Write Cleaner JavaScript with Functional Programming & Immutability

Table of contents
- 1. What is Functional Programming in JavaScript?
- 2. Key Principles of Functional Programming
- 3. The Role of Immutability in Functional Programming
- 4. Using map, reduce, and filter to Write Cleaner Code
- 5. Real-World Examples of Functional Programming in JavaScript
- 6. Functional Programming vs. Object-Oriented Programming
- 7. Conclusion: Functional Programming and Immutability in Practice
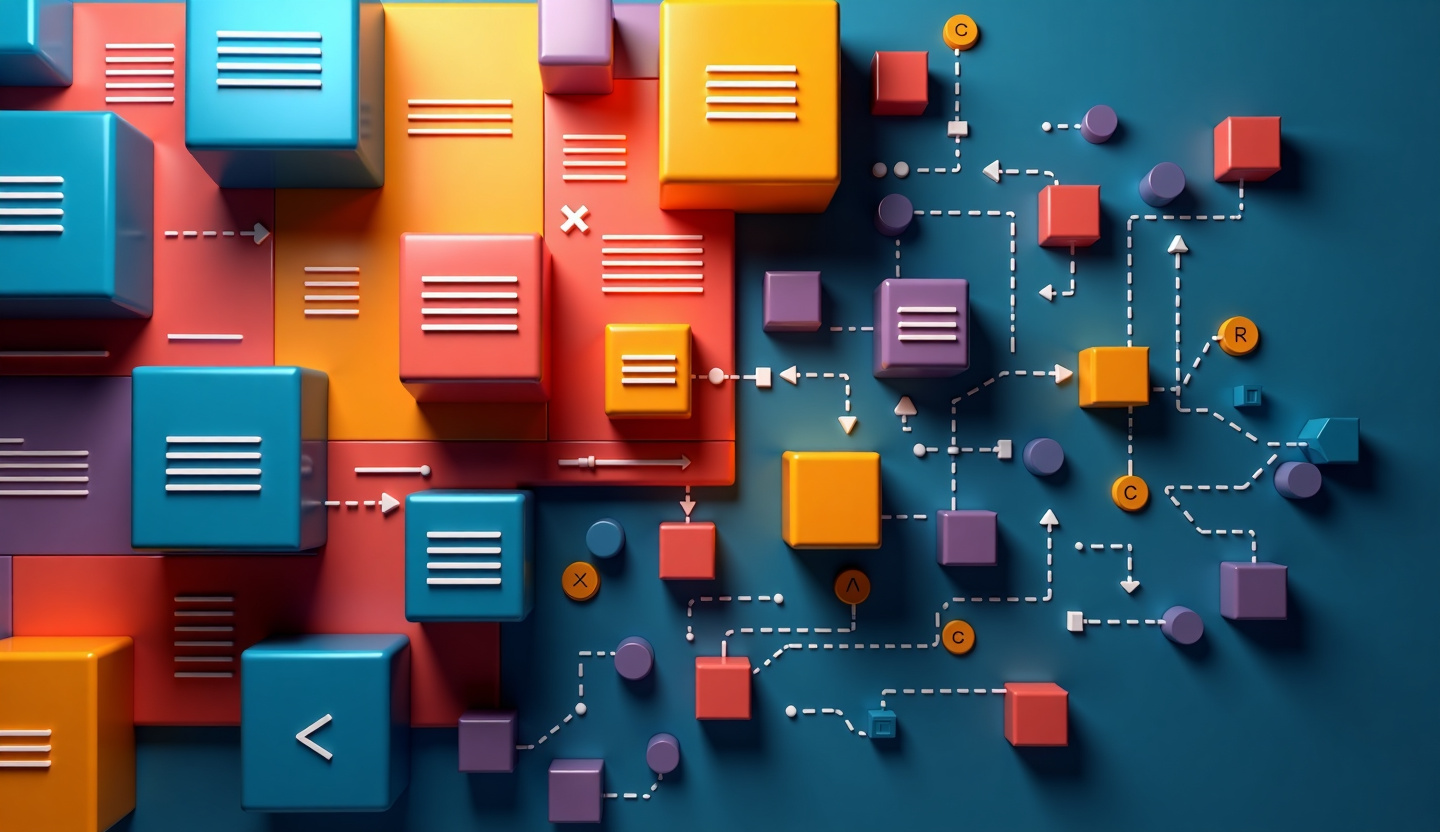
Introduction
Writing clean, maintainable code is one of the most important skills for any developer, and functional programming (FP) in JavaScript offers an elegant way to achieve this. By embracing core functional programming principles and leveraging immutability, you can ensure that your code is predictable, easy to test, and less prone to bugs. In this article, we’ll dive deep into the world of functional programming in JavaScript, exploring key principles, the importance of immutability, and essential methods like map
, reduce
, and filter
that can help you write cleaner code.
1. What is Functional Programming in JavaScript?
Functional programming (FP) is a programming paradigm focused on building software by composing pure functions, avoiding shared state, and utilizing immutability. In FP, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
JavaScript, while not a purely functional language, supports many functional programming concepts that you can use to write more declarative and predictable code.
Here’s a simple comparison between imperative (non-functional) and functional approaches:
Imperative (Non-Functional) Approach:
let numbers = [1, 2, 3, 4, 5];
let doubled = [];
for (let i = 0; i < numbers.length; i++) {
doubled.push(numbers[i] * 2);
}
console.log(doubled); // [2, 4, 6, 8, 10]
Functional Approach:
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
In the functional approach, you describe what should happen rather than how it should happen, making the code cleaner and easier to maintain.
2. Key Principles of Functional Programming
1. Pure Functions
A pure function is a function where the output solely depends on the input and has no side effects. This means no changes to external variables or states. Pure functions are predictable and easy to test.
// Pure Function
function add(a, b) {
return a + b;
}
// Impure Function (Has side effects)
let total = 0;
function addToTotal(a) {
total += a;
}
Pure functions are a core principle in functional programming as they guarantee that the same input will always produce the same output.
2. Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return functions as results. They allow for more abstract and reusable code.
function higherOrder(func) {
return func(5);
}
function multiplyByTwo(x) {
return x * 2;
}
console.log(higherOrder(multiplyByTwo)); // 10
Functions like map
, filter
, and reduce
are examples of higher-order functions in JavaScript.
3. Avoiding Side Effects
Side effects occur when a function modifies some state or interacts with the outside world, such as logging to the console or modifying a global variable. Functional programming aims to minimize side effects, leading to more predictable and bug-free code.
3. The Role of Immutability in Functional Programming
Immutability refers to the concept that once an object is created, it cannot be changed. Instead of modifying the existing object, you create a new one with the desired changes. This idea complements functional programming, where functions should avoid mutating data.
Why Immutability Matters
Immutability helps create predictable code by ensuring that data cannot be changed unexpectedly. When working with shared state in larger applications, it’s easy for bugs to emerge when one part of the program inadvertently changes data. Immutability prevents these issues by ensuring that data remains constant.
Using Immutability in JavaScript
JavaScript arrays and objects are mutable by default, but you can use techniques and libraries to enforce immutability. For example, using the spread
operator allows you to create new arrays and objects without modifying the original ones.
let person = { name: 'John', age: 25 };
// Creating a new object without modifying the original
let updatedPerson = { ...person, age: 26 };
console.log(person); // { name: 'John', age: 25 }
console.log(updatedPerson); // { name: 'John', age: 26 }
By always creating new objects or arrays when updating data, you can ensure that your code remains immutable.
4. Using map
, reduce
, and filter
to Write Cleaner Code
These methods are at the heart of functional programming in JavaScript. They allow you to transform and manipulate arrays in a declarative way, improving the readability and cleanliness of your code.
Using map()
The map()
method creates a new array by applying a function to every element in an existing array.
let numbers = [1, 2, 3, 4, 5];
let squared = numbers.map(num => num * num);
console.log(squared); // [1, 4, 9, 16, 25]
Using reduce()
The reduce()
method applies a function to each element of an array, resulting in a single output value. It's often used for aggregating values, such as summing up an array of numbers.
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((accumulator, current) => accumulator + current, 0);
console.log(sum); // 15
Using filter()
The filter()
method creates a new array with all elements that pass the test implemented by the provided function.
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4]
By combining these methods, you can write clear, functional code that’s easy to understand and maintain.
5. Real-World Examples of Functional Programming in JavaScript
Example 1: Manipulating Data
Imagine you have an array of objects representing products, and you need to get an array of discounted prices for products over $100. You can use filter()
, map()
, and reduce()
to achieve this.
let products = [
{ name: 'Laptop', price: 1200 },
{ name: 'Phone', price: 700 },
{ name: 'Tablet', price: 300 }
];
let discountedPrices = products
.filter(product => product.price > 100)
.map(product => product.price * 0.9);
console.log(discountedPrices); // [1080, 630, 270]
This functional approach makes it clear what the code is doing at each step and avoids side effects.
Example 2: Aggregating Data
Suppose you want to calculate the total price of all products. You can use reduce()
for this:
let totalPrice = products.reduce((total, product) => total + product.price, 0);
console.log(totalPrice); // 2200
This declarative approach is more concise and readable than using a loop.
6. Functional Programming vs. Object-Oriented Programming
Object-oriented programming (OOP) and functional programming are two different paradigms that can sometimes be combined in JavaScript. While OOP focuses on objects and their behaviors, FP emphasizes functions and immutable data.
Differences:
OOP uses classes and inheritance to organize code. It involves mutating object states.
FP is focused on avoiding side effects and using pure functions to transform data.
You can apply both paradigms in JavaScript, but functional programming is often preferred for writing cleaner, more maintainable code.
7. Conclusion: Functional Programming and Immutability in Practice
Functional programming principles and immutability allow developers to write cleaner, more maintainable code in JavaScript. By using pure functions, avoiding side effects, and leveraging built-in methods like map()
, reduce()
, and filter()
, you can improve the predictability and readability of your code. Immutability ensures that your data stays constant, preventing unintended bugs and making debugging easier.
If you haven’t yet embraced functional programming in your JavaScript journey, now’s the perfect time to start. Your code will not only become more predictable and testable but also more scalable as your projects grow in complexity.
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
