Unlocking the Potential of JavaScript Callbacks: Explained with Examples

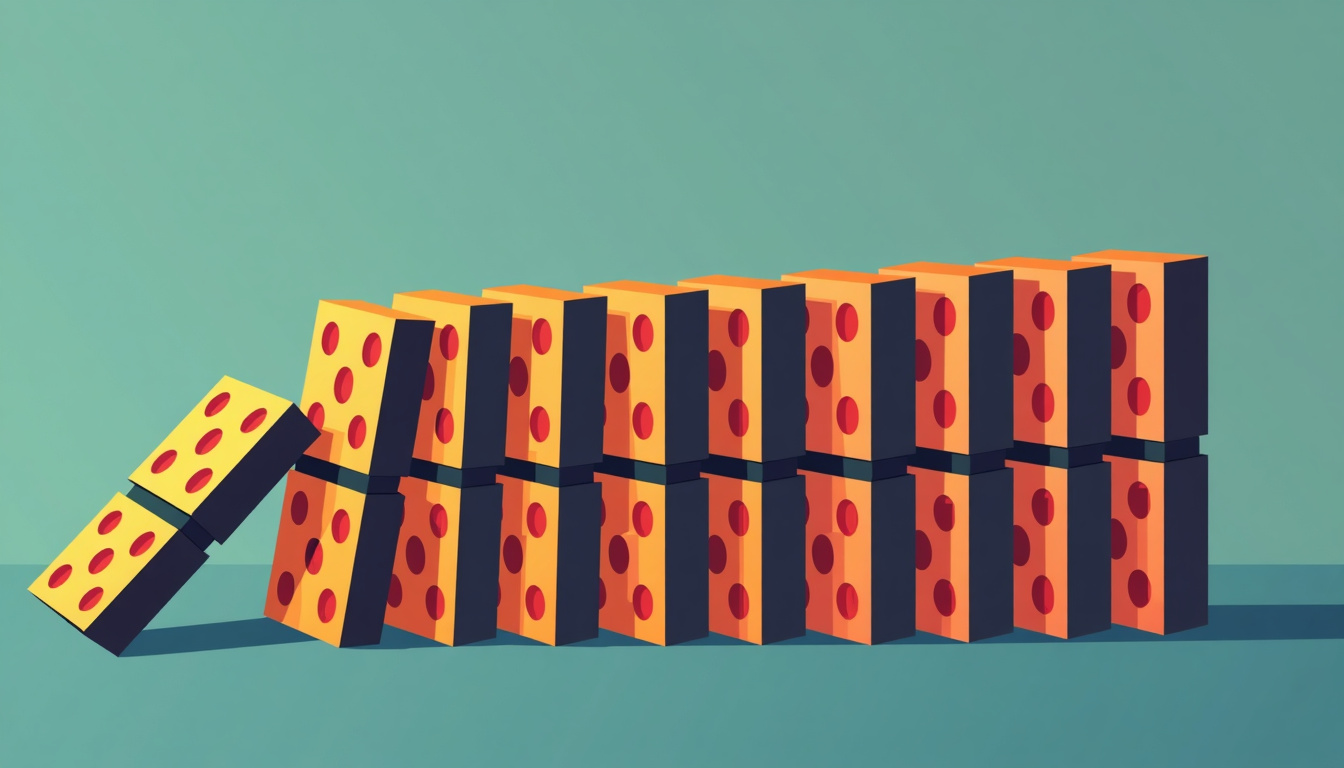
Introduction
JavaScript is a single-threaded, asynchronous programming language. While its synchronous nature can make things simpler, handling long-running operations (like network requests or file reads) requires a non-blocking approach. Enter callbacks, one of the foundational building blocks of asynchronous JavaScript. Callbacks provide a way to ensure certain code runs only after an operation is complete.
In this article, we’ll unlock the potential of JavaScript callbacks, diving into:
What callbacks are and how they work.
The dangers of callback hell and how to avoid it.
Practical examples of how to use callbacks effectively in code.
By the end, you’ll have a firm grasp on how callbacks operate and how to use them effectively for a more responsive, dynamic JavaScript application.
1. What Are Callbacks & How Are They Used in JavaScript?
At its core, a callback is simply a function that is passed as an argument to another function. This allows the receiving function to execute the callback at a certain point, usually after some asynchronous task is completed.
In other words, callbacks are functions that are "called back" once a particular task finishes, ensuring that JavaScript doesn’t block other code while waiting for an operation to complete.
Basic Example of a Callback Function
function fetchData(callback) {
console.log("Fetching data...");
setTimeout(() => {
callback("Data fetched!");
}, 2000);
}
function logData(data) {
console.log(data);
}
fetchData(logData);
In this example, fetchData
simulates fetching data with a setTimeout
function. After 2 seconds, it calls the logData
function, passing the fetched data as an argument. Notice that logData
is passed as a callback to fetchData
, which allows the data to be logged only after the asynchronous operation completes.
When Are Callbacks Used?
Callbacks are often used when dealing with asynchronous operations like:
Fetching data from an API.
Reading or writing files.
Executing timeouts or intervals.
Handling user input events.
These are scenarios where you don’t want the code to wait for an operation to finish before moving on to the next task. Instead, you tell the code, "Once you're done, execute this callback."
2. Understanding & Avoiding Callback Hell
One of the biggest challenges developers face when working with callbacks is what’s known as callback hell. This occurs when multiple nested asynchronous operations use callbacks, resulting in deeply indented and hard-to-read code.
What is Callback Hell?
Here’s an example of callback hell in action:
fetchUserData((user) => {
fetchOrders(user.id, (orders) => {
processOrders(orders, (result) => {
displayResult(result);
});
});
});
In this example:
We fetch the user’s data.
Once that’s complete, we fetch their orders.
After fetching the orders, we process them.
Finally, we display the result.
While this works, the code structure becomes deeply nested and increasingly difficult to maintain as more callbacks are introduced.
Strategies to Avoid Callback Hell
1. Modularize Functions
One way to avoid callback hell is to modularize your code. Break large, nested callbacks into smaller functions that handle individual tasks. This not only makes your code more readable but also easier to maintain.
function getUserData(userId, callback) {
// Simulate an async operation
setTimeout(() => {
console.log("User data fetched!");
callback();
}, 1000);
}
function getUserOrders(callback) {
setTimeout(() => {
console.log("User orders fetched!");
callback();
}, 1000);
}
function processUserOrders(callback) {
setTimeout(() => {
console.log("Orders processed!");
callback();
}, 1000);
}
getUserData(1, () => {
getUserOrders(() => {
processUserOrders(() => {
console.log("All tasks completed!");
});
});
});
By breaking down the code into smaller functions, the structure becomes easier to follow, even when dealing with multiple asynchronous operations.
2. Use Promises
The evolution of JavaScript introduced promises as an alternative to callbacks. Promises offer a more readable, structured way to handle asynchronous operations, helping you avoid callback hell. If you find yourself heavily nesting callbacks, consider switching to promises (or the newer async/await syntax).
Here’s the same example using promises:
function getUserData(userId) {
return new Promise((resolve) => {
setTimeout(() => {
console.log("User data fetched!");
resolve();
}, 1000);
});
}
function getUserOrders() {
return new Promise((resolve) => {
setTimeout(() => {
console.log("User orders fetched!");
resolve();
}, 1000);
});
}
function processUserOrders() {
return new Promise((resolve) => {
setTimeout(() => {
console.log("Orders processed!");
resolve();
}, 1000);
});
}
getUserData(1)
.then(() => getUserOrders())
.then(() => processUserOrders())
.then(() => {
console.log("All tasks completed!");
});
Using promises eliminates the need for deeply nested callbacks, creating more readable and maintainable code.
3. Practical Examples & Use Cases for Callbacks in Code
Callbacks are used across many JavaScript environments, from event handling to server-side Node.js applications. Let’s explore some practical examples.
Example 1: Callback in Event Handling
Event listeners in JavaScript often rely on callbacks. For instance, when a user clicks a button, the callback function is invoked to handle the event.
const button = document.getElementById("myButton");
button.addEventListener("click", function () {
console.log("Button clicked!");
});
In this example, the second argument to addEventListener
is a callback function. Whenever the button is clicked, the callback is executed.
Example 2: Callback in Timers
Callbacks are often used in conjunction with timers like setTimeout
or setInterval
. These functions allow you to execute code after a delay or repeatedly over a period of time.
function greet() {
console.log("Hello, World!");
}
setTimeout(greet, 2000); // Greet after 2 seconds
Here, the greet
function is passed as a callback to setTimeout
. After 2 seconds, the callback is executed, and "Hello, World!" is logged.
Example 3: Callback in API Requests
In Node.js, callbacks are commonly used for handling asynchronous tasks like reading files or making HTTP requests. For instance, reading a file might look like this:
const fs = require("fs");
fs.readFile("example.txt", "utf8", (err, data) => {
if (err) throw err;
console.log(data);
});
Here, the readFile
function takes a callback as its third argument. The callback is executed once the file has been read, allowing the data to be processed.
Example 4: Callback in Higher-Order Functions
Callbacks are also widely used in higher-order functions like map
, filter
, and reduce
. These functions accept a callback to determine how each element in an array should be processed.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(function (num) {
return num * 2;
});
console.log(doubled); // [2, 4, 6, 8, 10]
In this case, the callback function doubles each number in the array, creating a new array with the results.
Conclusion
Callbacks are an integral part of JavaScript, especially when dealing with asynchronous operations. While they allow for non-blocking code execution, they can lead to callback hell if not managed properly. By understanding how to use callbacks effectively, you can avoid common pitfalls and write cleaner, more maintainable code.
For modern JavaScript projects, consider using promises or the async/await syntax to handle complex asynchronous tasks more efficiently. However, callbacks still play a crucial role, particularly in event handling, timers, and higher-order functions.
By mastering callbacks, you unlock the potential to write dynamic, responsive JavaScript applications that perform well in real-world environments.
Subscribe to my newsletter
Read articles from Piyush kant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
