Methods in Java
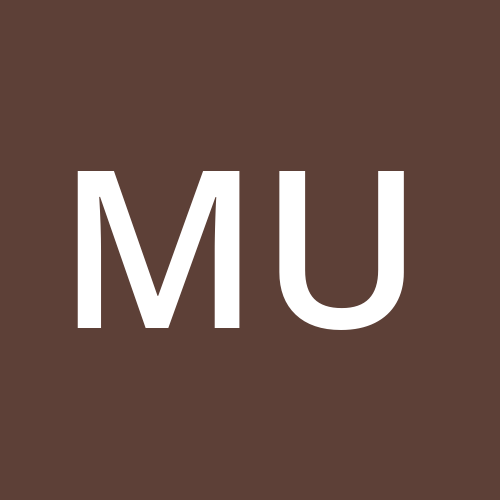
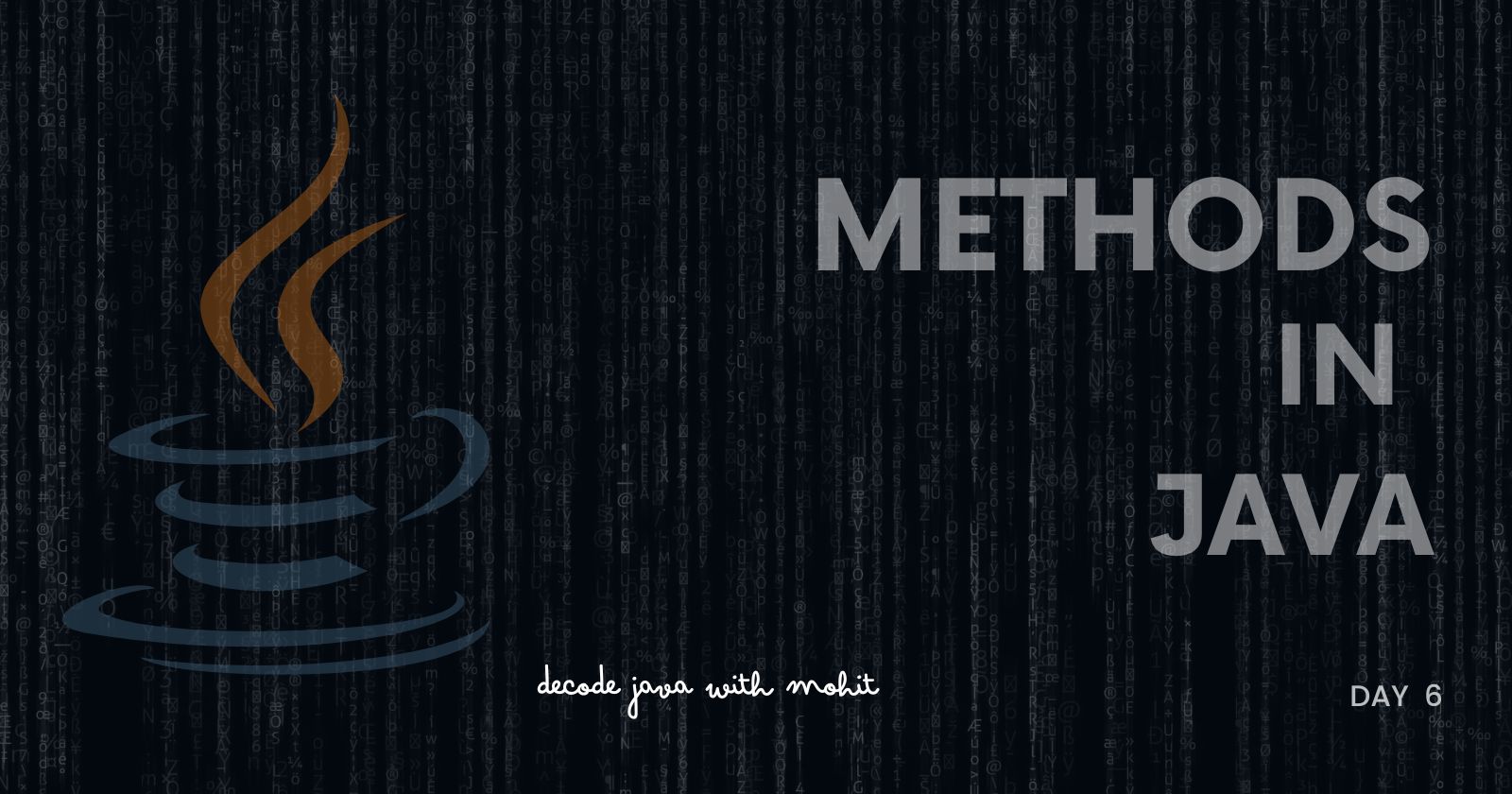
On Day 6, the focus will be on Methods in Java, which are the building blocks of any Java program. Methods are used to break down complex problems into smaller, manageable pieces, enhance code reusability, and make the program more modular and organized.
1. What is a Method in Java?
A method in Java is a block of code or statements that performs a specific task and can be executed when called. Methods allow us to avoid repeating code and help organize our logic in a more readable way.
Syntax of a Method:
returnType methodName(parameters) {
// method body
}
returnType
: Specifies the data type of the value that the method will return. If the method does not return anything, the return type isvoid
.methodName
: The name of the method.parameters
: Input values that a method accepts (optional). If no inputs are required, the parameter list can be empty.method body
: Contains the logic or code that defines the behavior of the method.
2. Defining and Calling Methods
Let's define a simple method and see how it is called:
Example:
public class MyClass {
// Defining a method
public static void printMessage() {
System.out.println("Hello, World!");
}
// Main method to call the printMessage method
public static void main(String[] args) {
printMessage(); // Calling the method
}
}
Explanation:
The method
printMessage()
is defined to print "Hello, World!" to the console.The method is called in the
main()
method using the method name followed by parentheses.
3. Method with Parameters
Methods can accept parameters (or arguments) to process data passed to them. This allows methods to be flexible and work on dynamic input.
Example:
public class MyClass {
// Method with parameters
public static void greet(String name) {
System.out.println("Hello, " + name);
}
public static void main(String[] args) {
greet("Alice"); // Output: Hello, Alice
greet("Bob"); // Output: Hello, Bob
}
}
Explanation:
The
greet
method takes aString
parametername
and prints a greeting message.By passing different names, the method outputs personalized greetings.
4. Method with Return Type
A method can also return a value after processing. The return type specifies the type of value the method will return.
Example:
public class MyClass {
// Method with a return type
public static int addNumbers(int a, int b) {
return a + b;
}
public static void main(String[] args) {
int result = addNumbers(5, 7);
System.out.println("Sum: " + result); // Output: Sum: 12
}
}
Explanation:
The
addNumbers
method takes two integer parameters and returns their sum.The return value is stored in the
result
variable, which is then printed.
5. Method Overloading
Java allows you to define multiple methods with the same name but different parameter lists. This is called method overloading. Overloaded methods provide flexibility by allowing different types or numbers of parameters.
NOTE : We will learn about Method Overriding
in the OOPs Concept.
Example:
public class MyClass {
// Method overloading
public static int add(int a, int b) {
return a + b;
}
public static double add(double a, double b) {
return a + b;
}
public static void main(String[] args) {
System.out.println(add(5, 7)); // Output: 12 (int version)
System.out.println(add(5.5, 7.3)); // Output: 12.8 (double version)
}
}
Explanation:
The
add
method is overloaded: one version works with integers, and the other works with doubles.Depending on the data type of the arguments passed, the appropriate version of the method is called.
6. Method Scope and Access Modifiers
Methods can have different access levels depending on the access modifiers applied to them. The most commonly used access modifiers are:
public: The method can be accessed from anywhere.
private: The method can only be accessed within the class.
protected: The method can be accessed within the package and by subclasses.
default (no modifier): The method is accessible within the same package.
Example:
public class MyClass {
private static void display() {
System.out.println("Private method");
}
public static void main(String[] args) {
display(); // Allowed since it's within the same class
}
}
Explanation:
- The
display
method is markedprivate
, so it can only be called within theMyClass
class.
7. Static vs Instance Methods
In Java, methods can be either static or instance methods.
Static methods: Belong to the class and can be called without creating an instance of the class. These methods use the
static
keyword.Instance methods: Belong to an instance (object) of a class and can only be called after creating an object of the class.
Example (Static Method):
public class MyClass {
public static void staticMethod() {
System.out.println("Static method");
}
public static void main(String[] args) {
staticMethod(); // Called without creating an object
}
}
Example (Instance Method):
public class MyClass {
public void instanceMethod() {
System.out.println("Instance method");
}
public static void main(String[] args) {
MyClass obj = new MyClass(); // Creating an object
obj.instanceMethod(); // Calling instance method
}
}
Key Difference:
Static methods are called using the class name or directly within the same class.
Instance methods are called through an object of the class.
8. Recursion in Methods
A method can call itself. This technique is known as recursion. It is useful for solving problems that can be broken down into smaller, similar sub-problems.
Example:
public class MyClass {
// Recursive method to calculate factorial
public static int factorial(int n) {
if (n == 1 || n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
System.out.println(factorial(5)); // Output: 120
}
}
Explanation:
- The
factorial
method calls itself until the base case (n == 1
&n == 0
) is reached.
9. Practical Example: Maximum of Three Numbers
Here’s a simple method that takes three numbers as arguments and returns the largest of the three.
Example:
public class MyClass {
// Method to find the maximum of three numbers
public static int max(int a, int b, int c) {
if (a > b && a > c) {
return a;
} else if (b > a && b > c) {
return b;
} else {
return c;
}
}
public static void main(String[] args) {
System.out.println("Max: " + max(10, 20, 15)); // Output: Max: 20
}
}
Explanation:
- The
max
method compares the three numbers using conditional statements and returns the largest number.
Summary
By the end of Day 6, we will have a comprehensive understanding of:
Defining and calling methods in Java.
Using parameters and return types in methods.
Overloading methods.
Static vs. instance methods.
Access control using modifiers.
Implementing recursion.
Practical problem-solving with methods.
Mastering methods in Java is crucial for writing clean, modular, and reusable code. Understanding how methods work will enhance your ability to design better programs. So Stay tuned! for further topics.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
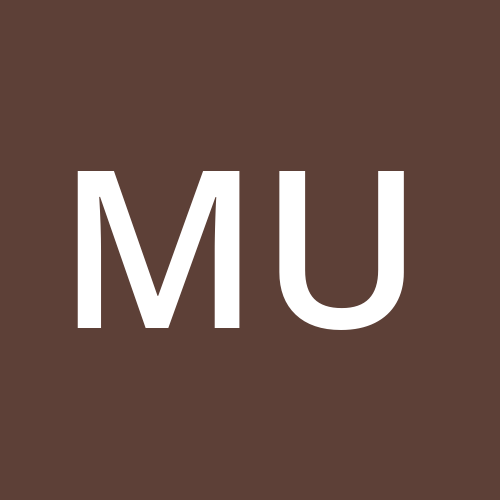