Best Practices for Securing Node.js Applications in Production
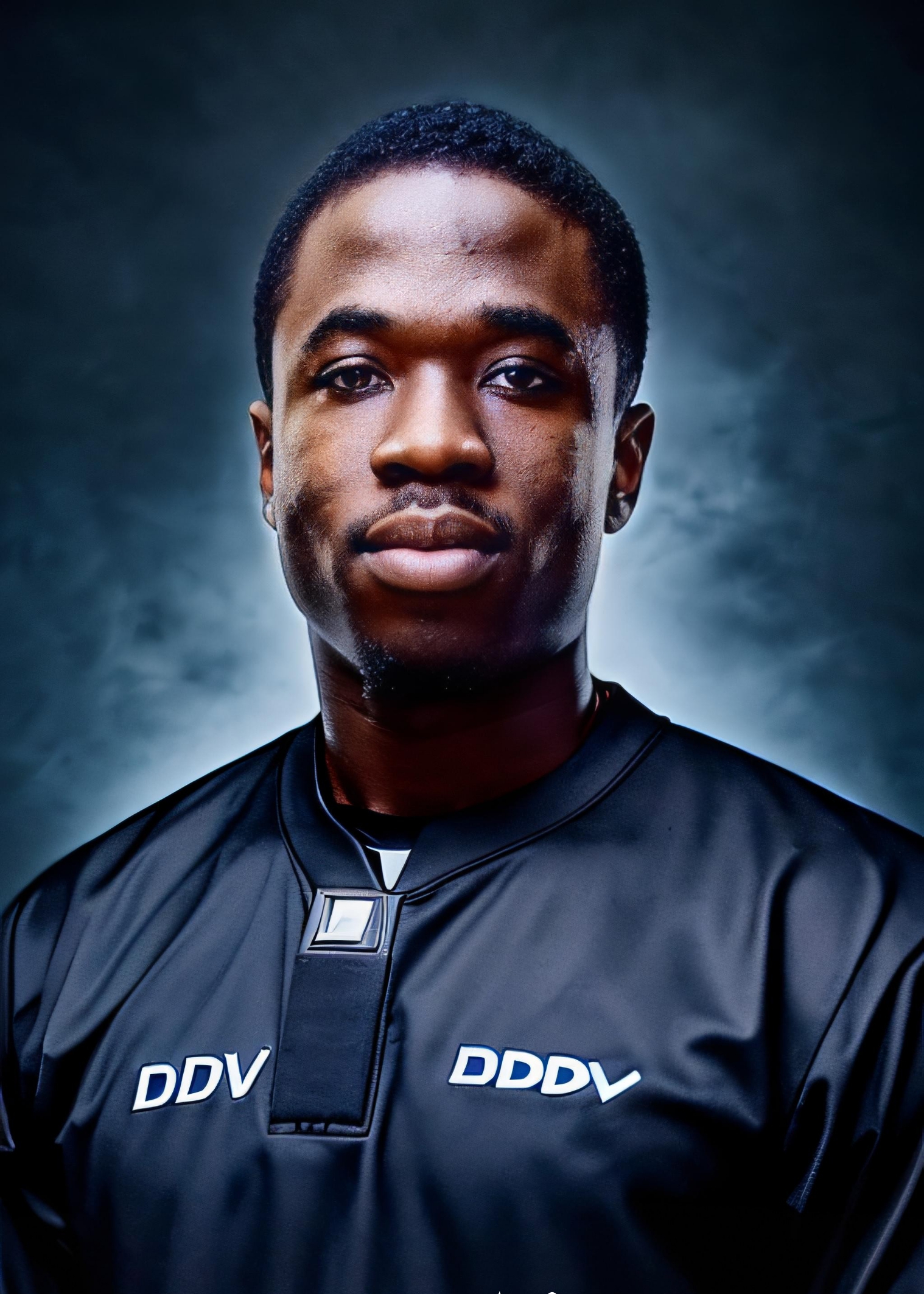
Table of contents
- 1. Keep Dependencies Updated
- 2. Use Proper Error Handling and Logging
- 3. Implement Strong Authentication and Authorization
- 4. Secure Sensitive Data
- 5. Mitigate Cross-Site Scripting (XSS)
- 6. Prevent Cross-Site Request Forgery (CSRF)
- 7. Secure Server Configuration
- 8. Rate Limiting and Throttling
- 9. Monitor and Respond to Threats
- Conclusion
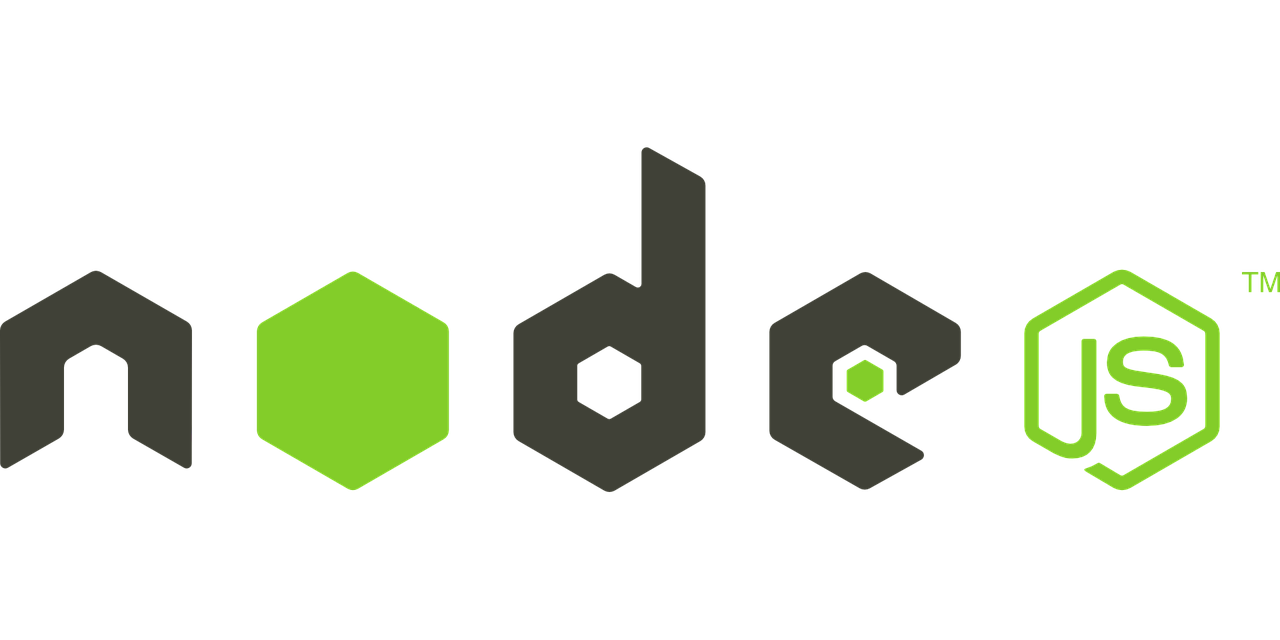
Node.js, a popular runtime for building server-side applications, brings high performance and scalability to web development. However, its flexibility can lead to security vulnerabilities if not properly managed. When deploying Node.js applications in production, adhering to security best practices is essential to prevent potential exploits, data breaches, and performance issues. This article outlines critical strategies to secure Node.js applications effectively.
1. Keep Dependencies Updated
Node.js applications typically rely on numerous third-party libraries and packages. While these can accelerate development, they also introduce security risks if not maintained properly. Here’s how you can manage dependencies safely:
Regularly Update Dependencies: Use tools like
npm outdated
to check for outdated dependencies andnpm audit
to automatically detect vulnerabilities in your packages.Lock Dependency Versions: Use a
package-lock.json
oryarn.lock
file to lock down exact versions of dependencies to prevent unexpected updates from introducing security risks.Remove Unused Dependencies: Regularly audit and clean up unused libraries and packages. Tools like
depcheck
can help identify which dependencies are no longer used in your codebase.Consider the Use of Vulnerability Scanning Tools: Integrate tools such as Snyk, npm audit, or OWASP Dependency-Check to automate vulnerability detection for known security issues in your dependencies.
2. Use Proper Error Handling and Logging
Poor error handling can inadvertently expose sensitive information, such as stack traces and internal server data. Implement the following practices to handle errors securely:
Disable Stack Traces in Production: Stack traces provide attackers with insight into the application’s structure, making it easier to exploit vulnerabilities. Avoid exposing stack traces by ensuring that detailed error information is disabled in production:
if (process.env.NODE_ENV !== 'production') { app.use(errorHandler()); // Use detailed error handler in dev } else { app.use(genericErrorHandler()); // Use generic error handler in production }
Centralized Error Handling: Use a centralized error-handling middleware to manage errors consistently across your application.
Log Wisely: Log errors and important events for auditing purposes, but never log sensitive data (e.g., user credentials, personal information). Utilize logging tools like Winston or Bunyan.
3. Implement Strong Authentication and Authorization
Implement robust access controls to safeguard against unauthorized access to your application’s resources:
Use Strong Password Policies: Enforce a strong password policy with complexity requirements (e.g., length, character types) and implement rate limiting to protect against brute-force attacks.
Enable Multi-Factor Authentication (MFA): Require users to provide a second form of verification (e.g., SMS code, authenticator app) during login.
Use Secure OAuth or JWT Tokens: Securely implement token-based authentication mechanisms, such as OAuth2 or JSON Web Tokens (JWT). Ensure tokens are signed and encrypted where applicable:
Use short-lived tokens and refresh tokens.
Store JWTs securely in
httpOnly
cookies to mitigate XSS attacks.
Implement Role-Based Access Control (RBAC): Implement fine-grained authorization using roles and permissions. Ensure that sensitive actions or resources are only accessible to authorized users:
if (!user.hasPermission('ADMIN')) { res.status(403).send('Forbidden'); }
4. Secure Sensitive Data
Protect sensitive user data from potential breaches through proper encryption and management:
Use HTTPS: Always encrypt data in transit using HTTPS. SSL/TLS certificates should be configured to ensure secure communication between clients and the server.
Encrypt Sensitive Data at Rest: Encrypt sensitive data (e.g., passwords, personal information) in databases. Use robust encryption standards like AES-256.
Hash Passwords Securely: Never store passwords in plaintext. Use a strong hashing algorithm like bcrypt or Argon2 with a sufficient work factor to make password cracking computationally expensive:
const bcrypt = require('bcrypt'); const hashedPassword = await bcrypt.hash(plainTextPassword, saltRounds);
5. Mitigate Cross-Site Scripting (XSS)
XSS attacks inject malicious scripts into web pages viewed by users. To prevent XSS, follow these steps:
Escape User Input: Sanitize and escape all user input, particularly data that is rendered on web pages. Use libraries like
validator
orDOMPurify
to sanitize HTML input.Set Content Security Policy (CSP): A CSP header restricts the sources from which content can be loaded, mitigating the impact of XSS attacks. Configure CSP headers in your response:
app.use(helmet.contentSecurityPolicy({ directives: { defaultSrc: ["'self'"], scriptSrc: ["'self'", 'trusted-cdn.com'], } }));
6. Prevent Cross-Site Request Forgery (CSRF)
CSRF attacks trick users into executing unwanted actions on a web application in which they are authenticated. Mitigate CSRF risks using the following measures:
Use CSRF Tokens: Implement anti-CSRF tokens to verify the legitimacy of requests. Libraries like
csurf
can be used to protect forms:const csrf = require('csurf'); app.use(csrf({ cookie: true }));
SameSite Cookies: Set the
SameSite
attribute on cookies to prevent them from being sent along with cross-site requests:res.cookie('token', token, { httpOnly: true, secure: true, sameSite: 'Strict' });
7. Secure Server Configuration
Proper server configuration plays a crucial role in minimizing security vulnerabilities:
Disable Unused Services: Disable any unnecessary services or ports to reduce the attack surface.
Limit Access to Critical Files: Ensure that sensitive configuration files (e.g.,
.env
,package-lock.json
,node_modules
) are not accessible publicly by configuring appropriate file permissions and web server settings.Avoid Exposing Server Information: Prevent the disclosure of information such as the Node.js version or application framework by configuring headers:
app.disable('x-powered-by');
8. Rate Limiting and Throttling
Rate limiting and throttling mechanisms help protect your application from denial-of-service (DoS) attacks and brute-force attempts:
Implement Rate Limiting: Use tools like
express-rate-limit
to restrict the number of requests a client can make within a time frame:const rateLimit = require("express-rate-limit"); app.use(rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100 // Limit each IP to 100 requests per window }));
Throttle Expensive Requests: Apply throttling to resource-intensive operations such as file uploads, database queries, and complex calculations.
9. Monitor and Respond to Threats
Continuous monitoring and incident response are key to maintaining a secure Node.js environment:
Use Monitoring Tools: Integrate monitoring tools like Prometheus or New Relic to detect unusual activity, such as excessive resource consumption or anomalous behavior in your application.
Establish Incident Response Plans: Prepare for security incidents by defining a clear response plan. Ensure that your team knows how to react promptly in the event of a data breach or attack.
Conclusion
Securing a Node.js application requires a comprehensive approach that spans from managing dependencies to hardening the server environment. By adhering to these best practices, you can significantly reduce the risk of attacks and ensure the safe and reliable operation of your application in production.
Security is an ongoing process, and regular audits, updates, and monitoring are essential to staying ahead of emerging threats. With a proactive and layered defense strategy, your Node.js application will remain resilient in the face of evolving security challenges.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
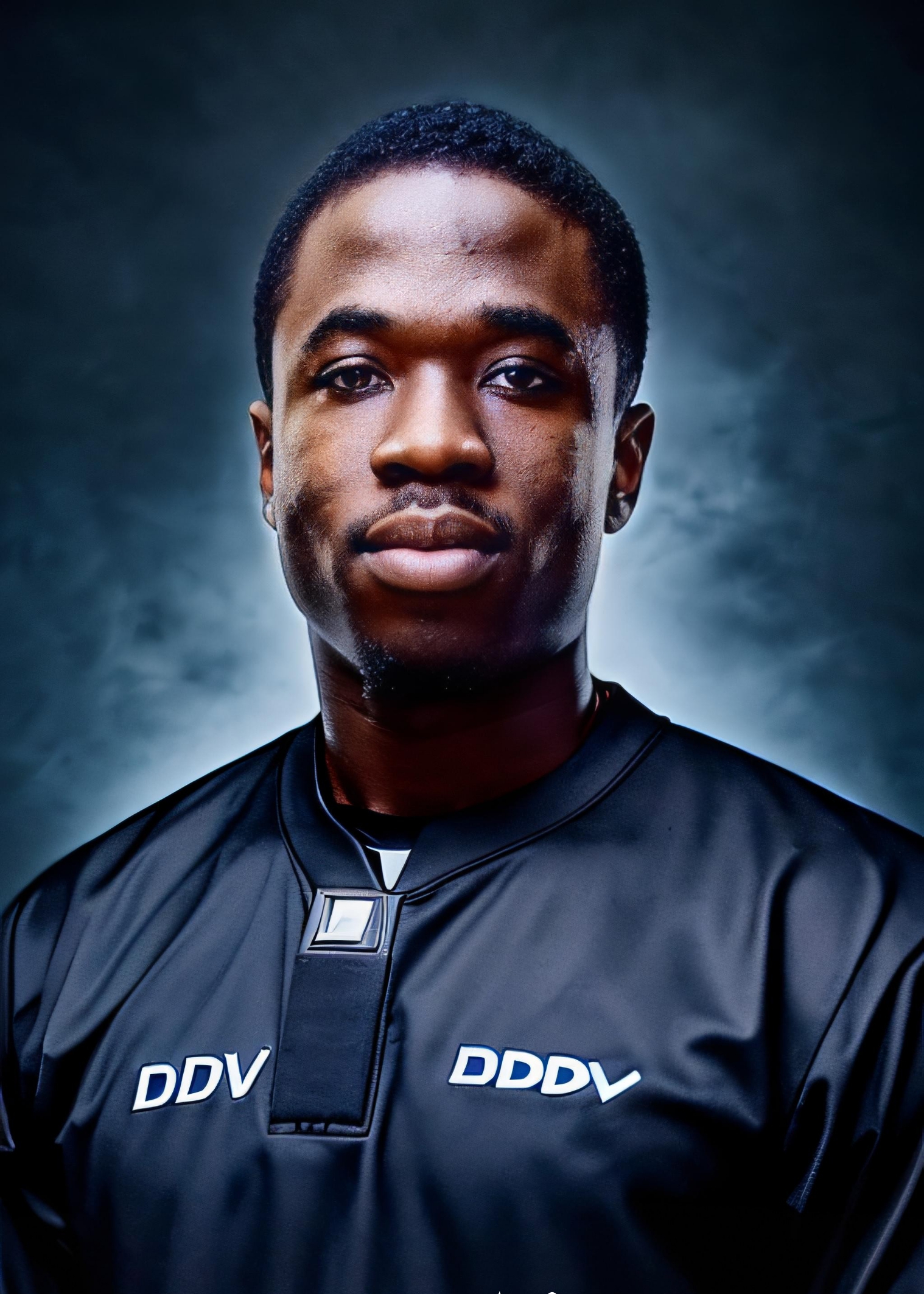
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.