How to Use .env with ES6 Modules
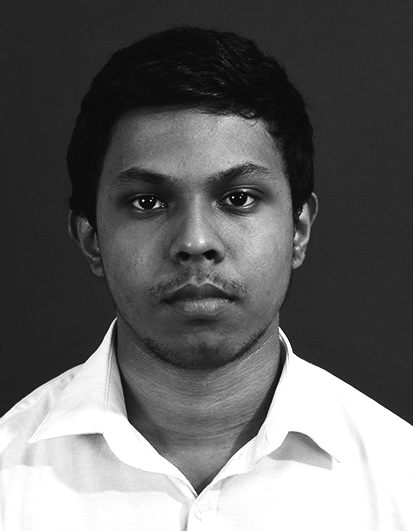
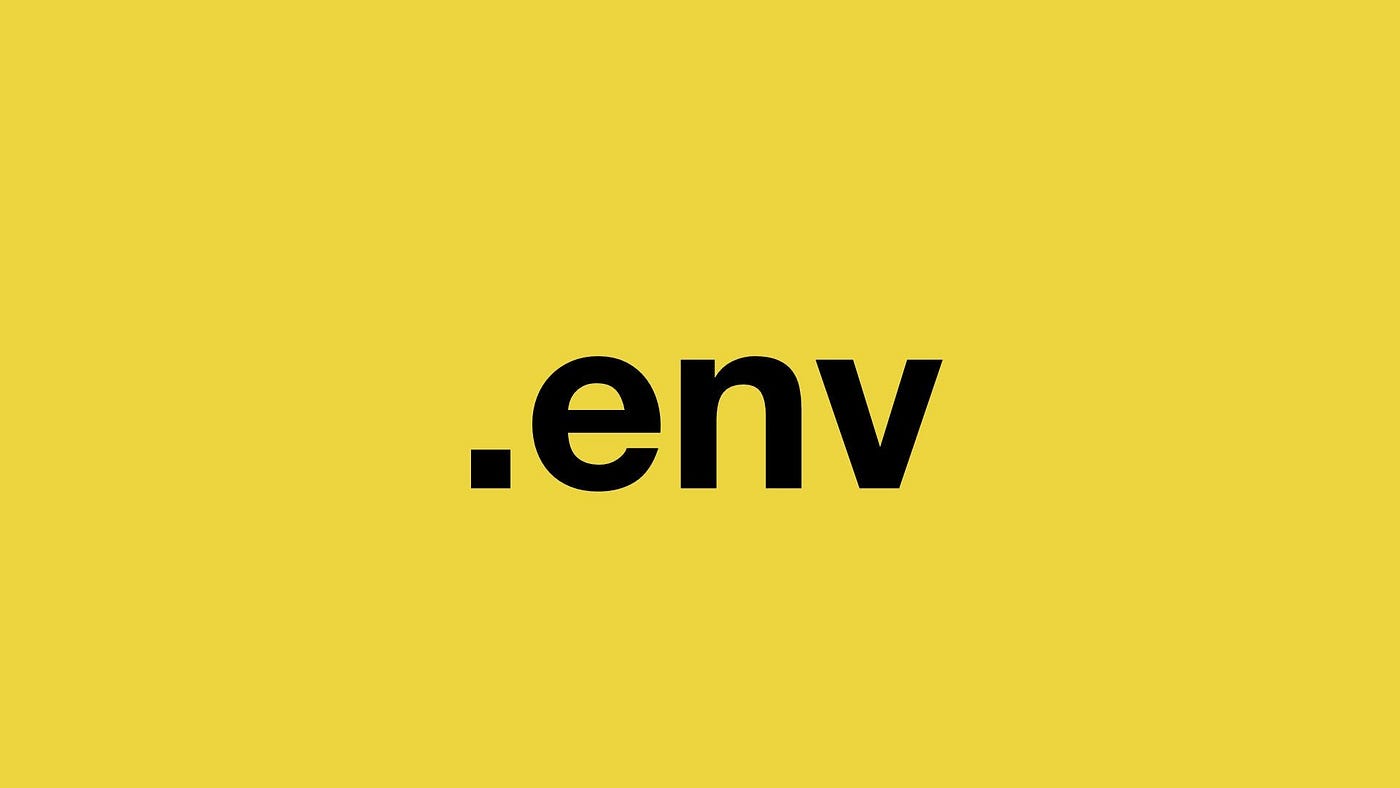
When building Node.js applications, it's common to store sensitive information like database credentials or API keys in environment variables. This is where the .env
file, managed by the dotenv
package, comes in handy. However, when using ES6 modules (import
/export
syntax), developers may encounter issues configuring dotenv
correctly. This guide will help you properly import and use dotenv
ES6 modules in a Node.js and Express app.
Step 1: Install dotenv
First, you need to install the dotenv
package, which allows you to load environment variables from a .env
file into process.env
.
npm install dotenv
Step 2: Create the .env
File
Create a .env
file in the root directory of your project (the same level as your server.js
or app.js
file). This file will store all your environment variables.
For example:
CONNECTION_URL=mongodb+srv://yourusername:yourpassword@cluster.mongodb.net/myDatabase?retryWrites=true&w=majority
PORT=5000
Make sure the .env
file is at the root of the project (and not inside subfolders like server
or client
if you have a monorepo structure).
Step 3: Use dotenv
with ES6 Modules
There are a couple of ways to configure dotenv
with ES6 modules. Let's go through the most commonly used approaches.
Option 1: Import dotenv/config.js
Directly
This approach allows you to load the environment variables as soon as the app starts, without explicitly calling dotenv.config()
. Here's how to implement it:
import 'dotenv/config.js'; // Import config without the need to call dotenv.config()
import express from 'express';
import mongoose from 'mongoose';
import cors from 'cors';
const app = express();
// Connect to the database using the environment variable
mongoose.connect(process.env.CONNECTION_URL, {
useCreateIndex: true,
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log("Connected to DB"))
.catch(err => console.error("Error connecting to DB:", err));
// Start the server
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
This method is straightforward and works well if you don't need to manipulate the environment variables dynamically.
Option 2: Explicitly Import and Call dotenv.config()
If you want more control or need to configure the path of your .env
file, you can use this approach.
import dotenv from 'dotenv'; // Import dotenv
dotenv.config(); // Load environment variables
import express from 'express';
import mongoose from 'mongoose';
import cors from 'cors';
const app = express();
// Use the environment variable for database connection
mongoose.connect(process.env.CONNECTION_URL, {
useCreateIndex: true,
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log("Connected to DB"))
.catch(err => console.error("Error connecting to DB:", err));
// Start the server
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Option 3: Handling Paths for .env
in Subdirectories
If your .env
file is located in a subdirectory, you may need to provide a custom path. Here's how to do it:
import dotenv from 'dotenv';
import path from 'path';
import { fileURLToPath } from 'url';
// Get the current file directory (required for ES6 modules)
const __filename = fileURLToPath(import.meta.url);
const __dirname = path.dirname(__filename);
// Load the .env file from a custom directory
dotenv.config({ path: path.join(__dirname, '../.env') });
import express from 'express';
import mongoose from 'mongoose';
import cors from 'cors';
const app = express();
// DB configuration
mongoose.connect(process.env.CONNECTION_URL, {
useCreateIndex: true,
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log("Connected to DB"))
.catch(err => console.error("Error connecting to DB:", err));
// Start the server
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
In this example, we're using path.join
to specify the relative location of the .env
file in case it resides in a different directory.
Step 4: Check .env
File Location
One common mistake that can cause process.env
variables to be undefined
is incorrect placement of the .env
file. Ensure that:
The
.env
file is placed at the root of the project (or the correct directory if you're using a custom path).The file is named exactly
.env
(no extra characters or extensions).
You can verify the loading of environment variables by logging them to the console:
console.log(process.env.CONNECTION_URL); // This should print the connection string from the .env file
When using dotenv
in a Node.js and Express application with ES6 modules, it’s essential to:
Ensure correct import of
dotenv
.Place the
.env
file in the proper directory.Use
dotenv.config()
or side-effect imports to load environment variables correctly.
With these steps, you should have no issues accessing your environment variables. Happy coding!
Subscribe to my newsletter
Read articles from Nirmal Sankalana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
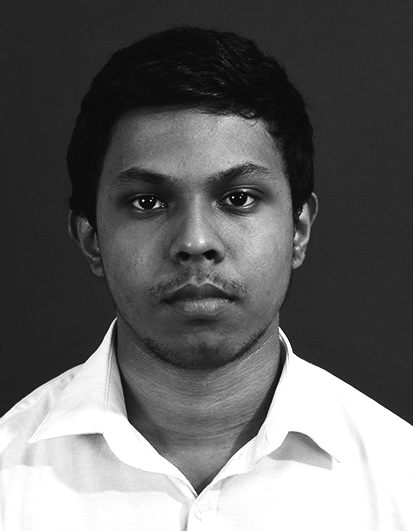