Data Preprocessing in Machine Learning with Scikit-learn

Table of contents
- 1. OneHotEncoder for Categorical Data
- 2. MinMaxScaler for Continuous Data
- 3. LabelEncoder for Categorical Labels
- 4. StandardScaler for Normalizing Features
- 5. ColumnTransformer for Simultaneous Transformations
- 6. Handling Missing Values with SimpleImputer
- 7. Splitting Data into Training and Testing Sets
- 8. Evaluating Model Performance with Accuracy Score
- Conclusion
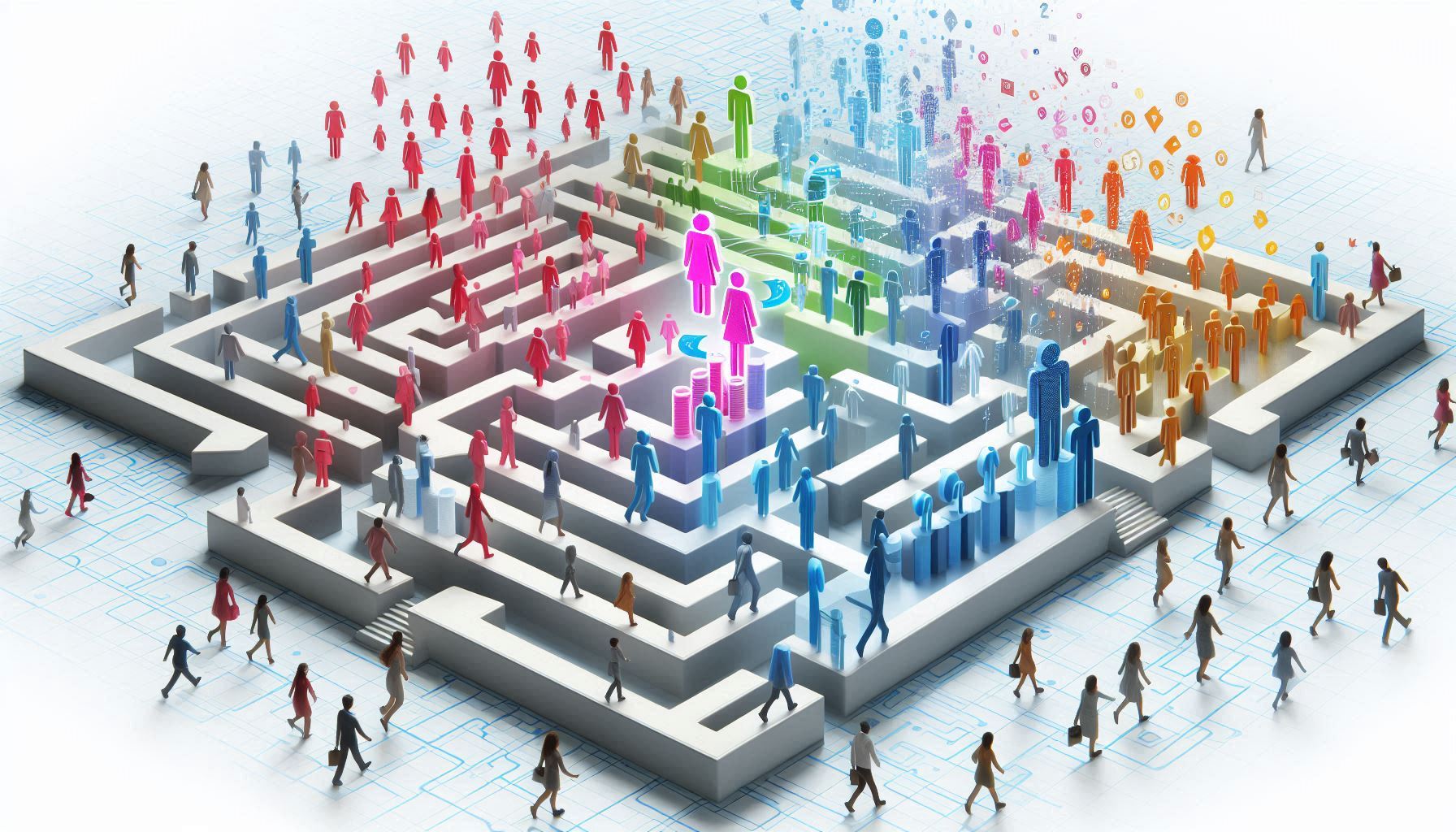
Data preprocessing is a crucial step in the machine learning pipeline. It helps in preparing the data for modeling by transforming features, scaling data, handling missing values, and encoding categorical variables. In this post, we will explore common data preprocessing techniques using the Scikit-learn library in Python.
1. OneHotEncoder for Categorical Data
The OneHotEncoder
is used to convert categorical values into a format that can be provided to machine learning algorithms to improve predictions.
from sklearn.preprocessing import OneHotEncoder
encoder = OneHotEncoder()
encoder.fit(df[['ColName']])
categories = encoder.categories_ # View categories and indices
encoded_data = encoder.transform(df[['ColName']]).toarray() # Transform and view array
.categories_
: Shows the unique categories and their column indices..transform()
: Converts categorical data into one-hot encoded values.
2. MinMaxScaler for Continuous Data
MinMaxScaler
is used to scale features between a given range, typically 0 and 1. It is useful for ensuring that the values fall within a uniform range.
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
scaler.fit(df[['ColName']]) # Fit the scaler to the data
df['NewColName'] = scaler.transform(df[['ColName']]) # Apply transformation
This is typically used for continuous variables where scaling between a set range is required.
3. LabelEncoder for Categorical Labels
The LabelEncoder
is used to encode target labels with values between 0 and n_classes-1
. This is useful for transforming string labels (such as class names) into numeric labels.
from sklearn.preprocessing import LabelEncoder
le = LabelEncoder()
df['NewColName'] = le.fit_transform(df['ColName']) # Fit and transform the column
This technique is helpful when converting non-numeric labels into numeric values.
4. StandardScaler for Normalizing Features
StandardScaler
standardizes features by removing the mean and scaling them to unit variance. It is useful when working with models sensitive to feature scaling.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
df['NewColName'] = scaler.fit_transform(df[['ColName']])
This scaler is particularly helpful for algorithms such as SVM or KNN, which assume normally distributed data.
5. ColumnTransformer for Simultaneous Transformations
ColumnTransformer
allows you to apply different preprocessing techniques to different columns in your dataset. For example, you can apply one hot encoding to categorical columns and scaling to numerical columns simultaneously.
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import StandardScaler, OneHotEncoder
ct = ColumnTransformer(transformers=[
('num', StandardScaler(), ListOfContinuousColumns),
('cat', OneHotEncoder(), ListOfCategoricalColumns)
])
X_train_transformed = ct.fit_transform(X_train)
X_test_transformed = ct.fit_transform(X_test)
This method is ideal for datasets with both numerical and categorical features.
6. Handling Missing Values with SimpleImputer
SimpleImputer
is used to handle missing values by replacing them with a statistical value such as the mean, median, or most frequent value.
from sklearn.impute import SimpleImputer
import numpy as np
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
df = imputer.fit_transform(df) # Apply the imputer to fill missing values
The SimpleImputer
is essential for datasets with missing data that could affect model performance.
7. Splitting Data into Training and Testing Sets
Splitting the data into training and testing sets is essential for evaluating the model's performance on unseen data.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42, stratify=y)
test_size
: Specifies the proportion of data to include in the test split (e.g., 20%).random_state
: Ensures reproducibility of the split.stratify=y
: Maintains the proportion of target labels in the split.
8. Evaluating Model Performance with Accuracy Score
The accuracy score is a common evaluation metric used to assess the performance of a classification model.
from sklearn.metrics import accuracy_score
y_pred = clf.predict(X_test) # Predict using your trained model
accuracy = accuracy_score(y_test, y_pred) # Calculate accuracy
This method is used after predicting the labels with a model to evaluate how accurately it performs.
Conclusion
Data preprocessing is an essential step in the machine learning pipeline. Using Scikit-learn, you can easily scale, transform, and encode your dataset to prepare it for modeling. Proper preprocessing helps ensure that your models receive data in the best format, improving both training time and model accuracy.
For more details on preprocessing, check out the Scikit-learn documentation.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.