Simplified OOP Concepts in Java (part-1)
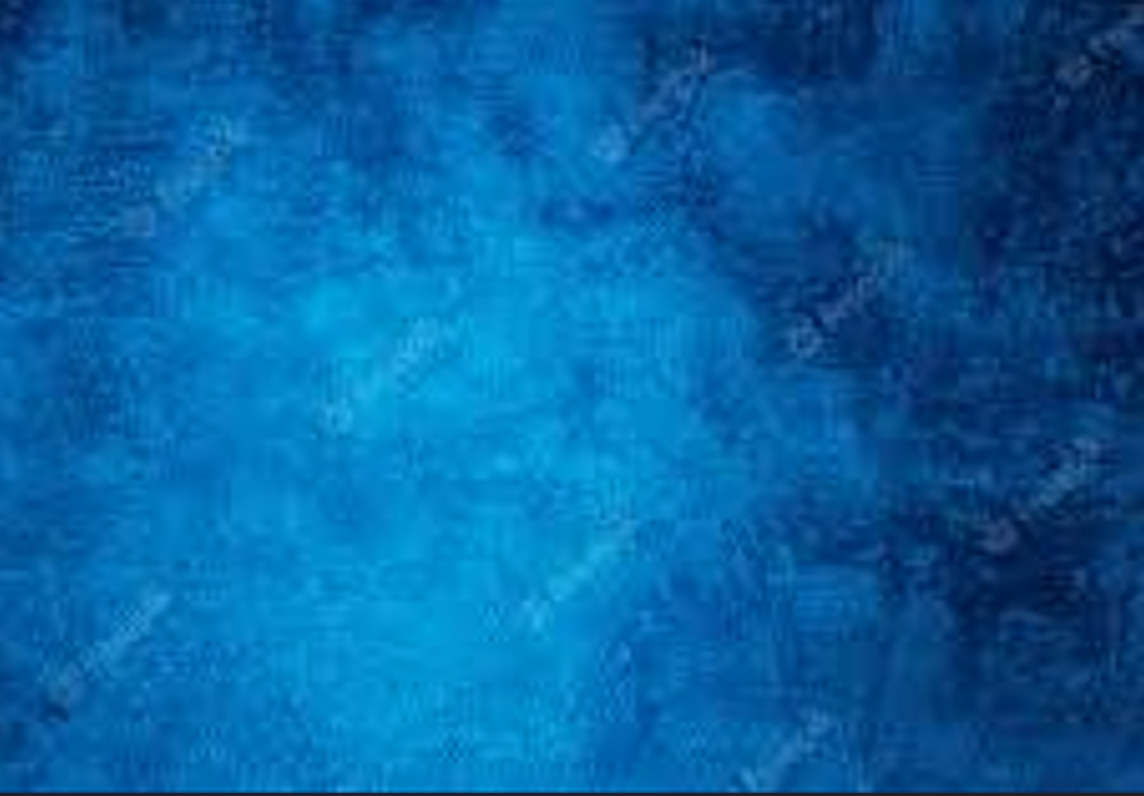
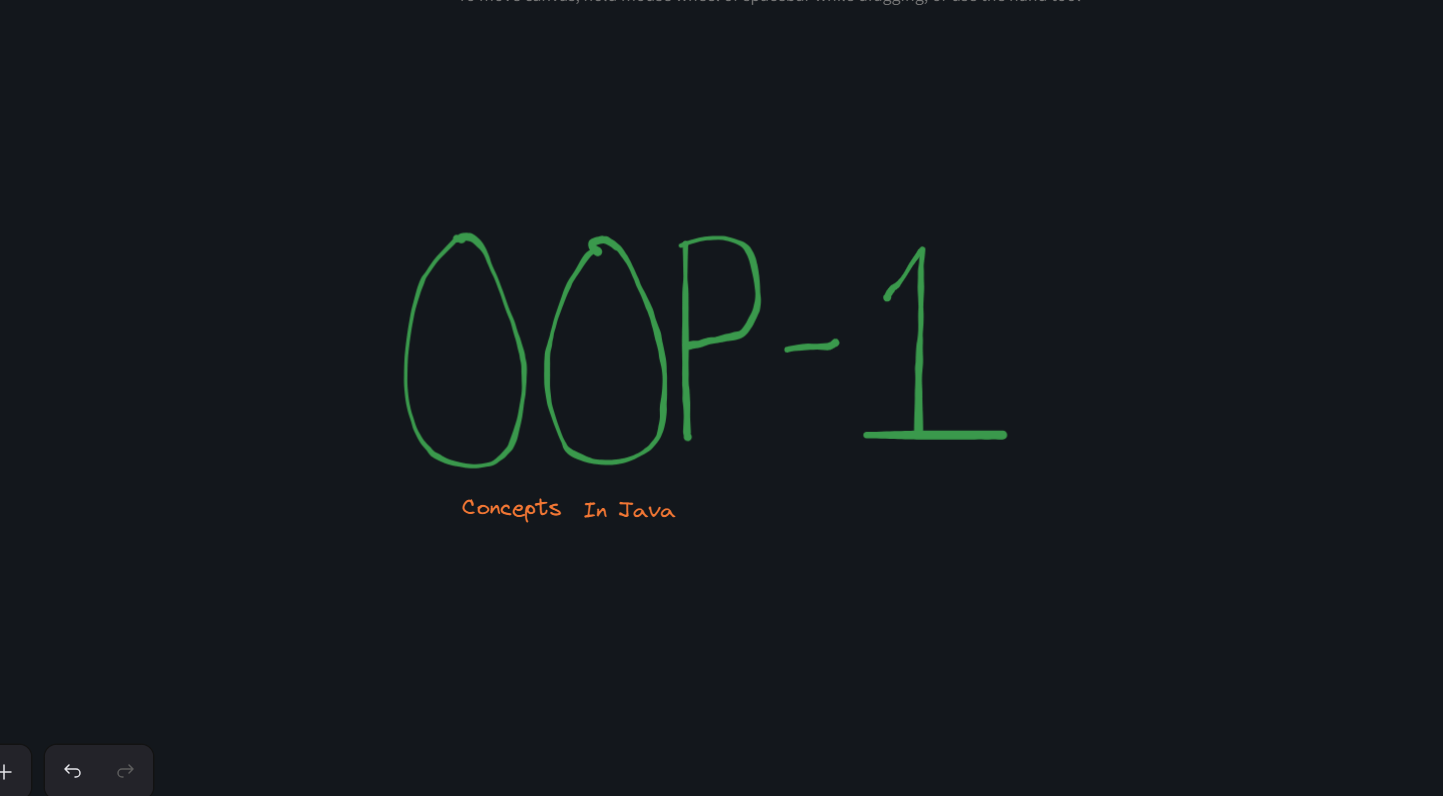
Classes:
Classes are simply user-defined data types. They are used to create objects, which are instances of the class. The class itself is the blueprint for the object.
Creating Classes :
syntax:
class ClassName{
int x;// instance variables
void disp(){ // instance functions
//code
}
// this is the main function which is excecuted when program starts
public static void main(String[] arguments){
//code
}
}
Declaration of Object :
syntax:
ClassName objectName;
This does not create an object but it creates an reference variable in the stack memory.
This reference variable points to null meaning it is equal to null.
In java objects(derived datatypes) are stored in heap and primitives are stored in stack.
Initialisation of Object :
syntax:
ClassName obj = new ClassName();
Here className() is the default constructor in java. U can make your own constructor which will be taught later.
This line of code creates an object of the class.
The new keyword is used to allocate memory dynamically for the class during runtime of the program.
Constructor :
syntax:
ClassName(){ //constructor code } ClassName(args){ //constructor code }
Here,
ClassName()
is the default constructor in Java provided by the compiler. However, when you use constructor overloading, you must also write the code for the default constructor. Otherwise, the program will throw an error.Constructor Overloading :
Using different constructors with the same name but different arguments allows the constructor to be used based on the passed parameters.
Constructor used in copying an Object:
Student(Student other){
this.name=other.name;
this.age=other.age;
}
this keyword :
The "this" keyword is used to access the instance variables inside the constructor or non-static methods.
#NOTE: (About memory Allocation)
Reference variables (identifiers) and primitive types (7 types) are stored in stack memory for quick access.
All other types like arrays and strings are objects stored in the heap, and the references to these objects are stored in the stack.
When we declare an object
Class obj = new Class();
, a reference variable is created in the stack memory during compile time, and this reference variable points to null. But during runtime, the object is created in the heap using the new keyword, and the reference variable points to this object.Since declaration happens during runtime, an uninitialized object reference points to null. You can check this using your debugger.
Now you might have realized that arrays, strings, and ArrayLists are all objects!
There is much more to learn in OOP. I will write more as I learn advanced concepts.
Subscribe to my newsletter
Read articles from Shaikh Faris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
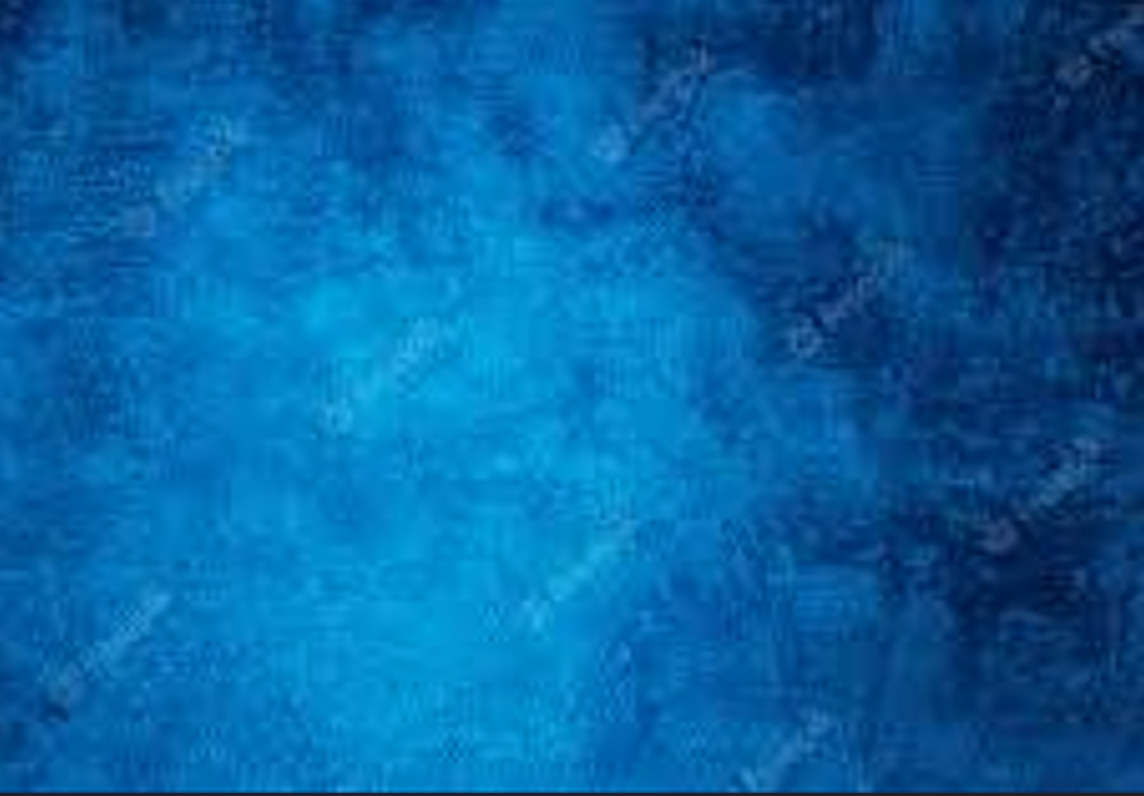