Build Automation in Java: Maven, Gradle, and Ant
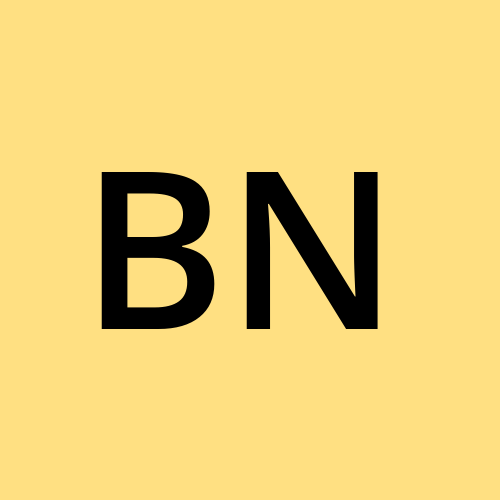
Table of contents
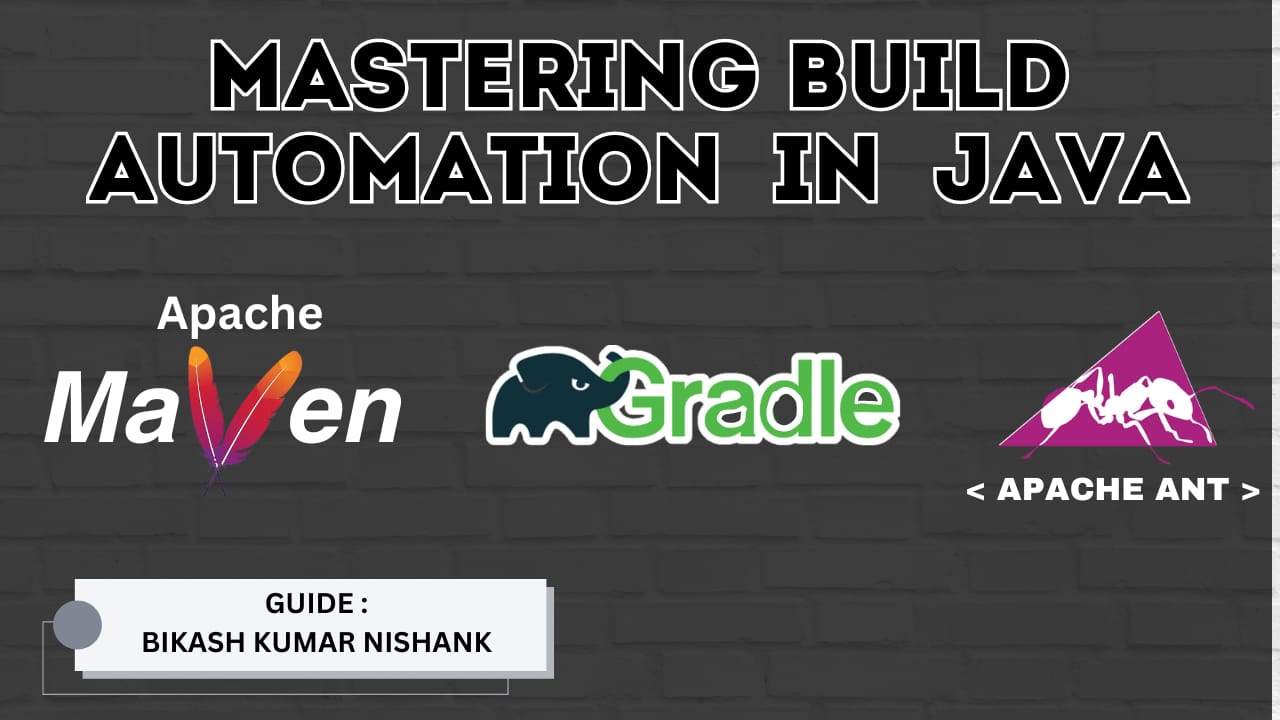
1. Introduction
What is Build Automation?
Build automation refers to the process of automating the various tasks involved in compiling, packaging, testing, and deploying software. In the context of Java development, it simplifies the workflow by allowing developers to automate repetitive tasks that would otherwise be performed manually, such as compiling source code into bytecode, running tests, and generating executables (JAR, WAR, EAR files). Build automation tools are essential for ensuring consistency, speeding up the development process, and reducing human error.
Importance of Build Automation in Java Development
In modern Java development, where projects can involve multiple modules, dependencies, and environments, manual builds can be error-prone and inefficient. Build automation tools streamline this by managing dependencies, handling different environments, and ensuring that builds are reproducible and consistent across teams. For Java developers working in Agile or DevOps environments, these tools become even more critical as they ensure rapid and reliable deployments.
Key reasons why build automation is crucial in Java development:
Consistency: Automated builds produce the same output every time, eliminating discrepancies caused by manual errors.
Speed: Developers don’t need to spend time on repetitive tasks like compiling and packaging code.
Error Reduction: Automation reduces the chance of human mistakes during the build process.
Integration with CI/CD Pipelines: It forms the backbone of continuous integration and delivery by allowing easy deployment of tested code.
Benefits of Using Build Automation Tools
Efficiency: Automates repetitive and time-consuming tasks like compiling, testing, and packaging, freeing up developers to focus on more important tasks.
Dependency Management: Tools like Maven and Gradle automatically resolve and manage project dependencies, ensuring compatibility and reducing errors.
Continuous Integration (CI): Build automation tools integrate with CI tools, ensuring that new code is continuously tested and built.
Reproducibility: Builds can be easily reproduced across different environments and teams, ensuring the same results in every environment.
Customisation: Build scripts can be tailored to meet the specific needs of a project, allowing for flexibility in handling complex workflows.
Common Build Automation Tasks
Build automation tools perform several tasks essential to software development, including:
Compilation: Converting source code into bytecode (.class files).
Packaging: Bundling compiled code into deployable formats like JAR or WAR files.
Dependency Management: Automatically downloading and managing external libraries required by the project.
Testing: Running automated unit, integration, and functional tests.
Deployment: Automating the process of deploying the packaged application to production or staging environments.
Documentation Generation: Creating Javadocs or other documentation from code comments.
Versioning: Managing versioning through tools like Git or SVN, integrated into the build process.
2. Overview of Build Automation Tools
What to Look for in a Build Automation Tool
When selecting a build automation tool, it’s important to consider the following factors:
Ease of Use: The tool should have a clear configuration system, whether through XML (Maven, Ant) or scripting (Gradle).
Flexibility: The tool should support custom tasks and workflows tailored to your project.
Dependency Management: The ability to manage external libraries and dependencies with ease is crucial.
Community Support: A large, active community ensures that the tool is well-documented, regularly updated, and has plugins available for common tasks.
Performance: Look for tools that can handle large builds efficiently, with options for parallel execution, caching, and incremental builds.
Integration with CI/CD: The tool should integrate smoothly with CI/CD platforms like Jenkins, GitLab, and others.
Scalability: For large projects or multi-module builds, the tool should be able to scale efficiently.
Support for Multiple Environments: It should be easy to configure for different environments (dev, staging, production).
Key Features of Build Automation Tools
Dependency Management: Automatically handling dependencies by downloading libraries from remote repositories (Maven’s central repository, Gradle’s build scripts).
Declarative vs. Imperative Approach: Some tools like Maven are declarative (you define what should be done), while others like Gradle are imperative (you define how it should be done).
Build Lifecycle: Tools like Maven provide a standard build lifecycle, where different phases (compile, test, package, install) are executed in sequence.
Extensibility: Build automation tools often support plugins or allow custom scripts to handle complex tasks.
Integration with Version Control: Most tools integrate with Git or SVN to pull specific branches or tags and create reproducible builds.
Multi-Project Build Support: Tools like Gradle are optimized for handling multiple projects or modules in a single build.
Overview of Popular Build Automation Tools
There are several popular build automation tools in the Java ecosystem:
Apache Maven: Maven is a declarative build tool that uses an XML-based configuration (pom.xml). It is known for its dependency management system and a standard lifecycle approach. It provides a clear structure to manage project configurations and dependencies.
Gradle: Gradle is a highly flexible and efficient build tool that uses a Groovy/Kotlin DSL for scripting. Gradle is more efficient than Maven for large projects due to features like build caching and parallel execution. Its ability to handle custom builds and multi-project setups makes it a favorite in the Java community.
Apache Ant: Ant is one of the oldest build tools and uses XML-based configuration (build.xml). Unlike Maven and Gradle, Ant does not provide a standard lifecycle, giving developers more control over the build process. However, it lacks dependency management out of the box and requires additional tools like Ivy for that purpose.
3.Apache Maven
Apache Maven is one of the most widely used build automation tools in Java projects. It provides a structured way to manage a project’s build, reporting, and documentation. Below is a detailed exploration of Maven and its key concepts.
Introduction to Maven
Apache Maven is a software project management and comprehension tool. Based on the Project Object Model (POM), Maven can manage a project's build, reporting, and documentation from a central piece of information.
Main Goal: Simplify the build process and manage dependencies automatically.
Scope: Maven is not just for Java projects; it can also be used in other programming languages, but it is primarily known for Java.
Key Features and Concepts
Project Object Model (POM)
The heart of a Maven project is the
pom.xml
file. It contains metadata about the project, dependencies, plugins, and build configurations.Example of a
pom.xml
:<project xmlns="http://maven.apache.org/POM/4.0.0"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>myapp</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> </dependencies> </project>
Convention Over Configuration
Maven follows the principle of Convention over Configuration, meaning it uses default behaviors for standard tasks, reducing the need for detailed configurations.
Default project structure:
my-app/ └── src/ └── main/ └── java/ # Application source code └── resources/ # Configuration files/resources └── test/ └── java/ # Test source code └── pom.xml # Maven configuration file
Dependency Management
Maven automatically downloads dependencies from a central repository like Maven Central. Dependencies are defined in the
pom.xml
and can include not only libraries but also plugins.Example dependency definition:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>2.5.4</version> </dependency>
Transitive Dependencies: Maven handles transitive dependencies by including libraries required by the libraries you specify.
Build Lifecycle
Maven has a fixed and well-defined build lifecycle with several phases that execute sequentially. The most common lifecycle phases include:
validate: Validate if the project is correct and all necessary information is available.
compile: Compile the source code.
test: Run unit tests.
package: Package the compiled code into a distributable format (JAR, WAR).
install: Install the package into the local repository.
deploy: Copy the final package to a remote repository.
Example to invoke the lifecycle:
mvn clean install
Repositories
Maven fetches dependencies from repositories. By default, Maven uses Maven Central, but custom repositories can be configured in the
pom.xml
.Example:
<repositories> <repository> <id>my-repo</id> <url>http://example.com/maven2</url> </repository> </repositories>
Maven Project Structure
The typical structure of a Maven project adheres to conventions, simplifying project organization:
my-app/
└── src/
└── main/
└── java/ # Application source code
└── resources/ # Configuration/resources (e.g., properties)
└── test/
└── java/ # Unit test code
└── pom.xml # Maven configuration file
Maven uses this standardized structure to determine where to look for source files, resources, and test files. You can modify this structure by configuring the pom.xml
file, though it is generally recommended to stick with the defaults.
Understanding Maven Lifecycle Phases
Maven automates the build process by following a well-defined lifecycle. There are three main lifecycles:
default: Handles project deployment, covering tasks from compiling to packaging.
clean: Handles project cleaning, such as removing old build files.
site: Generates project documentation.
Each lifecycle has phases. For example, the default lifecycle includes phases like:
validate: Ensures the project structure is correct.
compile: Compiles the source code.
test: Runs tests on the compiled code using a framework like JUnit.
package: Packages the code (usually into a JAR or WAR).
install: Installs the package into the local repository.
deploy: Copies the package to a remote repository.
Command example to run the entire lifecycle:
mvn install
Configuring Maven (pom.xml)
Maven is configured through the pom.xml
file. It includes information about the project, such as dependencies, plugins, and build configurations.
Basic Elements in pom.xml
:
groupId: The unique identifier for your project’s group (usually the company or organization name).
artifactId: The unique identifier for the project itself.
version: The project’s version (e.g., 1.0.0-SNAPSHOT).
dependencies: List of libraries the project depends on.
build: Configuration of how the project should be built (plugins, resources, etc.).
Example pom.xml
:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
Managing Dependencies with Maven
Maven’s most significant strength is its automatic dependency management. Dependencies are defined within the <dependencies>
section of the pom.xml
file.
Dependencies come with several scopes:
compile (default): Available in all phases (compile, test, runtime).
provided: Required to compile the project but provided by the runtime environment.
test: Used only during the testing phase.
For example:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
Using Maven Plugins
Plugins extend Maven’s functionality, helping to perform specific tasks during the build process. For example, you can use the maven-compiler-plugin
to specify which JDK version to use when compiling your project.
Example plugin configuration:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
Building and Testing Java Projects with Maven
To build your project, run:
mvn package
This will compile your code, run the tests, and package your project (typically into a JAR or WAR).
To run tests separately:
mvn test
Best Practices for Maven
Modularise Large Projects: Use Maven's multi-module project structure for large projects, allowing code to be split into reusable modules.
Dependency Management: Use dependency management features to maintain control over transitive dependencies and their versions.
Profiles: Create different build profiles (e.g., development, production) to customize your builds for different environments.
Version Control: Ensure
pom.xml
files are version-controlled to maintain consistency across teams and environments.Avoid “Fat” JARs: Rather than bundling all dependencies into a single JAR, use Maven to manage dependencies at runtime.
4.Gradle
Gradle is a modern build automation tool that provides flexibility and powerful features for building, testing, and deploying applications, especially for Java projects. It offers a faster and more expressive way to manage dependencies and build scripts compared to older tools like Apache Ant and Maven. Below is a detailed exploration of Gradle and its core concepts.
Introduction to Gradle
Gradle is a versatile build automation tool that supports multi-language development, but it is especially popular for Java projects. It combines the best aspects of other build tools (like Maven’s dependency management and Ant’s flexibility) with a more concise and expressive build script written in Groovy or Kotlin.
Main Goal: Simplify complex builds while offering flexibility and performance.
Scope: Gradle can handle multiple projects and languages in a single build, making it ideal for large and modular projects.
Key Features and Concepts
Gradle Build Script (
build.gradle
)Gradle uses a domain-specific language (DSL) based on Groovy (or Kotlin) to define its build scripts. These scripts are highly readable and concise.
Example
build.gradle
file:plugins { id 'java' } repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-web:2.5.4' testImplementation 'junit:junit:4.13.2' }
The script defines the plugins, repositories (from where dependencies are downloaded), and the dependencies required for the project.
Convention Over Configuration
Like Maven, Gradle follows the principle of Convention over Configuration. This means it uses sensible defaults for common tasks, but allows developers to configure and customize them as needed.
Default directory structure:
my-app/ └── src/ └── main/ └── java/ # Application source code └── resources/ # Configuration files/resources └── test/ └── java/ # Test source code └── build.gradle # Gradle configuration file
Dependency Management
Gradle handles dependencies using a powerful dependency management system, similar to Maven. Dependencies are declared in the
dependencies
block, with scopes likeimplementation
,api
,testImplementation
, etc.Example:
dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' testImplementation 'junit:junit:4.13.2' }
Transitive Dependencies: Gradle automatically resolves and includes transitive dependencies.
Tasks
A task is a basic unit of work in Gradle. Gradle tasks represent actions that perform the build process, like compiling code, running tests, or packaging the project.
Example of defining a custom task in
build.gradle
:task hello { doLast { println 'Hello, Gradle!' } }
To run the custom task:
gradle hello
Plugins
Gradle extends its functionality through plugins. Plugins provide pre-configured tasks and settings, making it easier to work with common technologies. For example, the Java plugin provides tasks like
compileJava
,test
, andjar
.To apply the Java plugin:
plugins { id 'java' }
Gradle Wrapper
The Gradle Wrapper (
gradlew
) is a script that comes with Gradle projects to ensure the correct version of Gradle is used, making the project portable and independent of the developer's environment.Running a Gradle build using the Wrapper:
./gradlew build
Gradle Build Script (build.gradle
)
The build script in Gradle is typically written in Groovy (or Kotlin) and controls the build configuration, dependency management, and task execution. It has the following main components:
Plugins Block: This section defines which plugins are applied to the project. The Java plugin is commonly used for Java projects.
plugins { id 'java' }
Repositories Block: Specifies where to download dependencies from. Common repositories include Maven Central, JCenter, and custom repositories.
repositories { mavenCentral() }
Dependencies Block: Defines the libraries and external tools the project relies on.
dependencies { implementation 'org.springframework.boot:spring-boot-starter-web:2.5.4' testImplementation 'junit:junit:4.13.2' }
Tasks Block: Defines tasks that are either provided by the plugins or custom tasks defined by the user.
task printMessage { doLast { println 'Building the project with Gradle!' } }
Understanding Gradle Tasks and Plugins
Tasks:
A task in Gradle is an action that performs part of the build process, such as compiling code, running tests, or creating JAR files.
Tasks can depend on other tasks, and Gradle ensures tasks are executed in the correct order.
Example task definition:
task hello { doLast { println 'Hello, Gradle!' } }
Run the task using the following command:
gradle hello
Plugins:
Gradle uses plugins to extend its functionality. For example, the Java plugin adds tasks for compiling Java code and packaging it into JAR files.
To apply the Java plugin, include the following in your
build.gradle
:plugins { id 'java' }
Configuring Multi-Project Builds with Gradle
Gradle supports multi-project builds, allowing you to split large projects into smaller, manageable modules. Each module can have its own build.gradle
file, and the root project can define common configurations for all subprojects.
Project Structure:
my-app/ └── build.gradle # Root build script └── settings.gradle # List of subprojects └── app/ # Subproject 1 └── build.gradle └── core/ # Subproject 2 └── build.gradle
Root
build.gradle
: Common configurations for all subprojects are placed in the rootbuild.gradle
:subprojects { apply plugin: 'java' repositories { mavenCentral() } dependencies { testImplementation 'junit:junit:4.13.2' } }
settings.gradle
: This file lists the subprojects:include 'app', 'core'
Dependency Management with Gradle
Gradle manages dependencies through the dependencies
block in build.gradle
. It handles both direct and transitive dependencies and can pull them from various repositories.
Dependency Types:
implementation: Compiles the dependency but hides it from other projects.
api: Allows other projects to access this dependency.
testImplementation: Adds dependencies used during testing.
Example:
dependencies {
implementation 'org.springframework:spring-core:5.3.9'
testImplementation 'junit:junit:4.13.2'
}
Building and Testing Java Projects with Gradle
To compile the source code, run:
gradle compileJava
To build the project, which includes compiling, testing, and packaging into a JAR, run:
gradle build
To run unit tests:
gradle test
Best Practices for Gradle
Use the Gradle Wrapper: Always use the Gradle Wrapper to ensure the project is built with the correct version of Gradle.
Modularize Large Projects: Use multi-project builds to split large projects into smaller, more manageable modules.
Caching: Take advantage of Gradle’s build cache to avoid rebuilding unchanged parts of the project, improving build times.
Version Control: Version control
build.gradle
andsettings.gradle
files to ensure consistency across builds.Dependency Locking: Use dependency locking to ensure stable and reproducible builds by locking specific versions of dependencies.
5.Apache Ant
Apache Ant (Another Neat Tool) is one of the oldest and most flexible build automation tools for Java projects. Although newer tools like Maven and Gradle have largely replaced Ant in many modern Java projects, Ant remains relevant due to its flexibility and simple task-based model.
Introduction to Ant
Apache Ant is a command-line tool that automates the process of compiling code, packaging binaries, and deploying applications. Unlike Maven or Gradle, Ant does not enforce a strict project structure or lifecycle. It allows developers to define custom build processes using XML configuration files (build.xml
).
Main Goal: Provide a flexible, platform-independent way to automate the build process.
Scope: Ant excels in projects that require full control over the build process without needing the predefined structure of Maven or the convention-based approach of Gradle.
Key Features and Concepts
Task-Based Model
Ant builds are based on "tasks." A task is an operation such as compiling source code, copying files, or creating JAR files. Tasks are defined in an XML file (
build.xml
), and developers have full control over the sequence in which tasks are executed.Example of tasks:
<project name="HelloWorld" basedir="." default="compile"> <target name="compile"> <javac srcdir="src" destdir="build/classes" /> </target> <target name="jar" depends="compile"> <jar destfile="build/helloworld.jar" basedir="build/classes" /> </target> </project>
No Fixed Project Structure
Unlike Maven and Gradle, Ant does not impose any particular project directory structure. You can organize your project files and directories in any way that suits your needs.
However, it is common practice to use directories like
src/
for source code andbuild/
for compiled binaries.
XML-Based Configuration (
build.xml
)- Ant uses an XML file (
build.xml
) to define the build process. This file contains information about targets, tasks, dependencies, and properties. Thebuild.xml
file is the core of any Ant project.
- Ant uses an XML file (
Targets
Targets in Ant define a group of tasks. A target can depend on other targets, ensuring that tasks are executed in the correct order.
Example:
<target name="compile"> <javac srcdir="src" destdir="build/classes" /> </target>
Properties
Ant allows you to define properties in the
build.xml
file to store configuration values, such as file paths or version numbers.Example:
<property name="src.dir" value="src"/> <property name="build.dir" value="build"/> <javac srcdir="${src.dir}" destdir="${build.dir}/classes" />
Ant Build Script (build.xml
)
The build.xml
file is the core configuration file in Ant. It defines the entire build process, including tasks, targets, and dependencies.
Basic Structure:
The
build.xml
file begins with a<project>
element, which defines the project’s name, base directory, and the default target.Inside the
<project>
element, you can define multiple<target>
elements, each containing a sequence of tasks.Example:
<project name="MyApp" basedir="." default="compile"> <target name="compile"> <javac srcdir="src" destdir="build/classes" /> </target> </project>
Defining Targets and Tasks:
A target contains one or more tasks, such as
javac
(for compiling Java code),jar
(for creating JAR files), andcopy
(for copying files).Tasks can be defined inside targets and executed sequentially. Targets can also depend on other targets.
Example:
<target name="jar" depends="compile"> <jar destfile="build/myapp.jar" basedir="build/classes" /> </target>
Running Ant Build:
To run the Ant build, navigate to the project directory containing
build.xml
and run the following command:ant
You can specify a specific target to execute, like this:
ant jar
Managing Dependencies with Ant
Unlike Maven and Gradle, Ant does not have a built-in dependency management system. However, developers can use the Ivy plugin to add dependency management functionality to Ant.
Adding Ivy for Dependency Management:
Ivy is a dependency management tool that integrates with Ant to allow automatic resolution and downloading of dependencies from repositories like Maven Central.
Example integration:
<ivy:resolve file="ivy.xml" /> <ivy:cachepath pathid="classpath"/>
Manual Dependency Management:
In many Ant projects, dependencies are handled manually by downloading JAR files and placing them in a
lib/
directory. Theclasspath
attribute of thejavac
task is used to include these dependencies during compilation.Example:
<javac srcdir="src" destdir="build/classes" classpath="lib/*" />
Building and Testing Java Projects with Ant
Compiling Source Code:
The
javac
task is used to compile Java source code.Example:
<target name="compile"> <mkdir dir="build/classes"/> <javac srcdir="src" destdir="build/classes"/> </target>
Creating JAR Files:
The
jar
task packages the compiled classes into a JAR file.Example:
<target name="jar" depends="compile"> <jar destfile="build/myapp.jar" basedir="build/classes" /> </target>
Running Tests:
Ant does not have built-in support for testing frameworks like JUnit, but you can integrate JUnit using the
junit
task.Example:
<target name="test" depends="compile"> <junit> <batchtest fork="true" todir="build/test-results"> <fileset dir="src/test/java"> <include name="**/*Test.java"/> </fileset> </batchtest> </junit> </target>
Best Practices for Ant
Use Properties for Configuration:
Always use properties to store file paths, version numbers, and other configurable values. This makes your build script more maintainable.
Example:
<property name="src.dir" value="src"/> <property name="build.dir" value="build"/>
Use Targets and Dependencies:
Break down the build process into multiple targets (e.g.,
compile
,jar
,clean
) and define dependencies between them. This ensures that targets are executed in the correct order.Example:
<target name="clean"> <delete dir="build"/> </target> <target name="compile" depends="clean"> <mkdir dir="build/classes"/> <javac srcdir="src" destdir="build/classes"/> </target>
Keep
build.xml
Modular:- As projects grow, Ant build scripts can become complex. Use modular targets and split large tasks into smaller, reusable targets to make the script easier to maintain.
Integrate Dependency Management:
- For large projects, use Ivy to manage dependencies automatically. This will simplify the process of managing external libraries.
Document Build Script:
Always document your
build.xml
file with comments, especially in larger projects where multiple developers may be working on the build script.Example:
<!-- Compile source code --> <target name="compile"> <javac srcdir="src" destdir="build/classes"/> </target>
6. Comparing Build Automation Tools: Maven vs. Gradle vs. Ant
Choosing the right build automation tool is crucial for managing and automating project builds in Java. Maven, Gradle, and Ant are three of the most widely used tools, each with its own strengths and weaknesses. In this section, we'll compare these tools based on various factors like features, performance, use cases, and community support.
Maven vs. Gradle vs. Ant: Strengths and Weaknesses
Maven:
Strengths:
Convention over Configuration: Maven follows a standard directory structure and a predefined build lifecycle, reducing the need for custom configuration.
Dependency Management: Maven’s built-in dependency management is one of its strongest features, allowing automatic downloading and version management of libraries from repositories like Maven Central.
Widely Adopted: It’s one of the most widely used tools in the Java ecosystem, making it easy to find tutorials, plugins, and community support.
Strong Ecosystem: Rich plugin support for continuous integration, testing, reporting, and more.
Weaknesses:
Verbose Configuration: The
pom.xml
file can become bloated and difficult to maintain in large projects.Slower Builds: Maven can be slower compared to Gradle, particularly in complex projects.
Limited Flexibility: Since Maven follows a strict lifecycle and structure, it can be challenging to implement custom builds or workflows without workarounds.
Gradle:
Strengths:
Flexible Build Configuration: Gradle allows developers to configure the build process using a Groovy or Kotlin-based DSL (Domain-Specific Language). It’s highly customisable, making it a great choice for complex builds.
Incremental Builds: Gradle excels in incremental builds, which can be much faster than Maven’s rebuild-everything approach. Gradle only recompiles the parts of the code that have changed.
Better Performance: Gradle's parallel and incremental build features provide better performance, especially for large projects.
Dependency Management: Like Maven, Gradle also offers excellent dependency management with repositories like Maven Central and JCenter.
Weaknesses:
Learning Curve: Gradle’s flexibility can make it harder for new users to learn, particularly when transitioning from Maven or Ant.
Less Standardisation: Because Gradle allows more customization, different projects may use different configurations, making it harder to standardise.
Ant:
Strengths:
Full Control: Ant is highly flexible and does not enforce any specific project structure or build lifecycle. This makes it ideal for projects with unique build processes.
Simple Task-Based Model: Ant’s task-based approach gives developers complete control over how the build process should be defined and executed.
Customizable: Ant is easy to customize and extend with new tasks, making it a versatile tool for non-standard projects.
Legacy Projects: Ant is still widely used in legacy projects and situations where full control over the build process is needed.
Weaknesses:
No Built-in Dependency Management: Unlike Maven and Gradle, Ant doesn’t have built-in dependency management. Users must manage external libraries manually or use external tools like Ivy.
XML Verbosity: Ant build scripts (
build.xml
) can become verbose and difficult to maintain as projects grow.No Standard Lifecycle: Ant doesn’t offer a predefined lifecycle like Maven, which requires developers to define every step of the build process manually.
Performance Considerations
Maven:
Maven tends to be slower than Gradle because it always runs the full lifecycle of the project, even if only minor changes are made.
However, for smaller projects or projects with simple configurations, Maven’s performance can be quite acceptable.
Gradle:
Performance Winner: Gradle offers incremental builds, parallel execution, and task-level caching, which significantly improve performance in large and complex projects.
Gradle is generally faster than Maven, particularly in scenarios where only a subset of the project needs to be recompiled.
Daemon Process: Gradle can run as a background process (daemon) to further reduce build times by keeping the build environment warmed up.
Ant:
Ant’s performance largely depends on how the build script is written since it doesn't impose a predefined structure or lifecycle.
It can be very fast in smaller projects but can become inefficient in larger projects because it lacks incremental build capabilities.
Use Cases and Scenarios
When to Use Maven:
Standardized Builds: Maven is perfect for projects that adhere to a conventional project structure and lifecycle.
Dependency-Heavy Projects: If your project has a large number of dependencies and requires complex dependency management, Maven simplifies this process.
Corporate Environments: Many large enterprises use Maven due to its standardisation and ease of integration with other tools like Jenkins.
When to Use Gradle:
Large and Complex Projects: Gradle is ideal for large, complex projects that require high levels of customization, performance optimization, or incremental builds.
Android Development: Gradle is the default build tool for Android development because of its flexibility and performance features.
Multi-Project Builds: Gradle is a great choice for handling multi-project builds with complex dependencies between modules.
When to Use Ant:
Legacy Projects: Ant is still relevant for maintaining and extending legacy systems that were originally built with Ant.
Unique Build Processes: If your project requires full control over the build process or doesn’t fit into Maven’s standard structure, Ant’s flexibility is a strong advantage.
Small or Simple Projects: For smaller projects where dependency management and standardisation are not a concern, Ant can provide a lightweight and simple solution.
Community and Ecosystem Support
Maven:
Strong Community: Maven has a large and active user base. It is widely used in enterprise environments and has excellent documentation, tutorials, and community support.
Rich Plugin Ecosystem: Maven’s plugin ecosystem is extensive, with plugins available for testing, reporting, code coverage, continuous integration, and deployment.
Enterprise Adoption: Many corporations and organisations standardise on Maven due to its maturity and stability.
Gradle:
Growing Community: Gradle has a growing and vibrant community, especially in mobile and modern Java projects.
Active Development: Gradle is actively developed, and new features are added regularly to improve performance and flexibility.
Strong Android Support: Gradle is the default build tool for Android, which means it has strong support in the mobile development community.
Plugins: Gradle’s plugin system is powerful, but not as mature or extensive as Maven’s, although it is catching up rapidly.
Ant:
Smaller Community: While Ant’s community is smaller than Maven or Gradle, it remains relevant in certain niche use cases like legacy systems.
Legacy Support: Ant is still supported and maintained by the Apache Foundation, though its active development has slowed compared to Maven and Gradle.
Limited Plugin Support: Ant’s plugin ecosystem is much smaller, and it requires additional tools like Ivy to manage dependencies.
Summary of Comparison:
Feature/Aspect | Maven | Gradle | Ant |
Project Structure | Standardized (Convention over Config.) | Flexible (DSL-based) | No enforced structure |
Build Speed | Slower (full lifecycle on every build) | Faster (incremental builds) | Fast, but no incremental builds |
Dependency Management | Built-in, strong dependency management | Built-in, flexible dependency mgmt. | Requires Ivy or manual management |
Flexibility | Limited by predefined lifecycle | Highly flexible | Fully flexible, but more manual work |
Ease of Use | Easier for standard Java projects | Steeper learning curve | Requires more configuration |
Performance | Acceptable but slower on larger builds | Excellent with incremental builds | Fast, but depends on manual setup |
Community Support | Large and mature | Growing rapidly, strong Android support | Smaller, focused on legacy projects |
Use Case | Standard Java projects, enterprise | Complex, large, or Android projects | Legacy projects, custom build processes |
7. Integrating Build Automation with CI/CD
Build automation tools like Maven, Gradle, and Ant play a vital role in enabling Continuous Integration (CI) and Continuous Deployment (CD) pipelines. CI/CD pipelines automate the process of building, testing, and deploying software, providing faster releases and reducing manual errors.
Overview of Continuous Integration (CI)
Continuous Integration (CI) is a software development practice where developers regularly integrate their code changes into a shared repository. Each integration triggers an automated build and test process, ensuring that the code remains in a deployable state.
CI tools like Jenkins, Travis CI, and CircleCI monitor the repository for changes and automatically trigger builds and tests when new code is committed.
Benefits:
Catch bugs early by running automated tests on every integration.
Ensure code is always in a buildable state.
Faster feedback loops, enabling quicker fixes.
Integrating Maven with CI Tools (e.g., Jenkins)
Setting Up Jenkins:
Install Jenkins on a server or use Jenkins-as-a-Service.
Set up a Jenkins Job to monitor the source code repository (Git, SVN, etc.).
Configuring Maven in Jenkins:
Install the Maven plugin in Jenkins to integrate Maven builds.
Specify the Maven installation in Jenkins' global tool configuration.
In the Jenkins job, choose "Invoke top-level Maven targets" as a build step and configure the desired Maven goals (e.g.,
clean install
).
CI Pipeline with Maven:
When code is committed to the repository, Jenkins pulls the latest changes and runs the Maven build.
Jenkins triggers the Maven lifecycle phases (e.g.,
compile
,test
,package
).Automated unit tests are executed as part of the
test
phase.
Best Practices:
Use
pom.xml
to manage build dependencies and configurations.Enable Jenkins to archive build artifacts and generate reports (e.g., JUnit reports).
Integrating Gradle with CI Tools (e.g., Jenkins)
Setting Up Jenkins for Gradle:
- Similar to Maven, install Jenkins and configure a job to monitor the repository.
Configuring Gradle in Jenkins:
Install the Gradle plugin in Jenkins.
Specify the Gradle installation in Jenkins' global configuration.
In the Jenkins job, use "Invoke Gradle Script" as a build step and specify Gradle tasks (e.g.,
clean build
).
CI Pipeline with Gradle:
Jenkins pulls code changes and triggers the Gradle build.
Gradle executes tasks like
compile
,test
, andbuild
, along with any custom-defined tasks.Gradle’s incremental build feature ensures only the changed parts of the code are rebuilt.
Best Practices:
Use build caching to optimise build performance in CI environments.
Configure multi-project builds in Gradle for modularised applications.
Integrating Ant with CI Tools (e.g., Jenkins)
Setting Up Jenkins for Ant:
- Install Jenkins and create a job to monitor the repository.
Configuring Ant in Jenkins:
Install the Ant plugin in Jenkins.
In the Jenkins job, use "Invoke Ant" as a build step and specify the targets from the
build.xml
file (e.g.,compile
,test
).
CI Pipeline with Ant:
Jenkins triggers Ant to execute predefined targets in the
build.xml
.Ant builds the project by compiling the code, running tests, and packaging the artifacts.
Best Practices:
Ensure modular and well-organized
build.xml
files to avoid complexity.Use external libraries like Ivy for better dependency management within CI pipelines.
Automating Builds and Deployments
Automating Builds:
CI tools like Jenkins automatically trigger builds when changes are pushed to the repository.
The CI tool pulls the latest code, runs the build automation tool (Maven, Gradle, or Ant), and tests the code.
Automating Deployments (CD):
After a successful build and testing process, CI/CD pipelines can automate the deployment to staging or production environments.
Tools like Jenkins integrate with cloud platforms (AWS, Azure) or container platforms (Docker, Kubernetes) to deploy the application.
Example Workflow:
- Developer pushes code → Jenkins triggers build → Maven/Gradle/Ant compiles and tests → Build artifacts are generated → Artifacts deployed to staging/production.
Best Practices for CI/CD Integration
Modular Pipelines: Break your CI/CD pipeline into stages such as build, test, and deploy.
Test Automation: Integrate unit, integration, and functional tests into the pipeline.
Environment Parity: Ensure that your build and deployment environments match production closely.
Continuous Feedback: Set up notifications (e.g., Slack, email) for build failures or issues.
Artifact Management: Use artifact repositories like Nexus or Artifactory to store build outputs.
8. Advanced Build Automation Topics
Custom Tasks and Scripts
Maven:
Custom tasks can be added via Maven plugins. For example, you can write custom goals or bind existing plugins to lifecycle phases.
Example: Use the
exec-maven-plugin
to run custom shell commands during the build process.
Gradle:
Gradle’s flexibility allows you to define custom tasks in the
build.gradle
file. Custom tasks can be written in Groovy or Kotlin.Example:
task hello { doLast { println 'Hello, Gradle!' } }
You can also define dependencies between tasks and create reusable task configurations.
Ant:
In Ant, custom tasks are defined in the
build.xml
file using the<target>
and<task>
elements.Example:
<target name="hello"> <echo message="Hello, Ant!" /> </target>
Build Caching and Optimisation Techniques
Maven:
- Maven offers limited caching features compared to Gradle. Using
mvn clean
always removes previous builds, so caching is not part of its default behavior.
- Maven offers limited caching features compared to Gradle. Using
Gradle:
Gradle provides build caching and incremental builds by default, which only rebuilds tasks that have changed since the last build.
Example: Gradle's build cache can store outputs from previous builds to reuse in subsequent builds.
Ant:
- Ant does not have built-in caching. However, you can use external tools or design the build script to avoid redundant tasks.
Handling Multi-Module Projects
Maven:
Maven supports multi-module projects where a parent
pom.xml
defines shared configurations for child modules.Example:
<modules> <module>module1</module> <module>module2</module> </modules>
Modules are built in a defined order, and dependencies between them can be managed via the parent POM.
Gradle:
Gradle handles multi-project builds by defining subprojects in the
settings.gradle
file.Example:
include 'projectA', 'projectB'
Ant:
- Ant doesn't natively support multi-module projects but can be configured to handle multiple builds by including or importing other build files.
Advanced Dependency Management
Maven:
Maven uses a centralised dependency management system via the
pom.xml
file.Example:
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.8</version> </dependency>
It handles transitive dependencies, automatically resolving and downloading required libraries.
Gradle:
Gradle also uses a centralised approach to dependency management in the
build.gradle
file.Example:
dependencies { implementation 'org.springframework:spring-core:5.3.8' }
Gradle's dependency management is more flexible, supporting multiple repository formats like Maven, Ivy, and flat directories.
Ant:
- Ant doesn't have built-in dependency management. Developers need to manually manage dependencies or use external tools like Ivy to achieve this functionality.
Integration with Other Tools and Services
Maven:
Maven integrates with testing frameworks (JUnit, TestNG), reporting tools (Surefire, JaCoCo), and deployment platforms (Docker, Kubernetes).
CI tools like Jenkins have strong support for Maven pipelines.
Gradle:
Gradle integrates with testing frameworks, reporting, and monitoring tools, and deployment services similar to Maven but allows more flexibility.
Gradle also works well with mobile development environments like Android Studio.
Ant:
Ant's integration with external tools is usually done through custom tasks or manually defining the integrations in the
build.xml
.Integration with CI tools like Jenkins often requires custom configuration for each environment.
9. Case Studies and Real-World Examples
Success Stories Using Maven
Apache Hadoop:
Context: Apache Hadoop, an open-source framework for distributed storage and processing, utilizes Maven to manage its large, multi-module project.
How Maven Helped:
Simplified dependency management, making it easier to handle Hadoop’s complex set of dependencies.
The ability to define parent POMs and modularise the project helped in breaking the huge project into smaller, manageable modules.
Result: Faster build times, better consistency in dependency management, and a more structured project layout.
Spring Framework:
Context: The Spring Framework uses Maven to manage its codebase and dependencies.
How Maven Helped:
Simplified multi-module project handling.
Easy integration with testing frameworks like JUnit and reporting plugins.
Result: Efficient dependency management and streamlined development processes for the Spring development team.
Success Stories Using Gradle
LinkedIn:
Context: LinkedIn switched from Ant to Gradle to build its Java-based projects due to its large-scale distributed system.
How Gradle Helped:
Gradle’s incremental build system allowed LinkedIn to reduce build times significantly.
The ability to define custom tasks and manage dependencies with flexibility was a huge advantage.
Result: Builds that were previously taking over an hour were reduced to just a few minutes. This also improved the productivity of LinkedIn’s development teams.
Android Development (Google):
Context: Google chose Gradle as the default build system for Android projects.
How Gradle Helped:
Gradle’s flexibility in managing complex project structures, especially for mobile development.
Ability to manage multi-project builds and resolve dependencies efficiently.
Integration with Android Studio and its support for dynamic build configurations.
Result: Gradle became an essential tool in the Android ecosystem, drastically improving development cycles and project modularization.
Success Stories Using Ant
Apache Tomcat:
Context: Apache Tomcat, a widely used application server, has historically used Ant to manage builds.
How Ant Helped:
Ant’s simple XML-based configuration allowed easy setup and customisation for Tomcat’s specific build and deployment requirements.
It provided flexibility in defining custom tasks and targets.
Result: Ant helped streamline Tomcat’s development process and allowed the project to grow rapidly, though later it transitioned to Maven for better dependency management.
Older Enterprise Projects:
Context: Many legacy enterprise projects, especially in the early 2000s, relied on Ant due to its simplicity and flexibility.
How Ant Helped:
Customisable build processes to suit the specific needs of enterprise-grade systems.
Easy integration with older, non-standard build requirements.
Result: Ant remained the go-to tool for many large enterprises before Gradle and Maven became more popular.
Lessons Learned and Key Takeaways
Maven:
Best for managing multi-module projects with complex dependency trees.
Simplifies the build process but can become rigid in large projects if customisation is required.
Suitable for environments where dependency management and consistency are top priorities.
Gradle:
Extremely flexible, making it ideal for both small and large projects, especially those requiring custom tasks or complex build logic.
Superior performance in terms of build caching and incremental builds.
The choice for Android development and modern DevOps pipelines.
Ant:
Still useful for legacy systems and projects that require very specific and customised build processes.
Lack of built-in dependency management is a major drawback, but its flexibility can be advantageous for certain use cases.
10. Conclusion
Recap of Key Points
Build Automation:
Essential for streamlining the development, testing, and deployment process.
Tools like Maven, Gradle, and Ant offer unique strengths based on project requirements.
Tool Comparisons:
Maven is ideal for dependency management and multi-module projects.
Gradle is the most flexible tool with excellent performance optimization.
Ant is great for customised builds in older or legacy systems but requires more manual configuration.
CI/CD Integration:
Build automation tools integrate smoothly with CI tools like Jenkins, ensuring continuous integration and deployment.
Best practices involve automating the entire pipeline, from build to testing to deployment.
Future Trends in Build Automation
Cloud-Based Build Automation:
More projects are moving towards cloud-native CI/CD solutions, allowing for scalable and distributed builds in cloud environments.
Serverless CI/CD services (like AWS CodeBuild) are gaining traction.
Containerised Builds:
- With the rise of Docker and Kubernetes, build tools are increasingly being used in containerised environments, allowing for consistent builds across different platforms.
AI-Powered Build Optimisation:
- Future build automation tools might integrate AI or machine learning to optimise build times, reduce redundancy, and predict build outcomes.
Recommendations for Choosing the Right Build Automation Tool
For Simple Projects:
Maven: If dependency management and standardisation are important.
Gradle: If flexibility and performance are key.
Ant: For projects with highly customised or legacy requirements.
For Complex Projects:
Maven: Ideal for large projects with extensive dependencies.
Gradle: Better for large, dynamic projects requiring custom logic and performance optimisation.
Subscribe to my newsletter
Read articles from Bikash Nishank directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
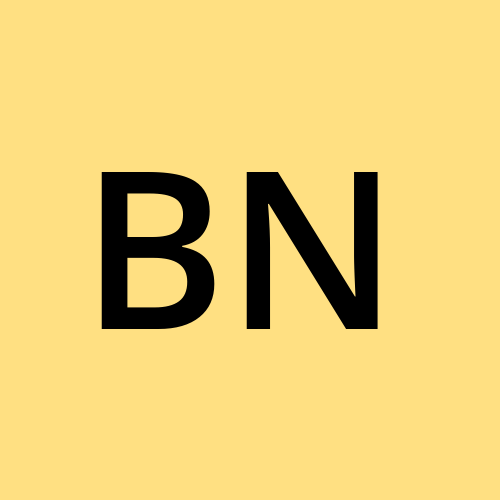