SCIM User Provisioning: Streamlining Identity Management with Python
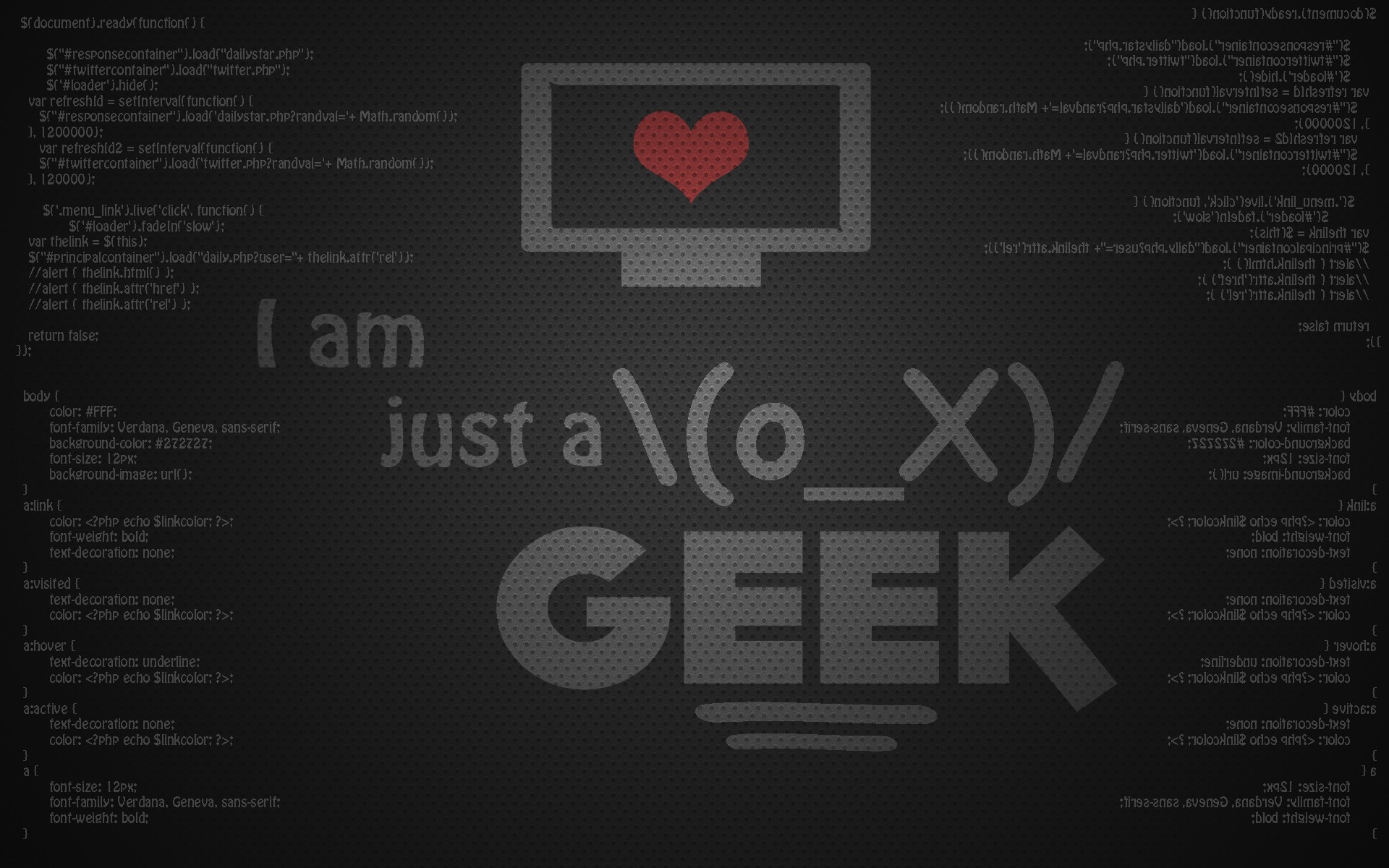
SCIM User Provisioning: Simplify Identity Management with Python
Meta Description: Learn how to implement SCIM user provisioning using Python. Streamline your identity management across Azure AD, Okta, and Google Cloud with step-by-step examples and best practices.
Introduction
In today's cloud-centric world, managing user identities across multiple systems can be a daunting task. Enter SCIM (System for Cross-domain Identity Management) – a game-changer in user provisioning. This blog post will dive deep into SCIM, showing you how to leverage Python to simplify your identity management processes.
What is SCIM and Why Does it Matter?
SCIM is an open standard designed to automate the exchange of user identity information between systems. Here's why it's crucial:
Efficiency: Automates user provisioning and deprovisioning
Consistency: Ensures uniform user data across platforms
Security: Reduces manual errors and improves access control
SCIM in Popular Cloud Active Directories
Let's explore how SCIM is implemented in three major cloud platforms:
1. Microsoft Azure AD
Azure AD supports SCIM 2.0 for seamless user synchronization. Key features include:
Automatic user provisioning
Group management
Real-time updates
2. Okta
Okta's SCIM implementation offers:
Lifecycle management
Just-in-time provisioning
Attribute mapping
3. Google Cloud Identity
Google Cloud Identity leverages SCIM for:
User and group synchronization
Custom schema support
Automated account reconciliation
Implementing SCIM with Python: A Step-by-Step Guide
Now, let's get our hands dirty with some Python code. We'll use the python-scim
library for our examples.
Setting Up
First, install the necessary library:
pip install python-scim
Creating a SCIM Client
Here's how to set up a basic SCIM client:
from scim import SCIMClient
client = SCIMClient(
base_url="https://your-scim-endpoint.com",
auth_token="your_auth_token_here"
)
Creating a User
Let's create a new user:
new_user = {
"schemas": ["urn:ietf:params:scim:schemas:core:2.0:User"],
"userName": "johndoe@example.com",
"name": {
"givenName": "John",
"familyName": "Doe"
},
"emails": [
{
"value": "johndoe@example.com",
"type": "work",
"primary": True
}
]
}
created_user = client.create_user(new_user)
print(f"User created with ID: {created_user['id']}")
Reading User Information
Retrieve user details:
user_id = created_user['id']
user_info = client.get_user(user_id)
print(f"User details: {user_info}")
Updating User Attributes
Update user information:
update_data = {
"name": {
"givenName": "Johnny"
}
}
updated_user = client.update_user(user_id, update_data)
print(f"Updated user: {updated_user}")
Deprovisioning a User
Remove a user from the system:
client.delete_user(user_id)
print(f"User {user_id} has been deprovisioned")
Best Practices and Security Considerations
When implementing SCIM with Python, keep these best practices in mind:
Secure your endpoints: Use HTTPS and proper authentication
Manage API tokens carefully: Rotate tokens regularly and use secure storage
Implement proper error handling: Gracefully manage API errors and rate limits
Audit and log operations: Maintain a detailed log of all SCIM operations for troubleshooting and compliance
Conclusion
SCIM user provisioning with Python offers a powerful solution for managing identities across cloud platforms. By leveraging SCIM with Azure AD, Okta, or Google Cloud Identity, you can streamline your user management processes, enhance security, and improve efficiency.
As identity management continues to evolve, SCIM will play an increasingly important role in maintaining a secure and efficient multi-cloud environment. Start implementing SCIM with Python today, and take your identity management to the next level!
Additional Resources
Remember to stay updated with the latest SCIM developments and Python libraries to ensure you're always using the best tools for your identity management needs.
Subscribe to my newsletter
Read articles from Tech Shots(RoamPals) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
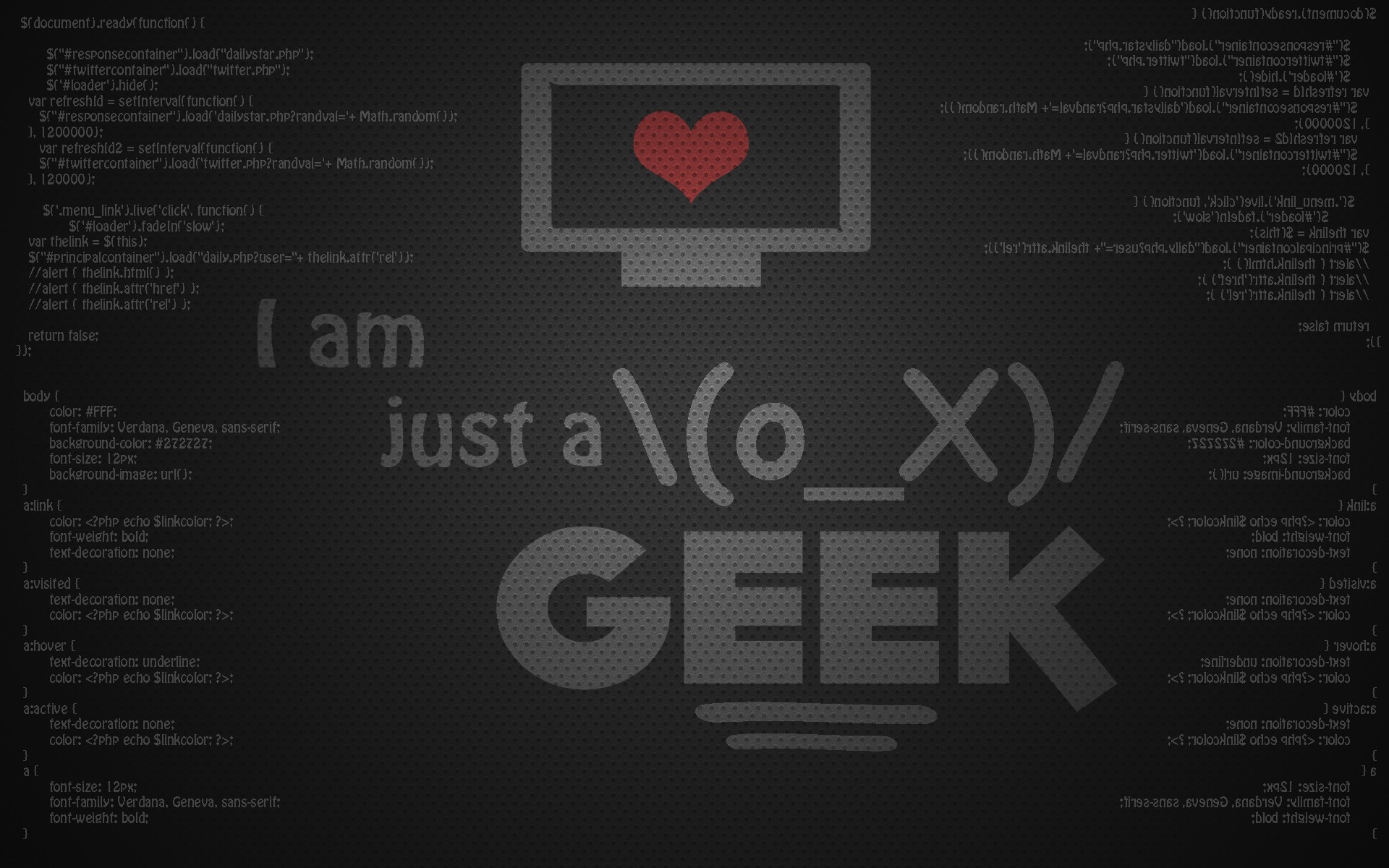