Seamless Flutter-Native Integration: The Power of Method Channels

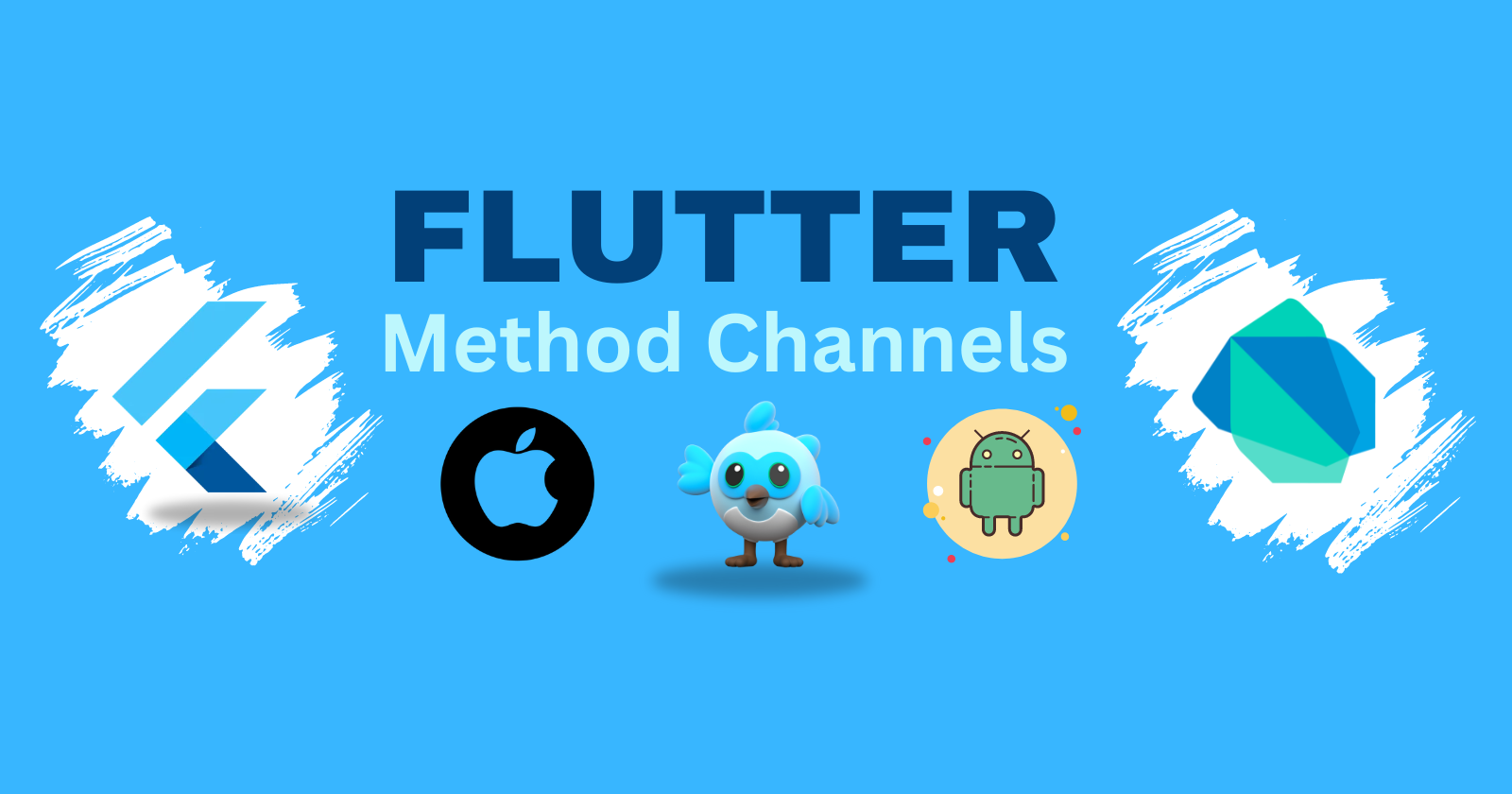
As Flutter developers, we enjoy the ease of building apps for both iOS and Android with a single codebase. But what if you need to tap into some native functionality? Whether it's accessing sensors, using a device-specific API, or integrating native libraries, you might hit a roadblock.
Enter Method Channels—Flutter's secret sauce for bridging the gap between Dart and native code. With method channels, you can call native platform APIs from Dart and bring that native power into your Flutter app. In this guide, we’ll explore how method channels work and walk you through a simple implementation step by step.
What is Method Channels?
Whenever someone asks about API, the first example that strikes our mind is the famous restaurant and waiter example. Let's understand method channels with the help of a similar example:
Think of it like this: you're in a restaurant (your Flutter app), and you're craving a dish that's not on the menu (a native feature). You call over the waiter (the Method Channel) and tell them what you want. The waiter heads over to the kitchen (the native platform) where the chef (Android or iOS) prepares your custom dish. Once it’s ready, the waiter brings it back to your table (Flutter) for you to enjoy!
In short, the Method Channel is your go-between when Flutter needs to ask Android or iOS for a specific feature or service that’s not readily available through standard Flutter plugins.
Method Channels: On a technical side
At its core, a method channel in Flutter is like a bridge between Dart (Flutter's language) and the native platform code (Android’s Java/Kotlin or iOS’s Swift/Objective-C). This "bridge" enables your Flutter app to call native platform APIs from Dart and, in return, receive the data or results from that native code.
The way communication works here is through a series of messages. These messages are sent through a lightweight platform channel, often in a JSON-like format, making it easy to send structured data between Dart and native platforms. When Flutter calls a method using a method channel, the native side listens for this call, processes it, and sends the result back to Flutter.
Why Do We Need Method Channels?
Although Flutter provides a rich set of plugins for accessing platform-specific features like camera, GPS, or sensors, not everything is covered out of the box. Here’s when method channels come into play:
Accessing Native APIs: Sometimes, you’ll need to tap into APIs that don’t have a Flutter plugin. Method channels allow you to write that native code yourself.
Using Existing Libraries: Maybe your app relies on an existing native library that you can’t easily recreate in Dart.
Platform-Specific Features: There are cases when the functionality differs across platforms (e.g., Android's specific system-level events that are not available on iOS).
How Do Method Channels Work?
Method channels work by setting up a communication bridge between Flutter and the native platform. This is achieved through a few simple steps:
Set up the method channel in Dart: You create a channel by specifying its name.
Implement native code on the platform side: This handles the actual request coming from Flutter.
Call methods across the bridge: Invoke platform-specific methods from Dart.
Let’s break this down with a step-by-step guide.
Step-by-Step Guide: Implementing Method Channels in Flutter
Step 1: Setting Up the Flutter Side
First, in your Flutter app, create a method channel in your Dart code. We’ll be using the MethodChannel
class for this.
import 'package:flutter/services.dart';
class NativeHelper {
static const platform = MethodChannel('com.example.platformChannel');
Future<String> getNativeData() async {
try {
final String result = await platform.invokeMethod('getNativeData');
return result;
} on PlatformException catch (e) {
return "Failed to get native data: '${e.message}'.";
}
}
}
Here, In this example:
We define a method channel named
'com.example.platformChannel'
.invokeMethod('getNativeData')
calls the native code to get data.If the method call fails (due to an exception or if the platform method is unavailable), it catches the error gracefully.
Step 2: Implementing Native Code on Android (Kotlin)
Now that the Flutter side is set up, let’s handle the native part for Android using Kotlin. You’ll modify the MainActivity.kt
file in your Android project.
package com.example.platform_channel
import android.os.Bundle
import io.flutter.embedding.android.FlutterActivity
import io.flutter.plugin.common.MethodChannel
class MainActivity : FlutterActivity() {
private val CHANNEL = "com.example.platformChannel"
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
MethodChannel(flutterEngine?.dartExecutor?.binaryMessenger, CHANNEL).setMethodCallHandler { call, result ->
if (call.method == "getNativeData") {
val nativeData = getNativeData()
if (nativeData != null) {
result.success(nativeData)
} else {
result.error("UNAVAILABLE", "Native data not available", null)
}
} else {
result.notImplemented()
}
}
}
private fun getNativeData(): String {
return "Hello from Android (Kotlin)!"
}
}
In this Kotlin implementation:
We define a
MethodChannel
with the same channel name ("com.example.platformChannel"
).The
getNativeData()
function returns a simple string when called from Flutter.If the native method is unavailable or unsupported, it handles errors using
result.error
.
Step 3: Implementing Native Code on iOS
For iOS, the process is similar. You’ll need to update the AppDelegate.swift
file.
import UIKit
import Flutter
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
let controller = window?.rootViewController as! FlutterViewController
let methodChannel = FlutterMethodChannel(name: "com.example.platformChannel",
binaryMessenger: controller.binaryMessenger)
methodChannel.setMethodCallHandler { (call: FlutterMethodCall, result: @escaping FlutterResult) in
if call.method == "getNativeData" {
result(self.getNativeData())
} else {
result(FlutterMethodNotImplemented)
}
}
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
private func getNativeData() -> String {
return "Hello from iOS!"
}
}
In the iOS implementation:
We set up a
FlutterMethodChannel
with the same name.The
getNativeData()
method returns a simple string.
Step 4: Testing the Method Channel
Now that both Dart and native code are set up, run your app. When you call the getNativeData()
method from your Dart code, it will fetch data from the platform-specific code—returning "Hello from Android!" on Android and "Hello from iOS!" on iOS.
Conclusion:
That wraps up the essentials of using method channels in Flutter to access native functionality. Got any more tips or questions? Drop them in the comments—I’d love to hear your thoughts!
Share your results: After following this guide, how did you implement method channels in your app? Let me know how it worked out for you!
💡 Hungry for more Flutter tips and insights? Subscribe now and stay ahead in your app development journey!
Subscribe to my newsletter
Read articles from Maharshi Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Maharshi Sinha
Maharshi Sinha
Open Source Enthusiast | Mobile App Developer