Profiling Tools and Techniques for Node.js Applications
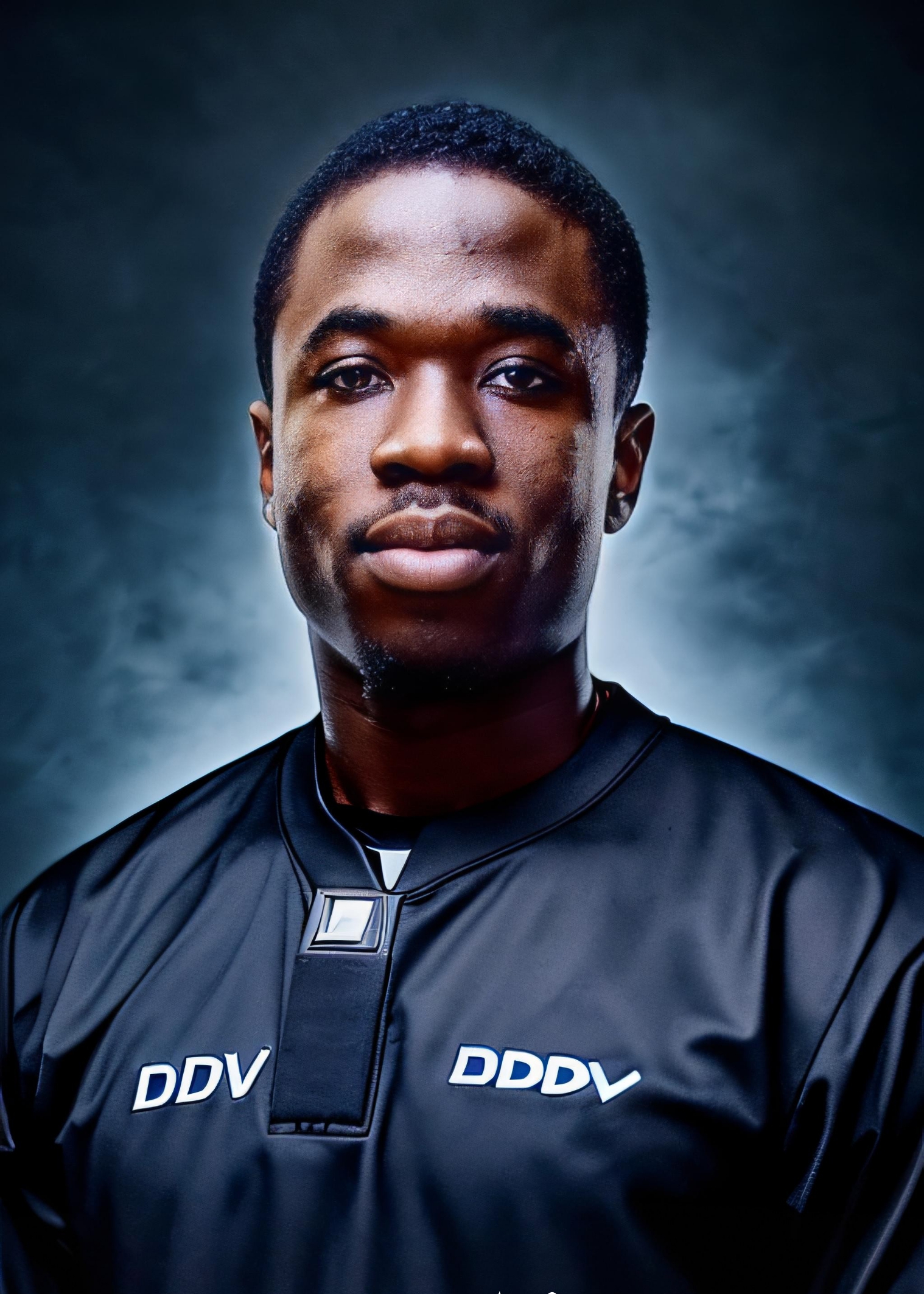
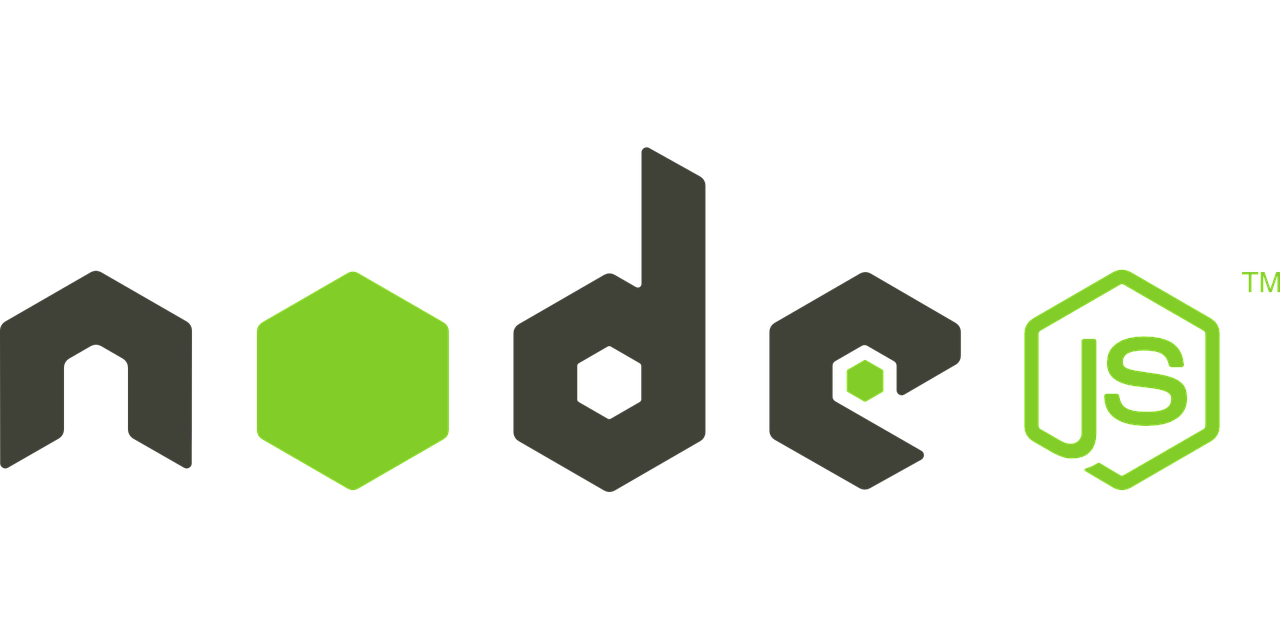
Profiling is an essential part of performance optimization in Node.js applications. It helps developers identify bottlenecks, memory leaks, inefficient code, and potential areas for improvement. With the growing complexity of modern applications, understanding and employing the right profiling tools and techniques can greatly enhance performance, scalability, and efficiency. This article explores the most effective tools and techniques for profiling Node.js applications, offering detailed guidance for developers aiming to optimize their applications.
Why Profiling is Important in Node.js
Node.js applications often run in environments where performance is critical—whether it's serving a large volume of web traffic, handling real-time interactions, or processing massive amounts of data. Profiling helps to:
Identify Bottlenecks: Detect slow parts of the code affecting response times.
Optimize Memory Usage: Pinpoint memory leaks or inefficient memory usage.
Improve Scalability: Ensure that the application can handle increased load efficiently.
Enhance CPU Utilization: Discover CPU-bound tasks that slow down event loops.
Ensure Stability: Avoid crashes due to excessive resource usage.
Key Areas for Profiling in Node.js
Before diving into tools, it's important to understand the aspects of Node.js applications that commonly require profiling:
CPU Usage: To measure and optimize how the CPU is being used during processing.
Memory Usage: To track heap memory consumption and garbage collection (GC).
Event Loop Delays: To monitor how efficiently the event loop processes asynchronous tasks.
I/O Performance: To profile disk and network operations for any slowdowns.
Startup Time: To optimize the application’s boot time, especially in serverless environments.
Profiling Tools for Node.js Applications
1. Node.js Built-in Profiler
Node.js comes with a built-in V8 profiler that allows developers to analyze CPU usage. It uses Chrome DevTools' JavaScript engine, making it accessible for quick profiling tasks.
How to use: You can start the profiler by running the following command:
node --prof app.js
This generates a log file that can be converted into a human-readable format using node --prof-process
.
Features:
Low overhead.
Provides CPU sampling data.
Generates performance profiles that can be viewed in Chrome DevTools or third-party tools.
Use case: Best suited for developers who need quick insights into CPU performance without relying on external tools.
2. Chrome DevTools (Inspector)
Chrome DevTools can be directly integrated with Node.js via the inspect
flag. It allows you to capture CPU profiles, memory snapshots, and even debug your application interactively.
How to use:
Run your application with the inspect flag:
node --inspect app.js
Open Chrome and navigate to
chrome://inspect
.Attach to the Node.js process and access profiling tools.
Features:
Visual representation of CPU and memory profiles.
Provides heap snapshots and memory leaks detection.
Event loop profiling.
Flame graphs to visualize call stack performance.
Use case: Chrome DevTools is ideal for developers who prefer a visual tool with real-time debugging capabilities.
3. Clinic.js
Clinic.js is a suite of tools that provides three distinct profiling tools: clinic doctor
, clinic flame
, and clinic bubbleprof
. Each of these focuses on different aspects of application performance.
clinic doctor: Analyzes the event loop, CPU usage, and memory for issues, providing suggestions for optimization.
clinic flame: Generates flame graphs to visualize CPU consumption across different parts of the application.
clinic bubbleprof: A unique tool for analyzing asynchronous operation bottlenecks and event loop behavior.
How to use: Install Clinic.js globally:
npm install -g clinic
Use one of the profiling tools:
clinic doctor -- node app.js
Features:
Comprehensive profiling, from CPU to asynchronous operation analysis.
Easy-to-read visual representations.
Suggests solutions for identified performance issues.
Use case: Best suited for diagnosing complex performance issues involving event loops, asynchronous operations, and CPU-intensive tasks.
4. node-tick
The node-tick
tool is part of Node.js' runtime and can generate detailed performance logs about CPU and memory usage. It produces a low-level, tick-based profiling report, allowing granular analysis.
How to use: Start the application with the tick profiler:
node --prof --log-timer-events app.js
Then, you can convert the generated logs into a report using the --prof-process
command.
Features:
Provides in-depth CPU profiling at the system level.
Tracks asynchronous operations and garbage collection timings.
Offers performance insights based on timers and tick events.
Use case: Suitable for low-level performance analysis where deeper insight into CPU cycles is necessary.
5. 0x
0x is a powerful flame graph generator for Node.js applications, useful for visualizing CPU usage. It captures and renders a flame graph that helps track down bottlenecks in the call stack.
How to use: Install 0x globally:
npm install -g 0x
Run the application with 0x:
0x app.js
Features:
Generates flame graphs with minimal overhead.
Provides real-time visualization of CPU-intensive functions.
Supports both synchronous and asynchronous code analysis.
Use case: Ideal for visualizing call stacks and identifying which parts of the code consume the most CPU time.
6. Heapdump
Heapdump is a tool for capturing heap snapshots to investigate memory leaks. A heap snapshot contains information about memory allocations in your Node.js application, which can be analyzed to find memory leaks or excessive allocations.
How to use: Install the heapdump module:
npm install heapdump
You can trigger a heap snapshot using:
const heapdump = require('heapdump');
heapdump.writeSnapshot('./heapdump.heapsnapshot');
Open the .heapsnapshot
file using Chrome DevTools for analysis.
Features:
Captures heap snapshots during runtime.
Helps track memory leaks and inefficient memory usage.
Integrates with Chrome DevTools for analysis.
Use case: Best suited for memory profiling and detecting memory leaks in Node.js applications.
7. Perf (Linux Only)
perf
is a performance analysis tool for Linux-based systems. It can be used to profile Node.js applications by providing detailed system-level profiling data, including CPU cycles, cache misses, and memory performance.
How to use:
Start the
perf
tool:perf record -g node app.js
Generate a report:
perf report
Features:
Provides system-level profiling insights.
Captures CPU cycles, cache usage, and other low-level performance metrics.
Works well for CPU-intensive applications.
Use case: Suitable for advanced users on Linux systems who require low-level system profiling for performance optimization.
Key Profiling Techniques
1. CPU Profiling
CPU profiling helps track how much CPU time each function in the code consumes. This is crucial when the application is CPU-bound, as it helps identify bottlenecks.
Use tools like the built-in Node.js profiler or Chrome DevTools.
Analyze CPU flame graphs generated by 0x or Clinic Flame to visualize bottlenecks.
2. Memory Profiling
Memory profiling focuses on identifying memory leaks and inefficient memory usage. The garbage collector plays a key role in Node.js, so it's essential to monitor heap memory.
Capture heap snapshots using Chrome DevTools or Heapdump.
Watch for growth patterns in memory usage over time, especially when dealing with large datasets or long-running applications.
3. Event Loop Profiling
Since Node.js is single-threaded, the event loop is the heart of the system. Monitoring event loop delays ensures smooth operation of asynchronous tasks.
Use Clinic Doctor or Chrome DevTools to analyze event loop behavior.
Check for blocking tasks that slow down event processing.
4. Garbage Collection Profiling
Profiling the garbage collector helps ensure that it is running efficiently and not causing long pauses in your application.
Tools like node-tick and Chrome DevTools provide insights into garbage collection activity.
Analyze heap snapshots to see which objects are kept in memory unnecessarily.
Best Practices for Profiling Node.js Applications
Profile in Production-like Environments: Always profile in an environment that closely resembles production to get accurate performance metrics.
Use Multiple Tools: No single tool provides a complete picture; use a combination of tools to gain insights into different performance aspects.
Minimize Overhead: Some profiling tools introduce overhead. Use lightweight tools like
0x
or the built-in profiler during critical performance diagnostics.Monitor Regularly: Profiling should be a regular part of the development process, not just when issues arise. Regular monitoring helps detect performance degradation early.
Conclusion
Profiling Node.js applications is critical to ensuring high performance, stability, and scalability. By using a combination of built-in and third-party tools like Chrome DevTools, Clinic.js, 0x, and Heapdump, developers can effectively diagnose CPU, memory, and event loop issues. Regular profiling helps ensure that your Node.js application is optimized and capable of handling production workloads efficiently.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
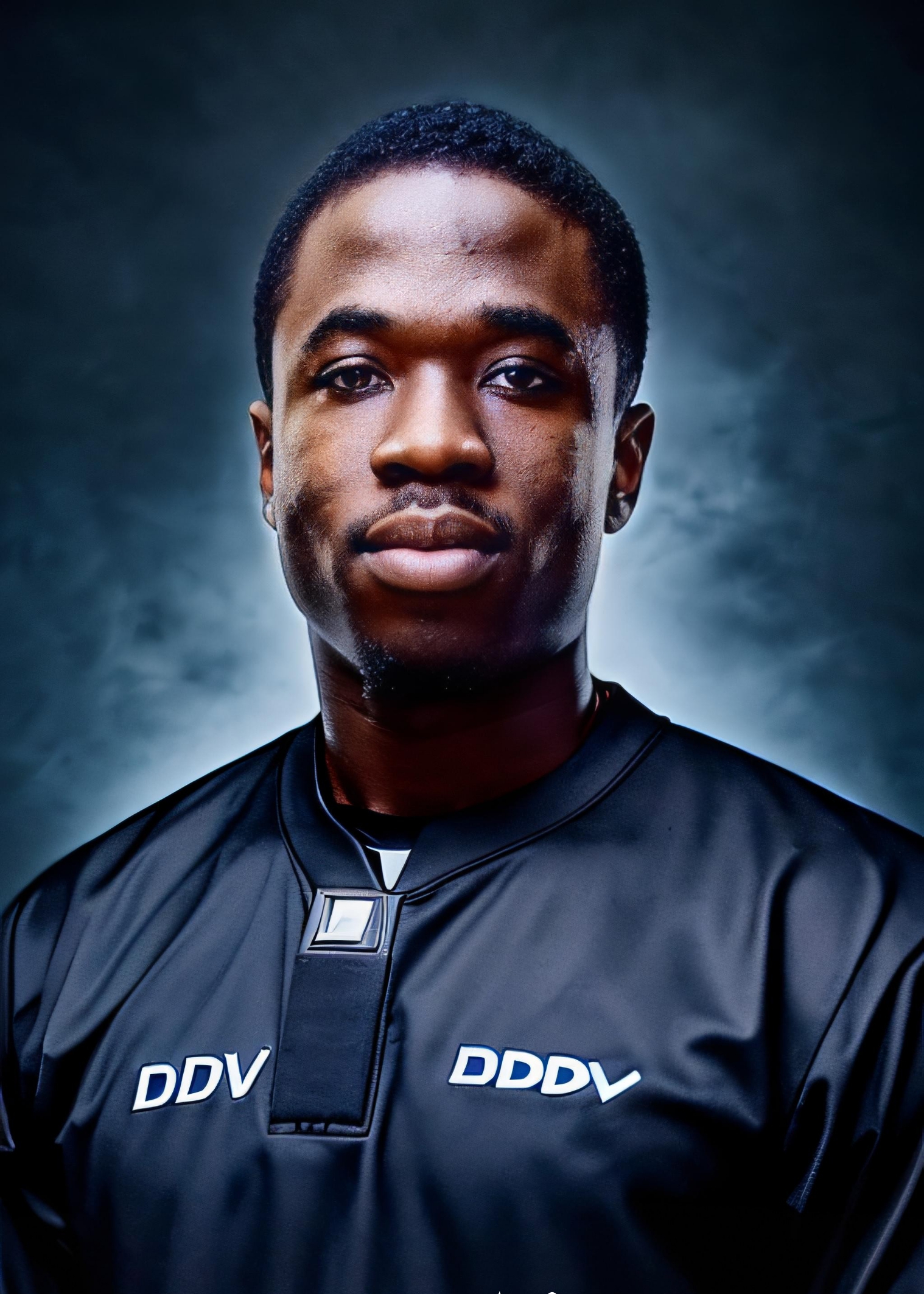
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.