A Beginner’s Guide to Contributing to Open Source: From Fork to Pull Request
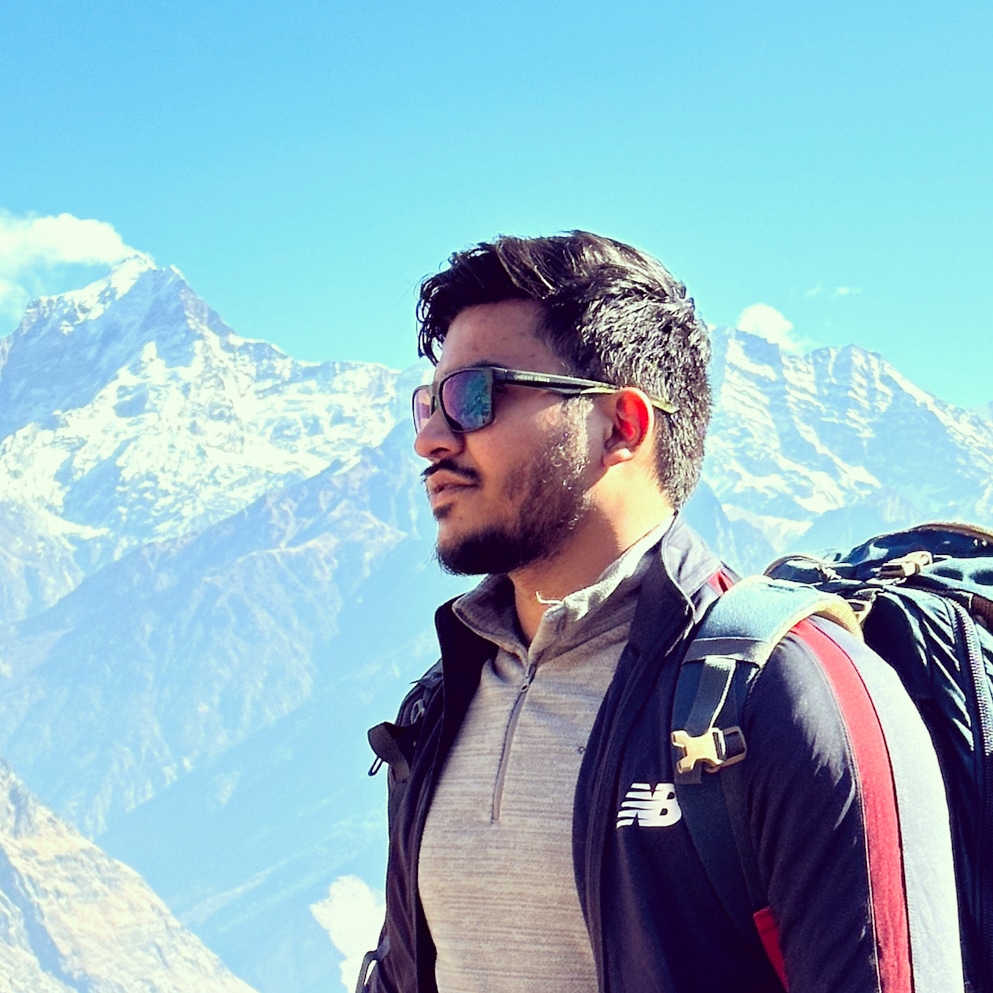
Table of contents

Contributing to open source can be a rewarding experience, offering opportunities to learn new skills, collaborate with others, and make a tangible impact on projects that matter. If you’re new to open source or looking for a project to contribute to, Open-Runtimes, a sub-project of Appwrite, offers an excellent starting point.
In this blog post, I want to share my experience contributing to Open Runtimes, particularly focusing on a Java function I added to the project. This function generates a QR code for any given text, a feature that can be quite useful in various applications. Whether you’re a seasoned developer or a newcomer eager to get involved, this guide will provide you with a clear, step-by-step approach to contributing to Open Runtimes. From setting up your environment to creating a pull request, you’ll gain insights into the process and learn how you can start making valuable contributions yourself.
Before diving into the details of my contribution, let’s take a moment to understand what Open Runtimes is and why it matters.
What is Open Runtimes?
Open Runtimes is a platform designed to provide runtime environments for serverless cloud computing across multiple programming languages. With Open Runtimes, you can develop serverless applications using your preferred language and leverage containerized environments to manage execution. This flexibility simplifies the development process and enables scalable, reliable cloud solutions.
Steps to Contribute to Open Runtimes
Contributing to an open source project like Open Runtimes involves several key steps. Here’s a detailed guide on how you can make your own contributions:
Fork the Repository
Start by forking the Open Runtimes repository on GitHub. This creates a copy of the repository under your own GitHub account, allowing you to make changes without affecting the original project.
Go to the Open Runtimes examples repository on GitHub.
Click the “Fork” button in the top right corner to create your own copy.
Clone the Repository
Next, download your forked repository to your local machine using the git clone
command.
git clone https://github.com/your-username/examples.git
Replace your-username
with your GitHub username.
Update Your Default Branch
Before creating a new branch, make sure your default branch (usually main
or master
) is up to date with the latest changes from the upstream repository. This ensures you’re working with the most recent version of the code.
cd examples
git pull origin main
Create a New Branch
Create a new branch for your changes. It’s a good practice to name your branch descriptively based on the feature or fix you’re working on. For example, I am creating a function to generate QR code so I can use branch name as feat-generate-qr-code-example-function-using-java
.
git checkout -b feat-generate-qr-code-example-function-using-java
This command switches you to the new branch so you can start making changes without affecting the default branch.
Create a Directory for Your Function
With your new branch set up, you’ll need to create a directory within the appropriate language directory for your function. Since our function is written in Java, navigate to the java
directory and create a new directory for your QR code generation function.
cd java
mkdir generate_qr_code
cd generate_qr_code
This creates and navigates into a new directory called generate_qr_code
.
Write Your Code
Now that you have your directory set up, you can start writing your code. For this example, I have created an Index.java
file where the core functionality of my QR code generation will be implemented. Additionally, you’ll need a deps.gradle
file to specify any dependencies your function requires.
Create
Index.java
: This file will contain the Java code to generate a QR code for the given text.Create
deps.gradle
: This file should include any dependencies needed for your function. For the QR code generation, I have used qrcodegen librarydependencies { implementation 'io.nayuki:qrcodegen:1.4.0' }
Build and Test Your Code
With your function written, the next step is to build and test it to ensure everything is working as expected.
Prerequisite: Install Docker
Before you can build and test your code, you need to have Docker installed on your computer. Docker is essential for creating containers that run your function in a consistent environment. If you don’t have Docker installed, follow the instructions on the Docker website to install it.
Build the Code
To build the code, we'lluse Docker to create a container that compiles the Java function. Run the following command:
docker run -e OPEN_RUNTIMES_ENTRYPOINT=Index.java --rm --interactive --tty --volume $PWD:/mnt/code openruntimes/java:v4-8.0 sh helpers/build.sh
This command does the following:
-e OPEN_RUNTIMES_ENTRYPOINT=Index.java
: Specifies the entry point of your function.--rm
: Removes the container after the build is complete.--interactive --tty
: Runs the container in interactive mode.--volume $PWD:/mnt/code
: Mounts your current directory to/mnt/code
in the container.openruntimes/java:v4-8.0
: Specifies the Docker image to use.sh helpers/build.sh
: Runs the build script inside the container.
After running this command, a code.tar.gz
file will be generated, containing the built code.
Start the Open Runtime
To start the Open Runtime and test the function, run the following command:
docker run -p 3000:3000 -e OPEN_RUNTIMES_SECRET="" --rm --interactive --tty --volume $PWD/code.tar.gz:/mnt/code/code.tar.gz:ro openruntimes/java:v4-8.0 sh helpers/start.sh "java -jar /usr/local/server/src/function/java-runtime-1.0.0.jar"
Here’s what this command does:
-p 3000:3000
: Maps port 3000 on your host to port 3000 in the container.-e OPEN_RUNTIMES_SECRET=secret-key
: Sets an environment variable for authorization.--volume $PWD/code.tar.gz:/mnt/code/code.tar.gz:ro
: Mounts thecode.tar.gz
file into the container as read-only.openruntimes/java:v4-8.0
: Specifies the Docker image to use.sh helpers/start.sh "java -jar /usr/local/server/src/function/java-runtime-1.0.0.jar"
: Starts the runtime with the specified JAR file.
Your function should now be running and listening on port 3000.
Test Your Function
In a new terminal window, you can test your function by sending a
curl
request:curl http://localhost:3000/?text=https://github.com/open-runtimes
This command sends a request to the running function with a JSON payload.
To learn more about the Java runtime and its usage, you can visit the Java runtime README.
Create a README for Your Contribution
Once your function is working as expected, it’s essential to document it properly. A well-crafted README will help others understand and use your function effectively. Here’s a sample README for the QR code generation function.
Add, Commit, and Push Your Changes
With your code and README ready, the final step is to add your changes, commit them to your local repository, and push them to your fork on GitHub. Here’s a step-by-step guide:
Add Your Changes
First, add all the changes to the staging area. This includes your new code files, README, and any other modifications.
git add .
The
.
adds all changes in the current directory and its subdirectories.Commit Your Changes
Next, commit the changes with a descriptive message. This message should briefly explain what your commit includes, such as the addition of a new function and README.
git commit -m "Add QR code generation function and README"
Ensure your commit message is clear and descriptive to make it easier for others (and your future self) to understand the changes.
Push Your Changes
Finally, push your changes to your remote repository on GitHub. This updates your fork with the new code and README.
git push origin feat-generate-qr-code-example-function-using-java
Generate a Pull Request on GitHub
Once you’ve pushed your changes to your fork, the next step is to create a pull request (PR) to propose your changes to the main repository. Follow these steps to generate a pull request:
Go to the Main Repository
- Navigate to the feature brach of forked Open Runtimes repository on your GitHub.
Create a Pull Request
Click the “Contribute” tab .
Click the “Open Pull Request” button.
Review Your Changes
Review the changes you’ve made in the diff view to ensure everything is correct.
Ensure that the changes look as expected and that no unintended modifications are included.
Add a Title and Description
Add a title for your pull request. A clear and descriptive title helps reviewers understand the purpose of the PR.
Provide a description detailing what your pull request does. This should include:
Short introduction about changes made.
Screenshots showing output (success response and failed response)
Any relevant details about the implementation.
Task Summary
Here's sample Pull request for the QR code generation function.
Create the Pull Request
- Once you’ve reviewed everything and added the necessary information, click the “Create Pull Request” button.
Monitor the Pull Request
After creating the PR, monitor it for feedback from the repository maintainers. They may request changes or approve the pull request.
Be responsive to any comments or requests for additional information.
You can reach out to apprwrite's awesome team on discord as well if you have any query.
Resources
PS: PR got merged into the main branch!!🚀
Subscribe to my newsletter
Read articles from Seemant Shekhar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
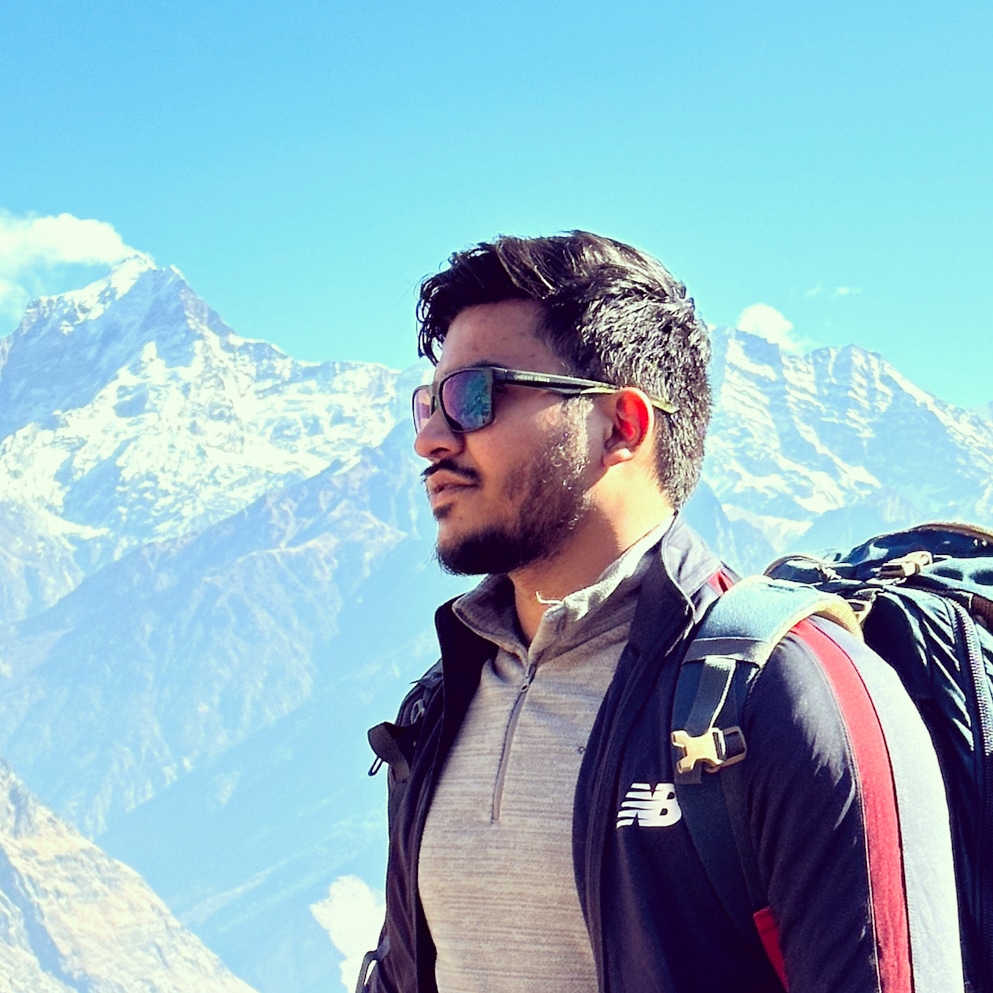