Crafting Elegant Code with Custom React Hooks

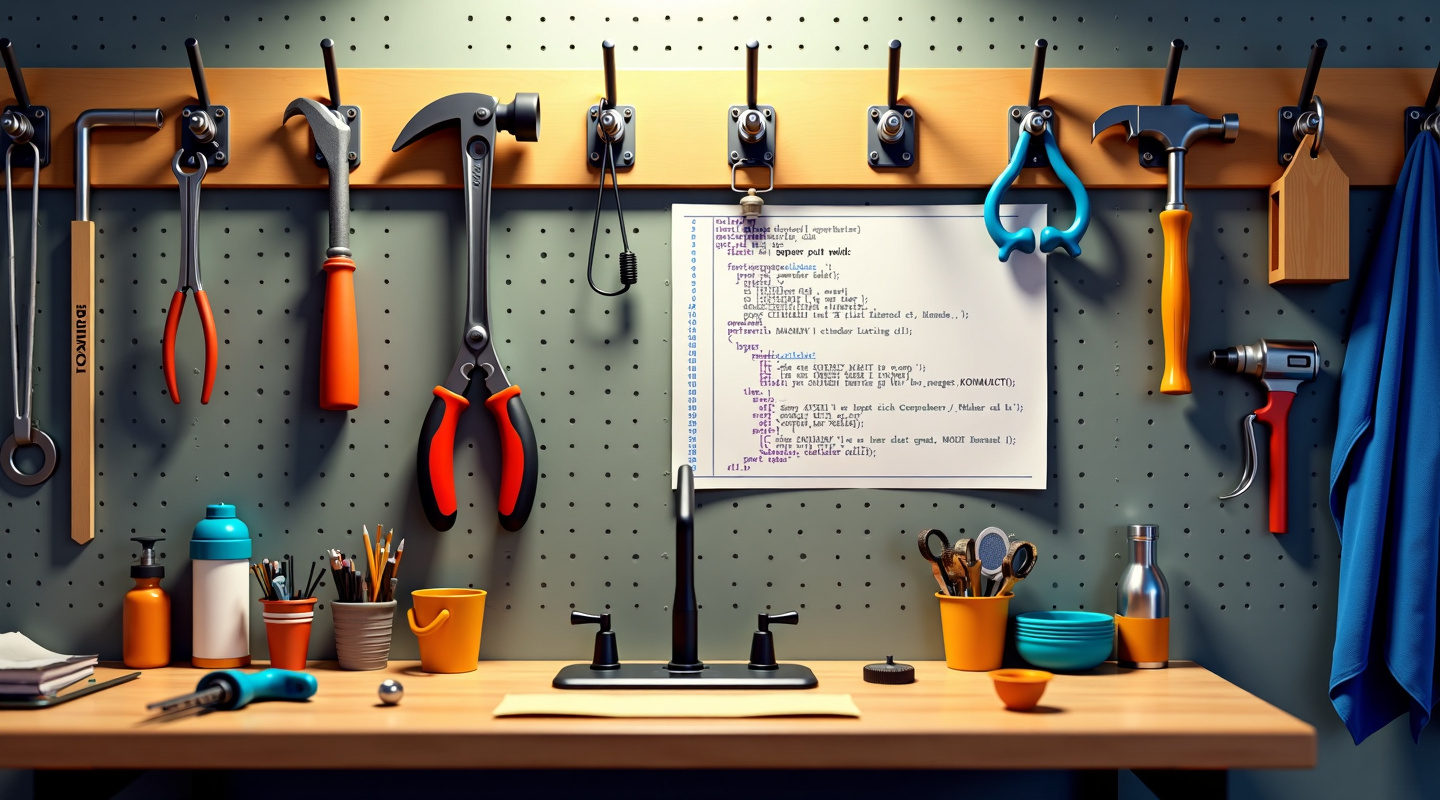
Introduction
React hooks revolutionized the way developers build modern web applications, offering a powerful tool to manage state, side effects, and other reusable logic inside functional components. Among these hooks, custom React hooks provide an elegant way to organize logic, enhance code reusability, and streamline your components. In this guide, we’ll explore what custom hooks are, their benefits, and how to create them from scratch. We’ll also dive into some best practices and examples to ensure you’re crafting clean, efficient code in your React projects.
1. What Are Custom React Hooks & Their Benefits?
Custom React hooks are functions that let you extract reusable logic from components. They start with the word use
(e.g., useFetch
, useForm
, etc.) and allow you to encapsulate common behaviors or side effects that multiple components may share.
Some key benefits include:
Reusability: You can share logic across components without duplicating code.
Separation of Concerns: Custom hooks help separate UI and business logic, making your components leaner and more readable.
Code Organization: Instead of having bloated components, hooks help modularize specific behaviors, like fetching data or handling form state, into small, testable units.
React provides built-in hooks like useState
, useEffect
, and useContext
, but custom hooks let you define reusable functionality that goes beyond built-in behavior.
2. Creating Your First Custom Hook
Let’s start by creating a simple custom hook that manages the logic for toggling a boolean value.
Example: useToggle Hook
import { useState } from "react";
function useToggle(initialValue = false) {
const [value, setValue] = useState(initialValue);
const toggle = () => setValue((prevValue) => !prevValue);
return [value, toggle];
}
export default useToggle;
Here’s how it works:
The
useToggle
hook manages a boolean state (value
) and provides a function (toggle
) to flip its state betweentrue
andfalse
.You can reuse this hook across multiple components wherever toggling is required, like toggling a modal, dropdown, or any other UI element.
Using the useToggle Hook in a Component
import React from "react";
import useToggle from "./useToggle";
const ToggleComponent = () => {
const [isOn, toggleIsOn] = useToggle(false);
return (
<div>
<button onClick={toggleIsOn}>
{isOn ? "Turn Off" : "Turn On"}
</button>
</div>
);
};
export default ToggleComponent;
In this example, the useToggle
hook is integrated into a component that toggles a button’s label between “Turn On” and “Turn Off.” The logic for toggling is neatly encapsulated within the hook, keeping the component simple and focused on rendering UI.
3. Organizing Logic with Custom Hooks
One of the most compelling advantages of custom hooks is their ability to organize complex logic into small, manageable functions. Hooks act as a central place for handling behaviors such as fetching data, form validation, or subscribing to events.
Example: useFetch Hook
Imagine you’re fetching data from an API in multiple components. Instead of writing the same fetch logic in each component, you can use a custom useFetch
hook.
import { useState, useEffect } from "react";
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
};
export default useFetch;
This useFetch
hook abstracts away the data-fetching logic. You can now reuse this hook in any component where API data is required, improving consistency and minimizing code repetition.
4. Reusability: Maximizing Efficiency with Custom Hooks
Reusability is one of the main reasons to use custom hooks. You can extract logic that’s commonly needed across different components, which leads to cleaner and more efficient code.
Example: useForm Hook
Let’s say you have several forms in your app, and you’re tired of repeating the same state-management logic for form inputs. A custom hook called useForm
can help.
import { useState } from "react";
const useForm = (initialState = {}) => {
const [formData, setFormData] = useState(initialState);
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prev) => ({ ...prev, [name]: value }));
};
const resetForm = () => setFormData(initialState);
return [formData, handleChange, resetForm];
};
export default useForm;
Here’s how you might use useForm
in a login form:
import React from "react";
import useForm from "./useForm";
const LoginForm = () => {
const [formData, handleChange, resetForm] = useForm({ email: "", password: "" });
const handleSubmit = (e) => {
e.preventDefault();
console.log(formData);
resetForm();
};
return (
<form onSubmit={handleSubmit}>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
placeholder="Email"
/>
<input
type="password"
name="password"
value={formData.password}
onChange={handleChange}
placeholder="Password"
/>
<button type="submit">Login</button>
</form>
);
};
export default LoginForm;
With this custom useForm
hook, you can easily handle form input changes and reset form data without duplicating code across multiple forms.
5. Best Practices for Custom Hooks in React
While custom hooks can significantly simplify your React codebase, there are a few best practices to keep in mind:
Prefix Hook Names with “use”: This helps React identify them as hooks. For example, always name your hook something like
useFetch
oruseForm
, never justfetch
orformState
.Keep Hooks Focused: Each custom hook should have a clear, single responsibility. For example,
useFetch
handles data fetching, whileuseForm
manages form state. Don’t overload hooks with too many concerns.Return Useful Values: Ensure your hook returns what the consuming component needs. This might be state, handler functions, or even other hooks.
Handle Cleanup: For hooks with side effects (e.g., subscriptions or API calls), ensure you clean up properly in the
useEffect
hook to avoid memory leaks.Test Custom Hooks: Since hooks are just JavaScript functions, you can (and should) write unit tests for them to ensure they behave correctly.
6. Practical Examples of Custom React Hooks
Here are a few real-world scenarios where custom hooks can be highly useful:
Authentication Management: A
useAuth
hook could manage authentication logic, handle login/logout, and store the authentication state.Window Resize Handling: A
useWindowSize
hook could track window dimensions and provide real-time resizing information.
import { useState, useEffect } from "react";
const useWindowSize = () => {
const [size, setSize] = useState([window.innerWidth, window.innerHeight]);
useEffect(() => {
const handleResize = () => {
setSize([window.innerWidth, window.innerHeight]);
};
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
return size;
};
This hook tracks the window size and can be used to make your application responsive.
7. Conclusion
Custom React hooks are a game-changer when it comes to organizing and reusing logic across your components. By encapsulating complex behaviors like state management, API calls, and event handling into hooks, you can create more efficient and readable code.
In this article, we covered the essentials of custom hooks:
How to build your first custom hook.
Why hooks improve reusability and code organization.
Best practices for ensuring your hooks are well-structured and easy to maintain.
Start implementing custom hooks in your next React project, and you’ll find your codebase more manageable, modular, and elegant.
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
