JavaScript and Node.js Naming Conventions
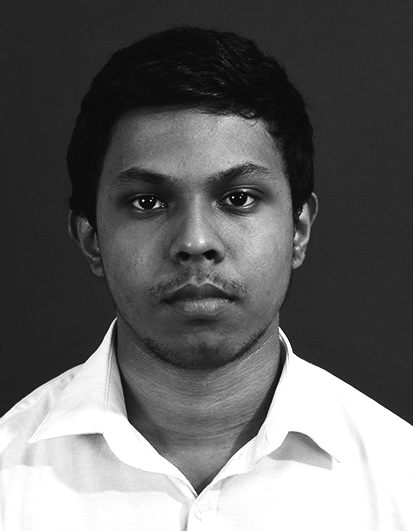
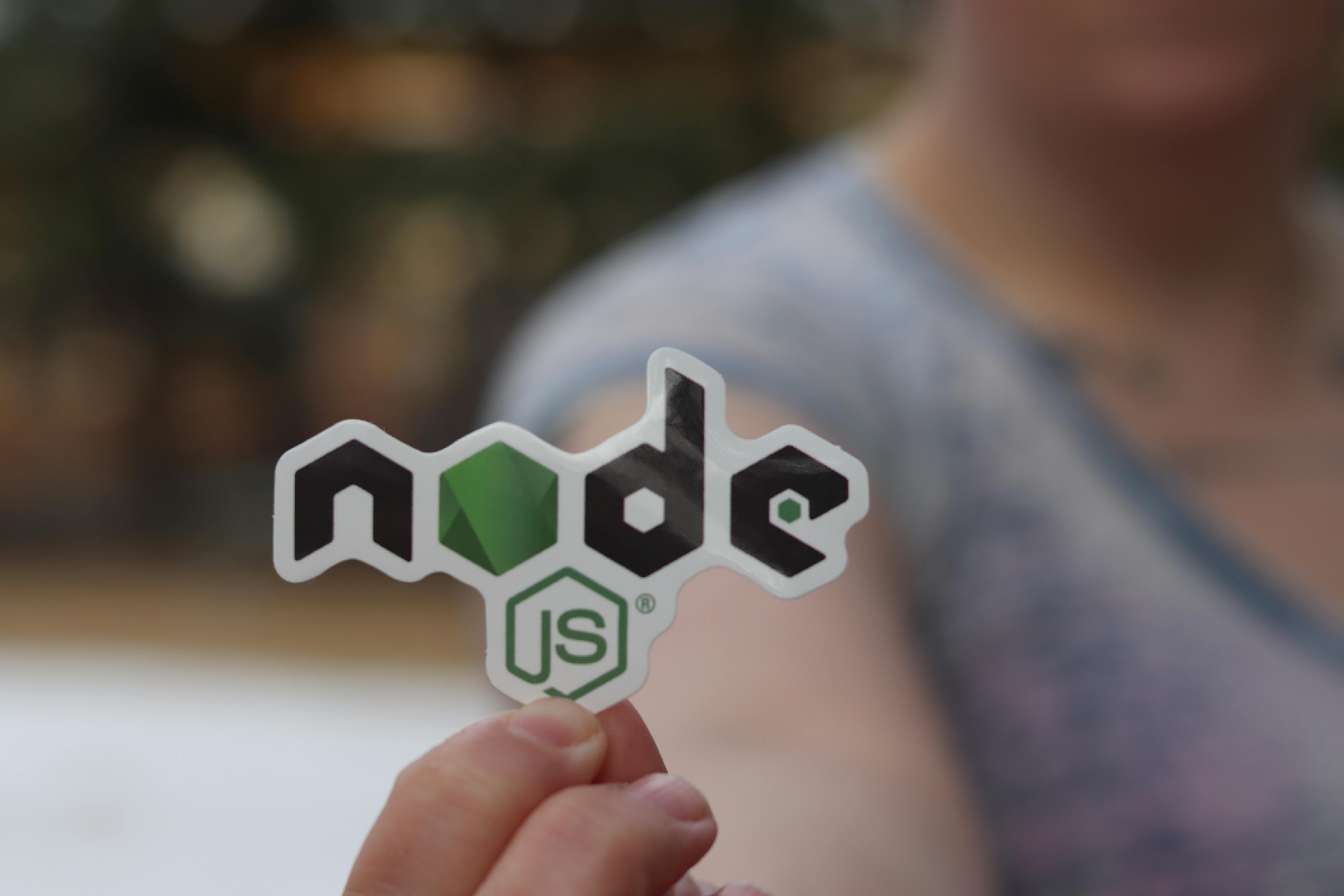
Naming stuff in JavaScript (JS) and Node.js isn’t just about looking cool. It helps avoid confusion, prevent errors, and make sure that when you or someone else looks at the code later, they get what’s going on.
1. Variables: The Backbone of Your Code
JS variable names are case-sensitive. This means dogName
, DogName
, and DOGNAME
are three different variables. To keep things clean, use camelCase—start with lowercase, then capitalize the next word.
let dogName = 'Scooby';
Bad naming examples:
let dogname = 'Scooby'; // not clear
let DOG_NAME = 'Scooby'; // reserved for constants, bro!
Use names that make sense. If you’re storing a dog’s name, call it dogName
, not just name
.
2. Booleans: Keep It Simple, Keep It True
Boolean variables (those that can only be true or false) should start with is, has, or should to make their purpose clear.
let isBarking = true;
let hasOwner = false;
3. Functions: What Does It Do?
Function names should clearly describe their job and use camelCase too. The name should be a verb since functions do something.
function getDogName() {
return 'Scooby';
}
Don’t name your function dog()
. What does that even mean? Functions should say what they do.
4. Constants: Immutable and Screaming
Constants should be in UPPER_SNAKE_CASE. They don’t change, so make them stand out.
const MAX_RETRIES = 5;
const API_KEY = '123xyz';
5. Classes: A Whole New World
Classes represent objects and should use PascalCase (capitalize the first letter of every word).
class DogCartoon {
constructor(dogName) {
this.dogName = dogName;
}
}
Classes are like molds—you create new instances (objects) from them. That’s why they have special treatment.
6. Private Variables: Keep It on the Down-Low
In JavaScript, we use underscore (_
) to signify that a variable or method is private and shouldn’t be messed with outside its scope.
class Dog {
constructor() {
this._bark = true; // private variable
}
}
Modern JS also has #private fields:
class Dog {
#bark = true; // truly private
}
7. Double Underscore: What’s the Deal?
In Node.js, double underscores (__
) are used in a couple of specific, built-in global variables __dirname
and __filename
. These give you the directory and filename of the running script. Don’t go using them just anywhere.
console.log(__dirname); // Prints the directory path
console.log(__filename); // Prints the full path to the file
These aren’t magic, but they’re super useful for working with file paths and directories in Node.js.
8. Files and Folders: Keep it Simple, Keep it Clean
When naming files, use lowercase and separate words with dashes (-
) or underscores (_
). No one likes a file named DogClass.js
when you could simply name it dog_class.js
.
app/
controllers/
user_controller.js
models/
user_model.js
Stick with lowercase and kebab-case (dog-barks.js
) for filenames—it’s cleaner and avoids headaches when moving between different operating systems.
By following these simple conventions, you keep your code readable, maintainable, and less prone to errors. It’s not about impressing anyone—it’s about making sure your future self (and others) can understand what the heck is going on in your code. So, next time you write some JS or Node.js, follow these best practices and thank yourself later!
And remember, naming things is hard, but it’s worth the effort!
Sources:
Subscribe to my newsletter
Read articles from Nirmal Sankalana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
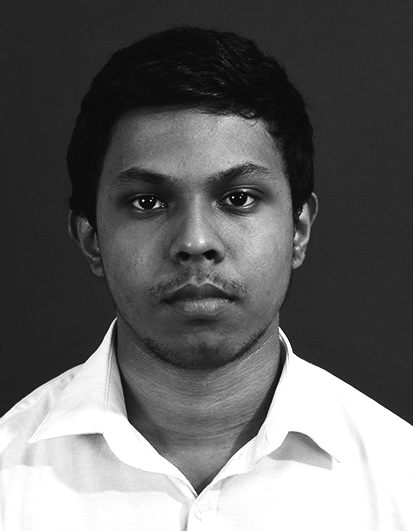