Understanding the Builder Pattern in C#
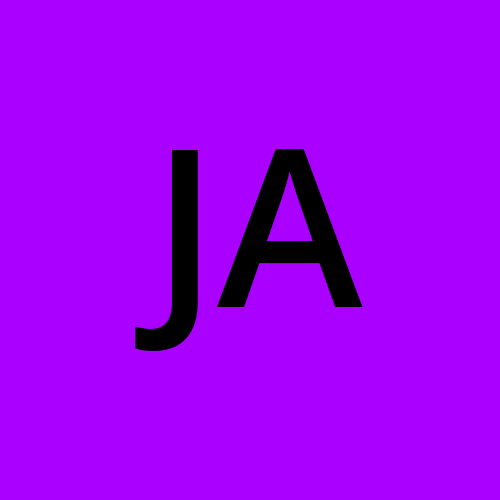
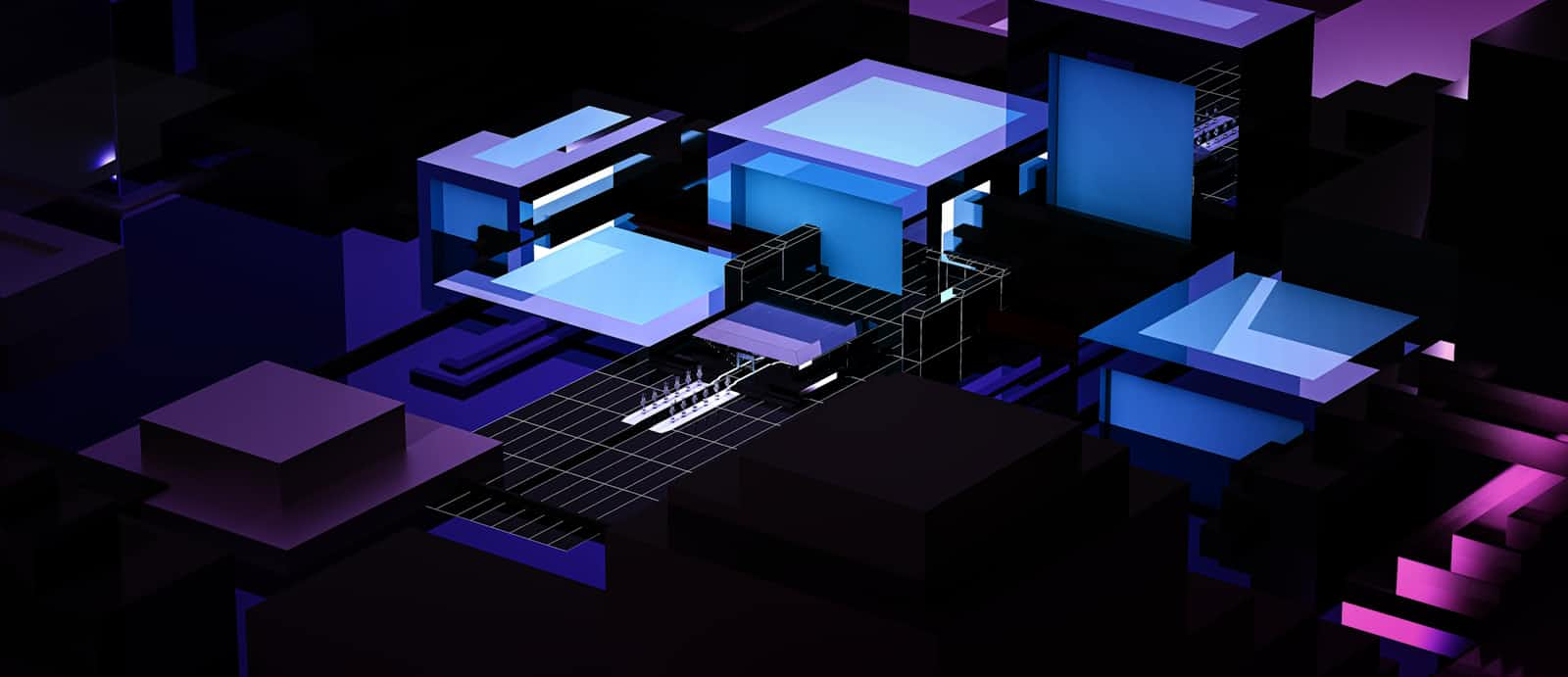
In software development, design patterns are essential for crafting flexible, reusable, and maintainable code. One such pattern is the Builder Pattern, a creational design pattern that simplifies the construction of complex objects. The Builder Pattern is particularly useful when an object has numerous attributes and its construction involves multiple steps. In this blog, we'll dive deep into the Builder Pattern in C#, its use cases, and how to implement it.
What is the Builder Pattern?
The Builder Pattern is a design pattern that separates the construction of a complex object from its representation. This allows the same construction process to create different representations. The Builder pattern is useful when you have:
Complex initialization with multiple steps or parameters.
Multiple variations of the object being created.
A need for immutability.
Instead of a large constructor with multiple parameters or numerous setters that lead to code that is hard to read and maintain, the Builder Pattern helps manage this complexity.
Key Components of the Builder Pattern
Builder: This is the interface or abstract class that defines methods for constructing different parts of the complex object.
Concrete Builder: This implements the
Builder
interface, creating specific components of the object.Product: This is the complex object that is being constructed.
Director: The director controls the construction process, ensuring that the steps are followed in the correct order (this component is optional in some implementations).
When to Use the Builder Pattern?
When an object requires a large number of parameters, especially if some of them are optional.
When the order of setting properties is important.
When object construction is complex or requires multi-step processes.
When you want to build multiple variations of an object.
Example: Implementing the Builder Pattern in C
Let's consider an example of building a Car object, which has numerous optional properties.
Step 1: Define the Product
The product is the final object that we want to build:
public class Car
{
public string Engine { get; set; }
public int Seats { get; set; }
public string Color { get; set; }
public bool HasAirConditioning { get; set; }
public override string ToString()
{
return $"Car [Engine: {Engine}, Seats: {Seats}, Color: {Color}, Air Conditioning: {HasAirConditioning}]";
}
}
Step 2: Create the Builder Interface
The ICarBuilder
interface defines the steps required to build the car:
public interface ICarBuilder
{
ICarBuilder SetEngine(string engine);
ICarBuilder SetSeats(int seats);
ICarBuilder SetColor(string color);
ICarBuilder SetAirConditioning(bool hasAirConditioning);
Car Build();
}
Step 3: Implement the Concrete Builder
The CarBuilder
class implements the ICarBuilder
interface and provides the logic for constructing different parts of the car:
public class CarBuilder : ICarBuilder
{
private Car _car = new Car();
public ICarBuilder SetEngine(string engine)
{
_car.Engine = engine;
return this;
}
public ICarBuilder SetSeats(int seats)
{
_car.Seats = seats;
return this;
}
public ICarBuilder SetColor(string color)
{
_car.Color = color;
return this;
}
public ICarBuilder SetAirConditioning(bool hasAirConditioning)
{
_car.HasAirConditioning = hasAirConditioning;
return this;
}
public Car Build()
{
return _car;
}
}
Step 4: Use the Builder to Construct Objects
Now that we have everything in place, we can use the CarBuilder
to construct a car:
class Program
{
static void Main(string[] args)
{
CarBuilder builder = new CarBuilder();
Car car = builder
.SetEngine("V8")
.SetSeats(4)
.SetColor("Red")
.SetAirConditioning(true)
.Build();
Console.WriteLine(car.ToString());
}
}
Output:
Car [Engine: V8, Seats: 4, Color: Red, Air Conditioning: True]
Step 5: Director (Optional)
In some cases, you may want to have a Director
class that defines the order of construction. While this isn't mandatory, it can be helpful for managing complex construction logic.
public class CarDirector
{
private readonly ICarBuilder _builder;
public CarDirector(ICarBuilder builder)
{
_builder = builder;
}
public Car ConstructSportsCar()
{
return _builder
.SetEngine("V12")
.SetSeats(2)
.SetColor("Black")
.SetAirConditioning(true)
.Build();
}
public Car ConstructSUV()
{
return _builder
.SetEngine("V6")
.SetSeats(7)
.SetColor("White")
.SetAirConditioning(true)
.Build();
}
}
Advantages of the Builder Pattern
Separation of Concerns: The Builder Pattern separates the construction of an object from its representation, making the code cleaner and easier to understand.
Immutable Objects: You can easily make the object immutable by not exposing its setters and only allowing modification via the builder.
Code Readability: The pattern leads to more readable and maintainable code by avoiding telescoping constructors or large parameter lists.
Flexibility: You can create different representations of the same object (e.g., a sports car vs. an SUV) by reusing the same building process.
Disadvantages of the Builder Pattern
Overhead: It introduces additional classes and complexity, which might not be justified for simpler objects.
Verbose Code: It can lead to verbose code if not used appropriately, especially when overused for small or straightforward objects.
Conclusion
The Builder Pattern is an excellent way to deal with complex object construction. In C#, it helps make your code more maintainable, readable, and flexible, especially when working with immutable objects or objects with many optional parameters. However, as with any pattern, it should be used judiciously to avoid unnecessary complexity.
By using the Builder Pattern, you can ensure that your code remains clean, flexible, and easy to work with, even as your project scales and becomes more complex.
Happy coding!
Subscribe to my newsletter
Read articles from Joshua Akosa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
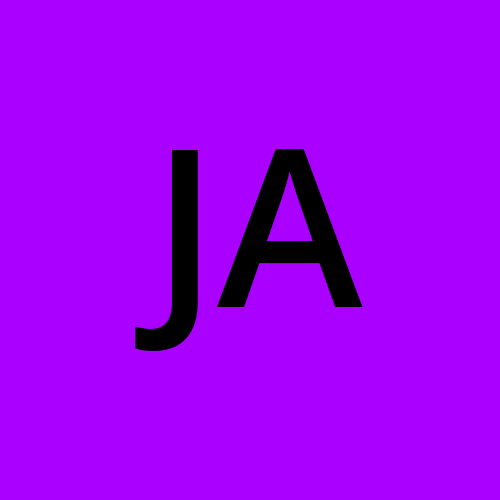