Building a CLI Application in Go

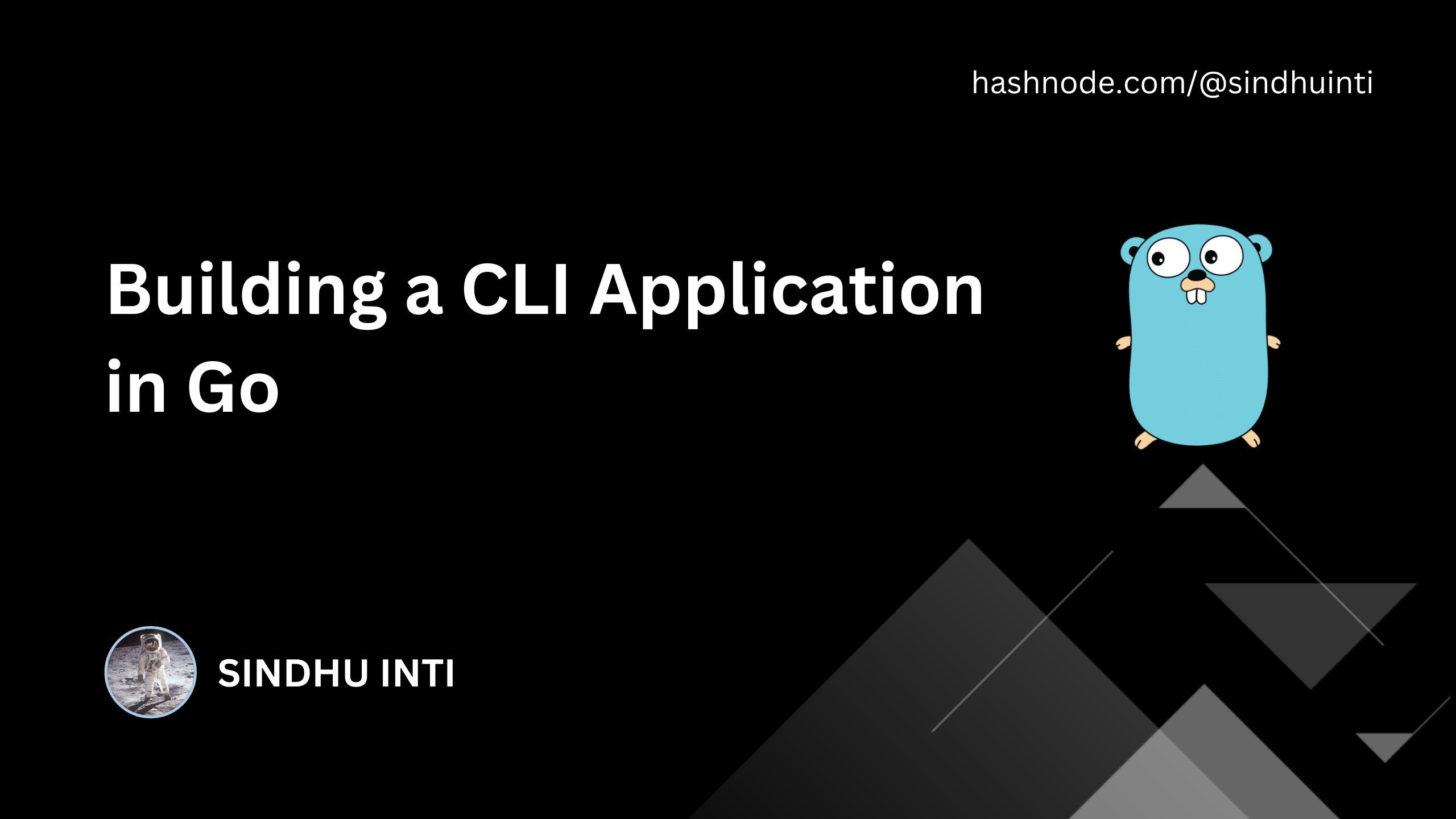
Go is an open source programming language that makes it simple to build secure, scalable systems. With its rich ecosystem and powerful libraries, Go simplifies the process of building robust tools for developers. One of the most popular libraries for building CLI applications in Go is Cobra
.
I have created a CLI app using Cobra
named Chronx
. It allows you to access your Google Calendar(s) from a command line. It's very easy to get your events, search for events, add new events, delete events from your terminal without any extra clicks.
Checkout here - https://github.com/Sindhuinti/Chronx
In this tutorial, I'll walk you through creating a simple CLI app using Cobra
. This CLI will include a greet
command that takes a user’s name as input and returns a greeting. We’ll also explore how to add flags to customize the behavior of the command.
Why Use Cobra?
Cobra
is a library for creating powerful modern CLI applications. Cobra is used in many Go projects such as Kubernetes, Hugo, and GitHub CLI to name a few. This list contains a more extensive list of projects using Cobra.
Step-by-Step Guide to Building a CLI App with Cobra
Let’s create a simple CLI tool called mycli
, which has one command, greet
, that prints a greeting message with optional customization through flags.
Step 1: Setting Up Your Go Project
First, create a directory for your Go project and initialize it with Go modules.
mkdir mycli
cd mycli
go mod init mycli
Next, install the Cobra
package:
go get -u github.com/spf13/cobra
Step 2: Creating the Root Command
The root command is the entry point of any Cobra-based CLI application. Create a main.go
file to define it:
package main
import (
"fmt"
"github.com/spf13/cobra"
"os"
)
var rootCmd = &cobra.Command{
Use: "mycli",
Short: "MyCLI is a simple CLI tool",
Long: "MyCLI is a simple command-line tool built with Cobra in Go.",
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("Welcome to MyCLI! Use the greet command to receive a greeting.")
},
}
func Execute() {
if err := rootCmd.Execute(); err != nil {
fmt.Println(err)
os.Exit(1)
}
}
func main() {
Execute()
}
This sets up a basic CLI structure where rootCmd
is the main entry point. When you run ./mycli
, it will print a welcome message by default.
Step 3: Adding the greet
Command
Now, let’s add a greet
command that takes a person’s name and prints a greeting. Create a new file called greet.go
:
package main
import (
"fmt"
"strings"
"github.com/spf13/cobra"
)
var shout bool
var greetCmd = &cobra.Command{
Use: "greet [name]",
Short: "Greets a person by name",
Long: "This command takes a name as an argument and prints a personalized greeting.",
Args: cobra.MinimumNArgs(1),
Run: func(cmd *cobra.Command, args []string) {
name := args[0]
greeting := fmt.Sprintf("Hello, %s!", name)
if shout {
greeting = strings.ToUpper(greeting)
}
fmt.Println(greeting)
},
}
func init() {
rootCmd.AddCommand(greetCmd)
greetCmd.Flags().BoolVarP(&shout, "shout", "s", false, "Shout the greeting in uppercase")
}
Here’s what’s happening:
The
greet
command takes a name as an argument and prints "Hello, [name]!".The
shout
flag, when set (--shout
or-s
), changes the greeting to uppercase.The command expects at least one argument (the name).
We also use greetCmd.Flags().BoolVarP()
to define a shout
flag, which allows users to customize the behavior of the command.
Step 4: Running the Application
Now that we have both the root and greet
commands, we can build and run the application.
First, build the project:
go build -o mycli
Now, try running it without any commands:
./mycli
Output:
Welcome to MyCLI! Use the greet command to receive a greeting.
Now, try using the greet
command:
./mycli greet Sindhu
Output:
Hello, Sindhu!
If you want to shout the greeting, use the --shout
flag:
./mycli greet Sindhu --shout
Output:
HELLO, Sindhu!
Step 5: Automatically Generated Help
One of the great features of Cobra is that it automatically generates a help menu for your commands. You can view the help by running:
./mycli greet --help
This will display the description of the greet
command and its usage, including any flags:
Usage:
mycli greet [name] [flags]
Flags:
-h, --help help for greet
-s, --shout Shout the greeting in uppercase
Conclusion
Cobra makes it easy to create scalable CLI applications with rich features like automatic help generation, argument parsing, and flag handling. Now that you have the basics, you can expand on this by adding more commands and features to suit your project’s needs.
Happy coding!
Subscribe to my newsletter
Read articles from Sindhu Inti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
