Java Programming Interview Questions with Answers (Part-1)

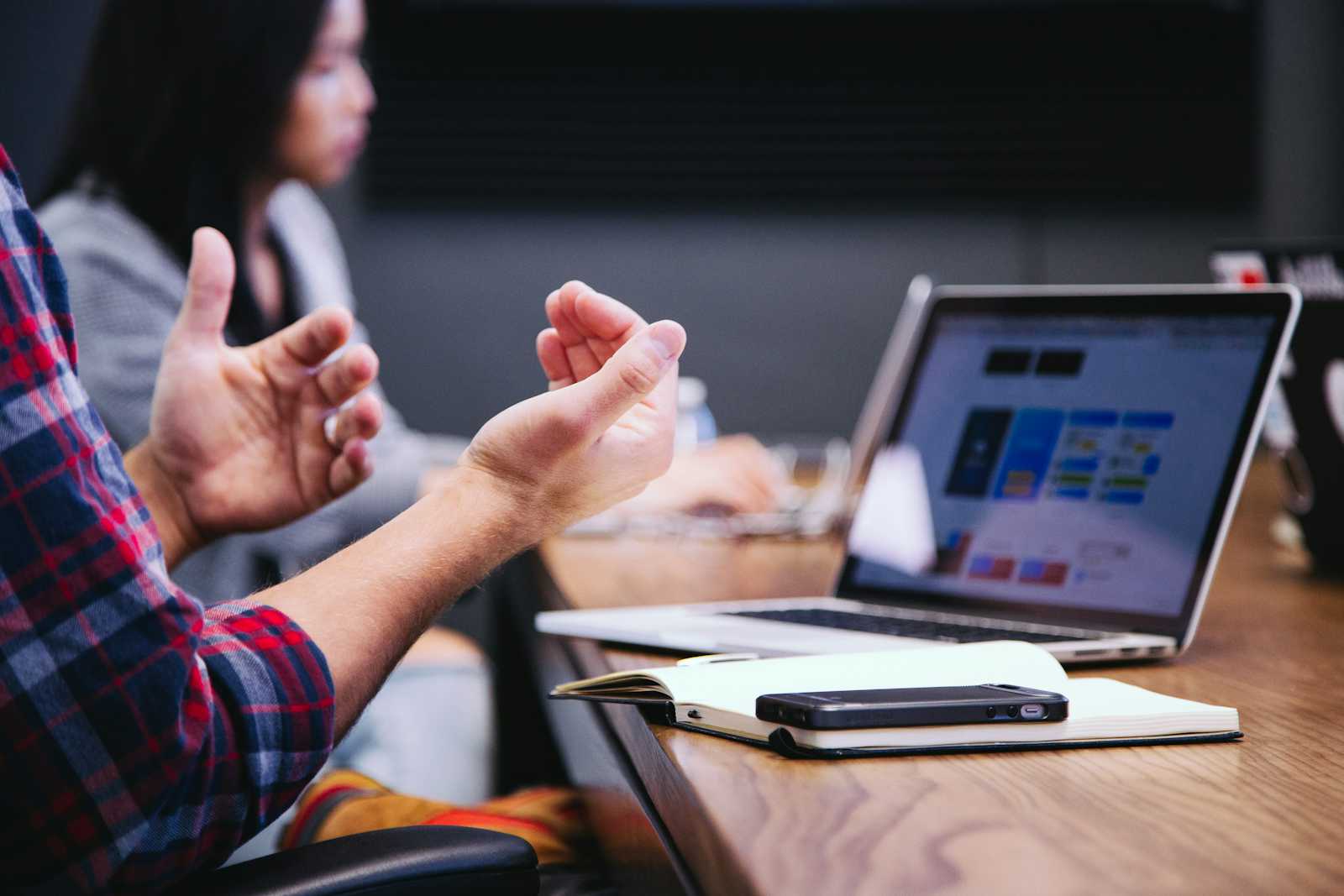
Hello Friends, I hope you guys are safe and doing great work. Today I am going to discuss some Java interview questions. I am trying to give as simple codes as possible. I have been preparing this MEGA list for quite some time and now It's ready to share with you guys.
I have also worked hard to keep this list up-to-date to include what interviewers are asking nowadays and what will be asked in the upcoming years
Question 1 - Calculate the Factorial of a number with and without Recursion in Java.
Before starting coding, I explain how we dry run the program. It will easily help you to understand the flow of the program.
Let's take an example 5. num = 5; fact = 1; //5*4*3*2*1
fact 1 = fact; // 1 *1 =1
fact *2= fact; // 1**2=2
fact * 3 = fact; *// 2**3=6
fact * 4= fact; *// 6**4=24
fact * 5 = fact; *// 24**5=120
Now, let's start Coding. The class name of my program is factorialclass.
public class factorialclass(){
public static void main(String[] args){
int num = 5;
fact = 1;
for(int i=1; i<=num; i++){
fact = fact * i;
}
System.out.println ("Factorial of " + num + " is - " + fact);
}
}
Using function:-
public class factorialclass(){
public static void main(String[] args){
int num = 5;
int ans = 1;
answer = fact(num);
System.out.println ("Factorial of " + num + " is - " + ans);
}
static int fact(int a){
int b = 1;
for(int i=1; i<=a; i++){
b = b* i;
}
return b;
}
}
Next, we write this code using the Recursive Function. Recursive means a function that calls itself to break down a problem into smaller, subproblems.
public class factorialclass(){
public static void main(String[] args){
int num = 5;
int ans = 1;
answer = fact(num);
System.out.println ("Factorial of " + num + " is - " + ans);
}
static int fact(int a){
if(a<=1){
return 1;
}else{
return a*fact(a-1);
}
}
}
Question 2 - Write a program to find the sum of Digits of a given number.
I am giving here a small overview of my program.
package com.mycompany.java_practice_questions;
import java.util.Scanner;
public class Sum_of_digits {
public static void main(String[] args) {
int num = 0, sum = 0, rem = 0;
Scanner scan = new Scanner(System.in);
System.out.println("Please enter a number");
num = scan.nextInt();
while(num > 0){
rem = num %10;
sum = sum + rem;
num = num /10;
}
System.out.println(sum);
}
}
Subscribe to my newsletter
Read articles from Shivani Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
