Linux Shell Scripting for Beginners: A Simple Guide

Table of contents
- What is Linux Shell Scripting?
- Why Shell Scripting?
- Fundamentals
- Basic Linux Commands
- Shebang
- Variables and Data Types in Shell Scripts
- Conditional Statements and Loops
- Functions and User Input
- Working with Files and Directories
- Text Processing and Manipulation
- Command Line Arguments and Options
- Error Handling and Debugging
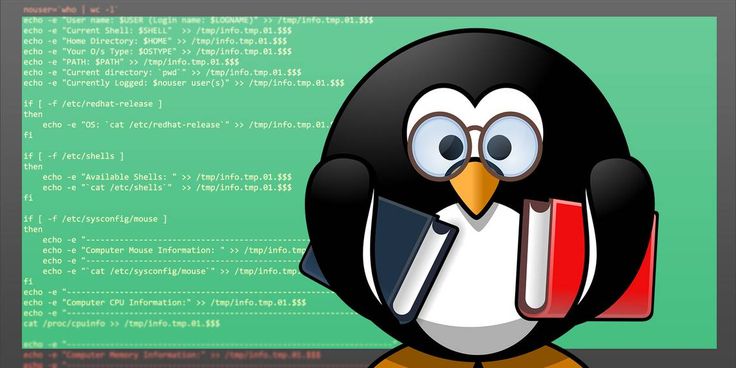
What is Linux Shell Scripting?
Linux shell scripting refers to the process of writing scripts or programs to automate tasks and execute commands on a Linux system through the command line interface, called the shell. The shell is a mediator between the user and the Operating system, it allows users to interact with the system by executing commands and running programs.
Why Shell Scripting?
Shell scripting is a powerful tool in the world of Unix/Linux systems, designed to streamline and automate routine tasks. Tasks like managing servers, automating backups, or simply want to execute a series of commands without manual intervention, shell scripts offer an efficient way to do it all.
By writing scripts that combine various commands, you can reduce the complexity of repetitive tasks, save time, and ensure consistency in your operations. In this blog, we’ll explore the fundamentals of shell scripting.
Fundamentals
Before going into shell, you must have a good knowledge on command line interface (CLI) it is a text based interface which allows us to communicate with the system through text commands, you must have an idea on these basic commands to get started
mkdir
: Create a new directory.cd
: Changes the current directory.ls
: List files and directories in the current directory.touch
: Used to create a filerm
: Remove files and directories.cat
: Display the contents of a file.
Every command has a set of flags which specify the suboperations done through these commands, you can find those in the reference manual, to access the reference manual, you can use the man
command followed by the required command
for example
man mkdir
it shows you the manual page for mkdir
command
Basic Linux Commands
Shell scripting often involves using basic Linux commands. Here are some commands you'll frequently use in your scripts:
echo
: This command displays messages or the values of variables. For example,echo "Hello, world!"
will print "Hello, world!" to the terminal.grep
: Use this command to search for specific patterns in files. For instance,grep "text" filename
finds occurrences of "text" infilename
.awk
: This tool processes and extracts data from text files. For example,awk '{print $1}' file.txt
prints the first column offile.txt
.cut
: Extract specific columns from text. For example,cut -d':' -f1 file.txt
extracts the first column fromfile.txt
, using:
as a delimiter.find
: Search for files and directories. For instance,find /path -name "file.txt"
searches forfile.txt
in/path
.chmod
: Change file permissions. For example,chmod 755 script.sh
sets the permissions ofscript.sh
to be readable and executable.chown
: Change file ownership. For example,chown user:group file.txt
changes the owner offile.txt
touser
and the group togroup
.sed
: A stream editor for text manipulation. For instance,sed 's/old/new/g' file.txt
replaces "old" with "new" infile.txt
.sort
: Sort lines of text. For example,sort file.txt
sorts the lines infile.txt
.
Shebang
Shebang (#!
): The shebang
line at the top of a script specifies the type of interpreter to be used, such as
#!/bin/bash
This line tells the system to use the Bash shell to interpret the script. Bash (Bourne Again Shell)
#!/bin/ksh
This line specifies the Korn Shell (ksh) as the interpreter
#!/bin/sh
This line indicates that the script should be executed using the Bourne Shell (sh).
#!/bin/dash
This line tells the system to use the Debian Almquist Shell (dash).
Variables and Data Types in Shell Scripts
Variables in shell scripting are used to store data. Unlike other programming languages, shell scripting doesn't have specific data types. You can store text, numbers, or command outputs. Here’s an example of how to define and use variables:
name="Bharadwaj reddy"
age=20
echo "My name is $name and I am $age years old."
Conditional Statements and Loops
Conditional statements and loops let you make decisions and repeat actions in your scripts:
If Statement: Executes code if a condition is true. For example:
if [ $age -ge 18 ]; then echo "You are an adult." fi
For Loop: Repeats actions for each item in a list. For example:
for file in *.txt; do echo "Processing $file" done
While Loop: Repeats actions while a condition is true. For example:
while [ $count -lt 10 ]; do echo "Count is $count" count=$((count + 1)) done
Functions and User Input
Functions help organize your code into reusable blocks, and user input can make scripts interactive:
#!/bin/bash
greet() {
echo "Hello, $1!"
}
# Prompt for user input
read -p "Enter your name: " name
# Call the function
greet "$name"
Working with Files and Directories
Shell scripting allows you to manage files and directories programmatically:
Creating a Directory:
mkdir new_folder
Creating an Empty File:
touch new_file.txt
Copying a File:
cp source.txt destination.txt
Moving or Renaming a File:
mv old_name.txt new_name.txt
Deleting a File:
rm file_to_delete.txt
Deleting a Directory:
rm -r directory_to_delete
Text Processing and Manipulation
Manipulate text files with powerful tools:
grep
: Search for text patterns.sed
: Replace text in files.awk
: Process and extract data.cut
: Extract columns from text.sort
: Sort lines of text.
Command Line Arguments and Options
Scripts can accept arguments to customize their behavior. Here’s an example:
#!/bin/bash
# Usage: ./script.sh <name> <age>
name=$1
age=$2
echo "My name is $name and I am $age years old."
Error Handling and Debugging
Handling errors and debugging are crucial for writing reliable scripts:
Exit Codes: Use exit codes to indicate success or failure.
Error Messages: Display meaningful messages to users.
Debugging Mode: Enable with
-x
to trace script execution. For example,bash -x script.sh
.Tracing: Add
set -x
at the beginning of your script to trace commands.
This week, I've delved into the basics of shell scripting, covering essential commands, variable handling, conditional statements, loops, functions, file operations, text processing, and error handling. I hope this guide helps you get started with writing your own shell scripts.
In future blogs, I’ll be exploring advanced shell scripting techniques and how to integrate AWS with shell scripting. Stay tuned for more in-depth content!
For a reference to the concepts covered, check out my GitHub repository: Linux shell scripting basics
Happy scripting! 😎✌️♾️
Subscribe to my newsletter
Read articles from Bharadwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bharadwaj Reddy
Bharadwaj Reddy
Hey there! I’m Bharadwaj Reddy, currently an undergrad at Keshav Memorial Institute of Technology. I’m a full stack developer who loves building cool web applications with React, Node.js, and MongoDB. On top of that, I also love exploring machine learning with TensorFlow and PyTorch. I’m pretty comfortable navigating GitHub for version control and love tinkering with AWS for deploying projects. I post my regular learnings on Devops and other studies