Introduction to Object-Oriented Programming (OOPs) Concept in Java
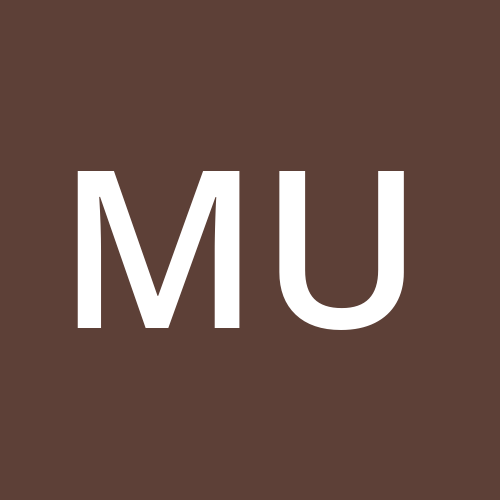
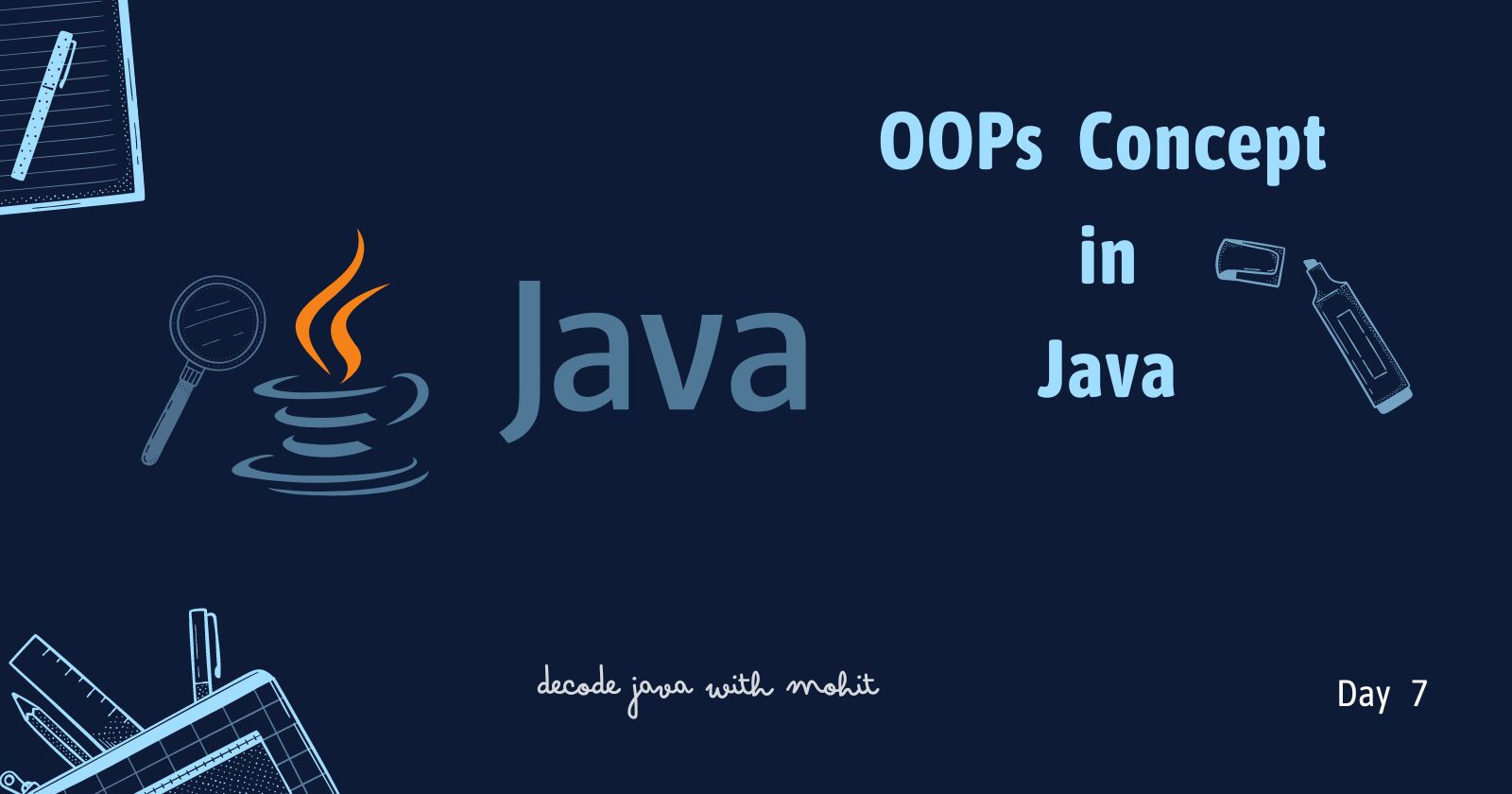
On Day 7, the focus will be on introducing the core concepts of Object-Oriented Programming (OOPs) in Java. OOPs is a programming paradigm that structures a program using objects and classes. It allows for modular, scalable, and reusable code, making it one of the most popular paradigms in modern programming.
1. What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of objects, which are instances of classes. Objects contain both data (fields or attributes) and methods (functions or behavior) that operate on the data.
The key idea behind OOP is to model real-world entities as objects and create a program that can interact with these objects.
2. Key Principles of OOP
There are four fundamental principles of OOPs that provide a structure for organizing and designing object-oriented programs:
1. Encapsulation
Definition: Encapsulation is the concept of wrapping data (variables) and methods (functions) that operate on the data into a single unit called a class.
It restricts direct access to some components of an object to ensure controlled access through methods.
Example: Use of private variables and public getter/setter methods to control access.
Example:
public class Student {
// Encapsulation: private fields
private String name;
private int age;
// Public methods to access private fields (getters & setters)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (age > 0) {
this.age = age;
}
}
}
Explanation:
The
name
andage
fields are private, meaning they cannot be accessed directly from outside the class.Access to the fields is controlled using public methods (
getName
,setName
, etc.), which allows validation or other logic to be applied.
2. Inheritance
Definition: Inheritance is a mechanism where one class can inherit properties and behaviors (fields and methods) from another class.
It promotes code reuse and establishes a hierarchical relationship between classes.
Example: A
Dog
class can inherit from aAnimal
class, reusing the attributes and methods common to all animals.
Example:
// Parent class
class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
// Child class inherits from Animal
class Dog extends Animal {
// Override method to provide specific implementation for Dog
public void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.sound(); // Output: Dog barks
}
}
Explanation:
The
Dog
class inherits the properties and methods of theAnimal
class.The
Dog
class overrides thesound
method to provide its specific behavior.
3. Polymorphism
Definition: Polymorphism allows objects to be treated as instances of their parent class, and methods can be implemented in different ways (method overriding and method overloading).
Types:
Compile-time Polymorphism (Method Overloading): The same method name can have different signatures (different parameter lists).
Run-time Polymorphism (Method Overriding): A subclass can provide a specific implementation for a method that is already defined in its parent class.
Example:
class Animal {
public void sound() {
System.out.println("Animal sound");
}
}
class Cat extends Animal {
// Polymorphism: overriding sound method in Cat class
public void sound() {
System.out.println("Cat meows");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Cat(); // Polymorphism: Animal reference, Cat object
animal.sound(); // Output: Cat meows (run-time polymorphism)
}
}
Explanation:
- The
Cat
class overrides thesound
method ofAnimal
, but when we create an instance ofCat
using anAnimal
reference, theCat
version of the method is executed at runtime.
4. Abstraction
Definition: Abstraction focuses on exposing only the essential features of an object while hiding the unnecessary details. It can be achieved using abstract classes or interfaces in Java.
Example: A
Vehicle
class that only defines general properties and methods, while specific types of vehicles likeCar
orBike
implement the details.
Example:
// Abstract class
abstract class Vehicle {
abstract void start(); // Abstract method (no body)
}
// Concrete class implementing the abstract method
class Car extends Vehicle {
public void start() {
System.out.println("Car starts");
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.start(); // Output: Car starts
}
}
Explanation:
The
Vehicle
class is abstract and defines an abstract methodstart
.The
Car
class provides the actual implementation of thestart
method.This allows hiding the internal details of how the vehicle starts while only exposing the method to users.
3. Classes and Objects in Java
Class:
A class is a blueprint or template that defines the structure and behavior (attributes and methods) of objects.
Example: A
Car
class defines attributes likecolor
,model
, andspeed
, as well as methods likestart()
andstop()
.
Example:
public class Car {
// Attributes
String color;
String model;
int speed;
// Methods
public void start() {
System.out.println("Car is starting");
}
public void stop() {
System.out.println("Car is stopping");
}
}
Object:
An object is an instance of a class. It represents an entity that has state (attributes) and behavior (methods).
We create objects to interact with the methods and data defined in the class.
Example of Creating an Object:
public class Main {
public static void main(String[] args) {
// Creating an object of the Car class
Car myCar = new Car();
myCar.color = "Red";
myCar.model = "Sedan";
myCar.start(); // Output: Car is starting
}
}
4. Advantages of Object-Oriented Programming
Modularity: OOP allows breaking down a program into smaller parts (objects and classes), which makes the code more manageable and modular.
Reusability: Code reuse is possible through inheritance and method overriding, reducing duplication.
Maintainability: Encapsulation ensures that changes within a class do not affect the rest of the program, making code easier to maintain.
Extensibility: Polymorphism and abstraction allow programs to be extended with new functionality without modifying existing code.
Summary
By the end of Day 7, we will have a clear understanding of:
The basic concepts of OOPs (Encapsulation, Inheritance, Polymorphism, and Abstraction).
How to define classes and create objects in Java.
The benefits of using OOPs concepts in Java for writing clean, modular, and reusable code.
Examples to illustrate the concepts of OOPs in action.
This day provides a foundation in OOPs concept, setting the stage for exploring advanced topics such as object design patterns and more in-depth OOPs practices in later stages of the blog series. So Stay tuned!!
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
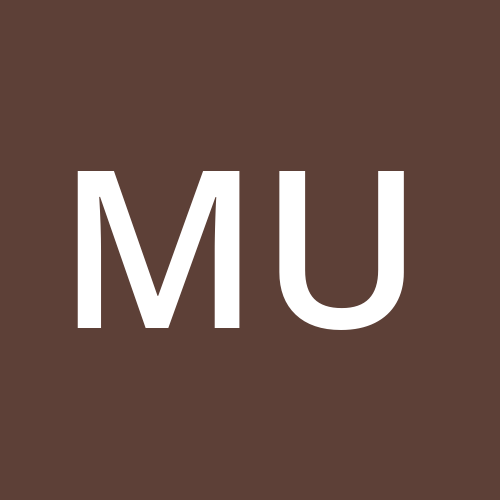