prototype-based. Understanding how to implement and utilize prototypical inheritance effectively can dramatically improve your JavaScript skills, helping you write more maintainable and efficient code. In this article, we’ll explore the ins and outs of prototypical inheritance, how to implement it, and real-world scenarios to see it in action.
1. What is Prototypical Inheritance in JavaScript?
Prototypical inheritance allows JavaScript objects to inherit properties and methods from another object. In JavaScript, every object has a hidden property called [[Prototype]]
that points to another object (or null
). This is the mechanism behind inheritance in JavaScript.
For example, if object A
inherits from object B
, and B
has a method, A
can access that method as if it were its own. When a property or method is not found in an object, JavaScript checks the object's prototype to see if it exists there. This process continues up the chain until the property or method is found or the prototype chain ends.
Understanding the Prototype Chain
Consider this simple example:
let animal = {
sound: "roar",
speak: function() {
console.log(this.sound);
}
};
let lion = Object.create(animal);
lion.speak(); // "roar"
In this example, lion
inherits the speak
method from the animal
object. Even though lion
doesn't have its own speak
method, JavaScript looks up the prototype chain and finds it in animal
.
2. How to Implement Prototypical Inheritance
There are different ways to implement inheritance in JavaScript using prototypes. Let's break them down:
Using Object.create()
The most direct way to create an object that inherits from another is by using Object.create()
. This method creates a new object, setting the first parameter as the prototype of that object.
let car = {
wheels: 4,
drive: function() {
console.log("Driving...");
}
};
let sportsCar = Object.create(car);
sportsCar.isConvertible = true;
console.log(sportsCar.wheels); // 4
sportsCar.drive(); // "Driving..."
In the example above, sportsCar
inherits the properties and methods from car
. You can add additional properties, such as isConvertible
, directly to the sportsCar
object.
Constructor Functions and Prototypes
Another way to implement prototypical inheritance is by using constructor functions. Constructor functions are typically used with the new
keyword to create instances of objects.
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + " makes a sound.");
};
let dog = new Animal("Dog");
dog.speak(); // "Dog makes a sound."
In this example, we define a constructor function Animal
and then assign a method to its prototype. This ensures that all instances of Animal
can share the same speak
method, rather than each instance having its own copy.
The class
Syntax (ES6)
Since ES6, JavaScript has introduced the class
syntax, which provides a more familiar and cleaner way to work with inheritance, but it's important to note that it's just syntactic sugar over JavaScript's prototypical inheritance model.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
constructor(name) {
super(name);
}
speak() {
console.log(`${this.name} barks.`);
}
}
let dog = new Dog("Max");
dog.speak(); // "Max barks."
In this example, Dog
inherits from Animal
using the extends
keyword, and the super()
function is used to call the parent class's constructor.
3. Real-World Scenarios of Prototypical Inheritance
Prototypical inheritance is not just a theoretical concept; it's widely used in real-world JavaScript applications. Let's explore some common scenarios.
Scenario 1: Extending Objects in Libraries
Many JavaScript libraries and frameworks use prototypical inheritance to extend existing objects and add new functionalities. For example, jQuery extends the base Element
prototype to add utility methods.
Scenario 2: Reusing Methods Across Objects
Suppose you're building a game with different characters. All characters might have a common method like attack
. Instead of duplicating this method across each character, you can define it in a prototype and allow all characters to inherit it.
function Character(name, health) {
this.name = name;
this.health = health;
}
Character.prototype.attack = function() {
console.log(`${this.name} attacks!`);
};
let warrior = new Character("Warrior", 100);
let mage = new Character("Mage", 80);
warrior.attack(); // "Warrior attacks!"
mage.attack(); // "Mage attacks!"
In this example, both warrior
and mage
objects share the same attack
method, saving memory and making the code more maintainable.
4. Advantages of Prototypical Inheritance
Memory Efficiency: With prototypical inheritance, methods and properties are shared between objects rather than being duplicated. This leads to better memory efficiency.
Flexibility: Prototypes are dynamic, meaning you can add or modify methods even after an object is created. This makes it easy to extend functionality as your application grows.
Code Reusability: By leveraging inheritance, you can avoid code duplication and ensure that your objects share common functionality.
5. Common Pitfalls and Best Practices
Pitfall 1: Modifying the Prototype
Be careful when modifying the prototype of an object, especially in libraries or large codebases, as this can have unintended side effects.
Example of a bad practice:
Array.prototype.push = function() {
console.log("Push is called!");
return 0;
};
This modifies the behavior of the push
method for all arrays, which can break existing functionality.
Pitfall 2: Constructor Overriding
When using constructor functions, ensure that you correctly call the parent constructor, especially when inheriting properties.
function Dog(name, breed) {
Animal.call(this, name); // Correct way to inherit the parent's properties
this.breed = breed;
}
Best Practice: Use Object.create()
for Clean Inheritance
When extending objects, prefer using Object.create()
for clean inheritance without affecting other parts of the prototype chain.
6. Hands-on Coding Exercises
Exercise 1: Creating a Prototype Chain
Create an inheritance chain where a Vehicle
class has a Car
subclass, and practice adding methods and properties to the prototype.
function Vehicle(type) {
this.type = type;
}
Vehicle.prototype.drive = function() {
console.log(`${this.type} is driving.`);
};
let truck = new Vehicle("Truck");
truck.drive(); // "Truck is driving."
Exercise 2: Using class
and extends
Write a class that inherits from another class and overrides one of the methods.
class Animal {
constructor(name) {
this.name = name;
}
eat() {
console.log(`${this.name} is eating.`);
}
}
class Rabbit extends Animal {
eat() {
console.log(`${this.name} is munching on carrots.`);
}
}
let rabbit = new Rabbit("Bunny");
rabbit.eat(); // "Bunny is munching on carrots."
7. Conclusion: Mastering Prototypical Inheritance
Prototypical inheritance is one of JavaScript’s most powerful features when used correctly. By understanding how the prototype chain works and knowing the different ways to implement inheritance, you can write more efficient and maintainable JavaScript code. Whether you use Object.create()
, constructor functions, or the modern class
syntax, prototypical inheritance provides the flexibility and power to extend objects and share functionality across instances.
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
