Build a Dynamic Pagination Component in React: A Step-by-Step Guide

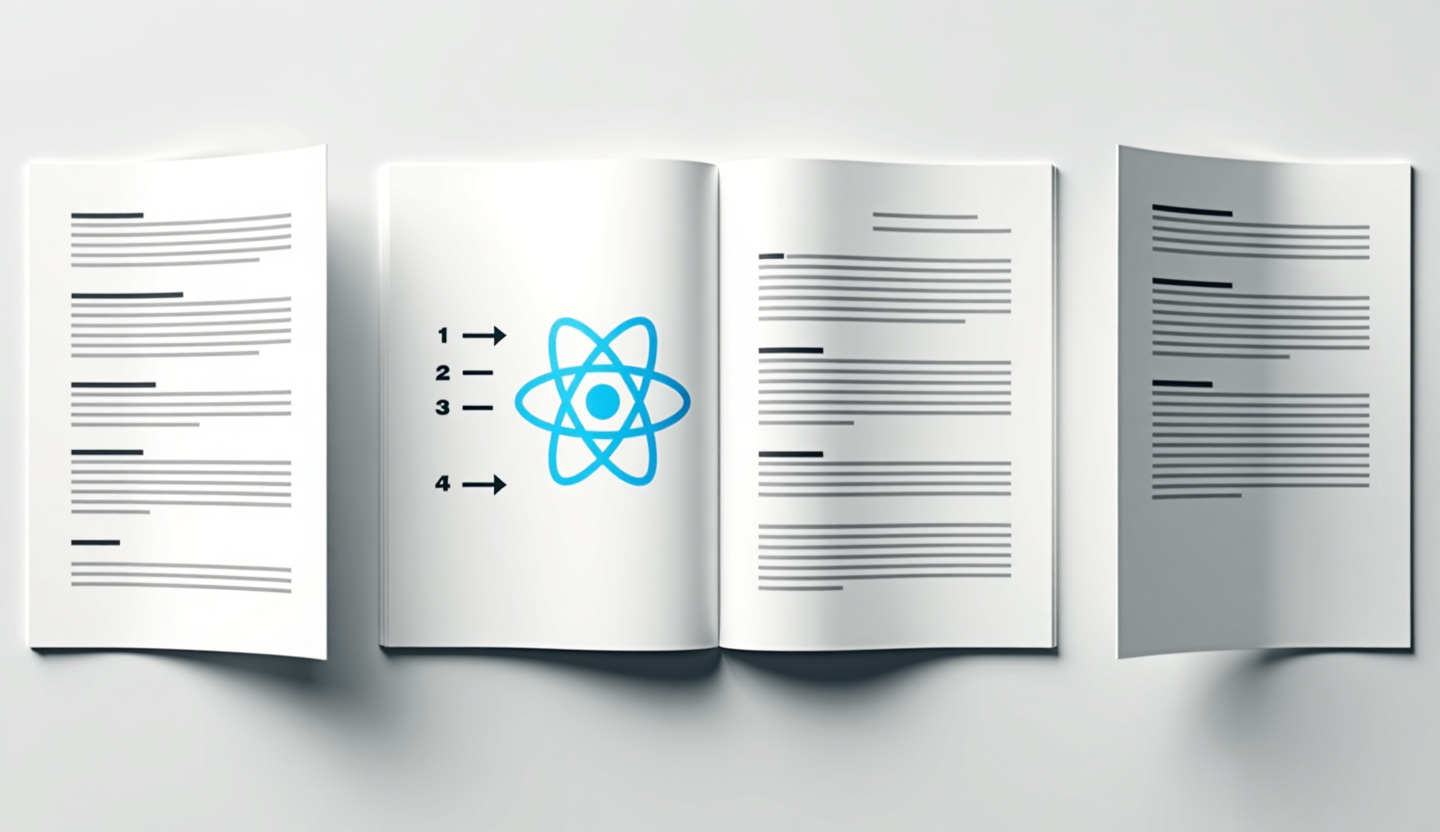
Introduction
Pagination is a crucial feature for improving the user experience (UX) when working with large datasets in web applications. Instead of overwhelming users with too much information at once, pagination breaks content into digestible chunks, making navigation more efficient and organized. In this step-by-step guide, we’ll walk you through building a dynamic pagination component in React from scratch. By the end, you’ll have a reusable pagination component that enhances UX, efficiently handles large datasets, and is fully customizable for various use cases.
1. What Is Pagination & Why Does It Matter?
Pagination refers to dividing content into discrete pages to improve navigation and performance, especially when dealing with large datasets. In modern web applications, pagination helps:
Enhance user experience by reducing the cognitive load.
Improve performance by rendering only a subset of data at a time.
Make the interface clean, preventing users from scrolling through endless lists.
Whether it's an e-commerce product listing, blog post archive, or search result, pagination is essential for displaying information in a structured manner. In React applications, creating a flexible pagination system will allow users to smoothly navigate through data without unnecessary delays.
2. Setting Up the React Project
Before we dive into building the pagination component, let’s quickly set up a React project. If you don’t have a React environment ready, follow these steps:
Open your terminal and create a new React project using
create-react-app
:npx create-react-app react-pagination cd react-pagination
Start the development server:
npm start
You should see a basic React app running in your browser at
http://localhost:3000
.
Now that we have a working environment, let’s create our pagination component.
3. Building the Pagination Component
To create a pagination component, we need to keep track of the current page, the total number of items, and how many items we want to display per page. Let’s start by defining these states and building the basic structure of our pagination component.
Step 1: Set Up Pagination Logic
Create a new file called Pagination.js
in the src
directory:
import React, { useState } from "react";
const Pagination = ({ itemsPerPage, totalItems, paginate }) => {
const [currentPage, setCurrentPage] = useState(1);
const totalPages = Math.ceil(totalItems / itemsPerPage);
const handlePageChange = (page) => {
setCurrentPage(page);
paginate(page);
};
return (
<nav>
<ul className="pagination">
{[...Array(totalPages).keys()].map(page => (
<li key={page + 1} className={`page-item ${currentPage === page + 1 ? 'active' : ''}`}>
<button onClick={() => handlePageChange(page + 1)} className="page-link">
{page + 1}
</button>
</li>
))}
</ul>
</nav>
);
};
export default Pagination;
Step 2: Implement Pagination in the Main Component
Next, import and use this Pagination
component inside your main component where you are rendering your list of items. We’ll implement a basic data set for demonstration purposes.
import React, { useState } from "react";
import Pagination from "./Pagination";
const data = Array.from({ length: 100 }, (_, index) => `Item ${index + 1}`);
const App = () => {
const [currentPage, setCurrentPage] = useState(1);
const itemsPerPage = 10;
const indexOfLastItem = currentPage * itemsPerPage;
const indexOfFirstItem = indexOfLastItem - itemsPerPage;
const currentItems = data.slice(indexOfFirstItem, indexOfLastItem);
const paginate = (pageNumber) => setCurrentPage(pageNumber);
return (
<div className="App">
<h1>React Pagination Demo</h1>
<ul>
{currentItems.map(item => (
<li key={item}>{item}</li>
))}
</ul>
<Pagination
itemsPerPage={itemsPerPage}
totalItems={data.length}
paginate={paginate}
/>
</div>
);
};
export default App;
Explanation:
Pagination.js
dynamically generates page numbers based on the total items and items per page.In
App.js
, we manage the data slicing and pass thepaginate
function to thePagination
component to change pages.
4. Handling Large Datasets Efficiently
When dealing with large datasets, it’s crucial to only fetch and render the items that are visible on the current page. The technique of loading a portion of data is called "lazy loading" or "server-side pagination."
Server-Side Pagination:
Instead of fetching the entire dataset at once, you can send a request to the server with the current page number and limit (items per page). This way, the server returns only the necessary data, reducing the load on both the client and the server.
Example of a server-side request using the fetch
API:
const fetchItems = async (page, itemsPerPage) => {
const response = await fetch(`/api/items?page=${page}&limit=${itemsPerPage}`);
const data = await response.json();
setItems(data.items);
};
This approach ensures that you’re efficiently handling large datasets and only loading the necessary data for each page.
5. Styling the Pagination Component
Styling the pagination component can improve its visibility and make it user-friendly. You can use CSS or libraries like Bootstrap to quickly style the component.
Here’s a simple CSS styling for the pagination component:
.pagination {
display: flex;
list-style: none;
justify-content: center;
padding: 0;
}
.page-item {
margin: 0 5px;
}
.page-link {
padding: 8px 12px;
cursor: pointer;
border: 1px solid #ddd;
background-color: #f9f9f9;
color: #333;
}
.page-item.active .page-link {
background-color: #007bff;
color: white;
}
To include the CSS, create a Pagination.css
file, and import it into Pagination.js
:
import './Pagination.css';
This will give your pagination a clean, centered layout with intuitive color changes when a page is active.
6. Adding Interactivity and UX Enhancements
To make the pagination component more interactive and user-friendly, you can add additional features such as:
Next and Previous buttons.
First and Last page links for quicker navigation.
A dropdown to change the number of items per page.
Here’s how you can extend the Pagination
component to include Next
and Previous
buttons:
const Pagination = ({ itemsPerPage, totalItems, paginate }) => {
const [currentPage, setCurrentPage] = useState(1);
const totalPages = Math.ceil(totalItems / itemsPerPage);
const handlePageChange = (page) => {
if (page < 1 || page > totalPages) return;
setCurrentPage(page);
paginate(page);
};
return (
<nav>
<ul className="pagination">
<li className={`page-item ${currentPage === 1 ? 'disabled' : ''}`}>
<button onClick={() => handlePageChange(currentPage - 1)} className="page-link">Previous</button>
</li>
{[...Array(totalPages).keys()].map(page => (
<li key={page + 1} className={`page-item ${currentPage === page + 1 ? 'active' : ''}`}>
<button onClick={() => handlePageChange(page + 1)} className="page-link">
{page + 1}
</button>
</li>
))}
<li className={`page-item ${currentPage === totalPages ? 'disabled' : ''}`}>
<button onClick={() => handlePageChange(currentPage + 1)} className="page-link">Next</button>
</li>
</ul>
</nav>
);
};
This adds buttons to move forward and backward through pages, enhancing the user’s ability to navigate data quickly.
7. Conclusion
Building a dynamic pagination component in React not only enhances the performance of your web applications but also significantly improves the user experience. From handling large datasets to optimizing user navigation, the pagination component is a fundamental feature for any scalable React application.
By following this guide, you now have the skills to:
Understand the importance of pagination.
Build a reusable, dynamic pagination component in React.
Efficiently handle large datasets by implementing server-side pagination.
Add interactivity and style the component to improve UX.
With pagination in place, your applications will be faster, more user-friendly, and ready to handle large-scale data operations. Happy coding!
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
